You can install a Python package directly from a Git repository using pip with the following command:
pip install git+https://github.com/username/repo.git
Understanding the Basics
What is a Git Repository?
A Git repository is a storage space where your project files and the entire history of their changes are kept. In essence, it tracks all modifications made to the files in your project over time. There are two types of repositories to be aware of—public repositories, accessible to everyone, and private repositories, which restrict access to only certain users.
Basic Git Commands
Familiarity with some basic Git commands is crucial when dealing with Git repositories. Here are a few that will be relevant:
- Clone a Repository: To create a local copy of a Git repository, you typically use:
git clone <repository-url>
This command sets up a local repository that you can interact with and modify as needed.
What is Pip?
Pip is the package installer for Python, allowing you to install and manage libraries and dependencies in your Python projects effortlessly. It simplifies the process of obtaining external packages and keeps track of them in your environment. Using Pip is an essential skill for any Python developer looking to enhance their productivity and manage their projects effectively.
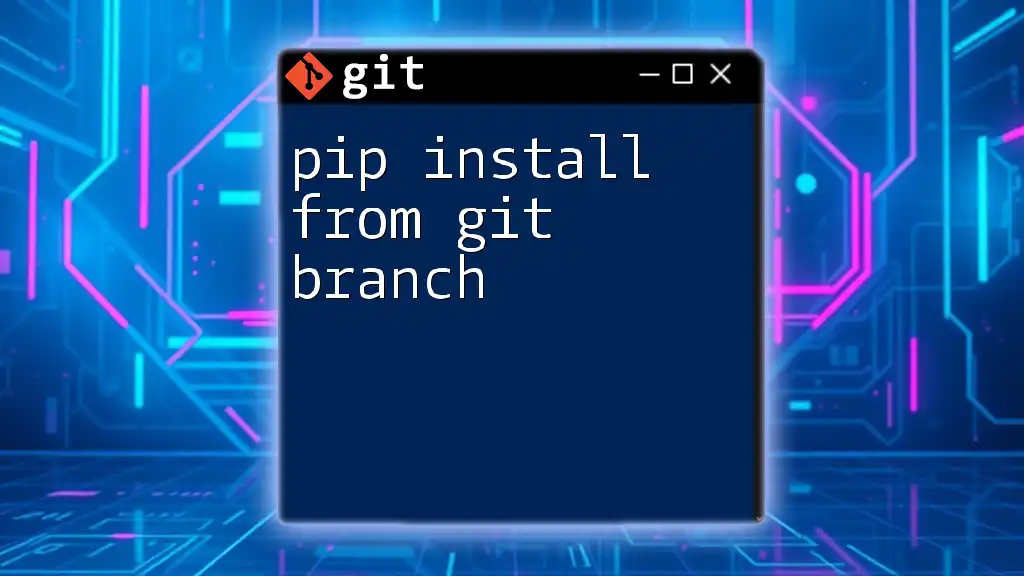
Pip Install from Git Repository
Requirements
To effectively use pip install from git repository, you need to meet a few prerequisites:
- An installed version of Python on your machine.
- Pip installed. If Pip isn't installed, you can set it up using:
python -m ensurepip --upgrade
You should also have a basic understanding of Git commands since working with Git repositories will require some foundational knowledge.
Syntax for Installing from Git
Understanding the syntax for using Pip with Git is crucial. The general structure for installing a package directly from a Git repository using Pip is:
pip install git+<repository-url>
For instance, to install a specific package from a public repository, you would use:
pip install git+https://github.com/user/repo.git
This command tells Pip to fetch the package directly from the specified Git repository URL.
Specifying Branches and Tags
In many scenarios, you may want to install from specific branches or tags of the repository. This is particularly useful for working with ongoing development or stable releases.
Installing from Specific Branches
To install a package from a specific branch, you can extend the command like so:
pip install git+https://github.com/user/repo.git@branch-name
This allows you to specify the desired branch you want Pip to pull from.
Installing from Specific Tags
Similarly, if you aim to install a version labeled with a tag, the command would look like this:
pip install git+https://github.com/user/repo.git@v1.0
Using tags can be beneficial when you want specific, stable versions of your software rather than the latest developments.
Using SSH for Private Repositories
For private repositories, using SSH keys might be necessary for authentication.
Setting Up SSH Keys
Setting up SSH keys on platforms like GitHub allows you to clone and pull from private repositories securely without needing to input your username and password repeatedly.
Installation Command with SSH
Once you have your SSH key configured, you can install from your private repository using the command:
pip install git+ssh://git@github.com/user/private-repo.git
This method ensures that you can securely access the repository without concerns about exposing your credentials.
