The command `git` is run in the shell to perform a variety of version control operations, such as cloning repositories, committing changes, and managing branches.
git clone https://github.com/username/repository.git
Understanding the Command Line
What is the Command Line?
The command line interface (CLI) is a way to interact with your computer by typing commands into a terminal. Unlike a graphical user interface (GUI), where you click buttons and menus, the CLI requires users to understand text-based commands. While it may seem intimidating at first, using the command line for Git provides enhanced speed and control over version control processes, making it an invaluable tool for developers.
Navigating the Shell
Before you can effectively use Git commands, it's essential to become familiar with basic shell navigation commands. Here are a few fundamental commands to get you started:
- `cd`: Change Directory. This command allows you to navigate between directories.
- `ls`: List Files. It displays the contents of the current directory.
- `pwd`: Print Working Directory. This command shows the path of your current directory.
By mastering these basics, you’ll find it easier to maneuver within your file system and utilize Git commands efficiently.
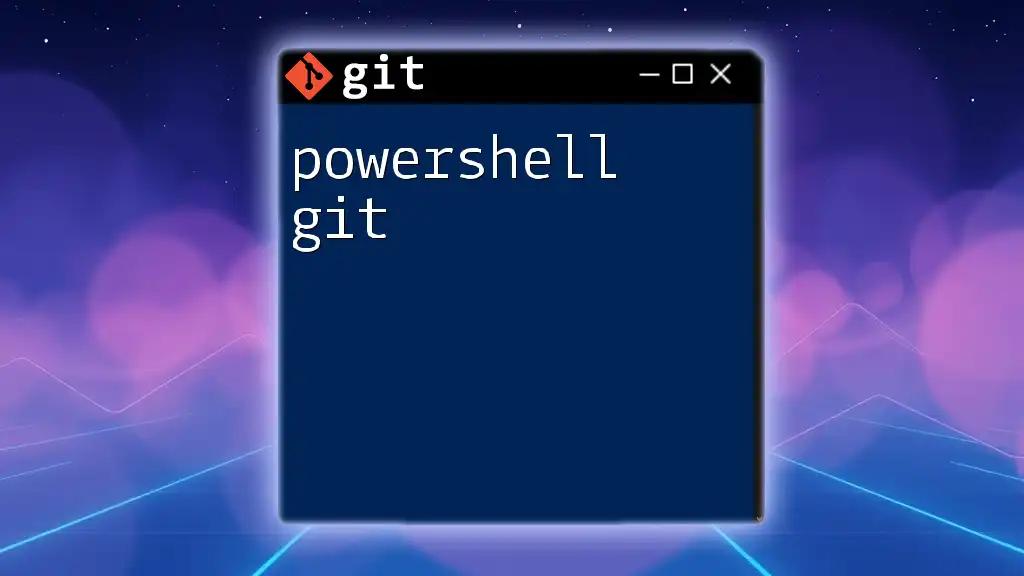
Setting Up Git in the Shell
Installing Git
To begin using Git in the shell, you first need to install it on your computer. The installation process varies slightly depending on your operating system:
- For Debian/Ubuntu, you can use the following command:
sudo apt install git
- For macOS, Git typically comes with Xcode tools. If you don’t have it installed, you can run:
xcode-select --install
- For Windows, you can download Git from the official Git website and follow the installation instructions provided there.
Configuring Git
After installation, you should configure your Git environment by providing your user name and email address. These details are crucial, as they will be associated with your commits.
Run the following commands to set up your configuration:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
To verify your configuration, you can check your settings with:
git config --list
This step ensures that every commit you make in the future will carry your name and email, helping you keep track of contributions.
