To exclude a directory from being tracked by Git, add the directory name to the `.gitignore` file in your repository's root directory.
Here's how you can do this with a code snippet:
echo 'your-directory-name/' >> .gitignore
Understanding `.gitignore`
What is `.gitignore`?
The `.gitignore` file is a powerful tool within Git that allows you to specify which files or directories should be ignored by the version control system. This means that any files or directories defined in `.gitignore` will not be tracked by Git, helping to keep your repository clean and free of unnecessary clutter. By excluding certain items, you can also prevent sensitive information, such as credentials or API keys, from being accidentally committed to your repository.
Creating a `.gitignore` File
Creating a `.gitignore` file is straightforward. To create a new `.gitignore`, open your terminal and navigate to the root of your project. You can create the file using the following command:
touch .gitignore
It’s essential to place the `.gitignore` file at the root of your Git repository for it to work effectively. This location ensures that the ignore rules apply to the entire project.
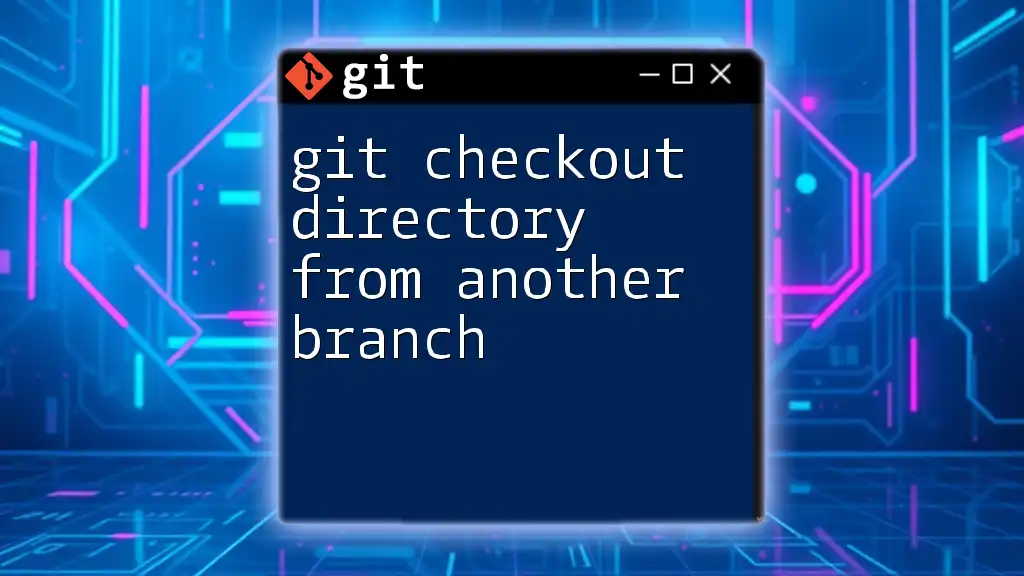
How to Exclude a Directory
Basic Syntax of `.gitignore`
The syntax of the `.gitignore` file is quite permissive, allowing you to specify patterns of files and directories to ignore. To ignore a specific directory, you simply write the directory's name followed by a trailing slash. For example, to ignore a directory named “logs,” you would add the following line to your `.gitignore`:
logs/
If you want to ignore a directory regardless of its location, you can use an asterisk as a wildcard. This is particularly useful when you need to ignore directories with common names that may exist in different parts of your project.
Multiple Directory Exclusions
It is also possible to exclude multiple directories in a single `.gitignore` file. Each directory should be listed on a new line. For instance, if you want to ignore several directories such as `node_modules`, `temp`, and `Logs`, your `.gitignore` would look like this:
/node_modules/
/temp/
/Logs/
This entry ensures that any directory matching these names will be effectively ignored by Git.
Using Wildcards to Exclude Directories
For broader matches, wildcards are incredibly useful. To ignore every directory starting with the word "temp," you can use the following syntax:
temp*/
This entry will ignore any directories that begin with "temp," such as `temp1`, `temp_data`, and so forth, while tracking any files or directories that do not meet the pattern.
