You can switch to another branch in Git by using the `git checkout` command followed by the branch name you want to switch to.
git checkout branch-name
Understanding `git checkout`
What is `git checkout`?
`git checkout` is a versatile command used in Git that allows you to switch between different branches or restore working tree files. This command is essential for navigating your version control system as it enables developers to manage multiple lines of development seamlessly. Beyond simply moving between branches, `git checkout` can also be used to pull specific files from different commits or branches, making it a powerful tool in your Git toolkit.
When to Use `git checkout`
Understanding when to use `git checkout` is crucial:
- Moving between branches: When you want to shift your work from one feature branch to another.
- Checking out files from another branch: If you need a specific file version from a different branch without merging or switching branches.
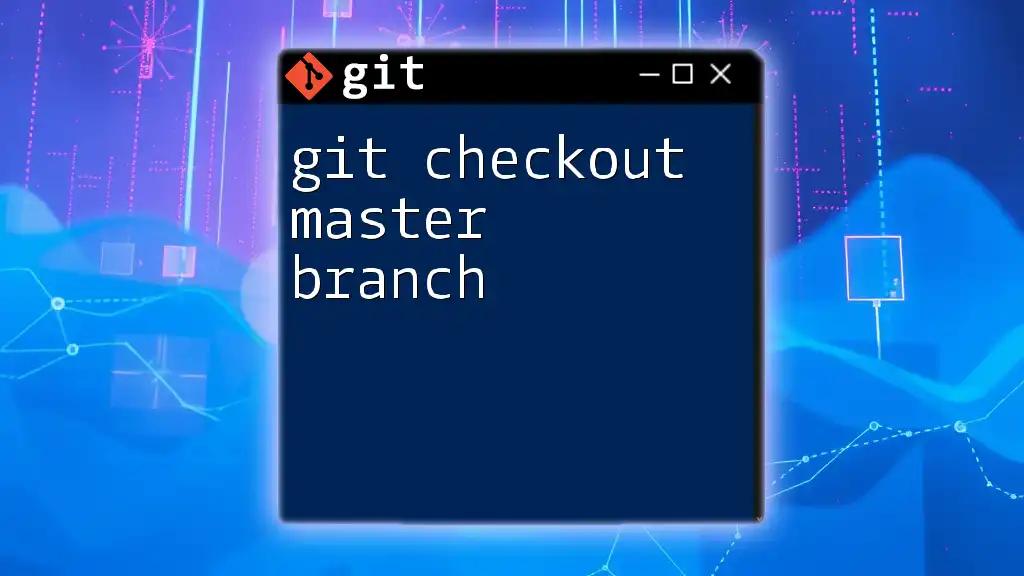
The Basics of Branching in Git
What is a Branch?
In Git, a branch is essentially a pointer to a specific commit in your version history. Think of branches as parallel lines of development that allow you to work on different features or fixes independently. This means you can develop new features without affecting the main codebase, fostering a more organized and manageable approach to coding.
Creating and Viewing Branches
Before using `git checkout`, you first need to get familiar with creating and viewing branches. You can create a new branch using:
git branch new-feature
To view your existing branches, simply run:
git branch
This will show you a list of all branches in your repository, helping you keep track of your development process.
