Godot Git integration allows developers to seamlessly manage version control for their projects directly within the Godot engine using Git commands.
git init # Initialize a new Git repository
git add . # Stage all changes for commit
git commit -m "Initial commit" # Commit the staged changes with a message
git push origin main # Push the committed changes to the remote repository
Setting Up Your Godot Project with Git
Initializing a Git Repository
The first step in establishing Godot Git integration is creating a Git repository for your project. This repository will track changes to your game files, enabling you to revert to earlier versions if needed.
To initialize a Git repository in your Godot project, open the terminal and navigate to your project directory. You can do this with the `cd` command:
cd /path/to/your/godot/project
Next, initialize the Git repository:
git init
This command creates a hidden `.git` directory which will store all of your version control data.
Creating a `.gitignore` file is essential next. This file prevents Git from tracking unnecessary files that Godot generates. In your project directory, create a `.gitignore` file and add common patterns to it:
.import/
.godot/
*.tmp
This ensures that temporary files and directories created by Godot do not clutter your repository.
Connecting to Remote Repositories
Connecting your local Git repository to a remote one allows you to collaborate with other developers or back up your project online. Tools like GitHub, GitLab, and Bitbucket offer such remote repositories.
If you haven't yet created a remote repository, go to your chosen Git hosting service and create a new repository. Once that is done, link it to your local repository. Use the following command to add your remote repository:
git remote add origin <repository-url>
Replace `<repository-url>` with the URL provided by your chosen Git hosting service. After setting this up, you can push your local commits to the remote repository using:
git push -u origin master
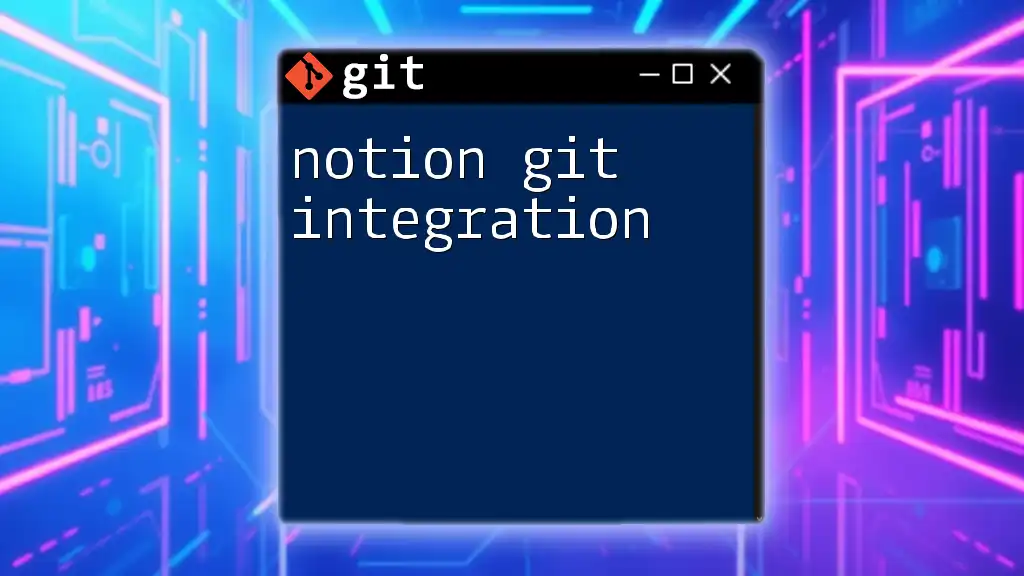
Essential Git Commands for Godot Developers
Cloning a Project
If you want to work on an existing Godot project stored in a Git repository, you can clone it to your local machine. This is done with the command:
git clone <repository-url>
This command makes an exact copy of the repository, including all branches and commit history.
Working with Branches
Branching is a vital part of the Git workflow, allowing you to develop features or fix bugs without affecting the main codebase. To create a new branch, use:
git checkout -b <branch-name>
Replace `<branch-name>` with a descriptive name reflecting the changes you plan to make.
Switching back to the main branch (usually `master` or `main`) can be done using:
git checkout master
Staging and Committing Changes
Once you make modifications to your project, you’ll want to commit those changes to your Git history. First, you need to stage your changes. You can add all changes with the command:
git add .
This stages all modified files for the next commit. After staging, commit your changes with a descriptive message about what you changed:
git commit -m "Your commit message"
Tip: Commit changes frequently and keep messages clear to make tracking your project's development easier.
Merging Branches
After completing a feature on a separate branch, you’ll want to merge it back into the main branch. First, switch to the main branch:
git checkout master
Next, perform the merge using:
git merge <branch-name>
If there are conflicting changes between the branches, Git will notify you, and you will need to resolve these conflicts before you can complete the merge.
