To install a Python package directly from a Git repository using pip, you can use the following command:
pip install git+https://github.com/username/repository.git
Replace `username` and `repository` with the appropriate GitHub username and repository name.
Understanding Pip
What is Pip?
`Pip` is a powerful package management system for Python that allows developers to install, manage, and uninstall packages with ease. It serves as the go-to tool for sourcing libraries and tools from the Python Package Index (PyPI) as well as from other repositories.
How Pip Works
Pip operates on the principle of fetching packages from a repository and installing them into your Python environment. This can happen locally (from a package on your computer) or remotely (from an online repository). The ease of fetching packages is what makes pip a cornerstone of Python development.
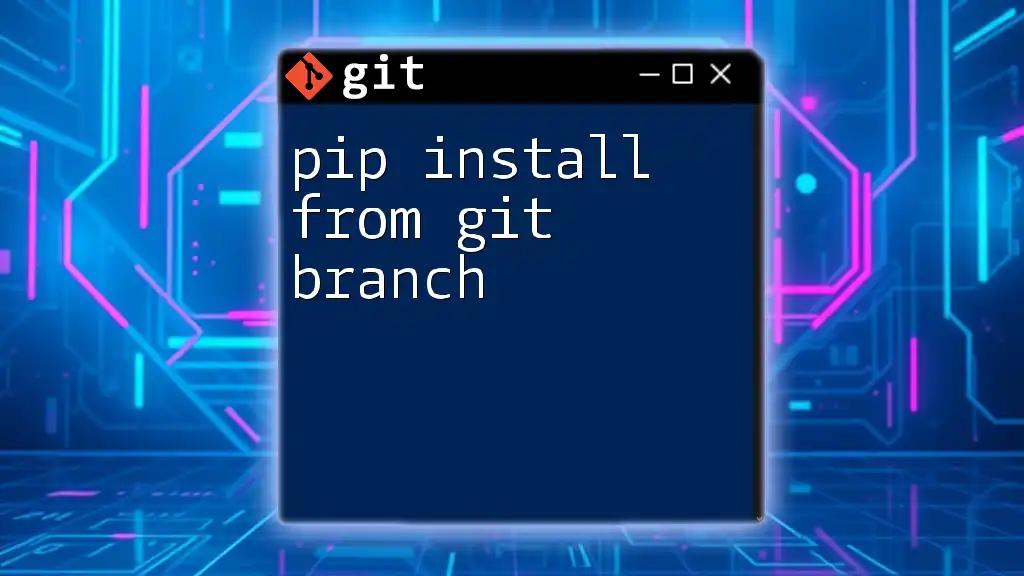
Why Install from a Git Repository?
Benefits of Installing from Git
Installing packages directly from a Git repository offers several advantages:
- Access to Latest Features or Bug Fixes: Developers who work on Git repositories can make updates that may not yet be available in the official version on PyPI. By installing directly from Git, you can access these updates immediately.
- Contribution to Open Source Projects: Many packages are available in open-source format. Installing from Git allows you to contribute back to these repositories by creating your own forks and improving the codebase.
- Customized Installations from Forks: If you have specific requirements that are not met by the official version, you can create your own fork of a package and install it with specific modifications tailored to your needs.
Use Cases for Git Installation
There are various scenarios where installing a package from Git may be the preferred option:
- Development: When working on your own projects, you may need to test a new feature that has not yet been released publicly.
- Integration: Collaborating with others on projects that reside in Git will often require pulling in specific commits or branches to test your code with others' changes.
- Experimentation: If you're trying out new libraries that are still under development, Git can provide immediate access to the cutting-edge features.