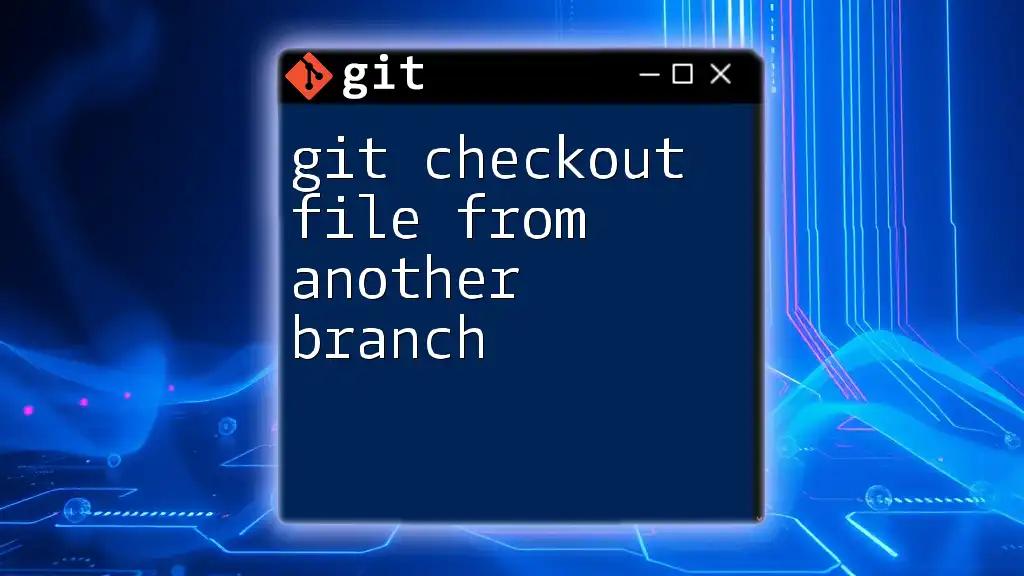
Checkout Another Branch
Command Syntax
The basic syntax of `git checkout` is straightforward:
git checkout <branch_name>
This command tells Git to switch your working directory to the specified branch.
Checking Out an Existing Branch
When you're ready to switch to another branch, follow these steps:
-
Ensure the working directory is clean. Before changing branches, make sure you have committed or stashed any changes to avoid losing work.
-
Use the command: To check out an existing branch, simply provide its name in the command. For example:
git checkout develop
After executing this command, your working directory will reflect the state of the `develop` branch.
Understanding Detached HEAD
When you check out a specific commit or branch that is not the current branch you’re on, you may encounter a Detached HEAD state. This occurs when you are no longer working on a branch but rather on a specific commit.
- What is a Detached HEAD? Simply put, it means you are working in a state where you are not on the tip of any branch.
- When does it occur? This typically happens when you check out a commit hash directly.
- How to recover from a detached HEAD state: To return to your previous branch, you can run:
git checkout master
This will bring you back to the `master` branch, where you can continue with your development.
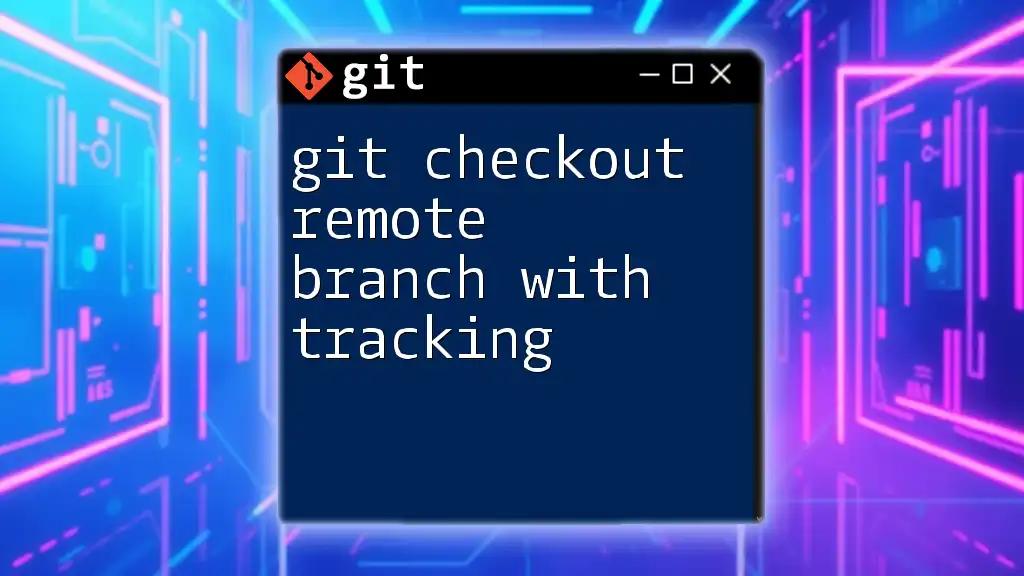
Checking Out Specific Commits
Using `git checkout` with Commit Hash
Aside from switching between branches, `git checkout` can be used to access a specific commit using its hash.
