Git training equips individuals with the essential skills to effectively use Git commands for version control in a streamlined and efficient manner.
Here's a simple example of a Git command to get started:
git init
What is Git?
Git is a powerful, open-source version control system widely used by developers to track changes in source code during software development. Its key features include:
-
Distributed Version Control: Unlike centralized version control systems, Git allows every developer to have their own complete copy of the project history on their local machine, promoting better flexibility and collaboration.
-
Data Integrity: Git uses a hashing method (SHA-1) to ensure the integrity of the content. This means that after you commit data, Git generates a unique identifier for that commit, ensuring that the content cannot be altered without recognition.
-
Branching and Merging: Git's branching model allows users to create separate lines of development, enabling experimentation without affecting the main codebase. Merging combines these branches back into one, facilitating collaboration and integration.
When compared to other version control systems like Subversion (SVN) and Mercurial, Git's distributed approach and speed give it a significant advantage, especially in large projects with numerous contributors.
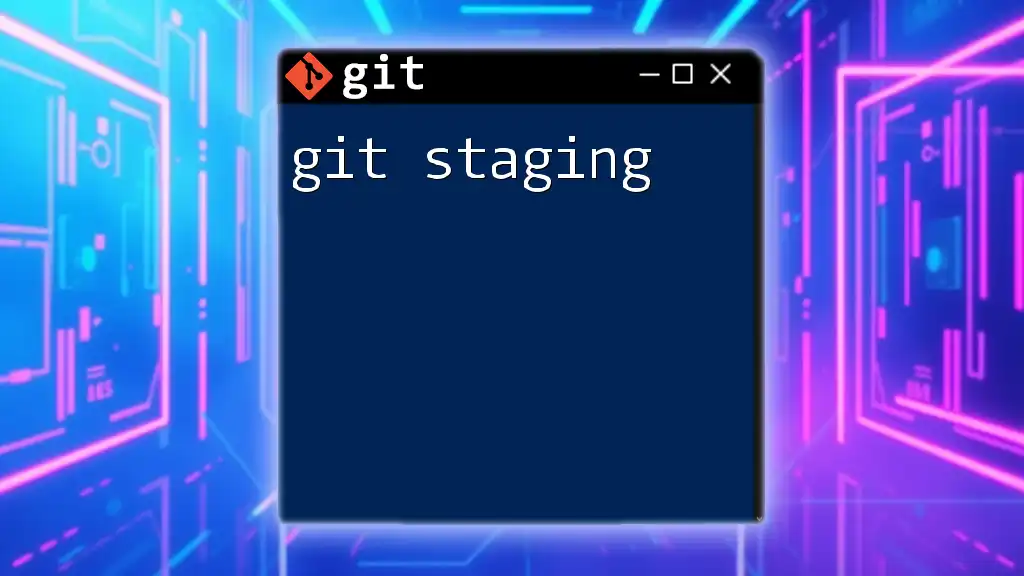
Setting Up Git
Installation
Installing Git varies depending on the operating system:
-
Windows: Download the Git installer from the official Git website and follow the setup wizard, making sure to enable "Use Git from the Windows Command Prompt."
-
macOS: You can install Git using Homebrew with the command:
brew install git
-
Linux: Use your package manager, for example:
sudo apt-get install git
After installation, it's crucial to do some basic configuration to personalize your Git environment.