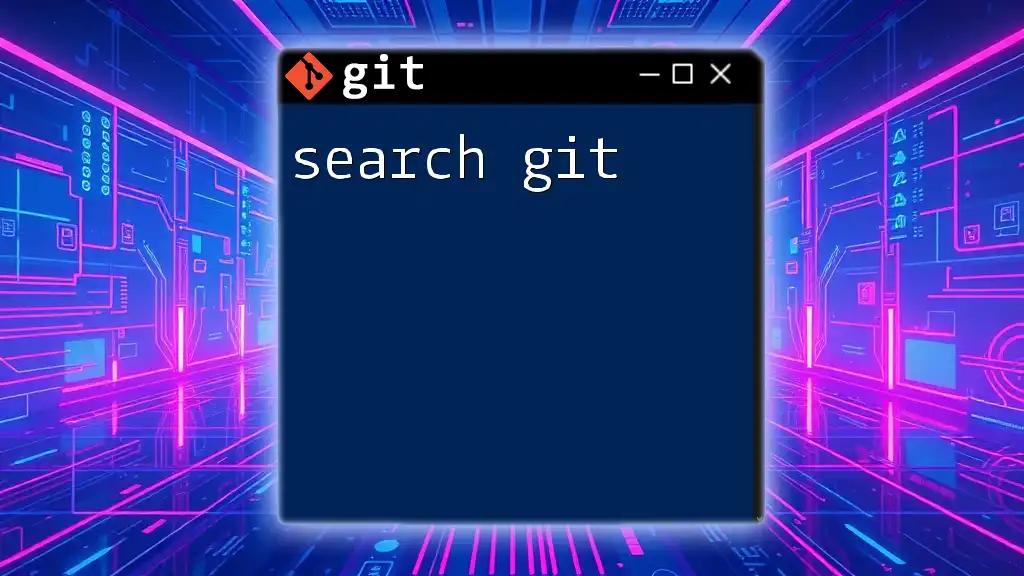
Basic Git Commands in the Shell
Creating a New Repository
To start a new project with Git, you’ll want to create a new repository. This is done by navigating to the desired directory and running:
git init my-repo
This command initializes a new Git repository in a folder called `my-repo`. Your project is now under Git's version control!
Cloning an Existing Repository
If you want to work on a project that is already hosted remotely, you can clone it using the following command:
git clone https://github.com/user/repository.git
This command creates a local copy of the repository on your machine, including all its commits and branches.
Checking Repository Status
To see the current state of your repository, including untracked and modified files, use:
git status
This command provides you with vital information, helping you decide your next steps, whether that means staging files or committing changes.
Adding Changes to Staging
Before you commit your changes, you must add them to the staging area. To stage a single file, use:
git add filename.txt
If you want to add all modified files at once, you can use:
git add .
Committing Changes
Once your changes are staged, you can commit them with a descriptive message using:
git commit -m "Your commit message here"
Crafting clear and meaningful commit messages is crucial, as they explain the purpose of the changes to anyone reading the logs in the future.
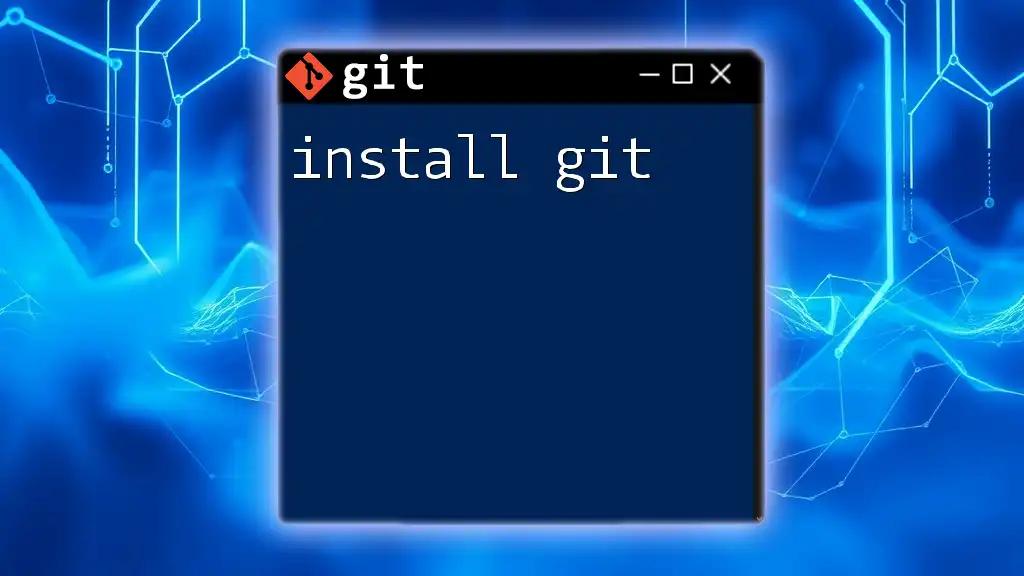
Working with Branches
Creating a New Branch
Branches allow you to work on features without affecting the main codebase. To create a new branch, run:
git branch new-branch
Switching Branches
You can switch to your newly created branch using:
git checkout new-branch
This command ensures that you're working in the correct context and that your changes won't interfere with others.
