To pull the latest changes from a remote Git repository into your current branch, use the following command:
git pull origin main
Replace `main` with the name of your desired branch if you're pulling from a different one.
Understanding `git pull`
What does `git pull` do?
The `git pull` command is essential for synchronizing your local repository with a remote one. It combines two critical operations: fetching the changes from a remote repository and then merging those changes into your current branch. This means when you execute `git pull`, it effectively retrieves the latest commits from the specified branch of the remote repository and integrates them into your active working directory.
When to use `git pull`
You should use `git pull` whenever you want to receive updates from a remote repository. This is especially important in collaborative projects where multiple developers are pushing changes. Regularly pulling helps ensure that you are working with the most up-to-date codebase, minimizes potential conflicts, and improves the overall efficiency of the development process.
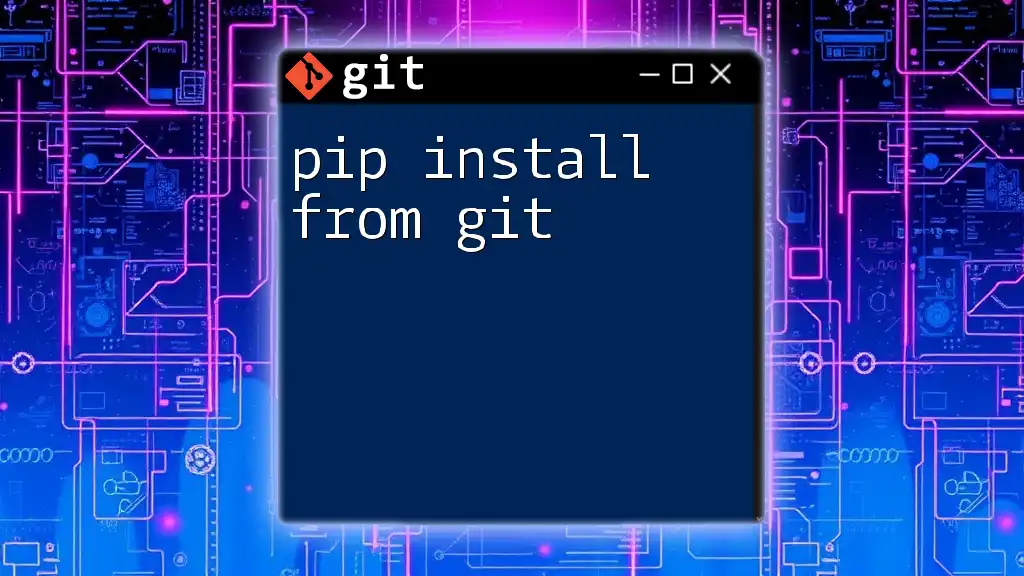
Basic Syntax of `git pull`
Command structure
The general syntax for the `git pull` command is as follows:
git pull [options] [<repository> [<refspec>...]]
Breaking this down:
- `options`: These are flags that modify how `git pull` behaves.
- `<repository>`: This typically refers to the remote repository you want to pull from (e.g., `origin`).
- `<refspec>`: This indicates the specific branch or tag you wish to pull from the remote repository.
Common Options
-
`--rebase`: This option allows you to reapply your local changes on top of the fetched changes. It can help maintain a cleaner project history by avoiding unnecessary merge commits.
-
`--no-commit`: This option prevents Git from automatically committing after merging changes. You might want to examine the changes before creating a commit.
-
`--quiet`: By using this option, you suppress the output messages during the pull operation, which can help in a cluttered terminal.
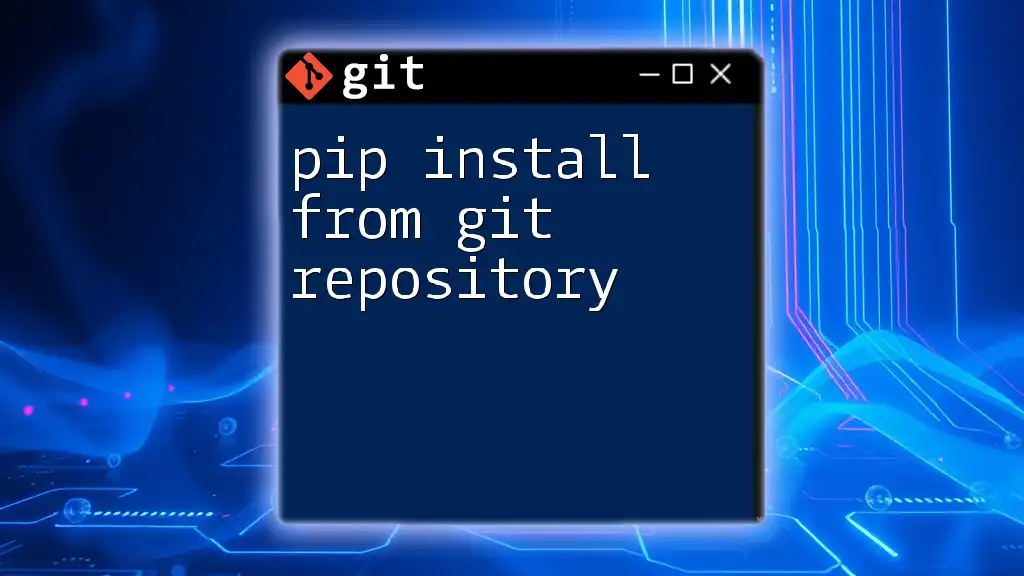
How to Perform a `git pull`
Pulling from the Default Remote
To pull changes from the default remote repository (commonly named `origin`), simply execute:
git pull
This command will pull the changes from the branch you are currently tracking. If your local branch is tracking the remote branch correctly, this command will seamlessly keep you updated with the latest changes.
