The Git terminal is a command-line interface that allows users to interact with Git repositories, facilitating version control through various commands.
Here’s an example of a basic Git command to check the status of your repository:
git status
Understanding Git Terminal
What is Git?
Git is a distributed version control system that enables multiple users to work on a project simultaneously without interfering with one another’s changes. Originally developed by Linus Torvalds in 2005, Git is indispensable for modern software development, allowing developers to track changes, revert to previous states, and collaborate seamlessly across teams. Its ability to manage code effectively is pivotal in preventing data loss and streamlining workflows.
What is the Git Terminal?
The Git terminal acts as a command-line interface that allows users to interact with the Git version control system. Unlike graphical user interfaces (GUIs), the Git terminal offers a more direct and efficient way to execute commands. Familiarizing yourself with the terminal is essential for maximizing control over your projects, as it generally allows for faster execution of commands and more powerful features not available in GUI tools.
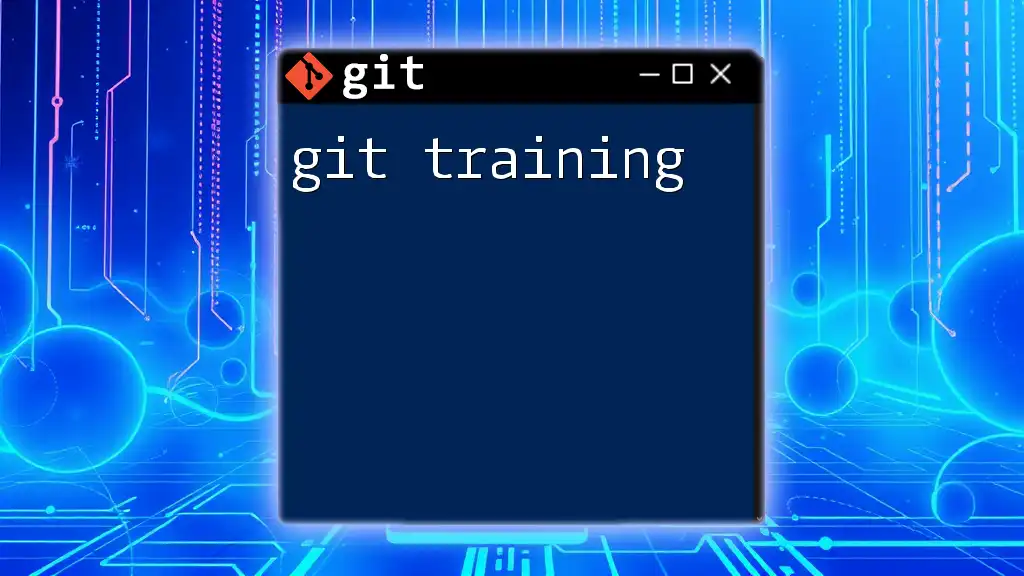
Setting Up Your Git Terminal
Installing Git
To start using Git in the terminal, you first need to install it.
-
Windows: Download the Git for Windows installer from the official website. Follow the installation prompts, ensuring you select options that suit your workflow.
-
macOS: If you have Homebrew installed, simply run:
brew install git
-
Linux: Use your package manager to install Git. For example, on Debian-based systems, you can run:
sudo apt-get install git
Configuring Your Git Environment
After installation, configuring your Git environment is the next vital step. This involves setting up your username and email, which are critical for your commit history and collaboration. Use the following commands in the terminal:
git config --global user.name "Your Name"
git config --global user.email "your_email@example.com"
It's important to have these details correct, as every commit you make becomes attributed to this identity.
Basic Commands Overview
Familiarity with fundamental Git commands can significantly enhance your productivity. Here’s a concise list of the core commands you should know:
- `git init`: Initializes a new Git repository.
- `git clone`: Copies an existing repository.
- `git add`: Stages changes for the next commit.
- `git commit`: Commits the staged changes to the repository.
- `git push`: Uploads local commits to a remote repository.
- `git pull`: Fetches and merges changes from a remote repository.