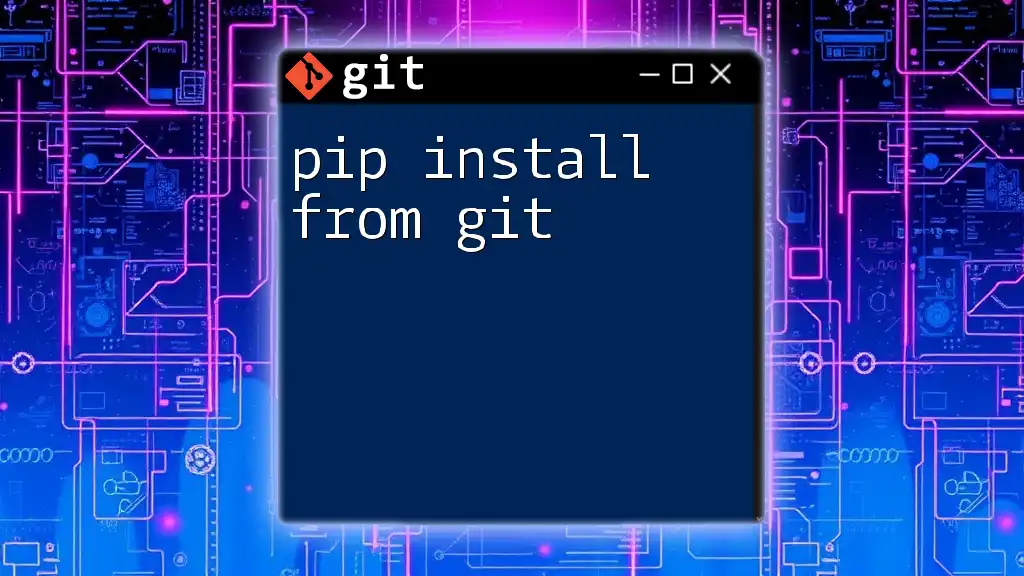
Project Structure and Requirements.txt
Including Git Dependencies in requirements.txt
Managing dependencies is crucial for any project, especially as it grows. One effective way of managing your dependencies in Python is through a `requirements.txt` file.
What is requirements.txt?
A `requirements.txt` file contains a list of all the packages your project requires, along with their respective versions. This ensures that anyone else running your project has the same setup and dependencies.
Format for Git Dependencies
You can define Git repositories directly in this file:
git+https://github.com/user/repo.git
This line in your `requirements.txt` instructs Pip to install the project directly from the given Git repository URL.
Examples of requirements.txt with Git Repos
You can mix regular packages and Git dependencies in your `requirements.txt` file. Here's an example structure that showcases both:
numpy==1.21.0
git+https://github.com/user/repo.git@branch-name
This enables you to install both standard and Git-based packages in a single command.
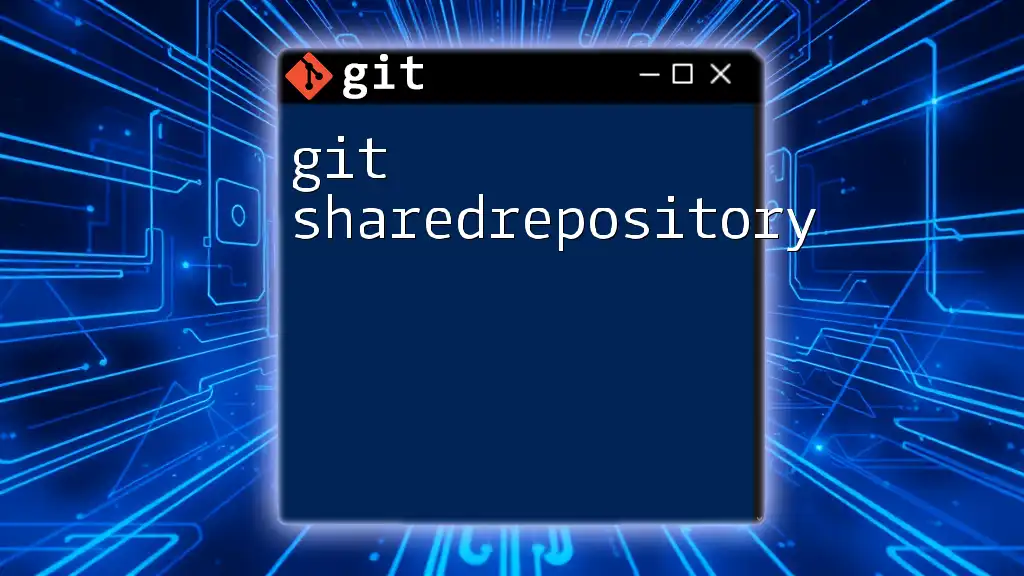
Best Practices
Keeping Packages Up-to-Date
When working with packages installed via Git, staying updated is essential. One way to do this is by using the upgrade flag:
pip install --upgrade git+https://github.com/user/repo.git
This command will fetch the latest version from the specified repository, ensuring you always have the newest features or fixes.
Troubleshooting Common Issues
During installations, you may encounter certain common problems:
- SSL Issues: If SSL verification fails, consider checking your Git configuration or using HTTP instead of HTTPS for cloning.