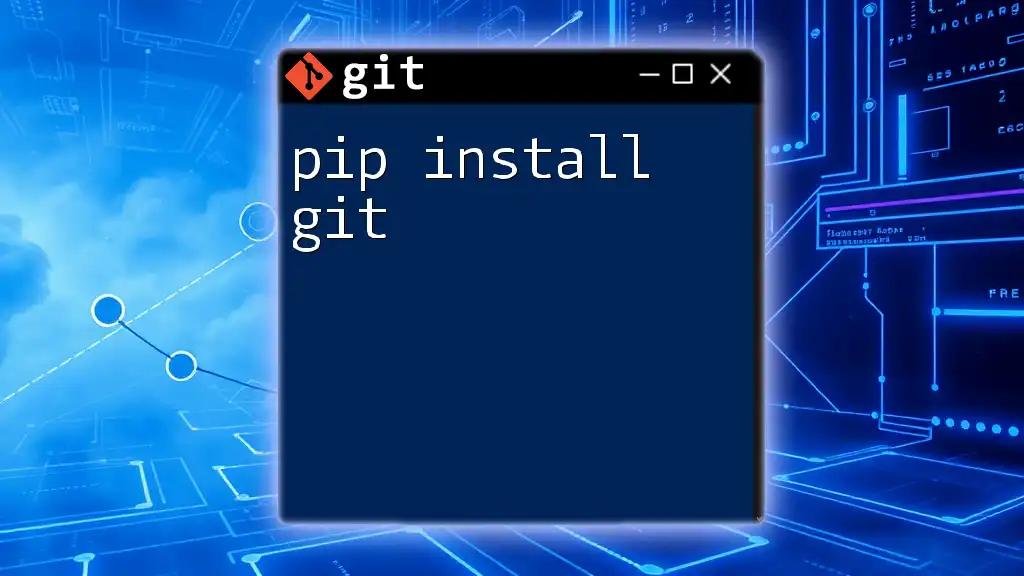
Installing Pip
Installing Pip on Your System
To utilize pip, you first need to ensure it's installed on your system. Here’s how to do it for various operating systems:
- Windows: You can download `get-pip.py` and run it with Python.
- macOS/Linux: Use the following command to install:
curl https://bootstrap.pypa.io/get-pip.py -o get-pip.py python get-pip.py
Verifying Pip Installation
Once pip is installed, you can verify that it’s functioning correctly by running:
pip --version
This command will display the current version of pip that you have installed, confirming it's ready for use.
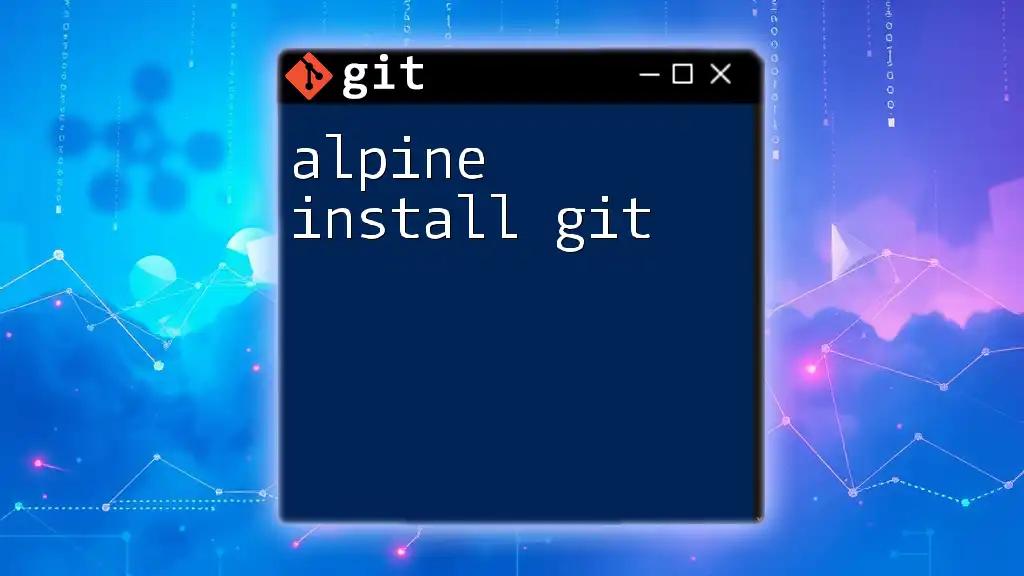
Basic Git Commands
Overview of Common Git Commands
Having a foundational grasp of essential Git commands will empower you to effectively use `pip` with Git repositories. Key Git commands include:
- `clone`: Clone a repository into a new directory.
