To checkout a specific directory from another branch in Git without switching the entire branch, you can use the following command:
git checkout other-branch -- path/to/directory
Understanding Git Branching
What is a Git Branch?
A Git branch is essentially a pointer to a specific commit in your project’s history. Branching allows you to work on multiple features or versions of a project simultaneously without affecting the main codebase. This is particularly useful in collaborative environments, where different developers might be working on separate features. Common branching strategies include using feature branches for new developments, release branches for preparing production releases, or hotfix branches for urgent changes.
When to Use `git checkout`
The `git checkout` command is versatile and is primarily used for switching branches, restoring files, and checking out specific commits or tags. One of its significant uses is the ability to retrieve a directory from another branch. This can be especially beneficial when undergoing code reviews or when you need to merge specific changes without switching entire branches.
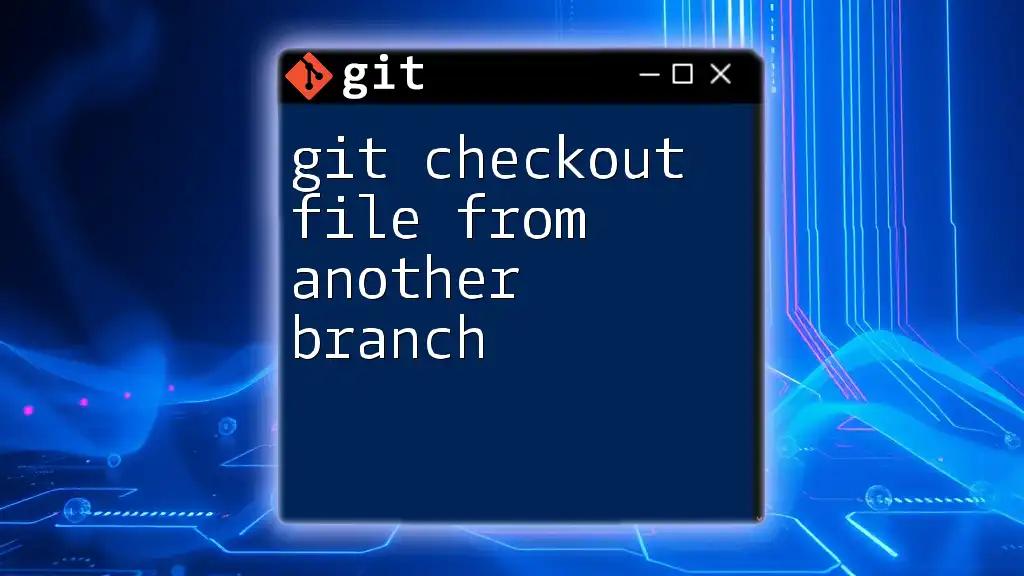
Using `git checkout` to Retrieve a Directory
Basic Syntax of `git checkout`
To check out a directory from another branch, you can use the following command structure:
git checkout [branch-name] -- [path/to/directory]
This syntax allows you to specify both the branch you want to check out from and the directory you wish to retrieve, ensuring that you only bring in the necessary files.
Checking Out a Specific Directory
Step-by-Step Process
-
Identify the Directory to Checkout: Before executing the `git checkout` command, identify the exact path of the directory you want to retrieve. Knowing the directory structure will prevent errors and ensure you get the right files.
-
Switching to Your Current Branch: Before checking out a directory, make sure you are on the branch where you want the changes to appear. You can do this by executing:
git checkout [current-branch]
-
Executing the Checkout Command: Once you’re on the correct branch, run the command to check out the desired directory from the other branch. For example:
git checkout feature-branch -- src/components
This command pulls the `src/components` directory from the `feature-branch` into your current branch. After executing this command, the files from the specified directory will be copied, leaving the rest of your working directory unaffected.
Verifying Changes
After checking out a directory, it’s crucial to verify that your changes have been applied correctly. Use the following command to check the status of your working directory:
git status
This command will display any modified files and confirm whether the checkout was successful. Expect to see the newly retrieved files listed as changes in your current branch.