For example, if you have a particular commit that you want to review or revert changes from, use:
git checkout 1a2b3c4
Here, `1a2b3c4` represents the unique identifier of the commit you wish to check out.
Reverting Back to Latest State
After examining a previous commit, you may want to return to the latest state of your branch. To do this, simply run:
git checkout master
This action restores you to the top of the `master` branch, keeping your workflow uninterrupted.
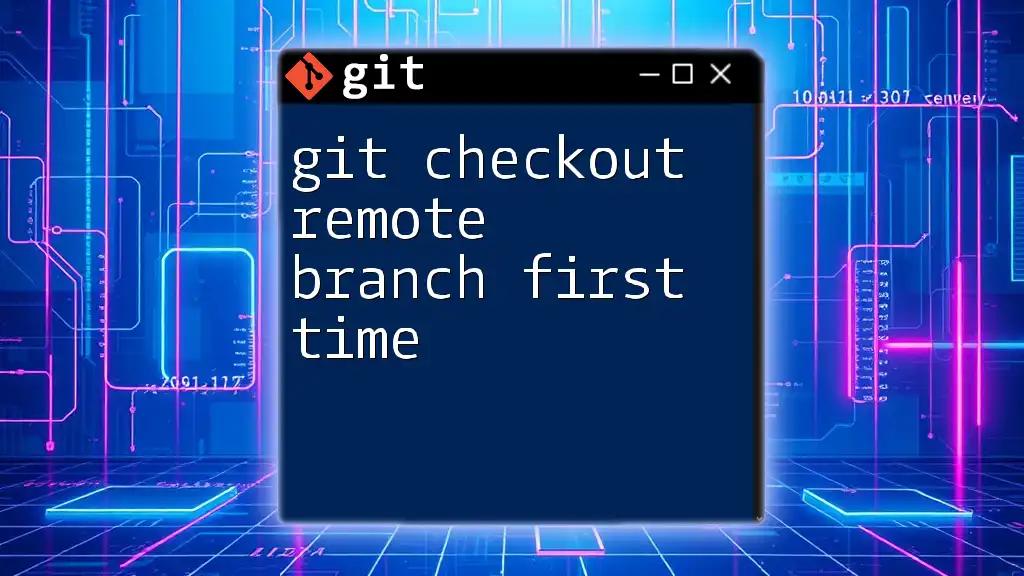
Checking Out Files or Directories from Another Branch
Command Syntax
You can also check out individual files from another branch using the following syntax:
git checkout <branch_name> -- <file_path>
This command allows you to grab a specific file without fully switching branches.
Use Cases
Checking out files can come in handy in various scenarios, such as:
- Retrieving a specific file version: If you need a file as it existed in another branch without merging it, this command allows for quick access.
- Undoing changes from a recent commit: If you accidentally modified a file and need the version that exists on another branch, simply check it out.
Code Example
For instance, if you want to retrieve `file.txt` from `feature-branch`, you would use:
git checkout feature-branch -- path/to/file.txt
This replaces your current working file with the specified version from the other branch, making it an efficient way to gain changes without affecting branch histories.