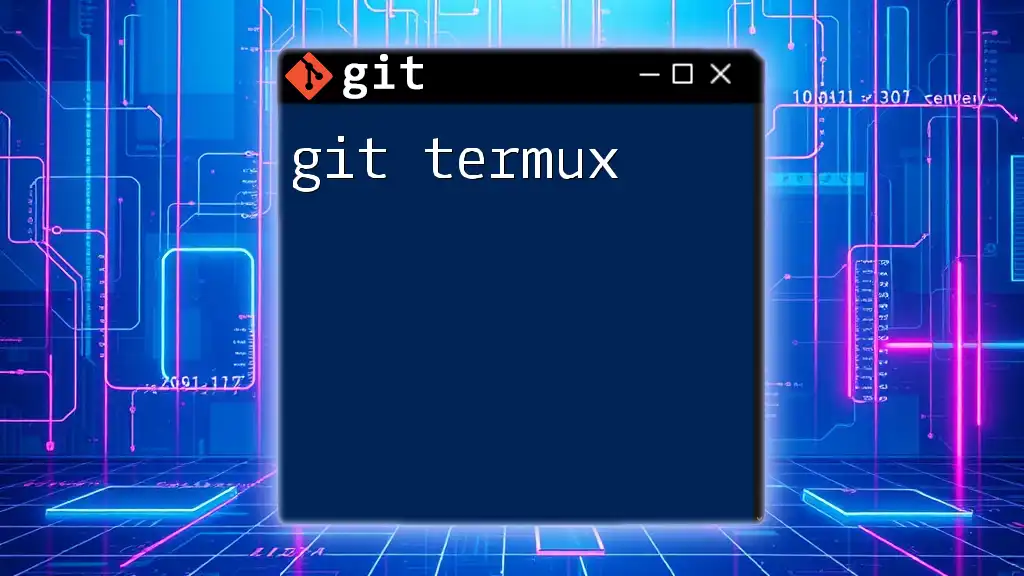
Navigating the Git Terminal
Basic Terminal Commands
Before diving into Git commands, you should know basic terminal navigation commands:
- `cd`: Change directory.
- `ls`: List files in the current directory.
- `pwd`: Print working directory.
Creating a New Git Repository
Creating a new repository is straightforward. You can initialize a new repository using the following commands:
mkdir my-project
cd my-project
git init
This creates a new directory named my-project and initializes an empty Git repository within it.
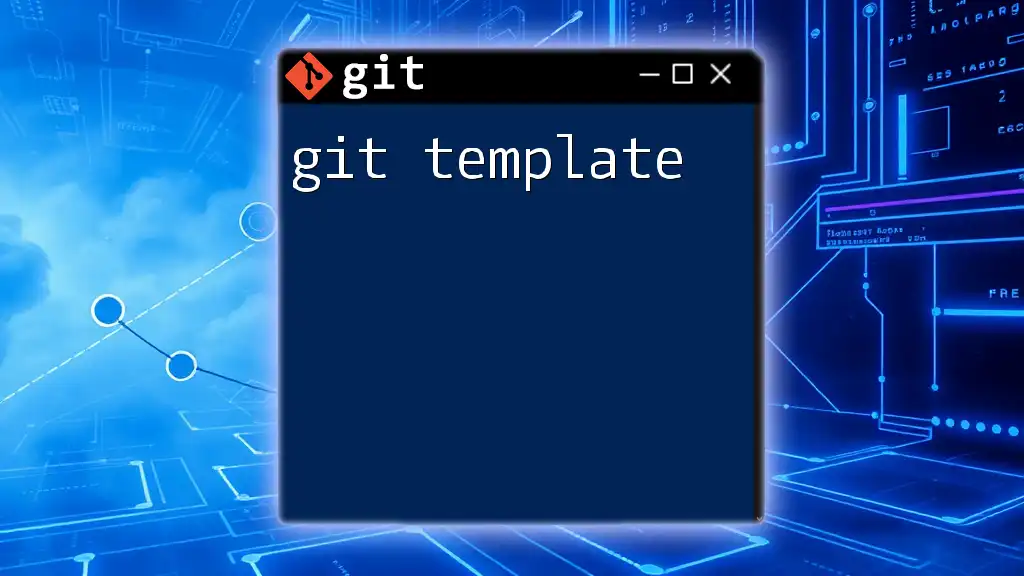
Core Git Commands Explained
Cloning a Repository
If you wish to work on an existing project, cloning is the way to go. It creates a local copy of a remote repository. The following command allows you to clone a repository:
git clone https://github.com/user/repository.git
On running this command, Git fetches the entire repository and sets it up for you to work on it independently.