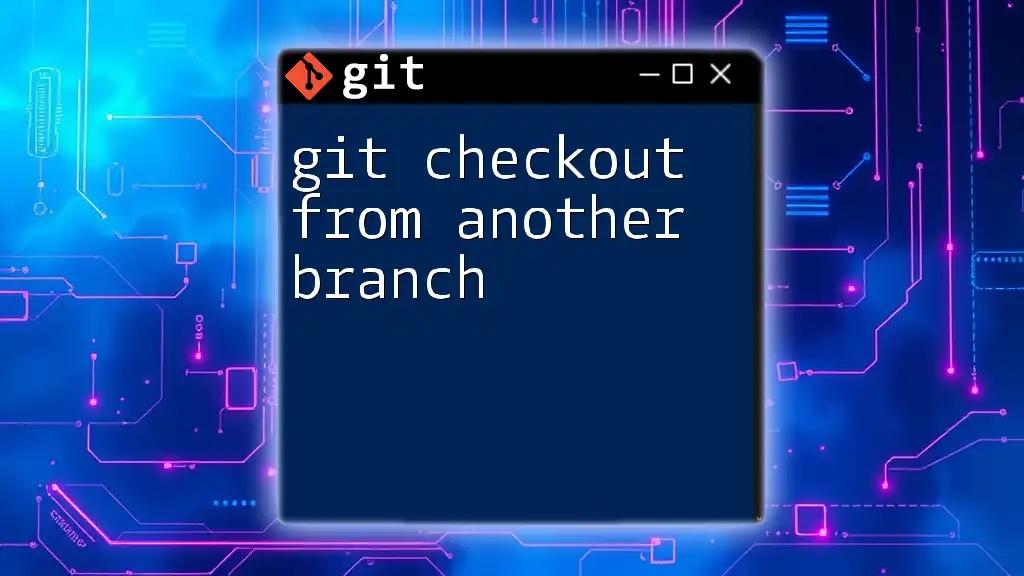
Potential Issues and Troubleshooting
Conflicts During Checkout
Conflicts can arise when you try to check out a directory that contains files already modified in your working directory. If there are conflicting changes, Git will alert you. To resolve conflicts, follow these steps:
-
Inspect the conflicting changes by running:
git diff
This will help you understand the differences between your current state and what you are trying to retrieve.
-
Once you identify the conflicts, resolve them manually by editing the affected files in your text editor.
-
After resolving conflicts, stage the changes using:
git add [path/to/file]
-
Finally, commit the changes to your branch:
git commit -m "Resolved conflicts while checking out directory"
Understanding the Impact of Checkout on the Working Directory
Use caution when executing the `git checkout` command, as it affects your working directory. If you have uncommitted changes, you risk losing those changes upon checking out another directory. Always ensure to commit or stash your changes before proceeding with a checkout.
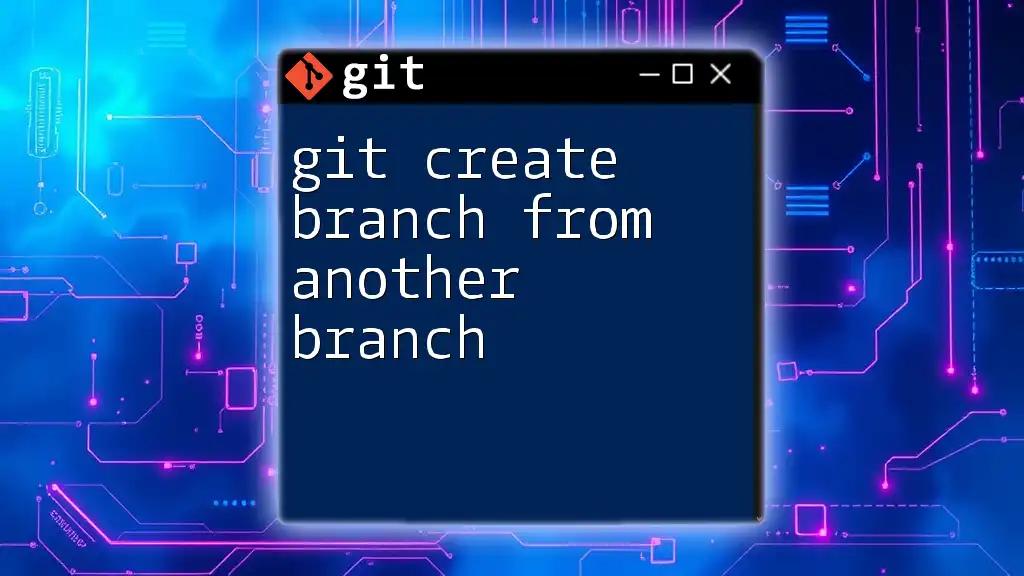
Best Practices for Using git checkout
Commit and Stash Changes Before Checkout
Before performing a `git checkout`, it’s best practice to either commit your changes or use Git's stashing feature to save your current modifications temporarily. This step is crucial to avoid losing any work. You have two options:
To commit changes:
git add .