- `commit`: Record changes to the repository.
- `push`: Upload local changes to the remote repository.
Example of Using Git Commands
To clone a repository from GitHub, you would use:
git clone https://github.com/username/repo.git
This command will create a local copy of the repository on your machine, allowing you to work with it directly.
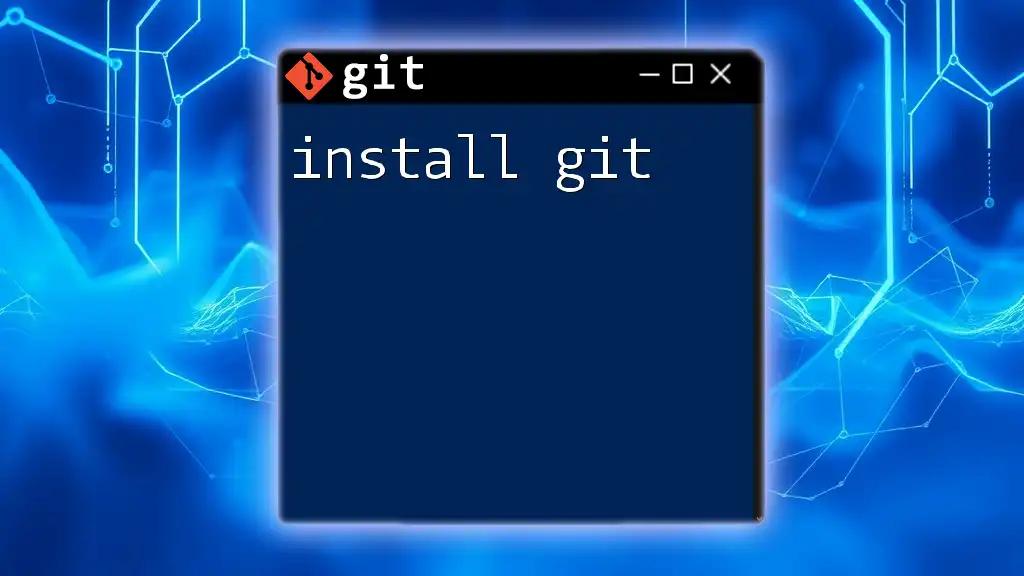
Using Pip to Install from Git
The Basic Syntax
The syntax for installing a Python package directly from a Git repository with pip is straightforward:
pip install git+https://github.com/username/repo.git
This command pulls in the package from the specified Git repository and installs it into your environment.
Different Methods of Cloning
HTTPS vs. SSH
Using HTTPS is generally easier as it does not require setting up SSH keys. However, SSH offers a convenient way to interact with repositories and is more secure. If you have SSH keys set up, you can clone a repository via SSH with:
git clone git@github.com:username/repo.git
Specifying a Branch
If you need to install a package from a specific branch, you can do so by appending the branch name:
pip install git+https://github.com/username/repo.git@branch_name
This is particularly useful when you know that a feature or fix exists in a non-default branch.
Installing Specific Commits
To install a package at a specific commit instead of the latest version or branch, provide the commit hash:
pip install git+https://github.com/username/repo.git@commit_hash
This enables you to lock your installation to a known state of the codebase, ensuring stability in your project.