Pulling from a Specific Branch
If you need to pull changes from a specific branch in a remote repository, you can specify the remote name and branch name like this:
git pull origin feature-branch
In this example, `origin` is the remote repository, and `feature-branch` is the branch you want to update your local branch with. This command will ensure that you are merging updates from the desired branch into your current working branch.
Handling Conflicts
When pulling changes, you might encounter merge conflicts. This happens when the changes in the remote repository overlap with your local changes, creating uncertainty about which changes to keep.
To resolve merge conflicts:
-
First, identify the files that Git flagged as having conflicts using:
git status
-
Open the conflicting files and look for conflict markers (e.g., `<<<<<<<`, `=======`, `>>>>>>>`). These markers delineate the differing changes between the local and remote versions.
-
Manually edit the file to remove the conflict markers and choose the appropriate changes to keep.
-
Once resolved, stage your changes and commit them:
git add <filepath> git commit -m "Resolved merge conflict"
Best Practices for Using `git pull`
-
Regularly Synchronize: Make it a habit to regularly use `git pull` to keep your local repository updated. This minimizes potential conflicts when you go to push your changes.
-
Pull Before Starting New Work: It’s advisable to pull from the remote before you start any new feature or modification. This ensures you work on the latest code and reduces the chances of introducing conflicts later.
-
Use Feature Branches: By developing features in separate branches and periodically pulling changes into them, you can maintain a clean and organized project history while making sure your branches are up-to-date.
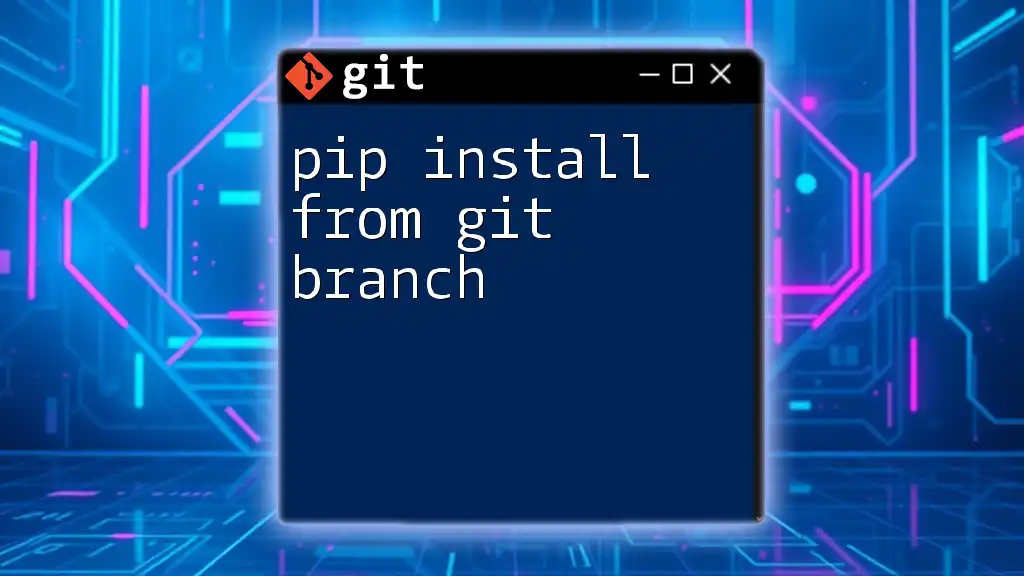
Advanced Usage of `git pull`
Pulling with Rebase
When using `git pull`, incorporating the `--rebase` option can significantly streamline your workflow. With rebase, instead of merging the changes, Git applies your local commits on top of the changes fetched from the remote. This keeps the commit history linear and avoids the clutter generated by unnecessary merge points.
