The `git show` command is used to display various types of objects in Git, including commits, trees, and tags, typically revealing the changes made in a specific commit.
git show <commit-hash>
Understanding the "Show" Command in Git
What is the "show" Command?
The “git show” command is an integral part of Git’s functionality, designed to display various types of Git objects in detail. It acts as a versatile tool that allows developers to quickly access critical information about commits, tags, files, and more. In essence, it serves to provide a clear view of specific parts of a repository's history, making it easier to track changes and understand project evolution.
Common Use Cases
You might wonder when to employ the “show” command. Here are some frequent scenarios:
- To inspect a particular commit and understand what changes it introduced.
- To review differences introduced by a commit clearly.
- To quickly identify files modified by a specific commit.
- To check the details of tags and branches without navigating to them.
Basic Syntax of git show
The basic syntax for the “git show” command is:
git show [options] <object>
Here, options can modify how the output is displayed, while <object> can refer to commits, tags, branches, or even specific file paths within the repository. The flexibility of this command lies in its ability to target various Git objects.
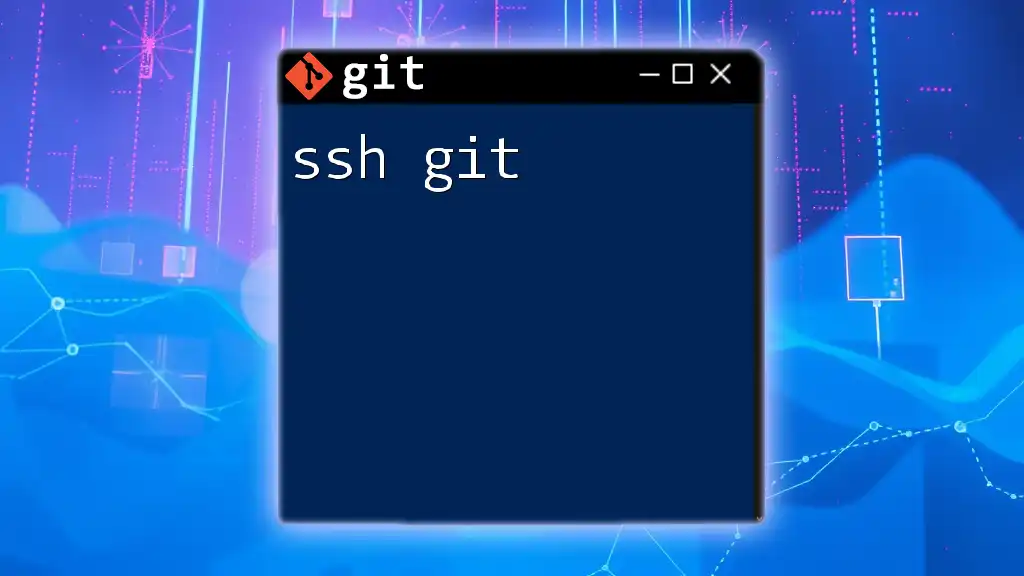
Using the "Show" Command: Practical Applications
Viewing Commit Details
One of the primary functions of “git show” is to examine the details of individual commits. This command reveals various commit properties such as the author’s name, date of the commit, and the commit message itself.
To view a specific commit, you can use:
git show 1234567
In this example, “1234567” refers to the commit hash. The output will present essential details such as:
- Author: The person who made the commit.
- Date: When the commit was created.
- Commit Message: A brief description of the changes made.
Understanding these details can provide context and background on why certain decisions were made in the codebase.
Viewing Differences Introduced by a Commit
In addition to showing basic commit information, the “git show” command can also provide a side-by-side comparison of changes introduced in that commit.
To display the patch indicating what has been changed, you can use:
git show --patch 1234567
This command will generate a diff output, showing lines that were added or removed. Lines added are typically marked with a “+” sign, while removed lines will be indicated with a “-” sign. This visual representation allows developers to understand exactly what alterations were made compared to the previous state of the code.
Viewing Files Modified in a Commit
Sometimes, you may want to focus solely on the files that were altered by a commit, without the additional noise of the commit message or diff output. For that purpose, you can execute:
git show --name-only 1234567
This command provides a clean list of filenames affected by the commit, making it easier to see the impact of changes without distraction. Each filename represents a file that has been modified, created, or deleted in the specific commit.