To set your user name and email, which will be associated with your commits, use the following commands:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
Initializing a Git Repository
Every Git project begins within a repository, often referred to as a repo. You can create a new local repository with:
git init
If you want to work on an existing project, simply clone it from a remote repository with:
git clone <repository-url>
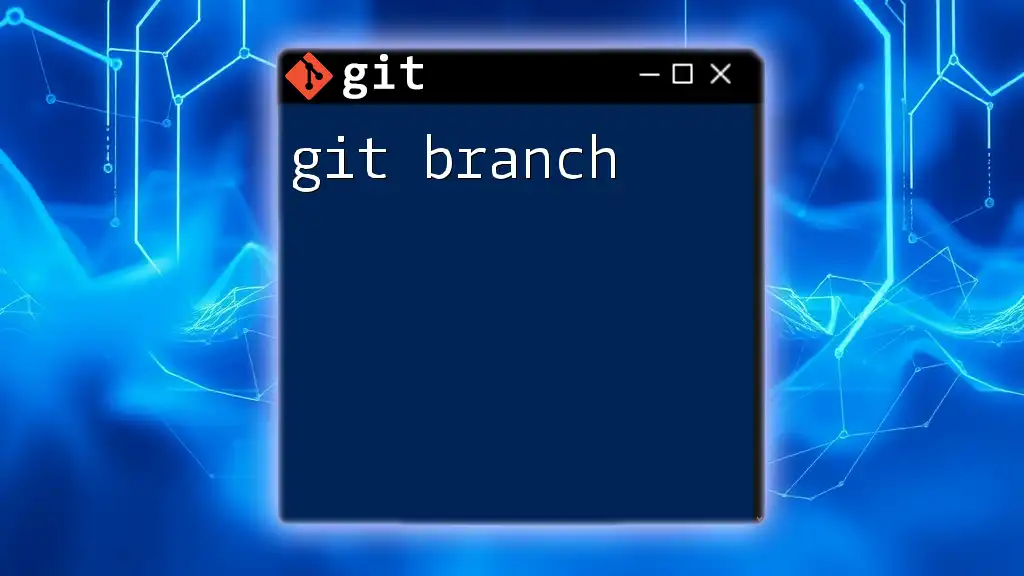
Basic Git Commands
Understanding the Git Workflow
Git's workflow standardizes the process of making changes to a codebase. It involves three key areas:
- Working Directory: Where you make all your changes.
- Staging Area: A space where you can prepare commits. Think of it as a clipboard for changes you want to commit.
- Repository: The complete history of changes you’ve committed to the database.
Common Commands
Checking the Status
To understand the current state of your working directory and staging area, use:
git status
This command will show you which files have been modified, staged, or are untracked, making it a vital step in your workflow.
Adding Changes
To stage changes for inclusion in your next commit, use:
git add <file>
If you're ready to stage all modified files, you can simply run:
git add .
This tells Git to prepare all changes in the working directory for committing.
Committing Changes
Committing changes in Git is crucial, as it captures your work and creates a historical snapshot. To commit your staged changes, use:
git commit -m "Commit message"
Crafting a clear and informative commit message is important! Make sure your commit message answers the question of what the change is and why it was made.
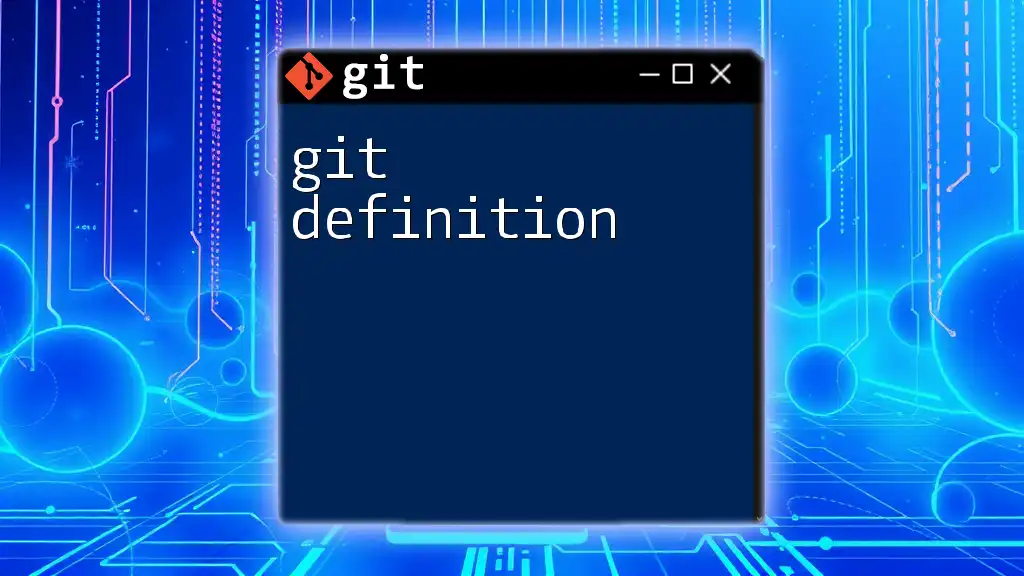
Branching in Git
What is Branching?