Merging Branches
Once your feature is complete, you can merge your changes back into the main branch. Assuming you are currently on the main branch, you’ll run:
git merge new-branch
If there are conflicts, Git provides guidance on how to resolve them, but it's essential to review conflicting files carefully.
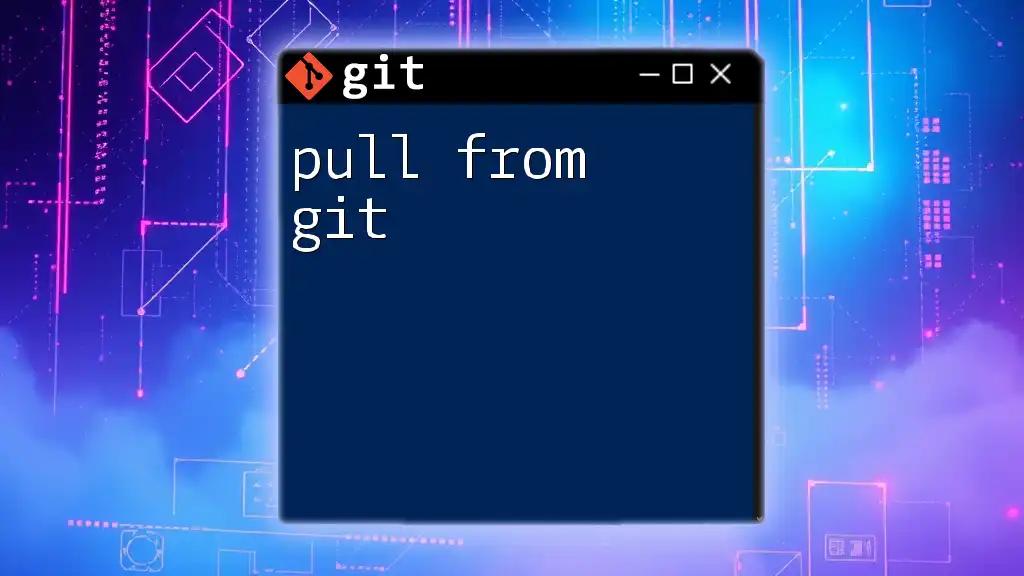
Remote Repository Operations
Connecting to a Remote Repository
To push your local changes to a remote repository, you first need to define the remote repository. Use the following command:
git remote add origin https://github.com/user/repository.git
Pushing Local Changes
After making your commits, the next step is to push those changes to your remote repository. Execute:
git push origin main
This command uploads your commits to the specified branch of the remote repository.
Pulling Updates from Remote
To sync your local repository with the remote one, you may want to pull updates created by other contributors. Use:
git pull origin main
This command merges the changes from the remote repository into your local branch.
