Git is a distributed version control system that allows multiple developers to track changes in source code during software development.
Here’s an example of a basic git command to initialize a new repository:
git init
Understanding Version Control
Definition of Version Control
Version control is a system that records changes to a file or a set of files over time so that you can recall specific versions later. This is incredibly important in software development as it allows multiple developers to work on the same codebase simultaneously without overriding each other’s changes. With version control, you can keep track of every modification, revert to previous states, and collaborate effectively by merging contributions.
Types of Version Control Systems
Version control systems can be broadly categorized into two main types:
-
Centralized Version Control Systems (CVCS): In this model, there is a single central repository that all users access. Changes are made directly to this central repository, making it easier for users to see the history of changes but problematic as it becomes a single point of failure.
-
Distributed Version Control Systems (DVCS): Git falls under this category. In DVCS, each user has a complete copy of the repository on their local machine, allowing them to work offline. Changes can be committed locally and later pushed to a remote repository. This decentralization enhances collaboration and reliability.
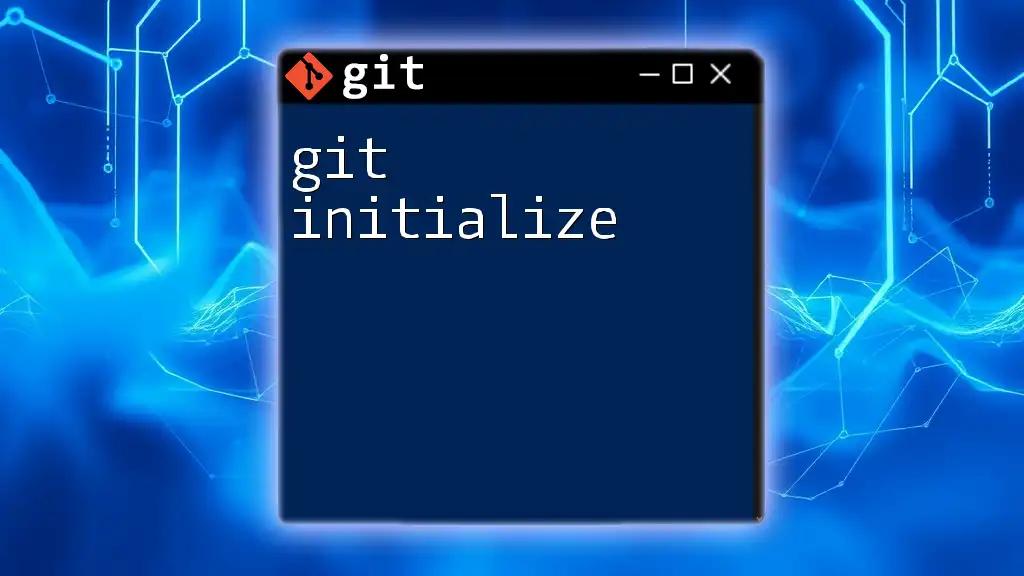
What is Git?
Definition of Git
Git is an open-source, distributed version control system designed to track changes in source code during software development. Created by Linus Torvalds in 2005, Git has evolved to become one of the most widely used VCS globally due to its speed and flexibility. Developers appreciate Git's ability to handle large projects and maintain a clean commit history, which is crucial for collaboration and deployment.
Key Features of Git
-
Distributed Nature: Unlike centralized systems, Git allows developers to work with a full repository on their computers. This means they can commit local changes, revert to previous versions, and create branches without a network connection.
-
Data Integrity: Each file and change in Git is tracked with a SHA-1 hash. This ensures that data integrity is checked constantly, as any alteration in the content will lead to a different hash. This mechanism guarantees that files have not been tampered with.
-
Branching and Merging: One of Git’s standout features is its branching model. Developers can create multiple branches to experiment without affecting the main codebase. Once the changes are ready, they can be merged back, ensuring that the main branch remains stable.
-
Speed: Git is designed for performance. Most operations, such as committing changes and creating branches, are performed locally, which makes them extremely fast compared to systems that rely on a central server.
