Git consists of three main parts: the working directory, the staging area, and the Git directory, each serving distinct roles in version control.
# View the status of your working directory and staging area
git status
What is Git?
Git is a powerful version control system designed to handle both small and large projects efficiently. It tracks changes in your files and enables collaboration with multiple contributors. The parts of Git include a variety of essential concepts and tools that empower developers to manage their code seamlessly.
Understanding Git is crucial for any developer, as it enhances their workflow by providing:
- Version control: Keep a historical record of code changes.
- Collaboration: Work concurrently with team members without overwriting each other's work.
- Branching: Experiment with new features while maintaining a stable main codebase.
Some key terms to familiarize yourself with when discussing the parts of Git are repositories, commits, branches, and the staging area.
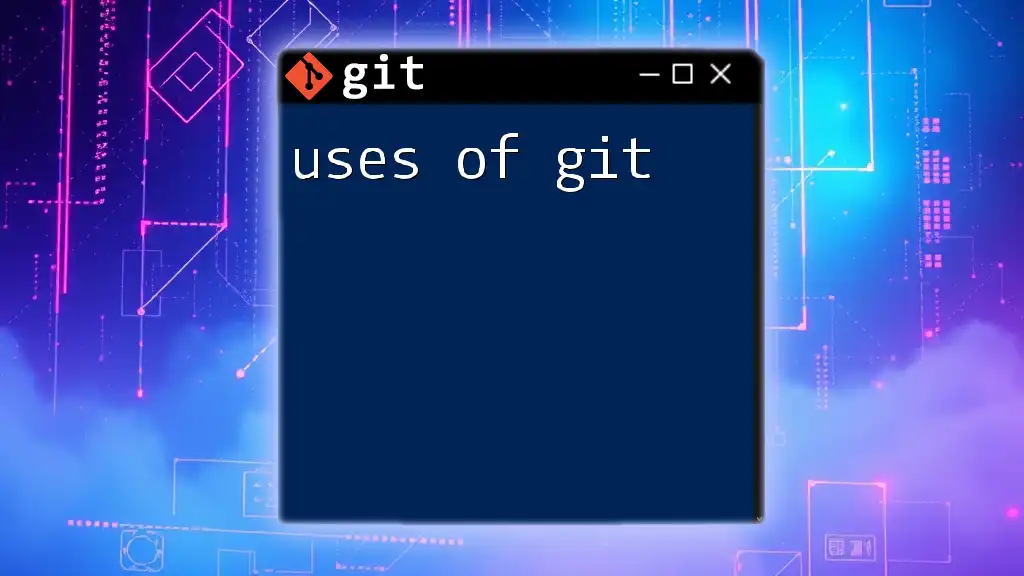
Components of Git
Git Repositories
A repository in Git is essentially a storage space for your project. It contains all the files and directories related to the project, as well as the complete history of changes made to those files. There are two primary types of repositories:
- Local Repositories: Stored on your personal machine where you track changes on a project without the need for an internet connection.
- Remote Repositories: Hosted on platforms like GitHub, GitLab, or Bitbucket that allow multiple users to access and collaborate on a project.
Creating a new repository is straightforward. Use the following command to initialize one in your desired directory:
git init <repository-name>
This command sets up your file structure to begin versioning your project.
Git Commits
A commit is a fundamental part of Git that records changes made to your files. Each commit acts as a snapshot of your project at a specific point in time. When making a commit, you must provide a commit message that succinctly describes the changes you've implemented. This practice not only helps you keep track of project history but also assists other collaborators in understanding the project's progress.
Here's how to make your first commit:
-
Add changes to the staging area with:
git add <file-name>
-
Next, create a commit with a meaningful message:
git commit -m "Initial commit"
A good commit message should be focused and informative, often following a format like "What changed? Why did it change?"
Git Branches
Branches are crucial to managing different lines of development within your project. They allow for parallel development, letting you work on new features, bug fixes, or experiments without affecting the main codebase.
To create a new branch, you can use:
git branch <branch-name>
Switching between branches is equally simple:
git checkout <branch-name>
After you've completed your work in a branch, you can merge it back into the main branch using:
git checkout main
git merge <branch-name>
This merges the changes from your feature branch into the main branch, ensuring that your main codebase is up-to-date with your latest developments.
Git Staging Area
The staging area acts as a buffer between your working directory and the repository. It allows you to prepare changes before committing them. By moving files to the staging area, you're deciding which changes to include in your next commit.
To stage files, you would use the command:
git add <file-name>
By staging changes meticulously, you control what gets included in your commit, keeping your history clean and meaningful.
Git Remote
Remote repositories are essential for collaboration. They enable you to store your project on external platforms while allowing others to contribute. Remotes help synchronize your local repository changes with the centralized version.