- Permission Denied: Make sure your SSH keys are correctly configured if you're dealing with private repositories.
By keeping these troubleshooting tips in mind, you can resolve many issues smoothly and maintain efficiency in your work.
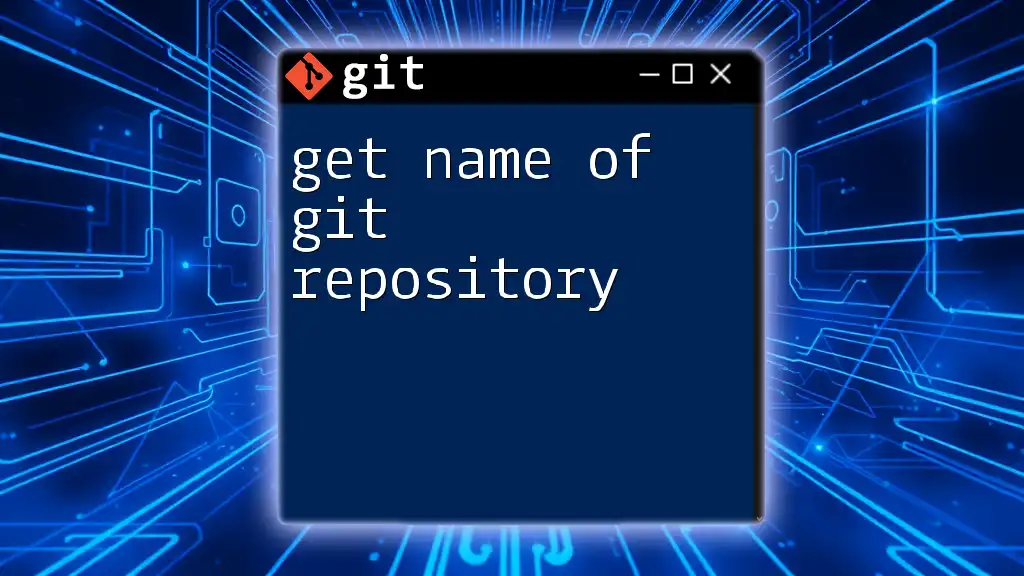
Conclusion
In summary, using pip install from git repository is a powerful feature that opens up many possibilities for software development with Python. Whether you're accessing the latest updates directly from developers or managing custom packages, this method enhances your development workflow significantly. Don’t hesitate to explore other repositories and contribute to open source; the Python community is vast and welcoming, and your participation can lead to more robust projects for everyone involved.
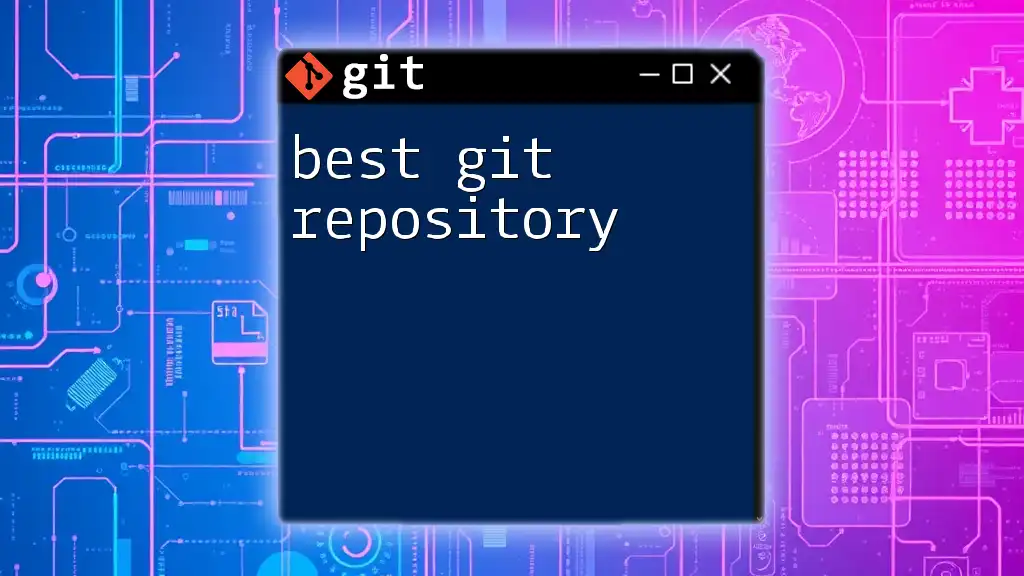
Additional Resources
For further learning, here are some helpful links:
- [Python Pip Documentation](https://pip.pypa.io/en/stable/)
- [Git Documentation](https://git-scm.com/docs)
- [Recommended Git and Pip Tutorials](insert relevant links here)
With these tools and knowledge, you're well-equipped to efficiently install and manage Python packages from Git repositories. Happy coding!