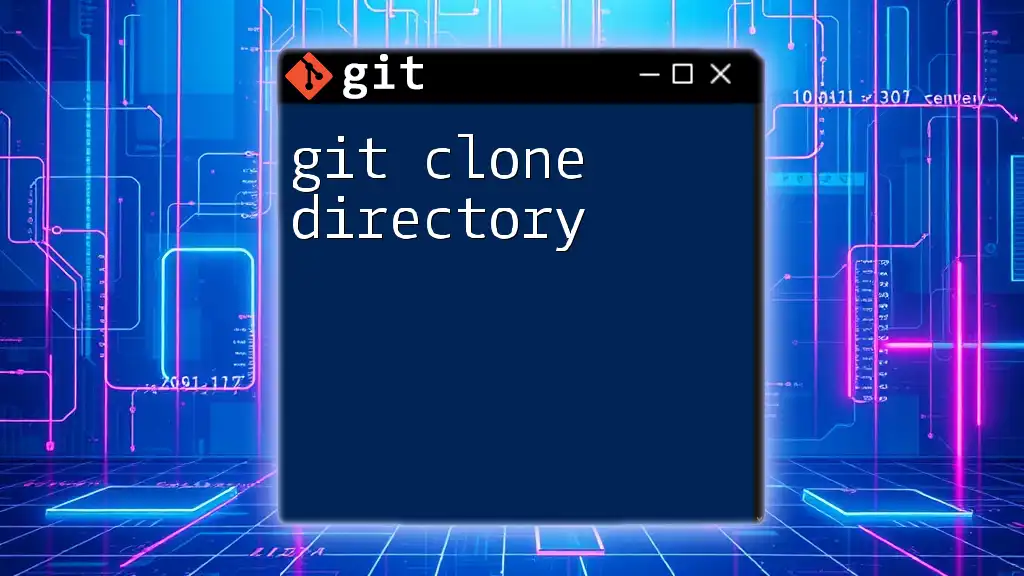
Excluding Previously Tracked Directories
Understanding Tracking in Git
It is crucial to understand that files or directories which have already been tracked by Git will not be ignored just by adding them to the `.gitignore`. This is because Git continues to track changes to files already included in the repository until explicitly told to stop.
Steps to Stop Tracking a Directory
To stop tracking a directory that has already been added to your repository, you can follow these steps:
-
Remove the directory from tracking: To do this, use the `git rm --cached` command. This command removes the specified directory from the index but keeps it on your local file system.
git rm -r --cached directory_name/
-
Re-commit the changes: After the removal, you’ll need to commit the changes to finalize the update.
git commit -m "Stop tracking directory_name"
Following these steps will ensure that Git no longer tracks the specified directory, while the directory remains on your machine.
Verify Exclusions
After making changes to your `.gitignore` and the tracked status of your directory, it’s crucial to verify that the exclusions are working correctly. You can check the status of files and directories tracked by Git with:
git status
Additionally, to verify if a specific file or directory is being ignored, you can use:
git check-ignore -v directory_name/file.txt
This command will display which rule in your `.gitignore` is causing the specified file or directory to be ignored, providing useful insight into your ignore logic.
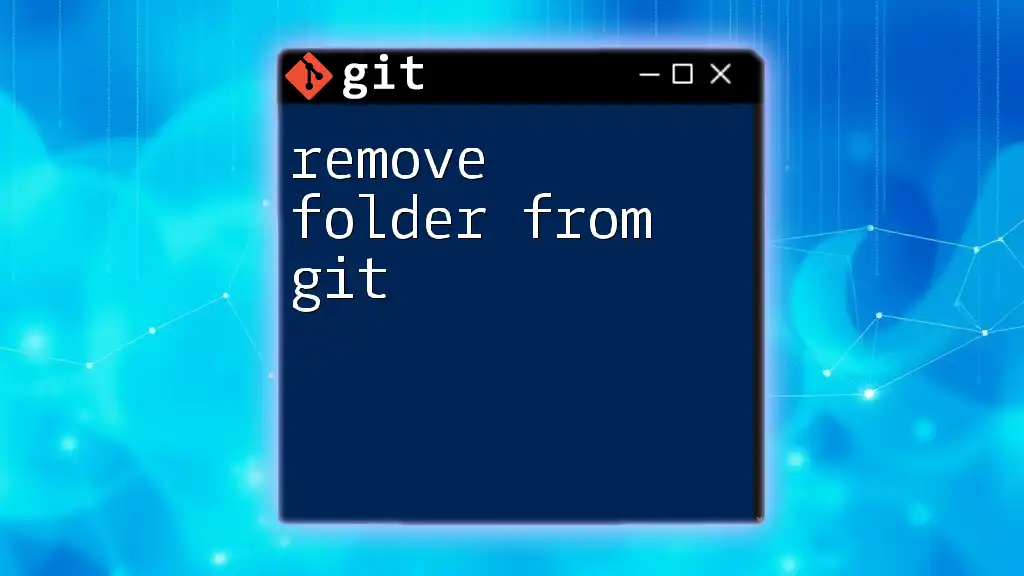
Common Pitfalls When Using `.gitignore`
Case Sensitivity
One common pitfall to be aware of is that Git is case-sensitive. For instance, if you have `Logs/` listed in your `.gitignore`, but your directory is actually named `logs/`, Git will not ignore it, and changes to that directory will still be tracked. Always ensure that the casing matches exactly to avoid oversight.