To view your current remote repositories, you can run:
git remote -v
If you want to add a new remote repository, use:
git remote add origin <remote-url>
Once you've made changes locally, you can push those updates to the remote repository with:
git push origin <branch-name>
This command reflects your local commits on the remote server, keeping your team updated about ongoing changes.
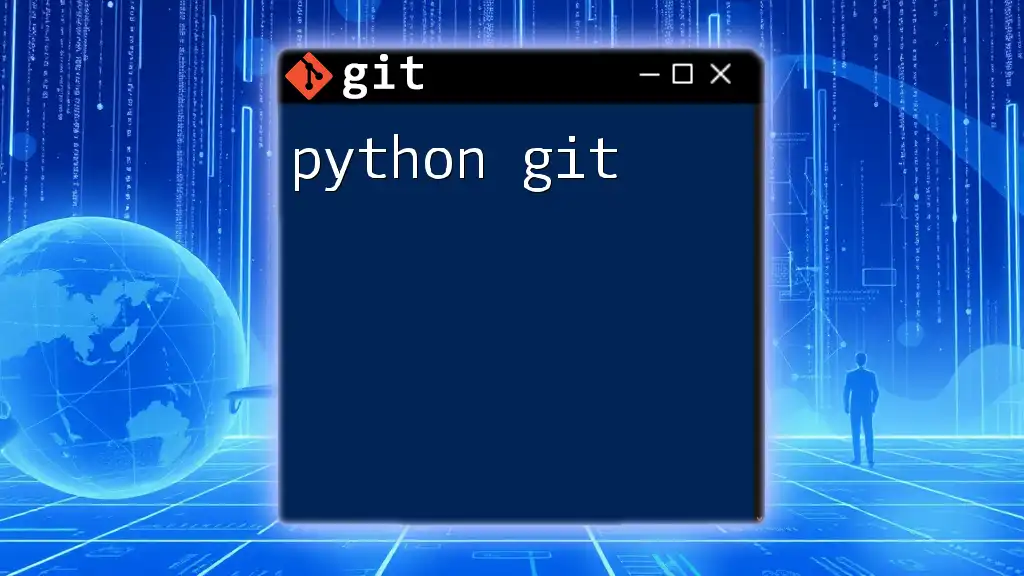
Understanding Git Workflows
A Git workflow describes the process by which developers propose, manage, and review changes. There are various workflows, each with its own advantages and challenges:
- Feature Branching: Developers create a new branch for each new feature. This keeps the main branch stable while ongoing work is happening in isolation.
- Gitflow Workflow: More structured, this model allows for different types of branches like feature branches, hotfix branches, and release branches.
- Forking Workflow: Commonly used in open-source projects, where developers fork a repository, work on changes, and then submit pull requests for integration.
Choosing a workflow depends on team size, project complexity, and the specific requirements of your development environment.
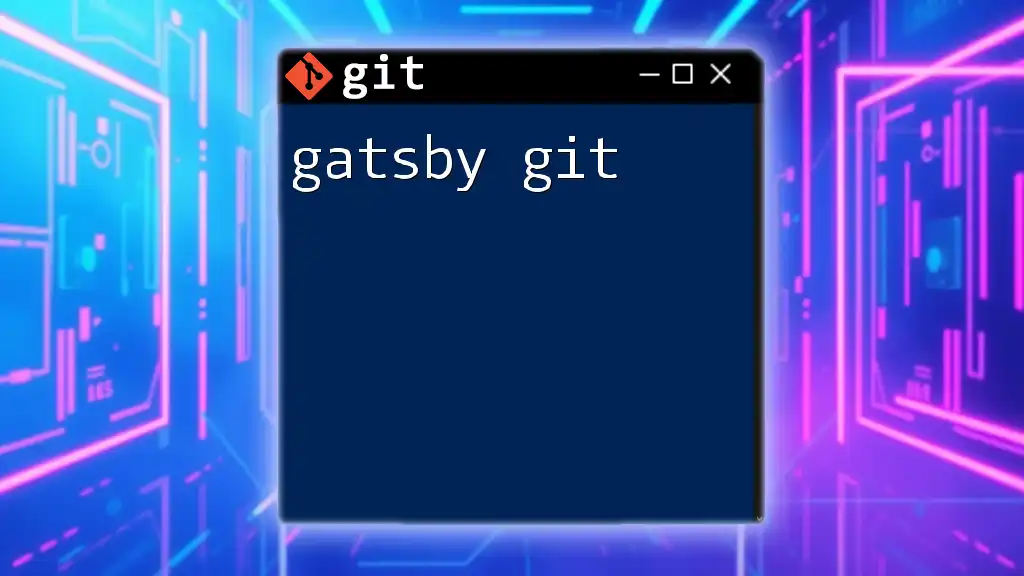
Git Configuration
Configuring Git is fundamental to ensure seamless interaction with your projects. This configuration process involves setting up your user information, which is recorded in each commit. To set your username and email, execute:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
You can verify your configuration settings with:
git config --list
Proper configuration allows for clear attribution of contributors in collaborative projects, making it easier to track contributions.
