The `git branch` command is used to create, list, or delete branches in a Git repository, helping manage different lines of development.
git branch <branch-name>
What is a Git Branch?
A Git branch is essentially a lightweight movable pointer to a commit. Think of branches as divergent paths in your project, allowing you to work on separate features or experiments without affecting the main code. When you create a branch, you are creating an independent line of development that enables you to develop your features, fix bugs, or experiment freely.
In Git, branches allow multiple developers to work concurrently on the same project without interfering with each other’s work. This means one team member can be focused on a feature while another can patch a bug, all while maintaining a clean and organized project history.
How Branches Work in Git
Branches are pointers to commits within the Git repository. The HEAD pointer is particularly significant because it always points to the current branch's latest commit. Essentially, when you switch branches, the HEAD pointer moves to the tip of the branch you’ve checked out. This allows Git to track which commit you are currently working on and ensures changes are applied to the correct branch.
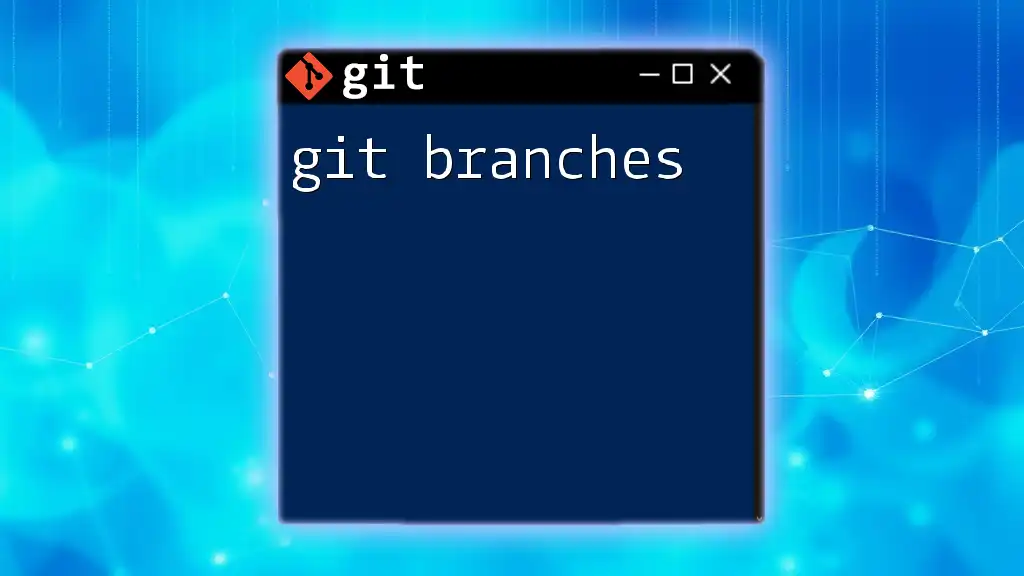
Creating a New Branch
The Basic Command
To create a new branch, you use the command:
git branch <branch-name>
This command simply creates a new branch pointer but does not switch you to that branch. For example, to create a branch called `new-feature`, you would use:
git branch new-feature
Although the branch is created, you remain on your current branch, which is usually the `main` or `master` branch until you switch to `new-feature`.
Switch to a New Branch
To move to your newly created branch, you need to utilize the checkout command:
git checkout <branch-name>
If you want to switch to `new-feature`, you would execute:
git checkout new-feature
This is where your workspace updates to reflect the state of `new-feature`, allowing you to continue development on that branch.
Creating and Switching in One Command
For convenience, you can combine branch creation and switching into a single command with the `-b` flag:
git checkout -b <branch-name>
For instance, you can create and navigate to `new-feature` in one go:
git checkout -b new-feature
This saves time and simplifies the process when starting new features or experiments.