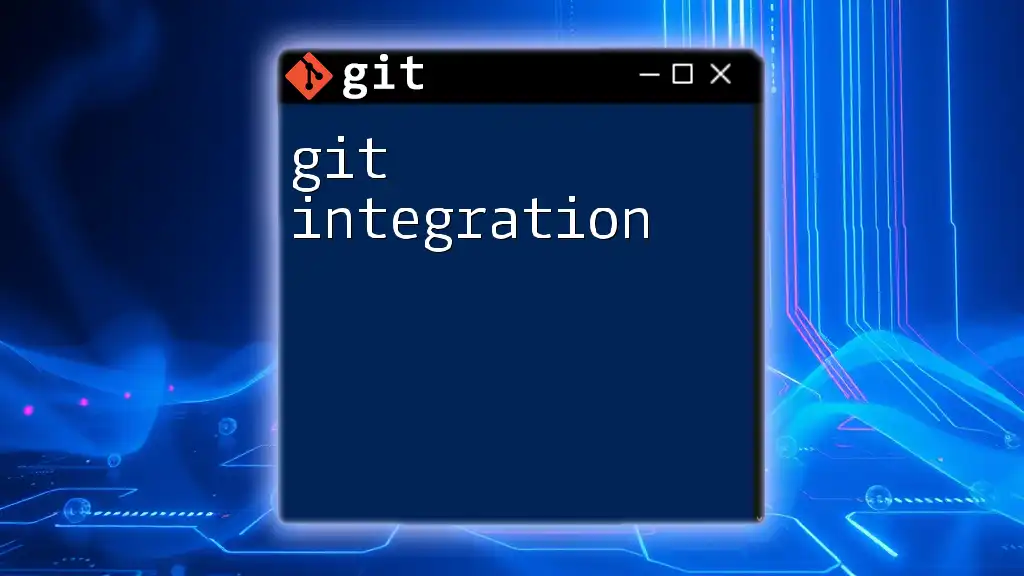
Best Practices for Using Git with Godot
Commit Often and Write Descriptive Messages
Adopting a habit of committing changes often helps you track your project’s development closely. By committing frequently, you can revert to earlier, known states of your project if needed. Always write meaningful commit messages that summarize the changes made; this will expedite understanding your project’s history when you revisit it in the future.
Using Tags for Versions
Tags are extremely useful for marking specific points in your project’s history, such as releases or important milestones. To tag your current commit, you can use:
git tag -a v1.0 -m "Version 1.0"
This tags the commit as version 1.0 and provides a message. Tags create a clean way to navigate between different versions of your game, allowing you to release a particular build easily.
Continuous Integration (CI) Strategies
Integrating CI tools can greatly enhance your workflow by automating testing and deployment processes. Services like GitHub Actions, CircleCI, or Travis CI can be configured to run tests every time you push changes to your repository. By implementing CI, you ensure that your code remains robust and functional as you develop your game.
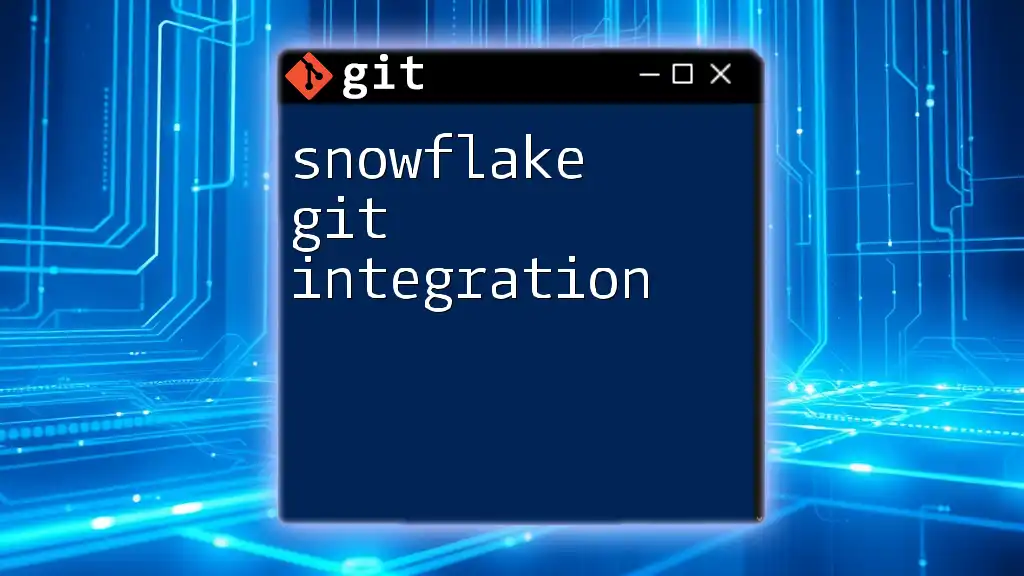
Troubleshooting Common Git Issues in Godot
Ignored Files That Shouldn’t Be
Sometimes you might want to track certain files that you forgot to add to your `.gitignore`. You can override the rules in `.gitignore` by using the `-f` option with the `git add` command:
git add -f <file-name>
This force adds the specified file, allowing you to track it without removing it from the `.gitignore`.