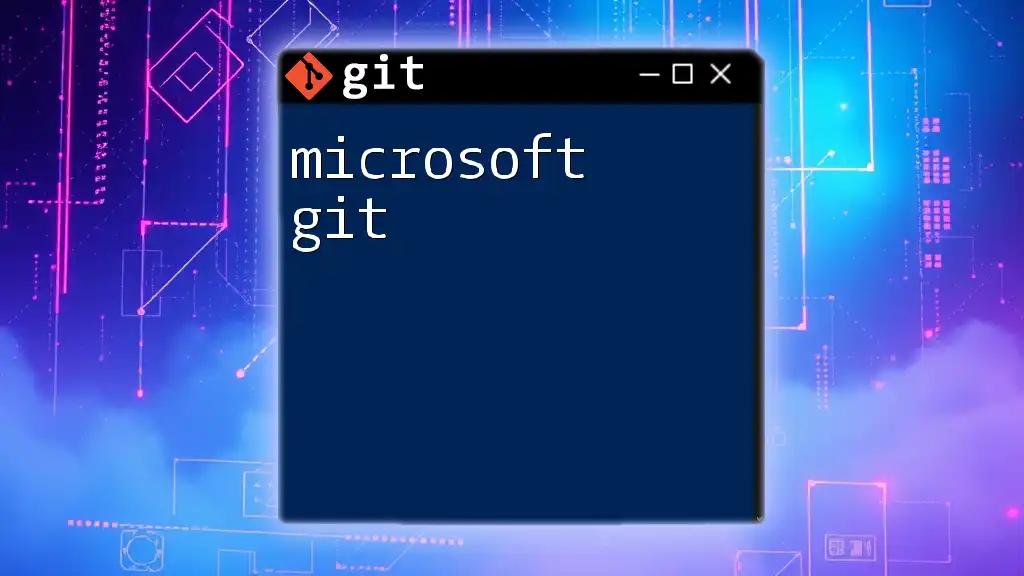
Conclusion
Understanding the parts of Git is essential for any developer. By grasping the concepts of repositories, commits, branches, the staging area, and remote repositories, you can navigate Git more effectively and manage your projects with confidence. The knowledge of Git workflows and proper configuration will further enhance your collaboration opportunities.
Now that you have a foundational understanding, don't hesitate to explore Git further. Experiment with commands, practice branching, and share your experiences. Happy coding!
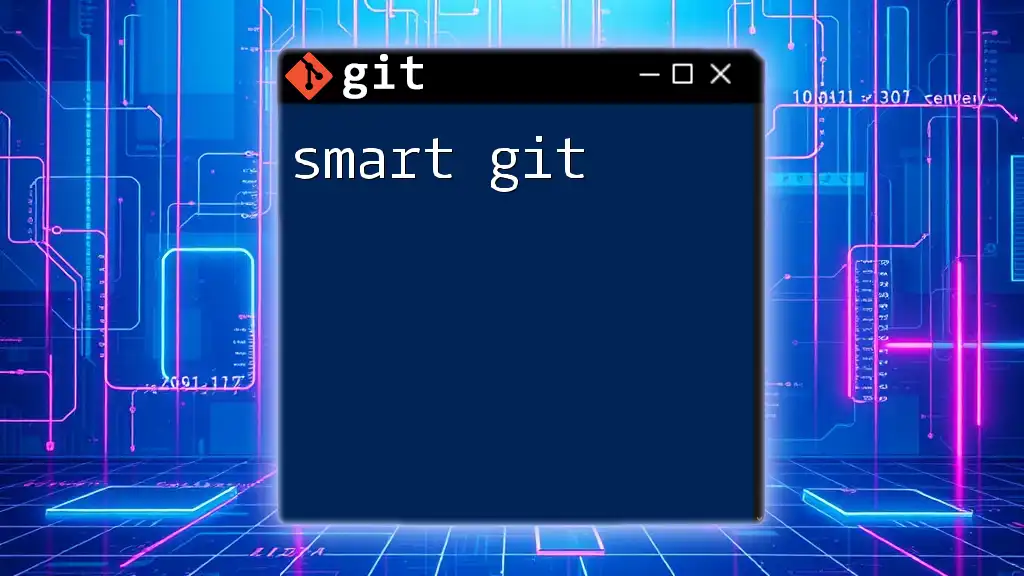
Further Resources
To deepen your understanding, consider exploring the following resources:
- Official Git Documentation
- Online Git tutorials and courses
- Books and community forums focused on version control and collaborative development.