Staging Changes
Staging is an intermediate step that allows you to review changes before committing them. To stage changes, use the `git add` command. You can specify individual files or use a dot (`.`) to stage all modified files:
git add filename.txt
git add .
Committing Changes
Once you have staged your changes, the next step is to commit them. This captures a snapshot of your tracked changes. Use the `-m` flag to include a concise message that describes the changes:
git commit -m "Your commit message here"
Effective commit messages are essential; they provide context for future reference and facilitate communication among team members.
Pushing Changes
After committing your changes locally, you may want to share them with others by pushing them to a remote repository. This is done using:
git push origin main
Here, `origin` refers to the remote repository, and `main` refers to the branch you are pushing to.
Pulling Changes
To keep your local repository up to date with changes made by others in the remote repository, you’ll use the `git pull` command:
git pull origin main
This command fetches the latest commits from the remote repository and merges them with your local branch.
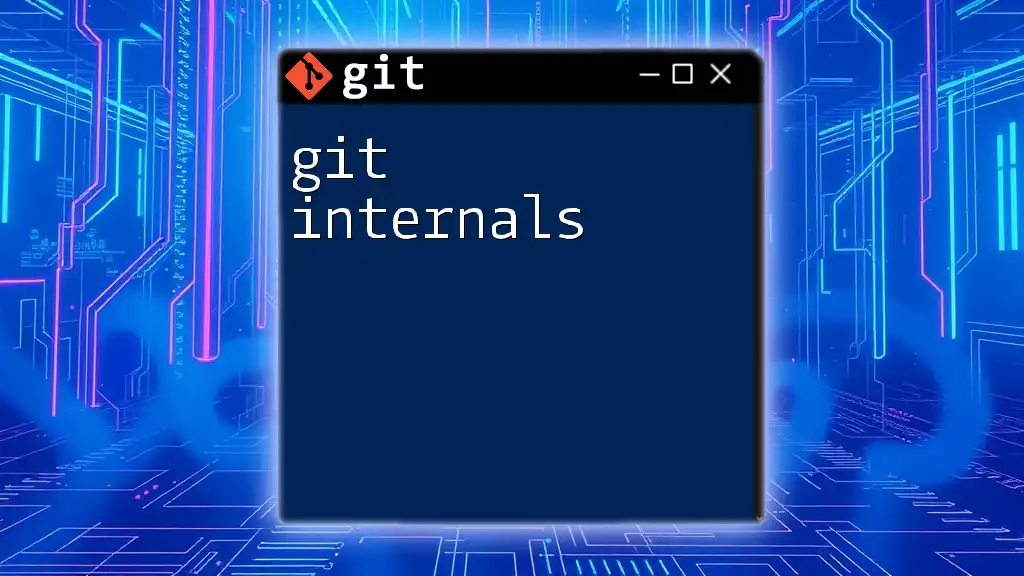
Branching and Merging
Understanding Branches
Branches in Git allow you to work on features or fixes without affecting the main line of development. This isolation makes it easier to experiment and iterate on changes.
Creating and Switching Branches
To create a new branch and switch to it, use:
git checkout -b feature-branch
This command creates a new branch named feature-branch and switches you to it in one go.
Merging Branches
Once you've made your changes in a feature branch, you might want to merge them back into the main branch. First, switch back to the main branch:
git checkout main
Then, merge your feature branch:
git merge feature-branch
During the merge, if there are conflicting changes, Git will prompt you to resolve them manually.
