Microsoft Git refers to the integration of Git version control within Microsoft tools like Visual Studio and Azure DevOps, facilitating streamlined development workflows for programmers.
Here's a basic Git command snippet to initialize a new repository:
git init my-new-repo
Setting Up Git with Microsoft Tools
Installing Git on Windows
To begin your journey with Microsoft Git, the first step is to install Git on your Windows machine. Here’s how you can do it seamlessly:
- Download Git: Visit the [Git for Windows](https://gitforwindows.org/) website and download the latest version of Git.
- Installation Process: Run the installer and follow the prompts. Most users can stick with the default settings.
- Configuring Your Git Environment: Once the installation is complete, it’s crucial to configure your Git environment to record your identity. This will help in attributing your commits.
Example Code: Basic Git Configuration
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
This setup ensures every commit is associated with your name and email, essential for collaborative projects.
Integrating Git with Visual Studio
Visual Studio offers robust integration with Git, enhancing productivity for developers who prefer a visual interface.
-
Creating a New Repository: Open Visual Studio and navigate to the "Team Explorer." Select “Connect” then “New” under “Local Git Repositories.” This creates a new Git repository for your project.
-
Cloning an Existing Repository: To work on an existing project, you can clone a repository using the “Clone” option in the “Team Explorer.” Just paste the repository URL and select your local directory.
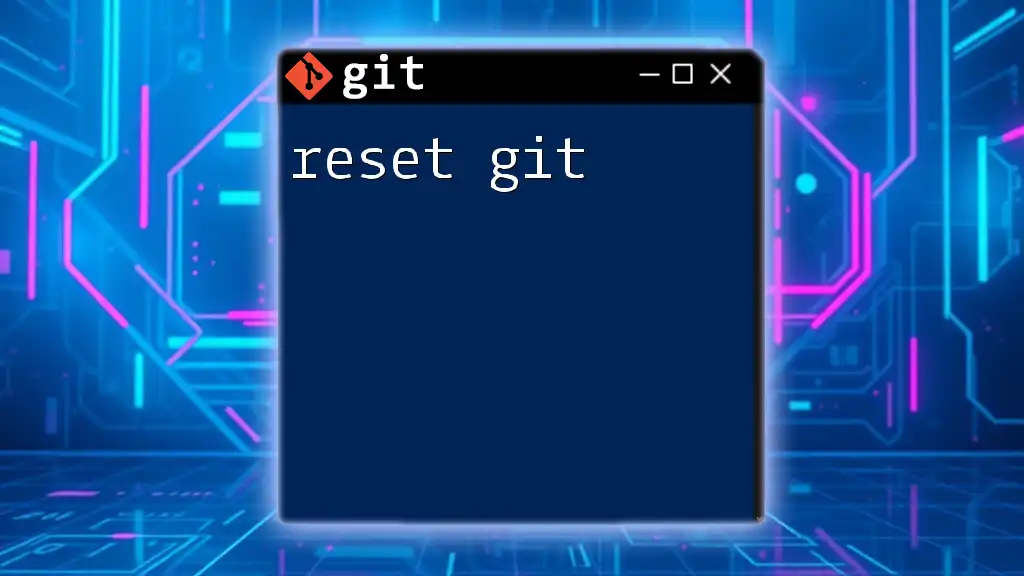
Essential Git Commands for Microsoft Developers
Fundamental Git Commands
Understanding the fundamentals of Git is key to mastering version control in software development.
Creating a New Repository: If you’re initializing a project from scratch, use the following command to create a new repository:
git init my-repo
This command sets up a new Git repository in a directory named `my-repo`.
Cloning a Repository: To get a project from a remote repository, clone it using:
git clone https://github.com/username/repo.git
This command copies all the files and the entire history of the repository to your local machine.
Adding Changes: To stage your changes, use the command below. This prepares your tracked changes for commit.
git add .
Committing Changes
Once you’ve made changes to your files, it’s important to commit them. A good commit message summarizes what has changed. This helps both you and your collaborators understand the history of the project.
Command Example:
git commit -m "Initial commit"
Using clear and descriptive messages is crucial for maintaining an understandable project history.
Branching and Merging
Branches allow you to work on different features or fixes independently from the main codebase, which can be a powerful part of your workflow.