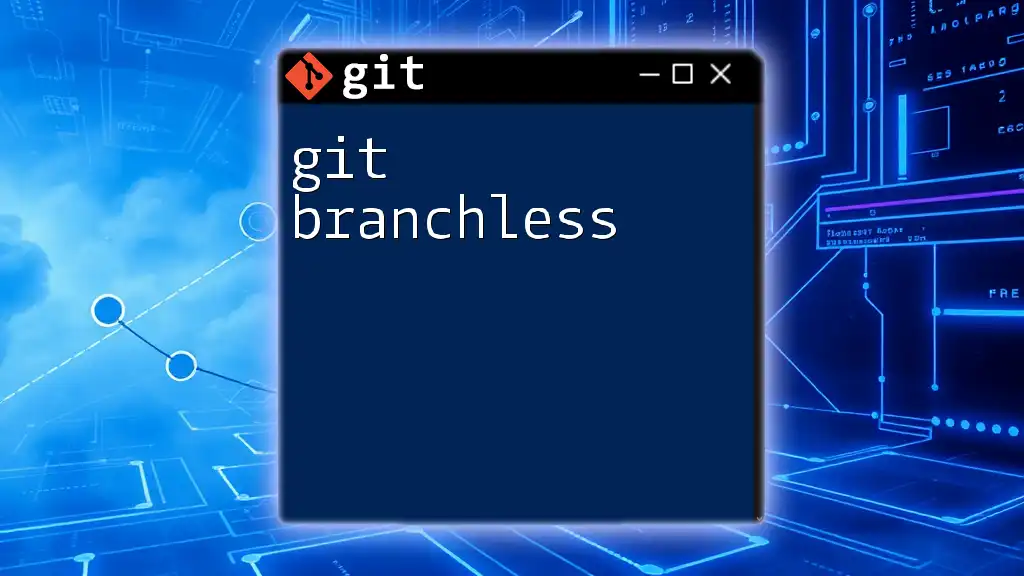
Listing Branches
Viewing All Branches
To see all branches available in your repository, you can use:
git branch
This command lists all local branches, highlighting the branch you are currently on.
Displaying Remote Branches
To view branches stored on a remote repository, you can execute:
git branch -r
This helps in identifying branches that are accessible in the repository hosted on remote servers, facilitating collaboration and awareness of others' work.
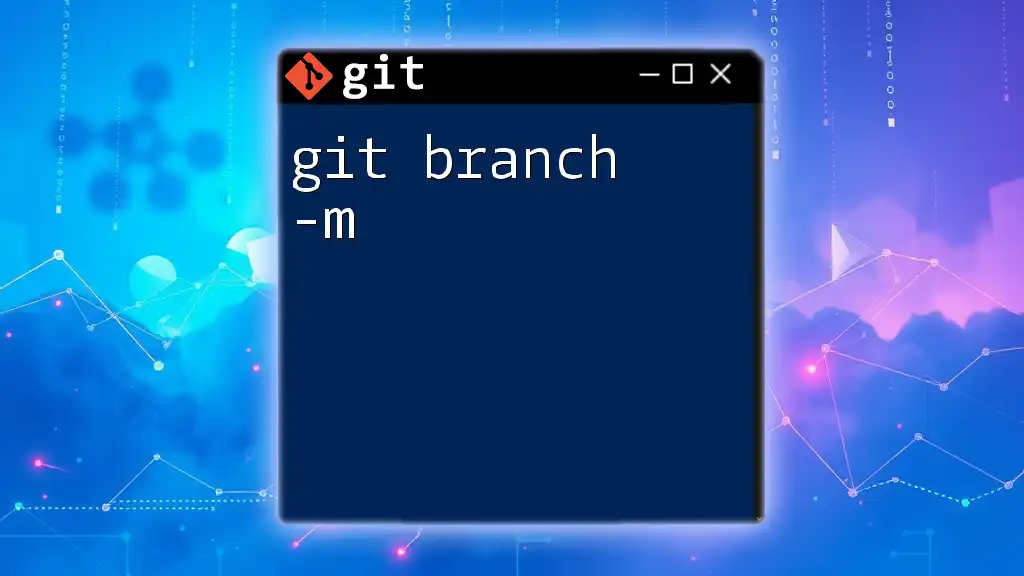
Deleting a Branch
Deleting Local Branches
When you are done with a feature or need to remove a branch that is no longer necessary, you can delete a local branch using:
git branch -d <branch-name>
For example, to delete `new-feature`, you would run:
git branch -d new-feature
This command will only allow deletion if `new-feature` has been fully merged with your main branch, ensuring you don’t lose any important work.
Forcing Deletion
In some cases, a branch might still hold unmerged changes that you wish to discard. To forcefully delete a branch, you can use:
git branch -D <branch-name>
For absolute deletion, even if there are unmerged changes, you would execute:
git branch -D new-feature
While this is effective, be cautious as it permanently removes any unmerged work.