git commit -m "Save changes"
Or you can stash your changes using:
git stash
This way, when you proceed with the checkout, your working directory remains clean, minimizing the risk of conflicts.
Using Tags as Alternatives to Checkout
Another effective strategy is to use tags. Tags are useful when you want to check out a versioned snapshot of your codebase without affecting your branch structure. You can execute the following command to check out a directory from a specified tag:
git checkout tags/v1.0 -- src/components
This method ensures that you retrieve the directory as it existed at a particular version, which can be helpful during backtracking or when working with specific release versions.

Conclusion
Mastering the `git checkout directory from another branch` command can significantly enhance your Git workflow. Knowing how to effectively retrieve directories allows for efficient code review processes and smooth feature integrations. As you familiarize yourself with these commands, your ability to manage changes in collaborative environments will improve dramatically.
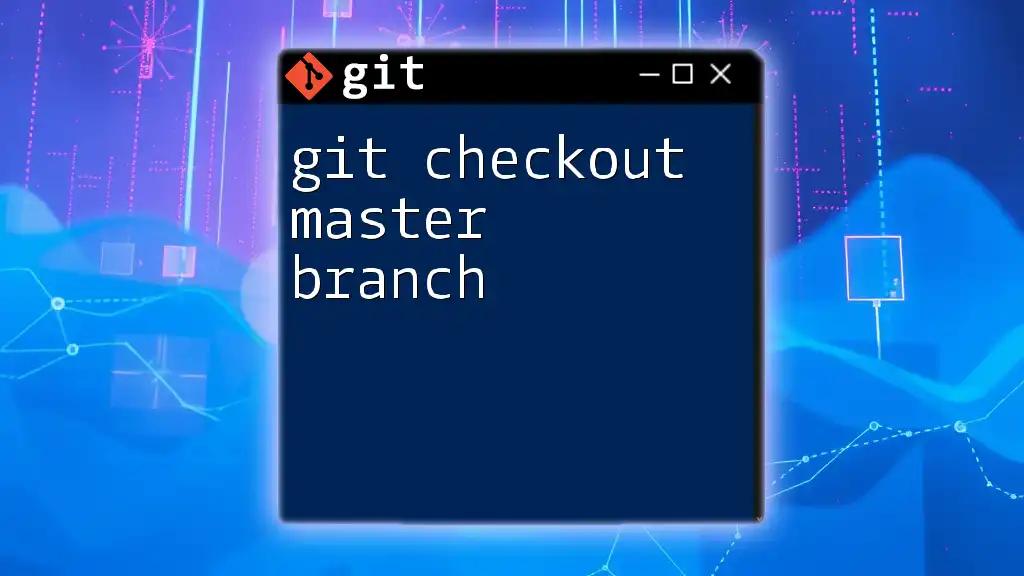
Call to Action
Feel free to comment below if you have questions or need further clarification. Also, don't forget to subscribe for more Git tutorials tailored to enhance your skills. Stay tuned for our next article on “Mastering Merging: Strategies for Combining Branches Effectively.”

Additional Resources
To continue your Git journey, explore these additional resources:
- Links to official Git documentation for deeper insights into functionality.
- Suggested video tutorials and courses that offer practical applications.
- Information on our upcoming webinars or workshops aimed at enhancing your Git knowledge.