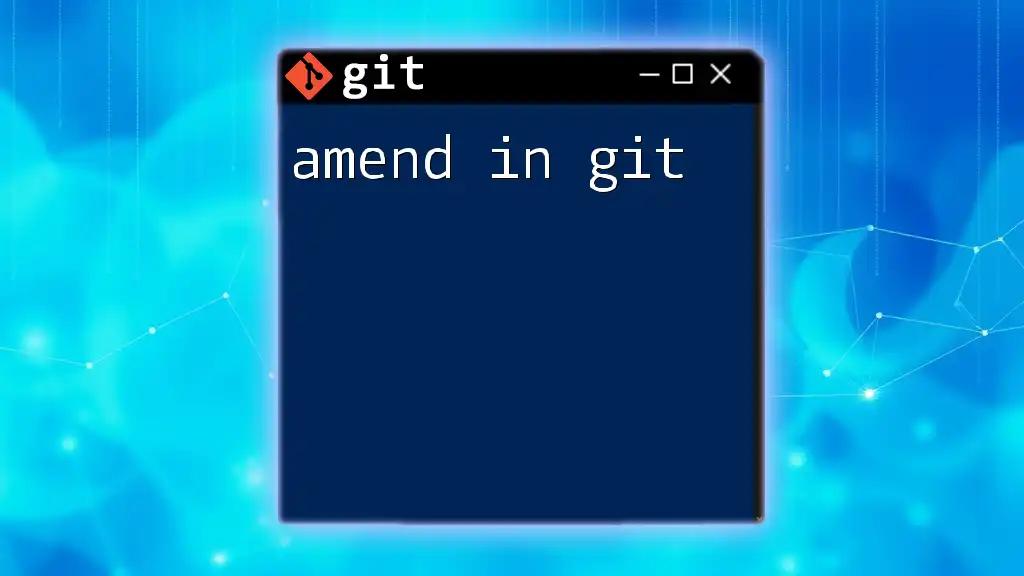
Advanced Git Commands
Stashing Changes
Sometimes you may need to switch branches but have uncommitted changes. `git stash` allows you to temporarily save these changes without committing them:
git stash
Later, you can retrieve your stashed changes using:
git stash pop
Viewing Commit History
To review the commit history of your repository, you can use:
git log
This command presents you with a chronological list of commits, including commit messages, hashes, and author information.
Undoing Changes
Reverting changes in Git involves a few commands depending on the situation. For example, if you want to discard changes in your working directory, you can run:
git checkout -- filename.txt
If you want to undo the last commit while preserving the changes in your working directory, use:
git reset HEAD~1
For a safe way to create a new commit that reverses changes, use:
git revert HEAD
This command is particularly useful for maintaining a clean project history.
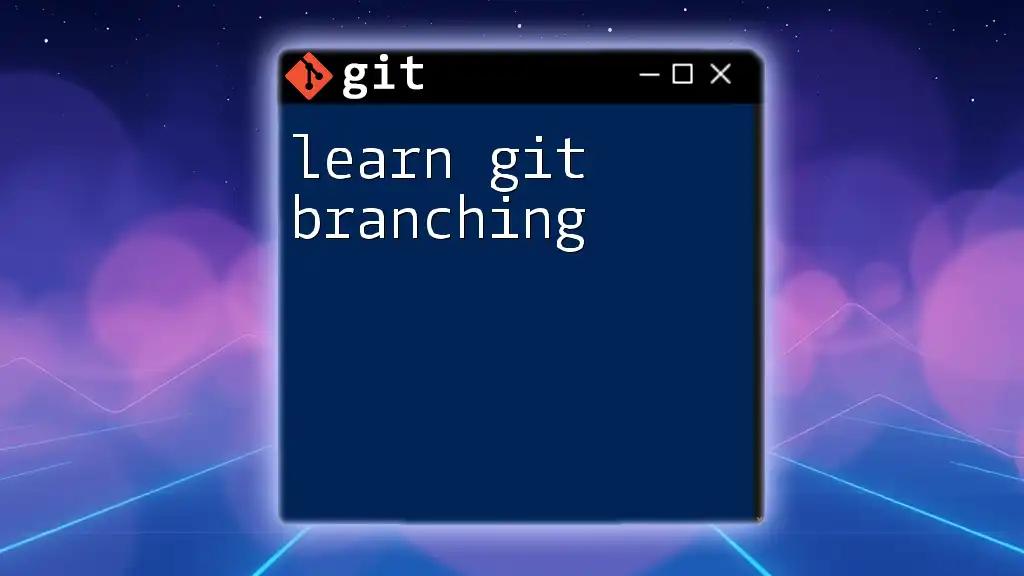
Conclusion
Utilizing the command line to run Git commands is a powerful skill that enhances your version control capabilities. This guide has equipped you with the foundational commands and techniques needed for effective version control using Git in the shell. By practicing these commands and integrating them into your workflow, you'll streamline your processes and collaborate more efficiently.