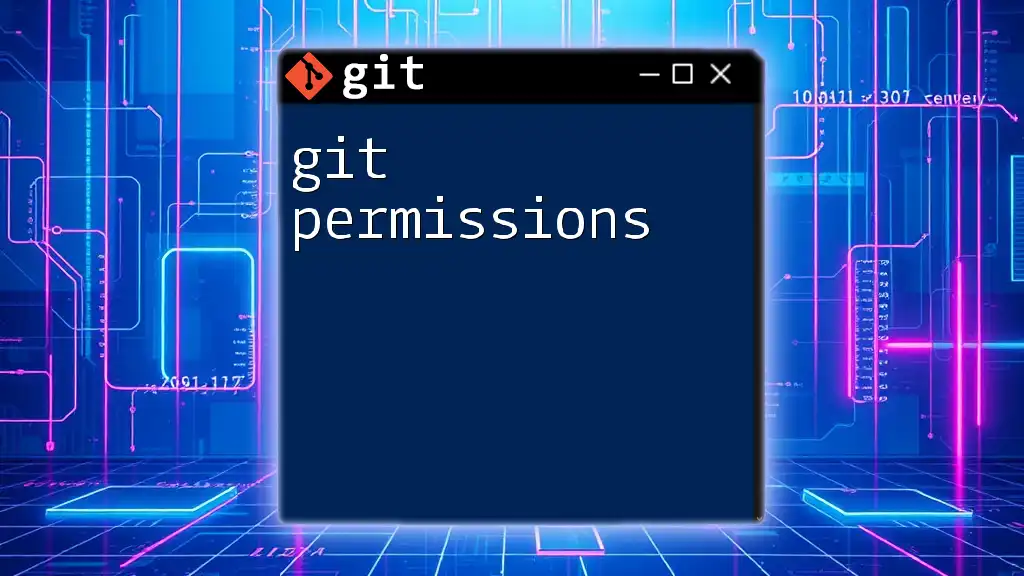
Advanced Git Terminal Commands
Viewing Your Commit History
To analyze your project's history, the `git log` command is invaluable. It displays the commit history in chronological order. You can customize the output using flags:
git log --oneline --graph
This command condenses the logs into a more visual representation and shows you a summary of each commit.
Undoing Changes
Mistakes happen, and knowing how to undo changes is crucial. You can use commands like `git checkout` to revert files back to the last committed state:
git checkout -- filename.txt
To unstage a file, use:
git reset HEAD filename.txt
This command returns the file to the staging area without losing your changes.
Working with Remote Repositories
Adding and managing remote repositories is also handled through the terminal. To add a remote repository, use:
git remote add origin https://github.com/user/repository.git
To see the list of remotes:
git remote -v
And to remove a remote:
git remote remove origin
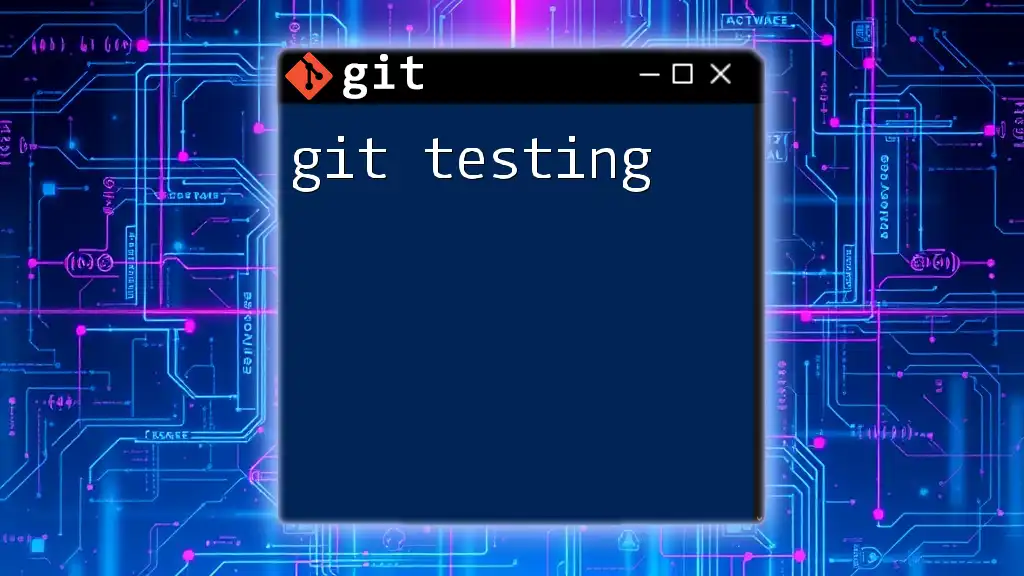
Best Practices for Using Git Terminal
Writing Good Commit Messages
Clear and descriptive commit messages significantly enhance project collaboration. A well-structured message should convey what has been changed and why. For example:
fix: resolve issue with login validation
Regularly Syncing with Remote
To keep your projects aligned and conflict-free, it’s good practice to frequently pull changes from the remote repository. This ensures you’re always working on the latest version.