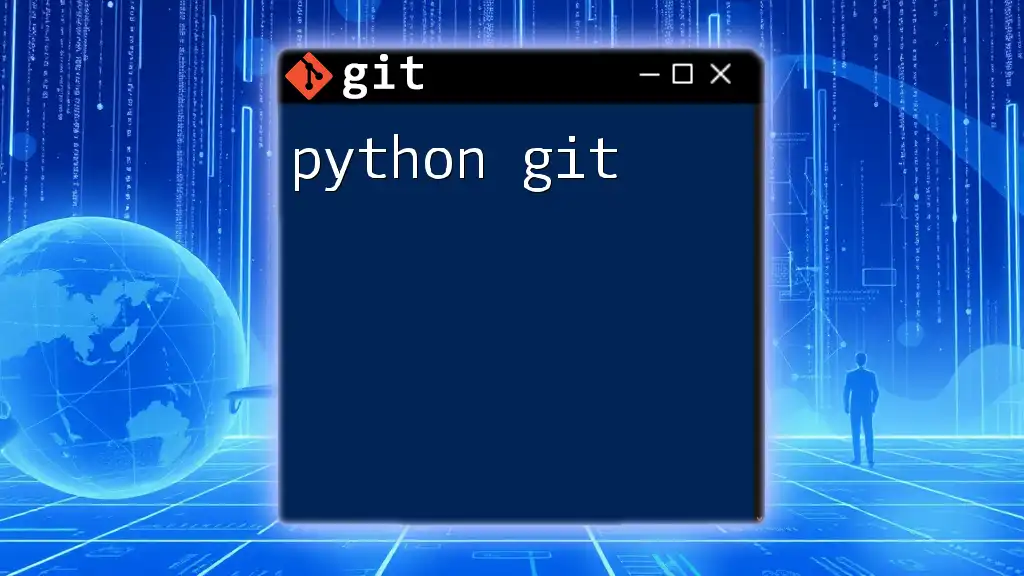
Exploring Other Uses of "git show"
Viewing Tags
Git tags are vital for marking specific points in the repository’s history, often corresponding to release versions. To examine a tag’s details, utilize:
git show v1.0
Here, “v1.0” refers to the tag name. The output includes information on the commit associated with the tag and its respective metadata, allowing you to trace back to milestones in project development.
Viewing Branch Information
If you aim to check what the latest commit in another branch looks like, you can use:
git show branch-name
This command will present the latest commit details for the specified branch, allowing team members to stay informed about ongoing developments in other parts of the project without switching branches.
Viewing Stashes
Git stashes are a mechanism for saving uncommitted changes temporarily. To explore details of a specific stash, you can run:
git show stash@{0}
This command displays information about the most recent stash, including which files were altered. Understanding stashes can significantly benefit developers working with experimental features or various stages of an application where changes are not yet ready to be committed.
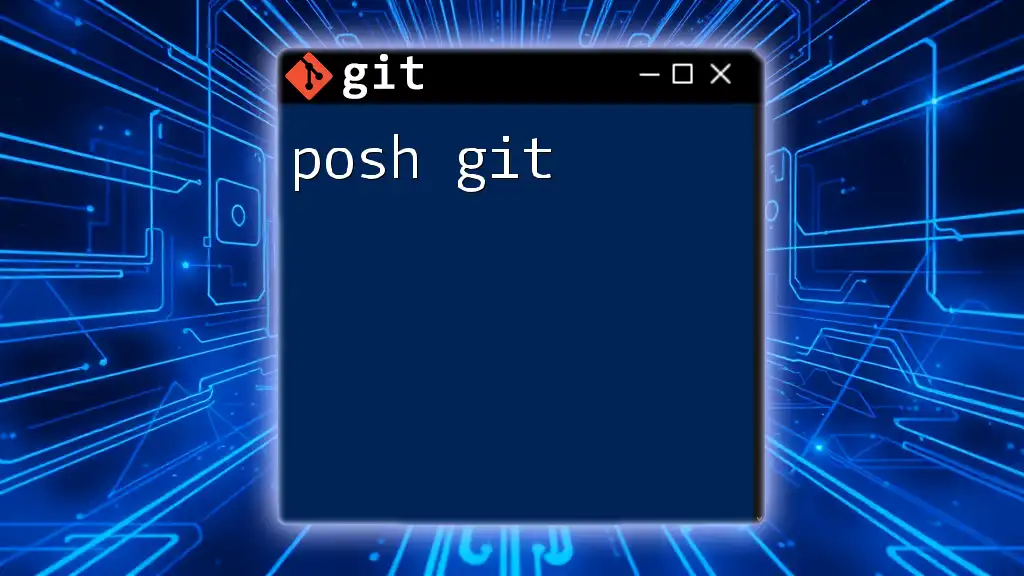
Options and Variations of git show
Common Options with git show
While the basic “git show” command is powerful, incorporating options can enhance its functionality. Some notable options include:
- `--pretty`: Customize the format of the output (e.g., `--pretty=short`).