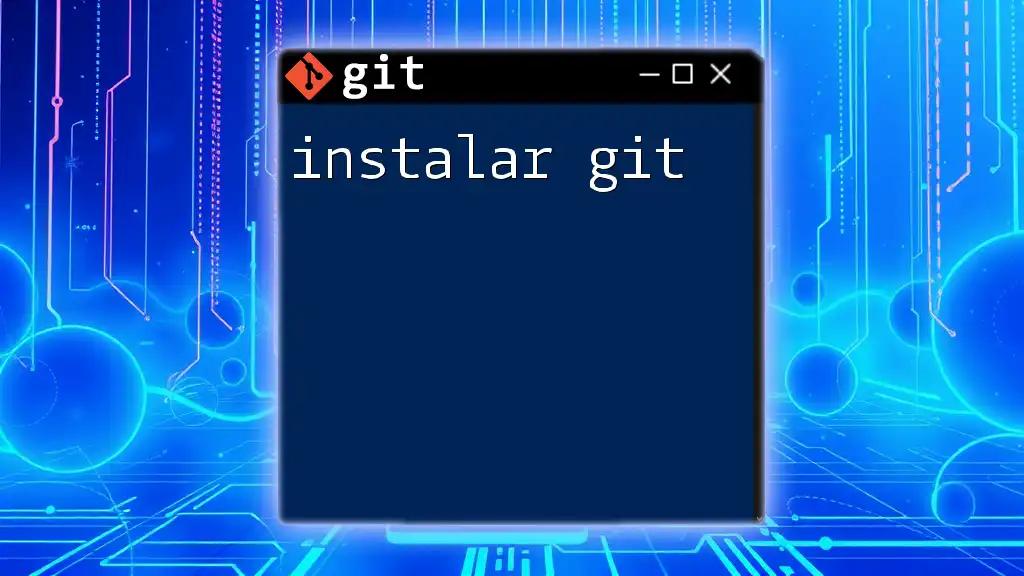
Troubleshooting Common Issues
Authentication Issues
If you encounter an authentication issue while using Git, verify that your credentials are correct and that you have permission to access the repository. For HTTPS, you might need to enter your username and personal access token instead of your GitHub password.
Dependency Conflicts
Sometimes, installing a package from Git can lead to conflicts with dependencies. If you experience this, consider using isolated environments to manage your dependencies better or examine the `requirements.txt` for compatibility issues.
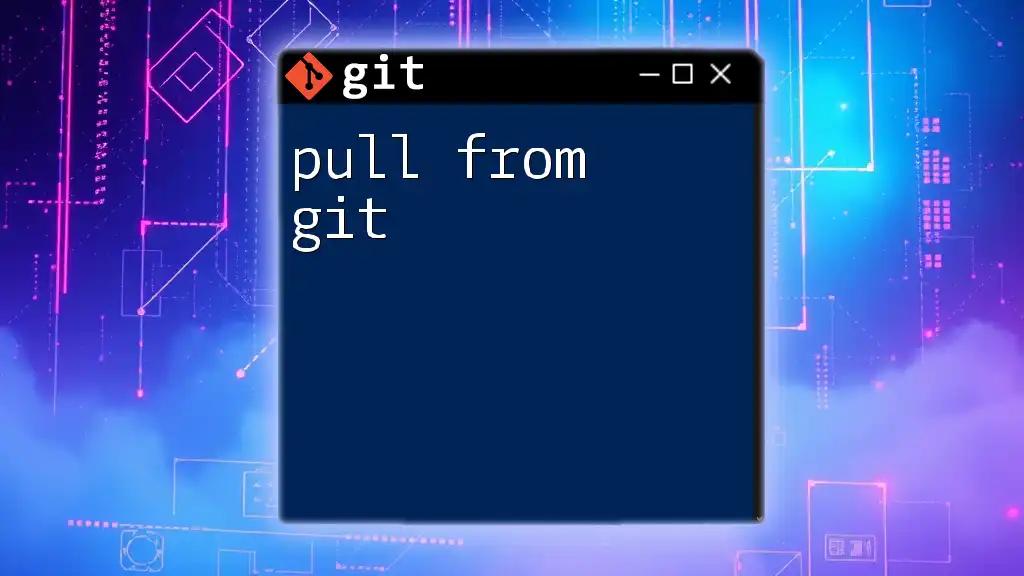
Best Practices for Using Pip with Git
Keeping Repositories Up-to-Date
To keep your installed package updated with the latest changes from the repository, you should frequently check for updates or reinstall the package:
pip uninstall package_name
pip install git+https://github.com/username/repo.git
This dual command refreshes your package based on the newest code.