Global vs Local `.gitignore`
Git allows for both local and global `.gitignore` files. The local `.gitignore` applies to a specific repository, while a global `.gitignore` can apply to all repositories on a machine, which is helpful for ignoring system-wide files such as editor backups.
To create a global `.gitignore`, you can use:
git config --global core.excludesFile ~/.gitignore_global
This command sets up a global ignore file, allowing you to specify patterns that Git should ignore for all repositories, which significantly reduces the need to replicate ignore rules across multiple projects.
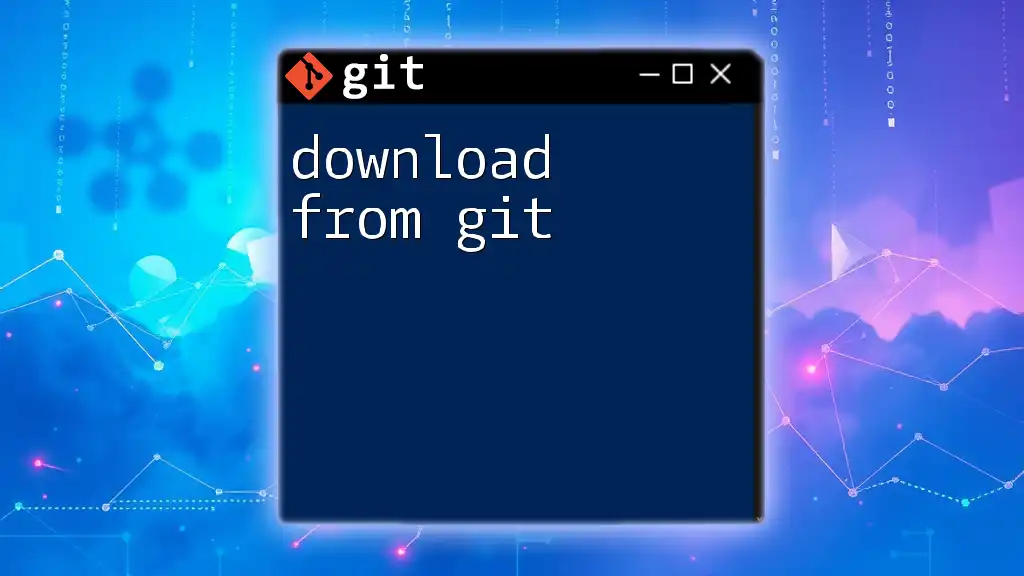
Conclusion
Excluding a directory from Git is a fundamental part of maintaining a clean and efficient project. Utilizing the `.gitignore` file correctly allows you to manage unwanted files, keeping your repository organized and devoid of unnecessary clutter. Remember that simply adding directories to `.gitignore` is not sufficient for those already being tracked; you must take additional steps to cease their monitoring.
By mastering these techniques, you can streamline your version control process and avoid common pitfalls associated with Git. Implement these guidelines in your projects, and watch your productivity soar as you manage your files more effectively.