Handling Merge Conflicts
Merge conflicts can arise when changes in your branch clash with changes in the branch you’re merging into. When this happens, Git will notify you which files have conflicts. Open those files, look for the conflict markers, and manually resolve the discrepancies by choosing which change to keep or combining the changes sensibly.
Once resolved, mark the files as resolved using:
git add <file-name>
Complete the merge with:
git commit
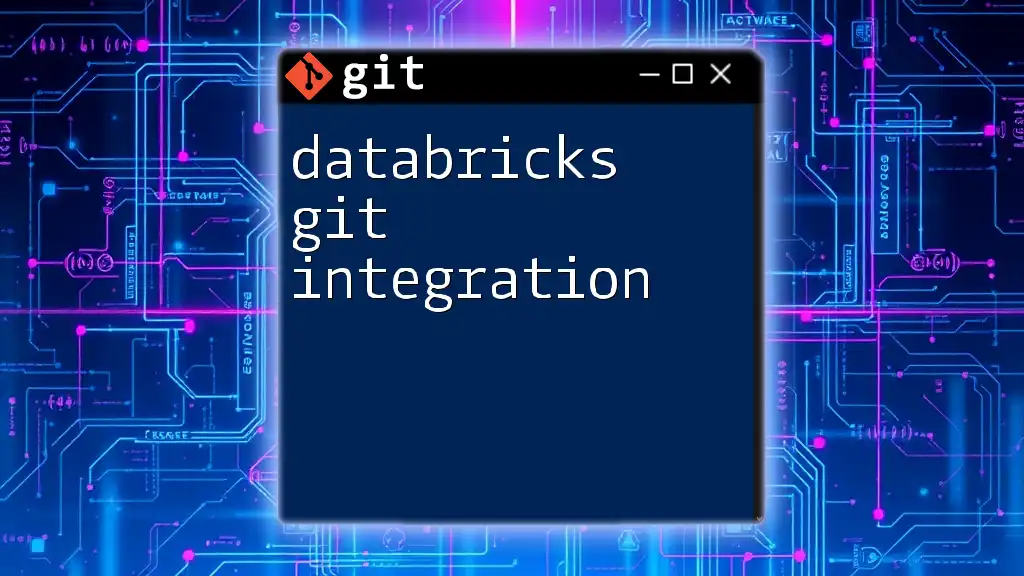
Additional Resources for Learning Git with Godot
To further enhance your understanding and mastery of Godot Git integration, consider checking out various online courses, tutorials, and community forums. The official Git documentation is a valuable resource for in-depth learning about version control. Additionally, participating in Godot community forums can provide support, tips, and tricks that are specific to game development.
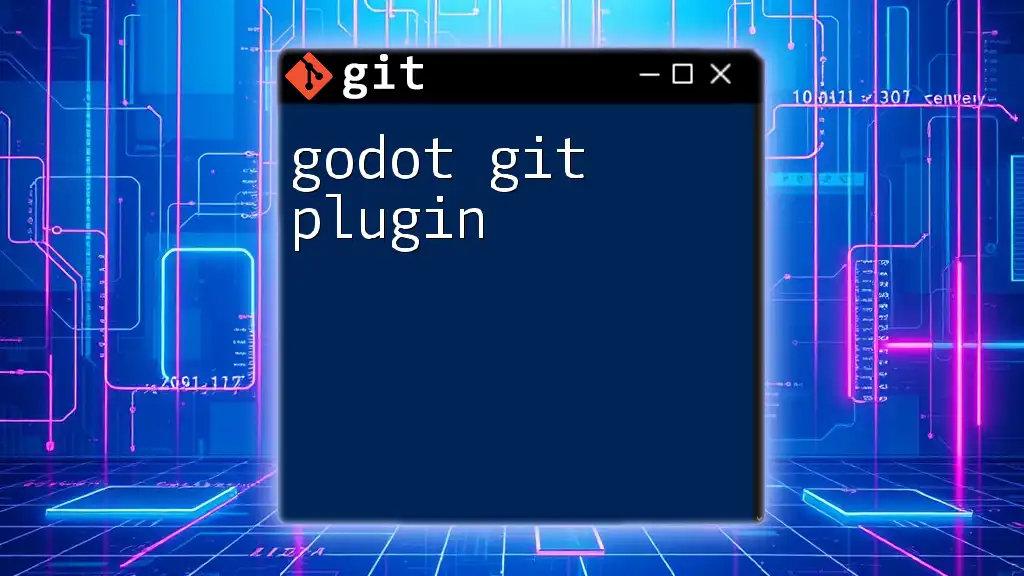
Conclusion
Integrating Git with Godot significantly enhances your development workflow by providing a systematic approach to version control. By following the steps outlined above and adopting best practices, you can ensure that your projects are organized, collaborations are seamless, and your game development process is efficient. Embracing these tools and practices will ultimately lead to a stronger, more scalable game development process.