Branching allows different developers to work on features or bug fixes independently from the main codebase, often referred to as the master or main branch. This method not only enhances code collaboration but also minimizes conflicts.
Creating and Switching Branches
You can easily create a new branch with:
git branch <branch-name>
To switch to the newly created branch, execute:
git checkout <branch-name>
As of newer Git versions, you can create and switch branches in a single command using:
git checkout -b <branch-name>
Merging Branches
Once your feature development is complete, you’ll want to merge your branch back into the main branch. First, switch to the target branch:
git checkout main
Then, perform the merge:
git merge <branch-name>
If there are any conflicts during the merge, Git will provide markers in the files where the conflict occurs. Carefully resolve these conflicts and finalize the merge.
In case a merge seems problematic, you can abort the merge process and revert back to the previous state by using:
git merge --abort
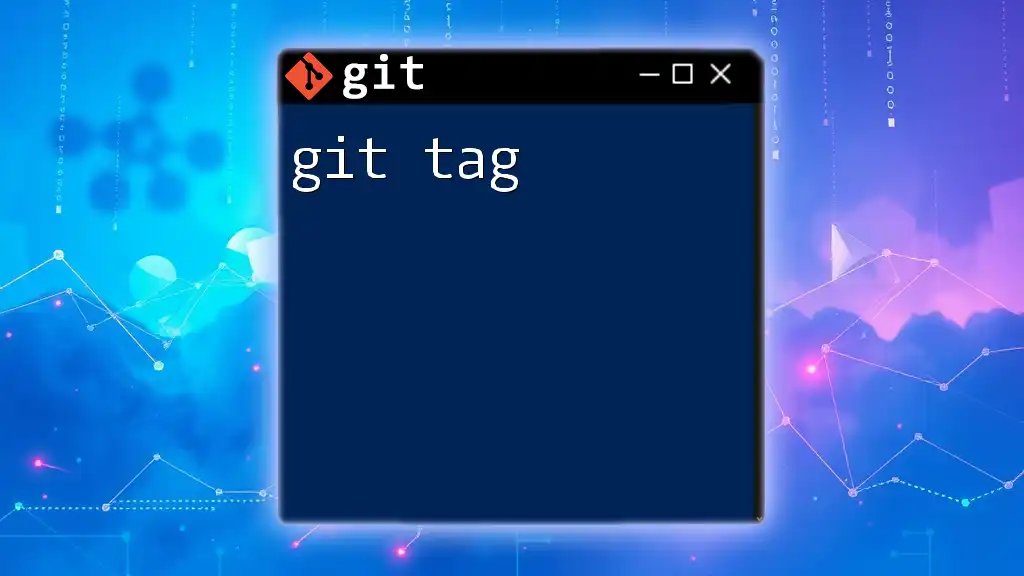
Tracking Changes
Viewing Commit History
To view the history of your commits, run:
git log
This command displays all your commits along with details such as commit hash, author information, and timestamps. For a more concise view, use:
git log --oneline
The `--graph` option visualizes the branching structure.