- `--name-only`: List only filenames affected by a commit.
- `--patch`: Show the detailed diff of changes made.
These options allow developers to tailor the command usage to fit various development needs efficiently.
Combining Commands
For more detailed output, you can combine several options. For instance, to get a concise view of the commit message along with a list of modified files, you could execute:
git show --pretty=short --name-only 1234567
The combined output will effectively provide a snapshot of the commit, summarizing critical details and related files succinctly.
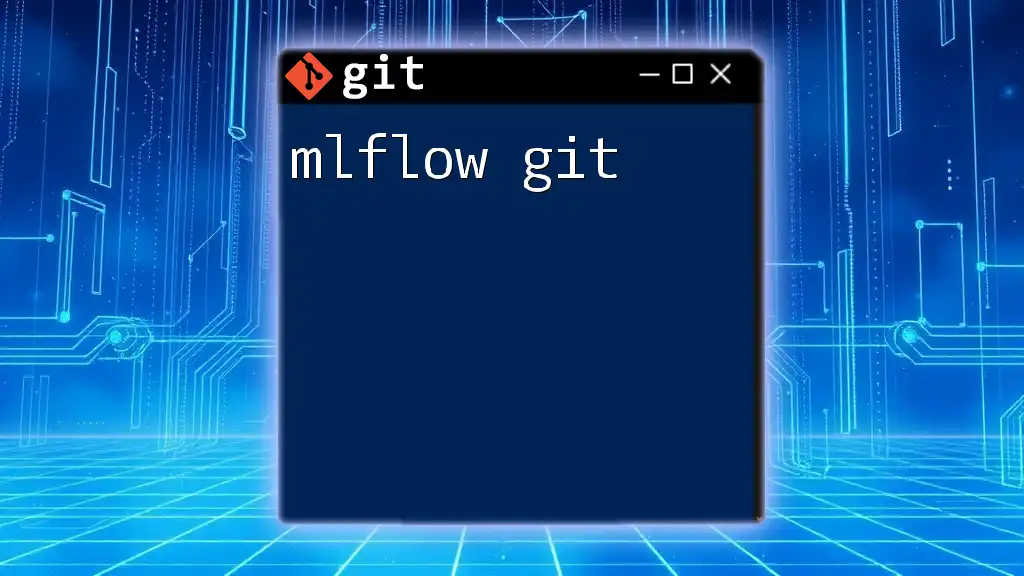
Best Practices for Using "git show"
When to Use git show
The “git show” command becomes particularly valuable in certain situations, such as:
- When documenting code changes for team members or new hires.
- Before applying a merge or rebase, to ensure understanding of what changes are being integrated.
Avoiding Overload of Information
While the “show” command can produce extensive information, it’s important to manage this output to avoid overwhelming yourself. Focus on filtering unnecessary details by using specific options like `--name-only` or `--pretty`. A clear, concise output fosters better understanding and decision-making.