Using the command looks like this:
git pull --rebase
Pulling with Specific Paths
If you are working with large repositories and wish to pull changes only for a specific directory or file, you can leverage the path specification feature:
git pull origin master -- path/to/directory/
This command pulls changes only for the specified path rather than the entire repository, which can save time and resources in big projects.
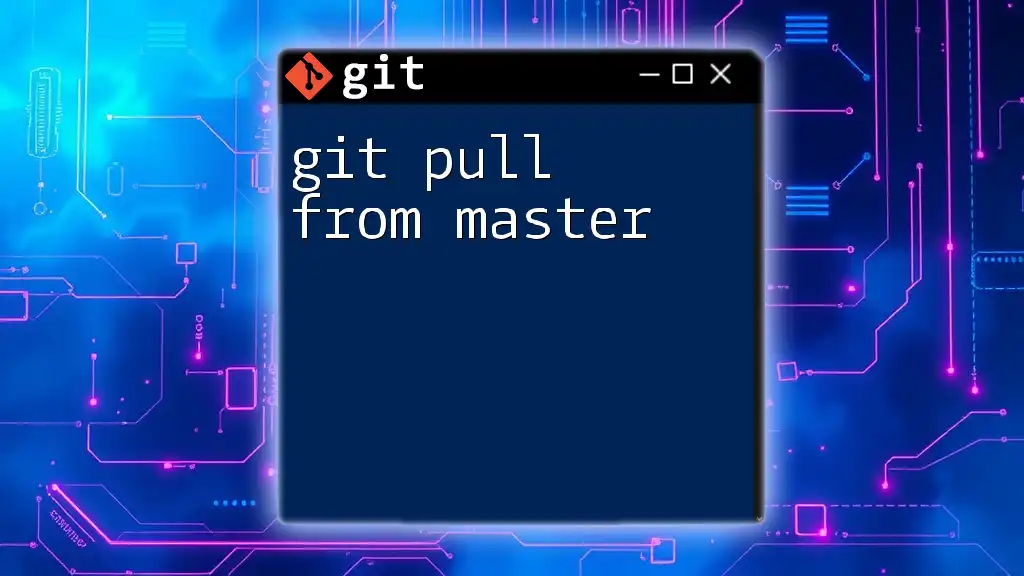
Troubleshooting `git pull`
Common Issues and Solutions
-
Pull Fails Due to Uncommitted Changes: If you attempt to pull while having uncommitted changes, Git will prevent the operation. To resolve this, you can temporarily stash your changes:
git stash git pull git stash pop
This process saves your uncommitted changes, allows you to pull the new changes, and then reapplies your stashed changes.
-
Unmerged Paths Error: Sometimes, you might encounter an error indicating unmerged paths. This usually happens when there are conflicts that need resolving. The recommended steps include:
- Check status using:
git status
- Troubleshoot and resolve the conflicts.
- After resolving all conflicts, stage and commit.
- Check status using:
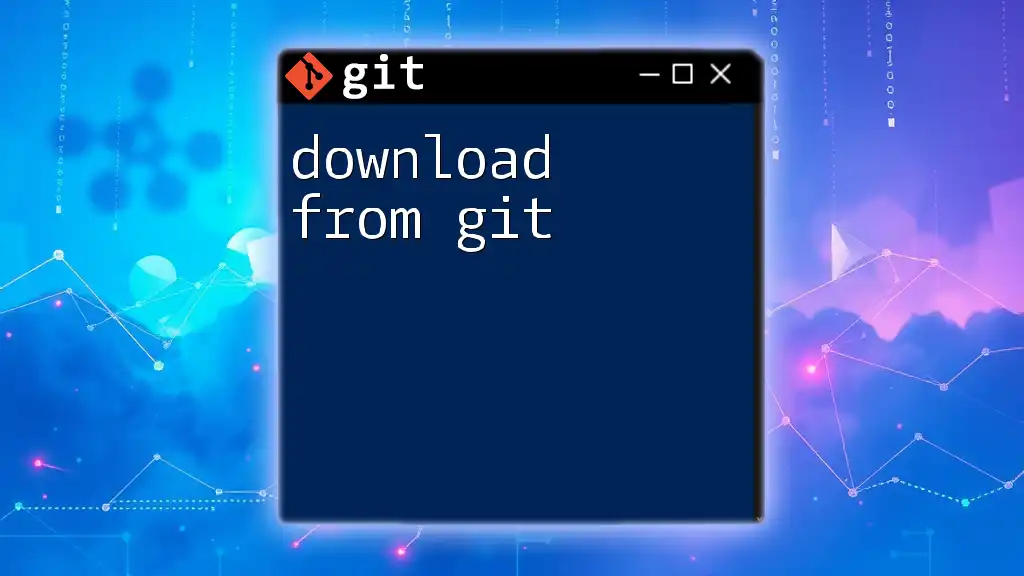
Conclusion
The `git pull` command is a powerful tool that every developer should master. By understanding its operations and best practices, you can maintain an efficient and organized workflow in collaborative projects. Regularly updating your local repository not only reduces the likelihood of conflicts but also enhances your productivity and collaboration with your team.