Checking Differences
To see what changes you have made in your working directory that are not yet staged, use:
git diff
If you want to view the changes that you've staged but not yet committed, the command is:
git diff --cached
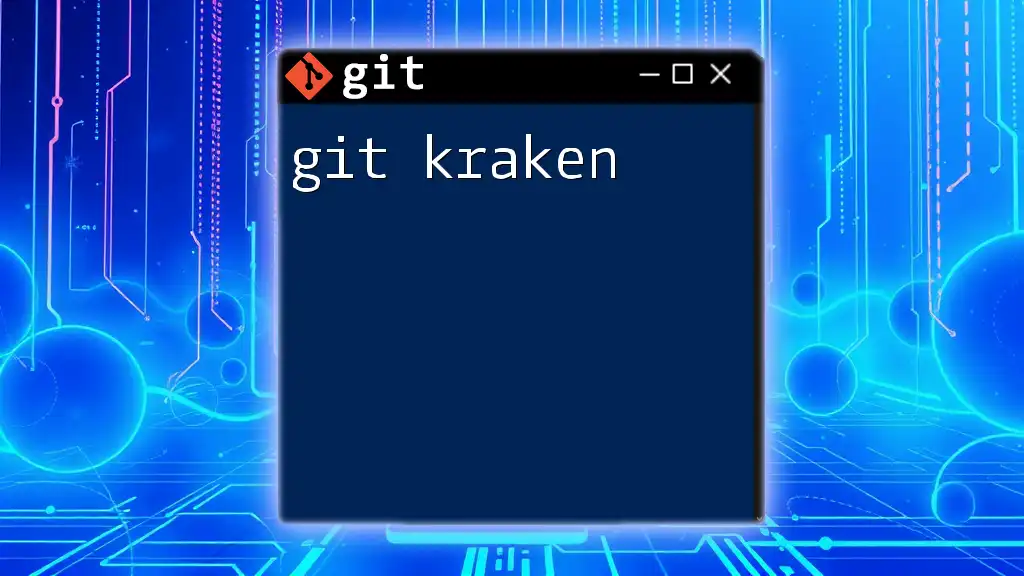
Collaboration with Git
Working with Remote Repositories
A remote allows you to collaborate with others by sharing your repository on servers like GitHub or Bitbucket.
To add a remote repository, use:
git remote add <remote-name> <repository-url>
To fetch updates from a remote repository without merging them, run:
git fetch <remote-name>
When you're ready to incorporate the fetched changes, use:
git pull <remote-name> <branch-name>
Once you have made your changes and committed them, push your local commits to the remote repository with:
git push <remote-name> <branch-name>
Handling Collaboration
Best practices in Git collaboration require frequent syncing with remote repositories to minimize merge conflicts. Always communicate with your team about major changes and use pull requests to facilitate code reviews before merging.
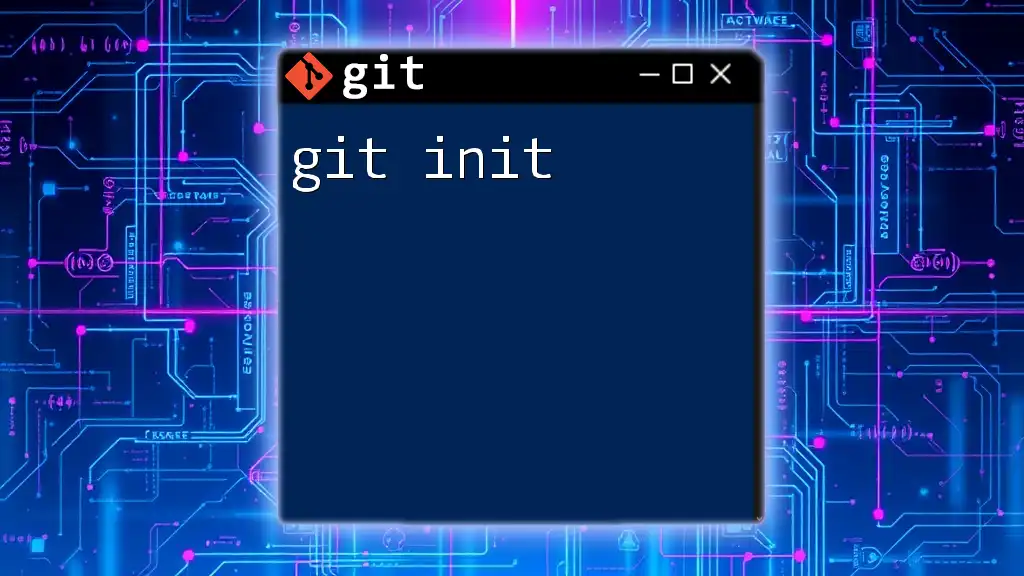
Conclusion
This guide has explored the essential aspects of Git as a version control system and the commands you’ll frequently use for efficient git training. Understanding the Git workflow, mastering basic commands, and knowing how to collaborate effectively are paramount for any developer. As you continue to learn and practice, the mastery of Git will enhance your development capabilities significantly.