Using Virtual Environments
Utilizing virtual environments is highly recommended when installing packages from Git. This protects your project from dependency conflicts with globally installed packages. To create a virtual environment:
python -m venv myenv
source myenv/bin/activate # for Linux/Mac
myenv\Scripts\activate # for Windows
After creating your environment, you can use pip to install packages without affecting other projects.
Documenting Your Installation Process
Keeping track of the packages you install is essential for future reference and can be done via a `requirements.txt` file. To create this file, you can use:
pip freeze > requirements.txt
This command records all installed packages along with their versions, simplifying project sharing and dependency management in the future.
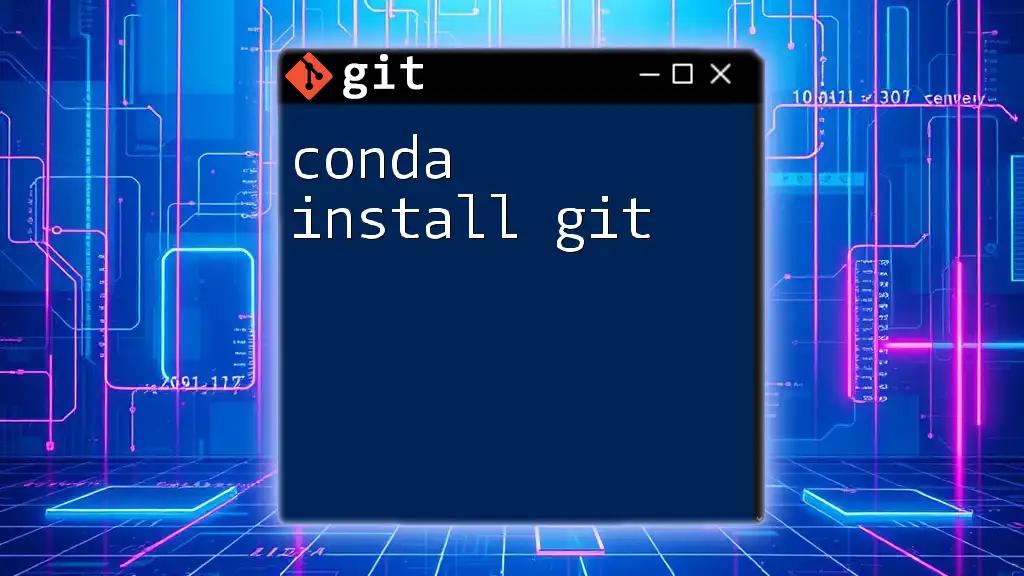
Conclusion
Understanding how to use the `pip install from git` command can greatly enhance your Python development capabilities. Whether you’re accessing the latest updates, contributing to open source, or customizing packages for your specific needs, Git is a vital tool in your arsenal. As you explore more about integrating Git with your Python projects, consider diving deeper into best practices and community resources to maximize your development efficiency.