Understanding Branches: When you create a branch, you’re essentially creating a snapshot in time to work on features independently.
Commands for Creating and Merging Branches:
To create a new branch, use:
git branch my-feature
Switch to the new branch with:
git checkout my-feature
When you’re ready to integrate your feature into the main branch, merge it with:
git merge my-feature
Advanced Git Features in the Microsoft Ecosystem
Pull Requests with Azure DevOps
Pull requests are fundamental for code review and collaboration in teams. With Azure DevOps, creating a pull request encourages collaboration and improves code quality.
How to Create a Pull Request in Azure DevOps: Navigate to your repository in Azure DevOps, select "Pull Requests," and then "New Pull Request." Follow the prompts to specify the source and target branches and add a descriptive title and comments.
Using Git with GitHub Codespaces
GitHub Codespaces provides a cloud-based development environment, which allows developers to work without local setup impediments.
When you set up a Codespace for your project, it automatically loads your repository, enabling you to code instantly in a fully-configured environment. You can leverage the same Git commands in Codespaces like you would locally.
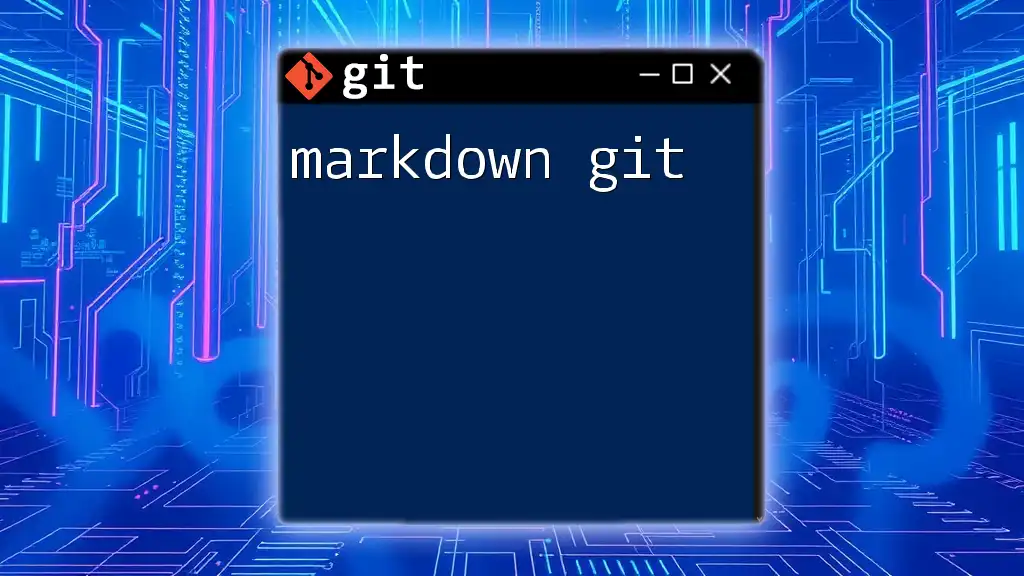
Troubleshooting Common Git Issues in Microsoft Environments
Resolving Merge Conflicts
Merge conflicts occur when changes in different branches clash. Understanding how to resolve these is vital for effective collaboration.
When a conflict arises, Git will mark the files that need attention. Using Visual Studio, you can visually resolve conflicts by selecting the changes you want to keep.
Common Git Error Messages and Solutions
Being familiar with common Git errors can save you time.
- Error: `fatal: destination path 'repo' already exists`
This error indicates that the directory you are trying to clone already exists. To solve this, you can either remove the existing directory or clone the repository into a new directory.