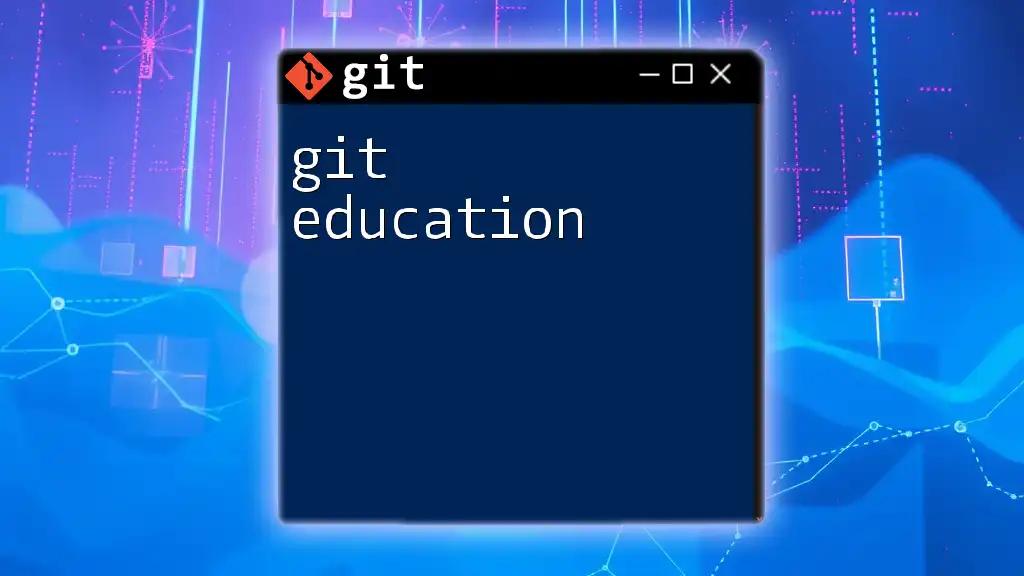
The Architecture of Git
Git Objects
Git stores data in three types of objects:
-
Blobs: A blob (binary large object) represents file data. It holds the actual contents of a file without any metadata like filenames or directories.
-
Trees: Trees are Git's way of organizing blobs. A tree object can link to multiple blobs (the actual files) and can also link to other trees (representing directories).
-
Commits: Every commit in Git represents a snapshot of the repository at a given point in time. Each commit points to its parent commit, forming a chain of changes that establish the project’s history.
Repositories
In Git, the concept of a repository is fundamental:
-
Local vs Remote Repositories: A local repository exists on a developer's machine, while a remote repository is hosted on a server (like GitHub or GitLab). Developers typically clone a remote repository to create a local repository for their work.
-
Cloning Repositories: Developers use cloning to create a copy of a remote repository on their local machine with the command:
git clone <repository-url>
Cloning enables them to start contributing to the project quickly and easily.
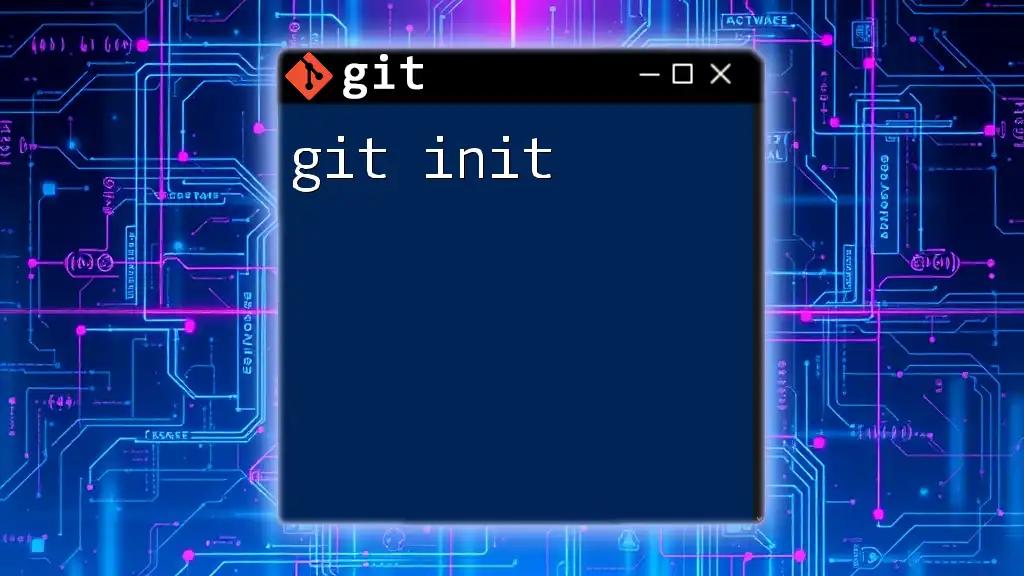
Basic Git Commands
Initializing a Git Repository
To begin tracking your project with Git, you need to initialize a repository using the following command:
git init
This command creates a `.git` directory in your project, transforming it into a Git repository.
Cloning a Repository
When you want to get a local copy of an existing repository, you can use the clone command:
git clone <repository-url>
This command pulls all the files and commits from the specified remote repository to your local environment, allowing you to work on it instantly.
Tracking Changes
Adding Files
To track new or changed files, you must stage them using:
git add <file>
This command adds the specified file to the staging area, preparing it for the next commit, which acts like a 'checkpoint' in your project.