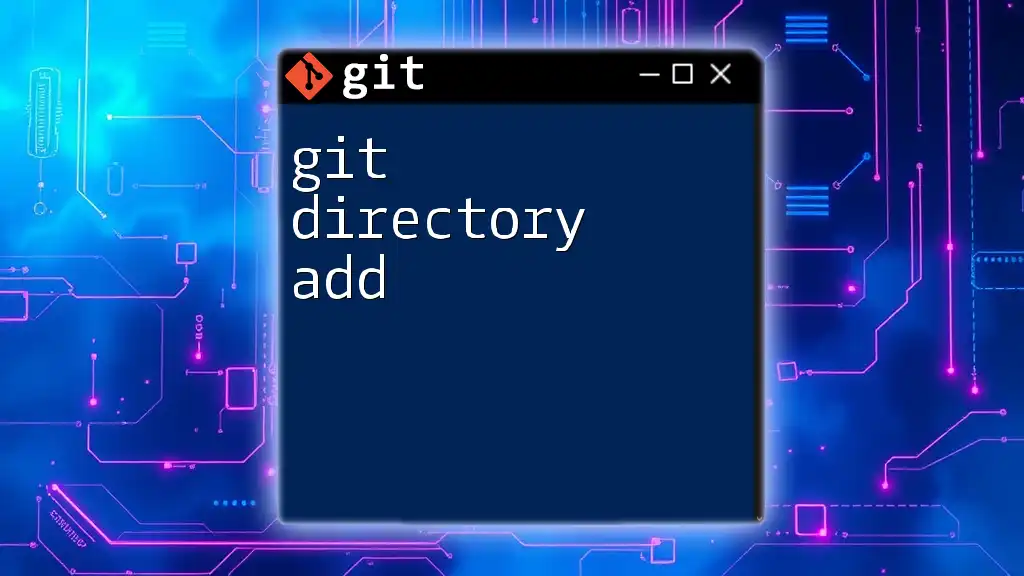
Additional Resources
For further reading, consider checking the official Git documentation regarding `.gitignore`. Joining Git tutorials or courses will greatly enhance your understanding and proficiency in using Git.
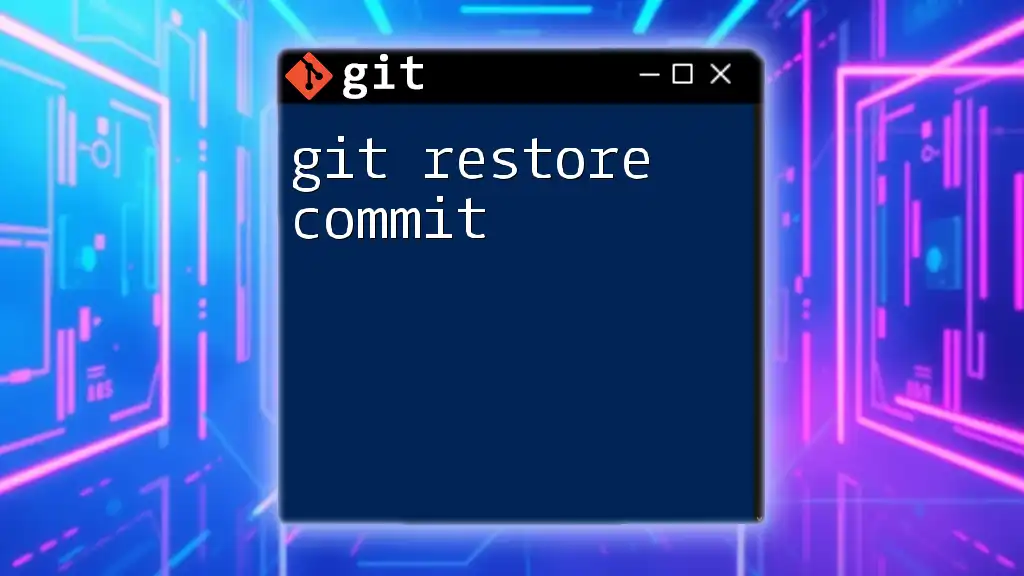
Call to Action
If you found this guide helpful and want to learn more quick techniques on effectively using Git, be sure to subscribe and stay tuned for more articles. Share this guide with fellow developers and help them in their journey to mastering version control!