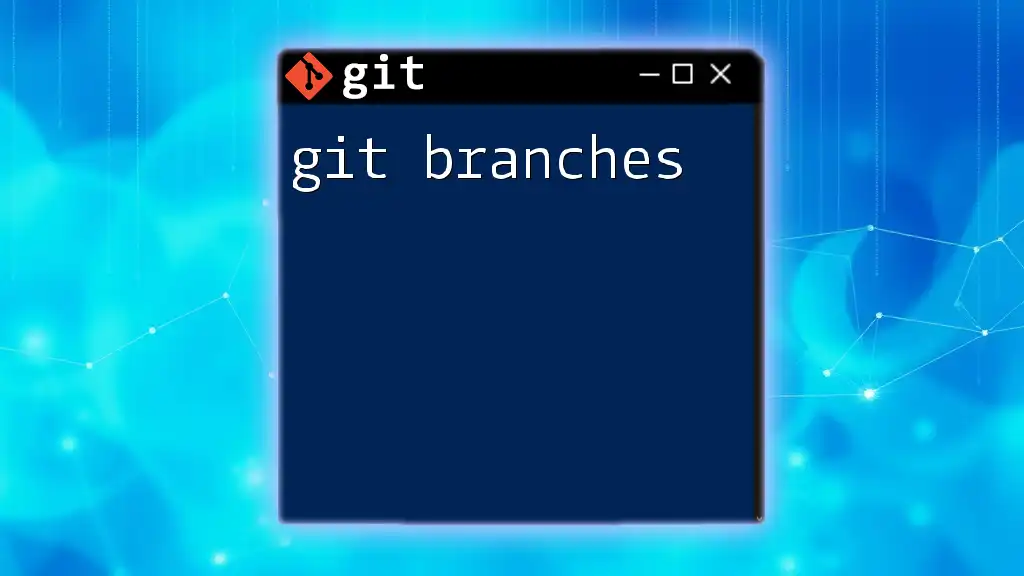
Additional Resources
To further your knowledge, consider exploring the official Git documentation or enrolling in dedicated Git training tutorials online. Engaging in community forums can also provide valuable insights and tips from experienced developers.
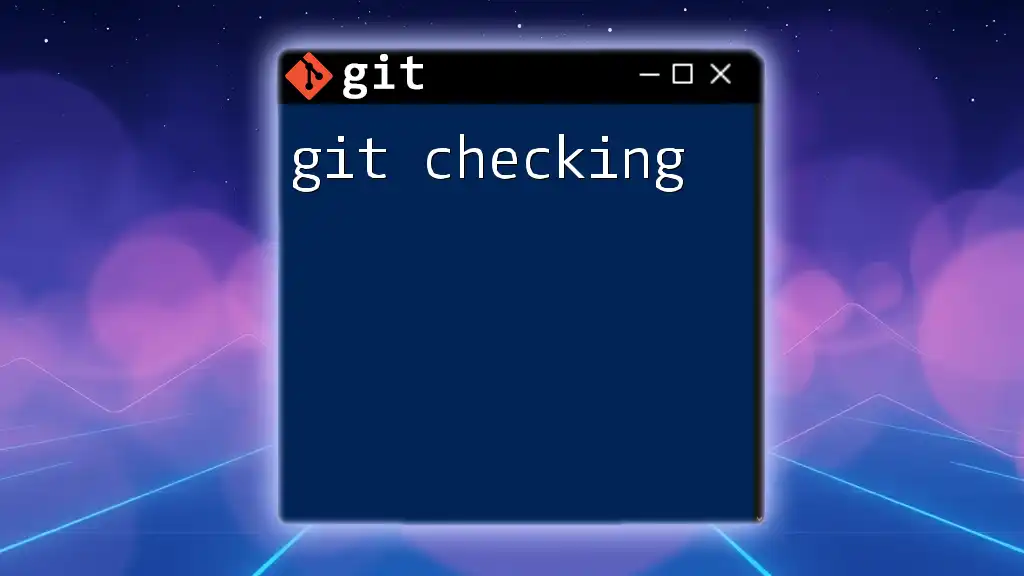
FAQs
As you dive deeper into Git, you may encounter questions about its functionality or the best practices for use. Troubleshooting common errors, such as merge conflicts or authentication issues, can minimize disruptions in your workflow. Keep exploring and enhancing your skills; becoming proficient with Git is an invaluable asset in the development world.