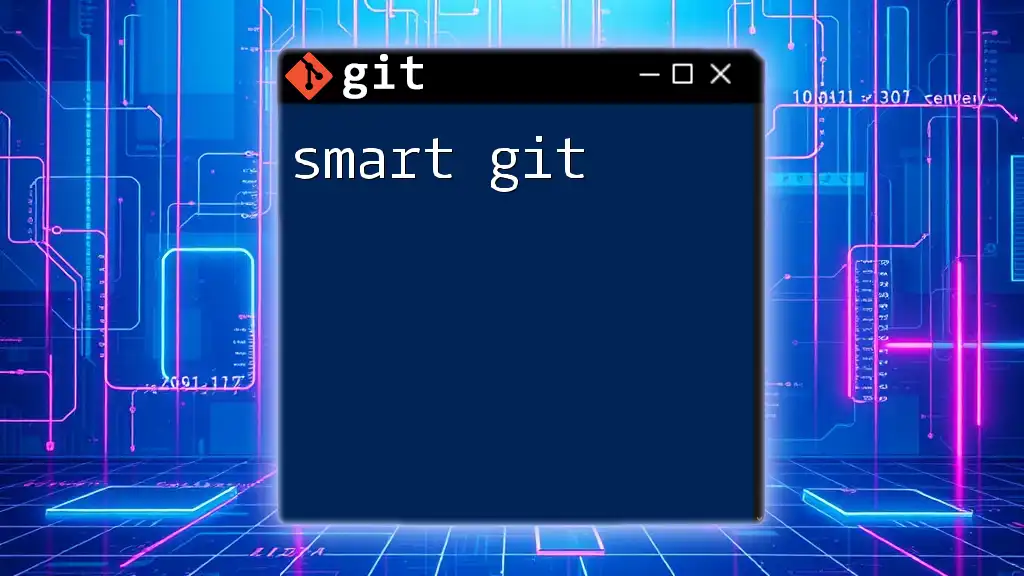
Best Practices for Using Git in Microsoft Projects
Writing Effective Commit Messages
Effective commit messages are essential for a clear project history. Aim for a structure that includes a short summary followed by a detailed description where necessary. This helps future developers understand the purpose of changes quickly.
Regularly Syncing with Remote Repositories
To ensure you’re working with the latest code, it's crucial to regularly sync your local repository with the remote one.
Commands for Synchronization:
To fetch updates from the remote repository, use:
git fetch
To incorporate the changes into your local branch, execute:
git pull
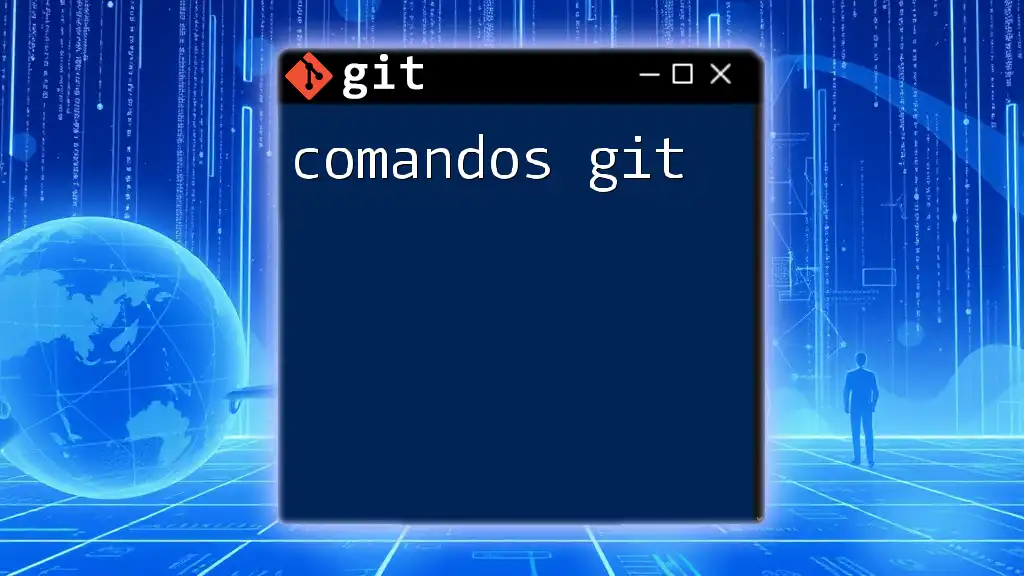
Conclusion
By understanding Microsoft Git and incorporating its features into your workflow, you’re well on your way to becoming proficient in using version control systems. Remember to practice continually, as hands-on experience is key to mastering Git.
For further information, consider exploring Microsoft’s Git documentation and various online learning platforms available to sharpen your skills in Git and version control practices.