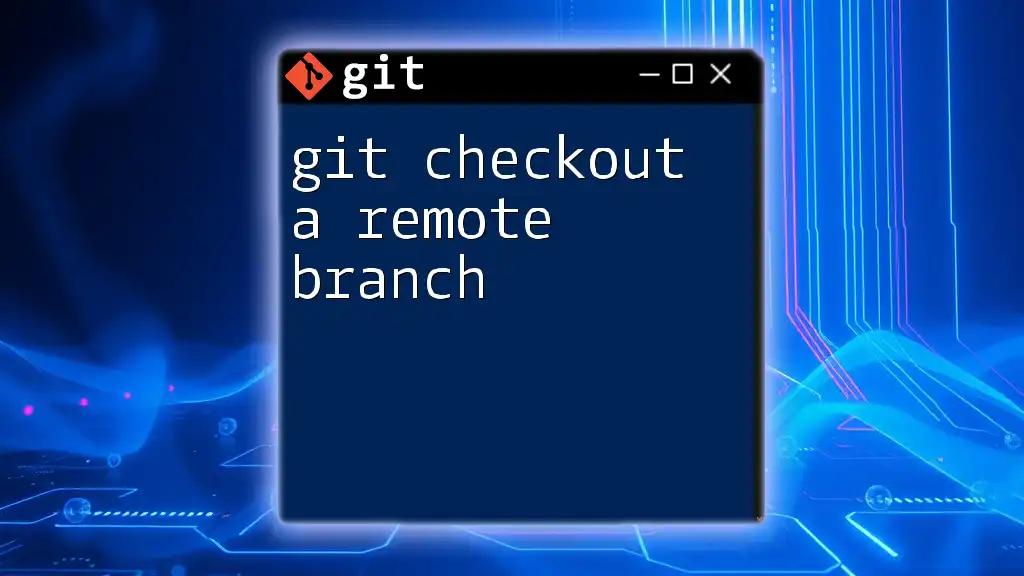
Best Practices for Using `git checkout`
When to Checkout Instead of Merge or Rebase
Use `git checkout` when switching contexts or when you want to examine the state of different branches without integrating changes. In contrast, merging or rebasing is better suited for when you want to combine changes from one branch into another.
Keeping Your Branches Organized
- Organize and regularly clean up inactive branches to avoid clutter.
- Use descriptive names for branches related to the feature or fix you are working on, which can help in collaboration and understanding your project structure.
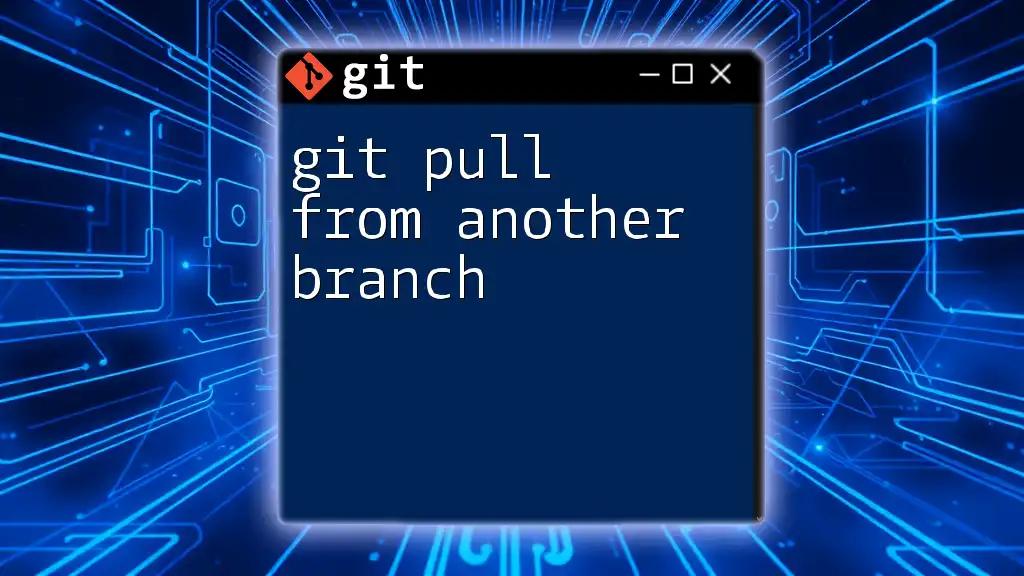
Troubleshooting `git checkout` Issues
Common Problems and Solutions
Sometimes you might run into issues while using `git checkout`. Here are a couple of common errors and how to resolve them:
-
Error: "Your local changes to the following files would be overwritten"
- This means you have uncommitted changes in your working directory that conflict with the branch you are trying to switch to. To resolve this, you can either commit or stash your changes first.
-
Error: "pathspec '...' did not match any file(s) known to git"
- This error indicates that you are trying to check out a file or branch that does not exist. Ensure you have spelled everything correctly and that the branch or file exists.
Useful Resources for Further Learning
For more in-depth learning, consult the official [Git documentation](https://git-scm.com/doc) and explore recommended tutorials that can deepen your understanding of commands and overall best practices.