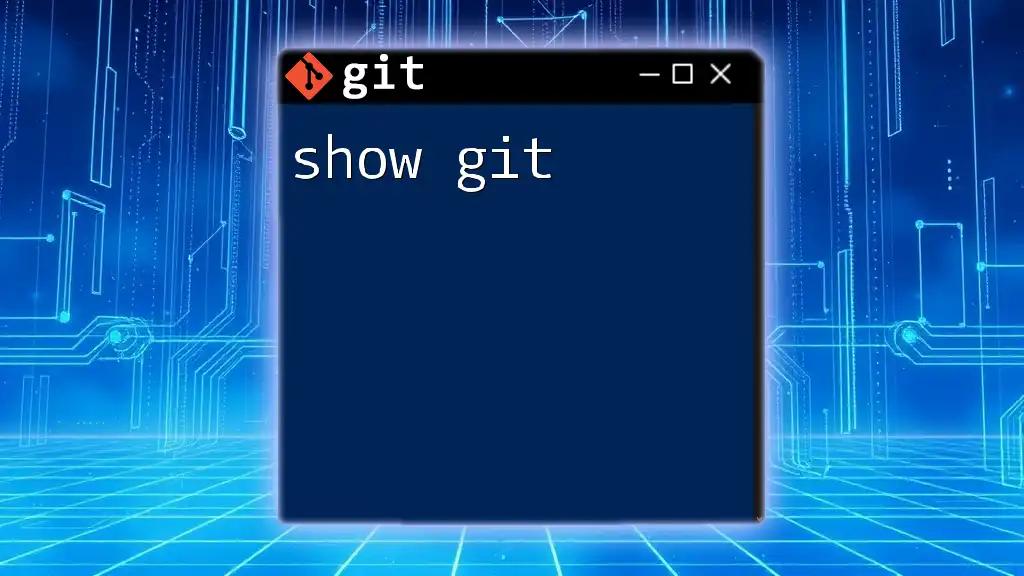
Additional Resources
- For detailed guidelines and advanced topics, check the [official Git documentation](https://git-scm.com/doc).
- Consider referring to recommended books and online courses to further your understanding and mastery of Git.
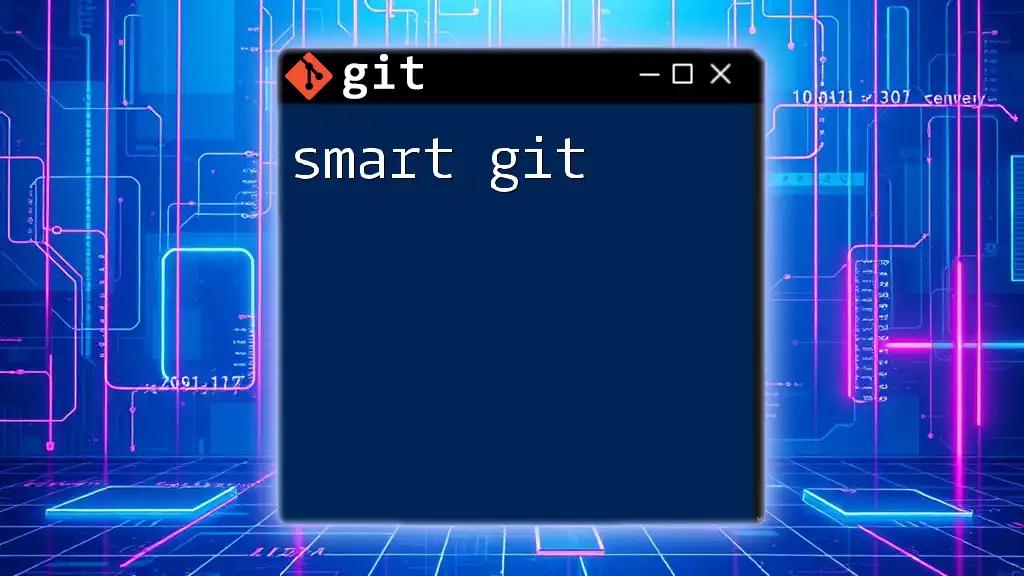
FAQs
Q: What is the difference between `git pull` and `git fetch`? A: `git pull` updates your current branch with changes from the remote. In contrast, `git fetch` only retrieves new data from the remote without merging it into your branch.
Q: How can I see the differences between the working directory and the last commit? A: You can view differences using:
git diff
This command displays all changes you've made since the last commit.
By following this guide, you can become proficient at using Git with the command line, paving the way for successful version control in your projects.