Keeping Your Repository Clean
Maintain branch cleanliness by frequently merging and deleting branches that are no longer needed. This practice facilitates a smoother workflow and keeps the repository manageable.
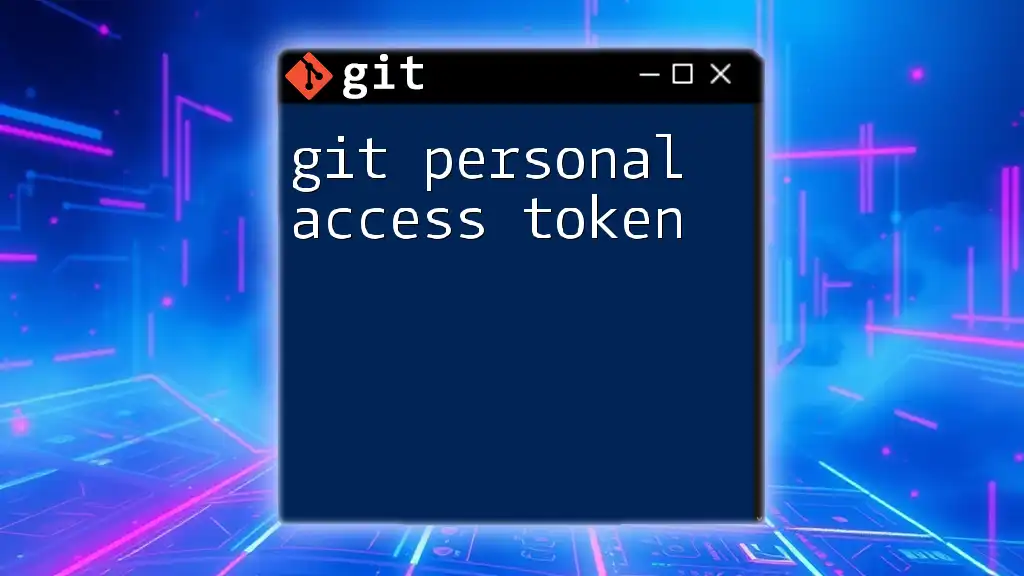
Troubleshooting Common Issues
Resolving Merge Conflicts
When merging branches, conflicts may arise if two or more changes affect the same part of a file. Git marks these conflicts, which you must resolve manually before you can complete the merge. Use a text editor to navigate through the conflicts and decide which changes to keep.
Common Error Messages
Frequent errors can include:
- non-fast-forward updates when trying to push changes that are not fast-forwardable.
- fatal: repository not found indicates issues with the remote URL or access rights.
Understanding these errors helps you employ solutions more efficiently.