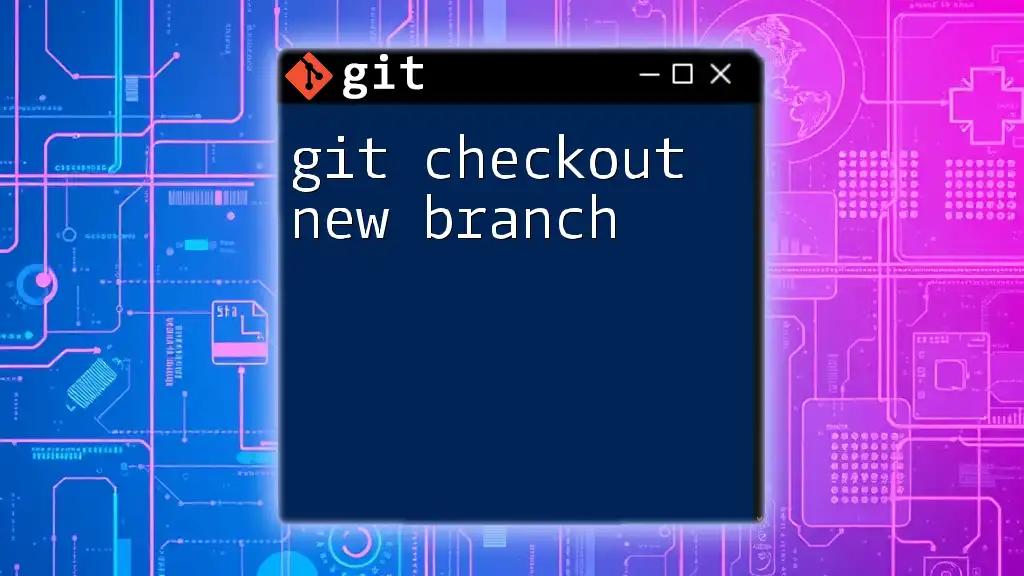
Conclusion
In summary, mastering `git checkout from another branch` is crucial for any developer who aims to work efficiently in a collaborative environment. By understanding how to switch between branches, revert to specific commits, and manage files across different branches, you position yourself to handle code smoothly and effectively.
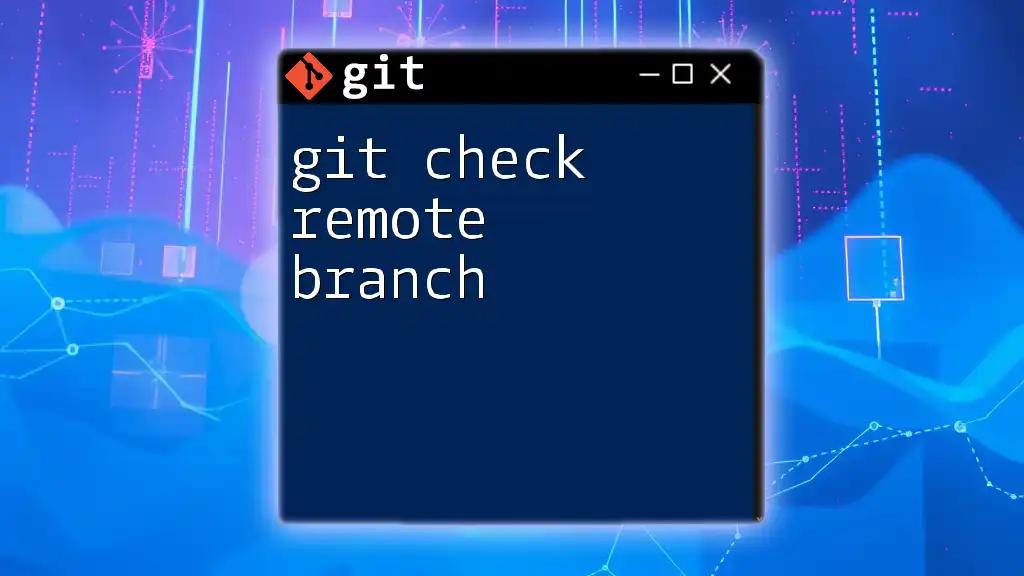
Call to Action
Join our community today and enhance your Git skills! Stay tuned for more tutorials and upcoming workshops that will take your understanding of version control to the next level.