Committing Changes
Once your changes are staged, you can commit them with:
git commit -m "Commit message"
A commit represents a snapshot of your project at a specific point in time. Writing clear and descriptive commit messages is crucial as it helps other developers (and your future self) understand the context of changes.
Viewing History
You can view your project’s commit history with:
git log
This command provides a detailed list of commits, including the commit hash, author, date, and message. Understanding your commit history is vital for tracking project evolution and decision-making.
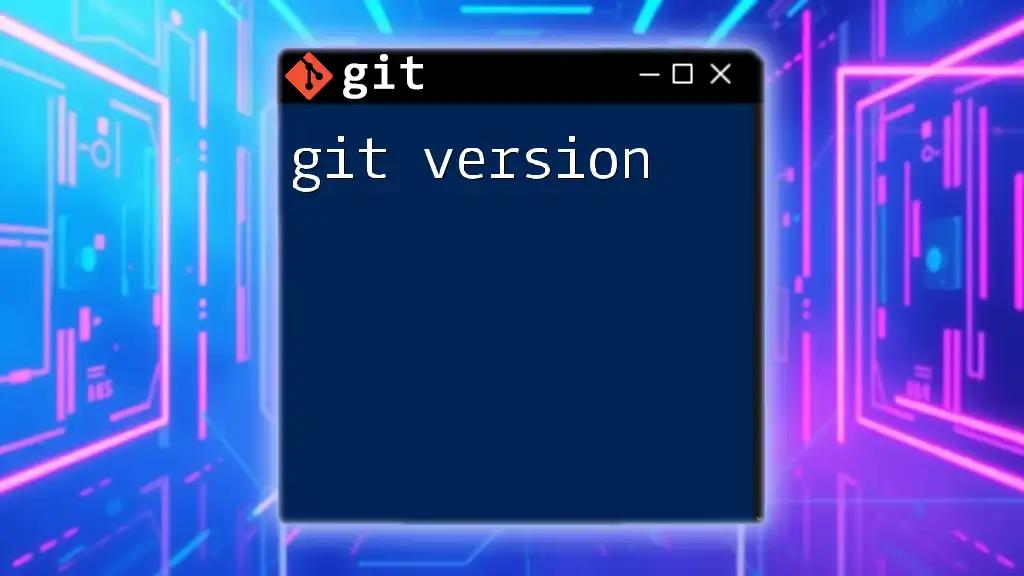
Conclusion
Understanding Git is fundamental for any modern developer. Version control systems like Git not only streamline collaboration but also safeguard your code against loss and mismanagement. Equipped with the power of Git, developers can coordinate more effectively, experiment freely, and maintain a meticulous history of their work. We encourage you to dive deeper into Git and leverage these powerful tools for your projects!
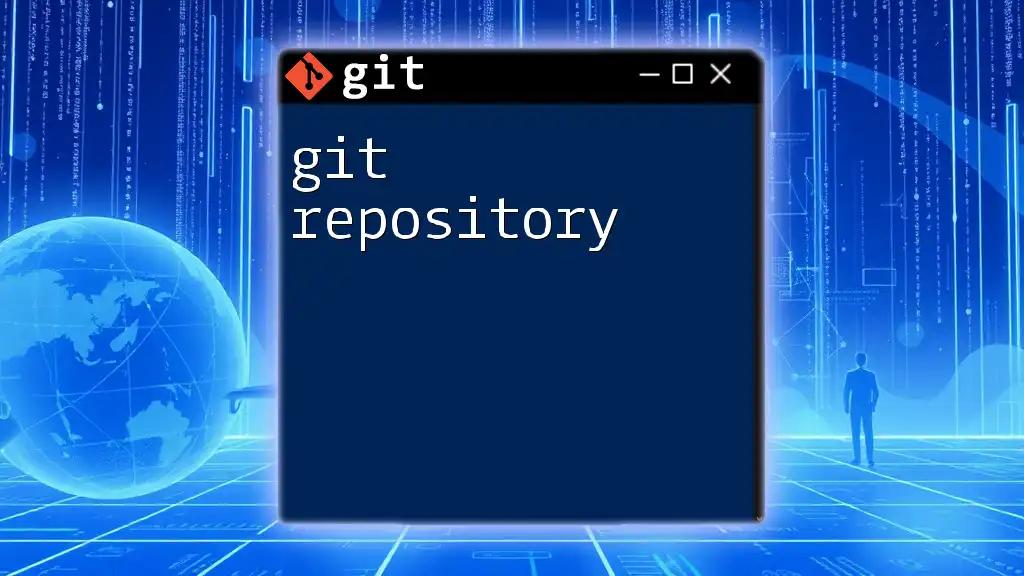
Additional Resources
For those eager to enhance their Git skills, consider referring to the official Git documentation, exploring Git GUI clients, and checking out various online tutorials. The more you practice using Git, the more proficient you will become, unlocking even greater potential in your software development journey.