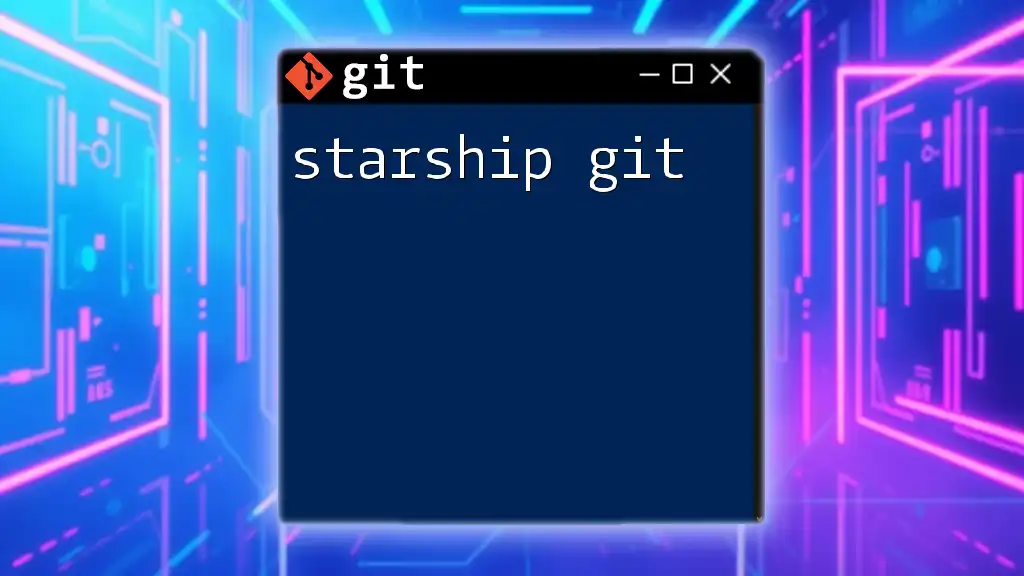
Conclusion
The versatility of the “git show” command makes it an indispensable tool in the Git toolbox. Whether you’re navigating through commit history, examining changes, or managing branches and stashes, mastering this command will enhance your productivity and understanding of Git workflows. Consider practicing with “git show” in your own projects to develop fluency in this essential command.
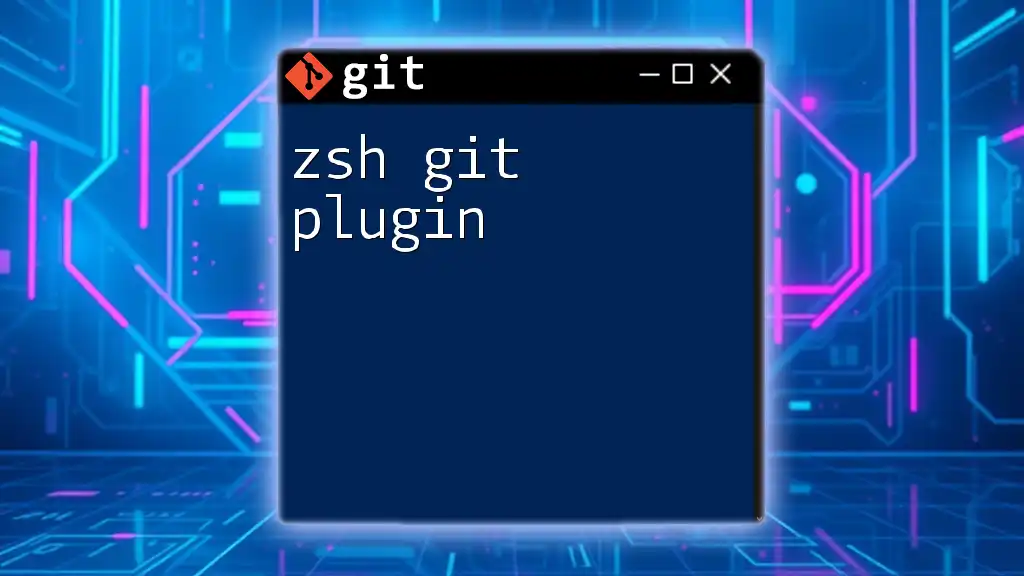
Additional Resources
For further exploration, consider diving into the [Git Documentation](https://git-scm.com/doc) or [tutorials](https://git-scm.com/docs/gittutorial) that delve deeper into other Git functionalities, ensuring a comprehensive understanding of this powerful version control system.