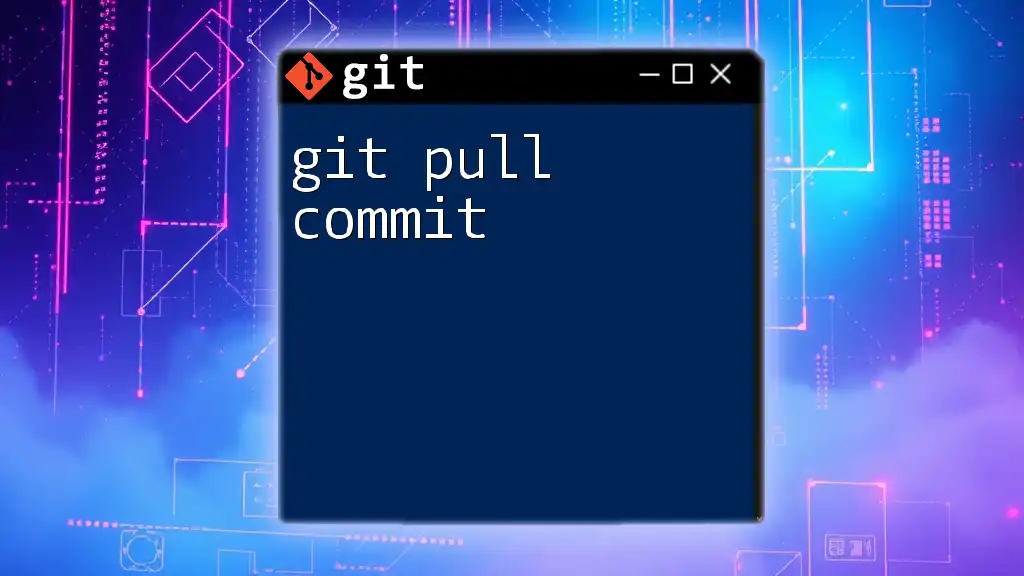
Additional Resources
For further learning about Git, consider exploring other tools and articles that delve deeper into its functionalities. Review the official Git documentation on `git pull` for comprehensive insights and advanced usage.
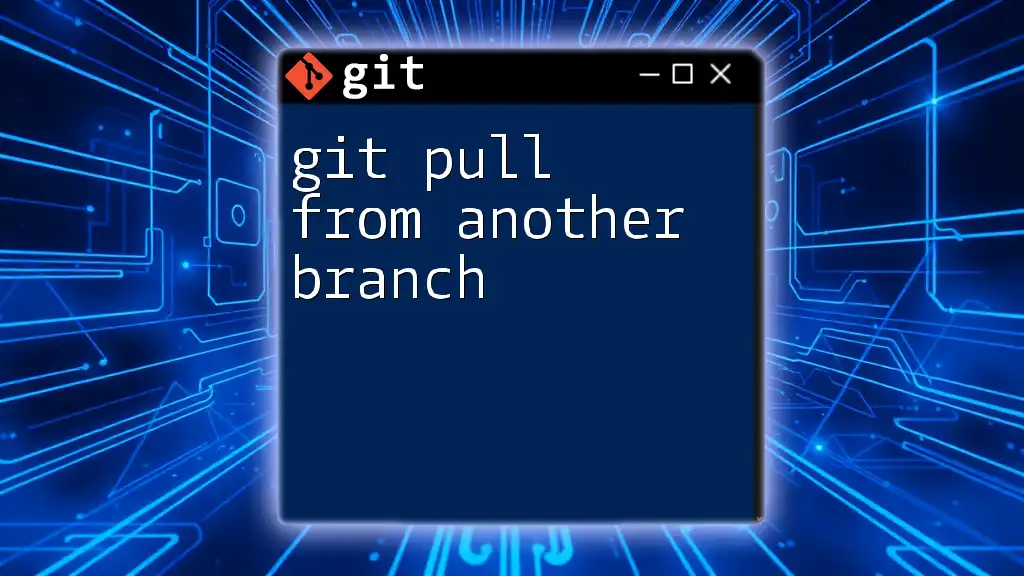
Call to Action
We encourage you to share your experiences with `git pull` and how it has impacted your workflow. Subscribe to our blog for more tutorials and tips on mastering Git commands!