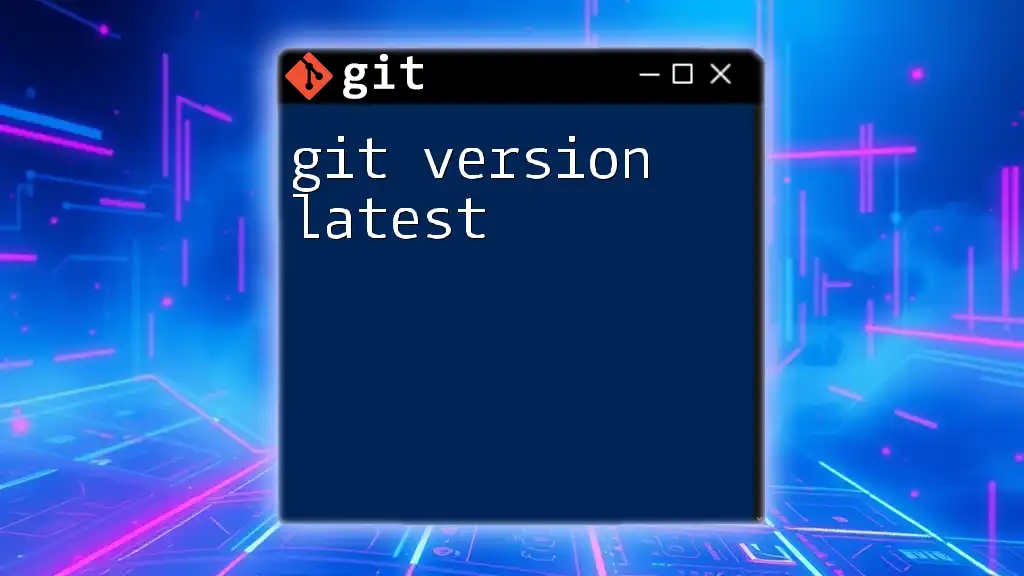
Conclusion
Mastering the Git terminal is an invaluable skill that empowers developers to manage and collaborate on projects effectively. With practice, the fundamental commands will become second nature, allowing you to focus on writing cleaner code and executing complex workflows. The Git terminal opens a world of possibilities for your development endeavors, making it essential to embrace this powerful tool.
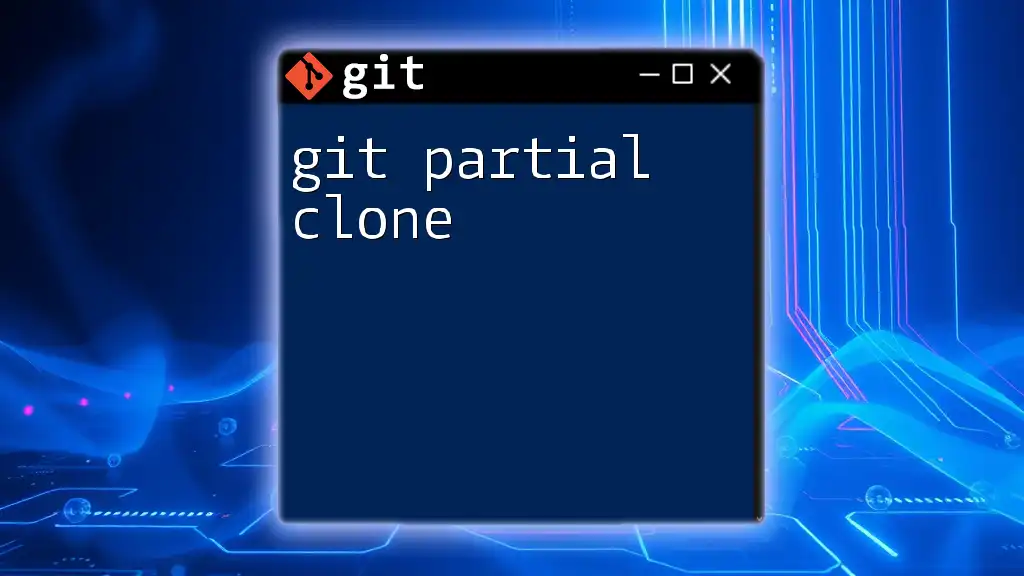
Resources for Further Learning
For additional insights and deep dives into Git, consider visiting the official [Git documentation](https://git-scm.com/doc), which is a comprehensive resource for learning Git commands and configurations. Online platforms like GitHub also offer tutorials and community discussions to further sharpen your Git skills.