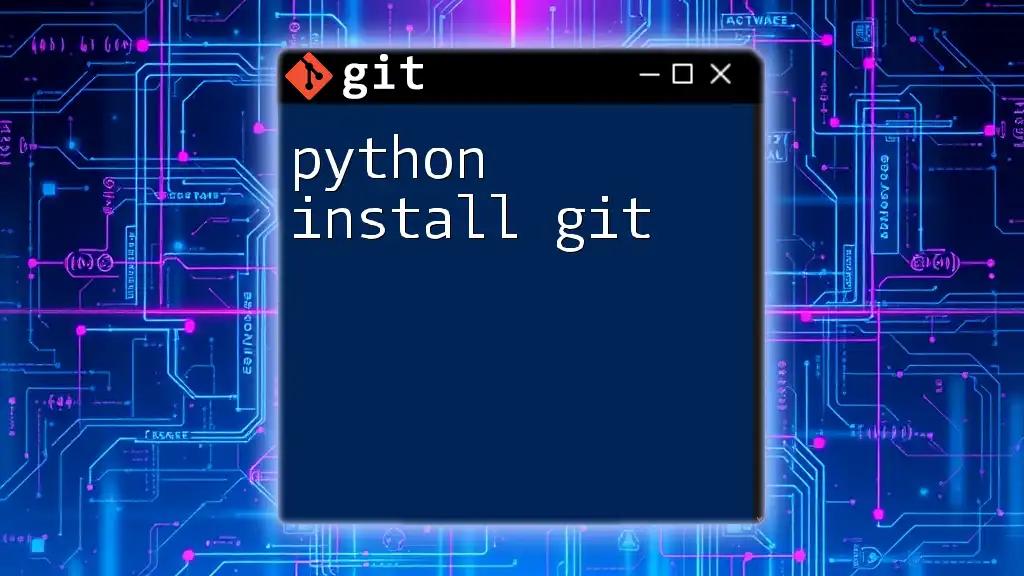
Additional Resources
Further Reading
For in-depth learning, refer to the official documentation of both Git and pip. This will enrich your understanding and proficiency in managing packages effectively.
Community and Support
Engage with the vibrant Python and Git communities through forums like Stack Overflow or Reddit, where vast amounts of knowledge and experience are shared daily.
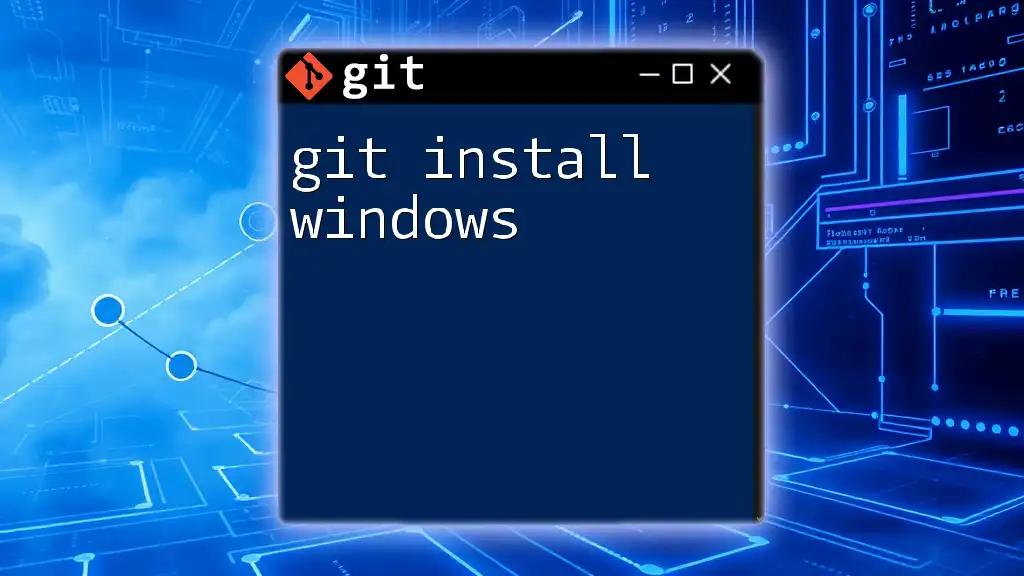
Call to Action
Stay ahead in your Python and Git journey by subscribing to our updates for practical tips, courses, and resources designed to empower your development skills!