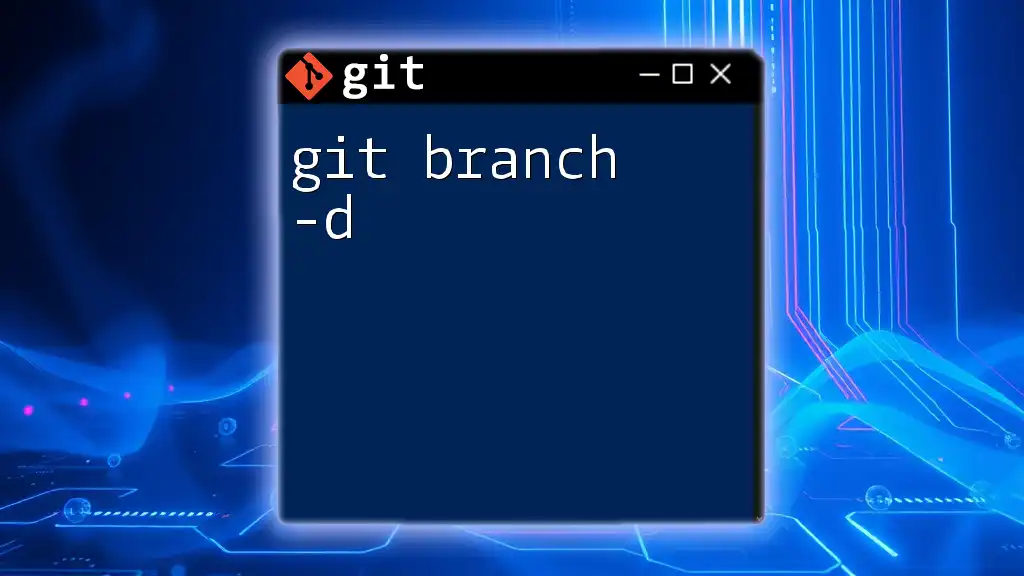
Merging Branches
Introduction to Merging
Merging is a critical part of the branching process, allowing you to integrate your changes from one branch into another. This step is often taken to roll the new feature into the primary trunk of the project after its development.
Basic Merge Command
To merge your current branch into another (typically the main branch), follow these steps:
- First, switch to the branch where you want to integrate the changes (e.g., `main`):
git checkout main
- Then, issue the merge command:
git merge <branch-name>
In practice, if you were merging `new-feature`, you’d use:
git merge new-feature
This command will incorporate all the commits from `new-feature` into `main`.
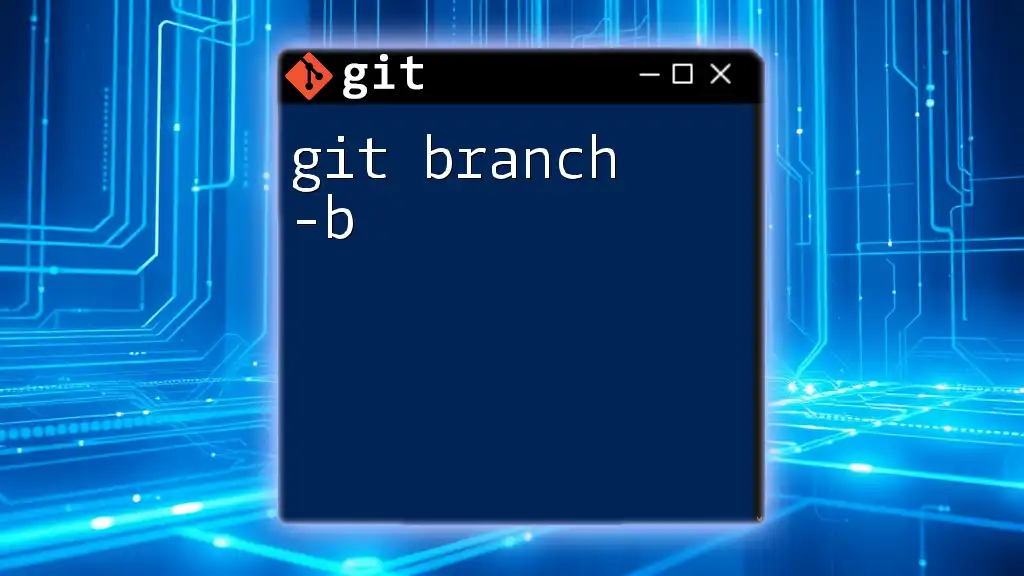
Resolving Merge Conflicts
Understanding Merge Conflicts
Merge conflicts occur when Git encounters conflicting changes in two branches that cannot be automatically reconciled. This situation commonly arises when two branches have modified the same line of a file differently.
Steps to Resolve Merge Conflicts
When a merge conflict arises, Git will notify you which files are involved. You will need to:
- Open the conflicted files.
- Look for conflict markers (`<<<<<<<`, `=======`, and `>>>>>>>`) that Git has inserted.
- Manually edit the file to resolve the conflict, keeping the changes you want.
Practical Example
Suppose you're merging `new-feature` into `main`, and a conflict occurs in `file.txt`. After identifying conflicts in `file.txt`, you would edit it, resolving the differences by selecting the correct lines. Once resolved, mark the conflict as done:
git add file.txt
git commit
This process ensures that you maintain control over what changes are kept.
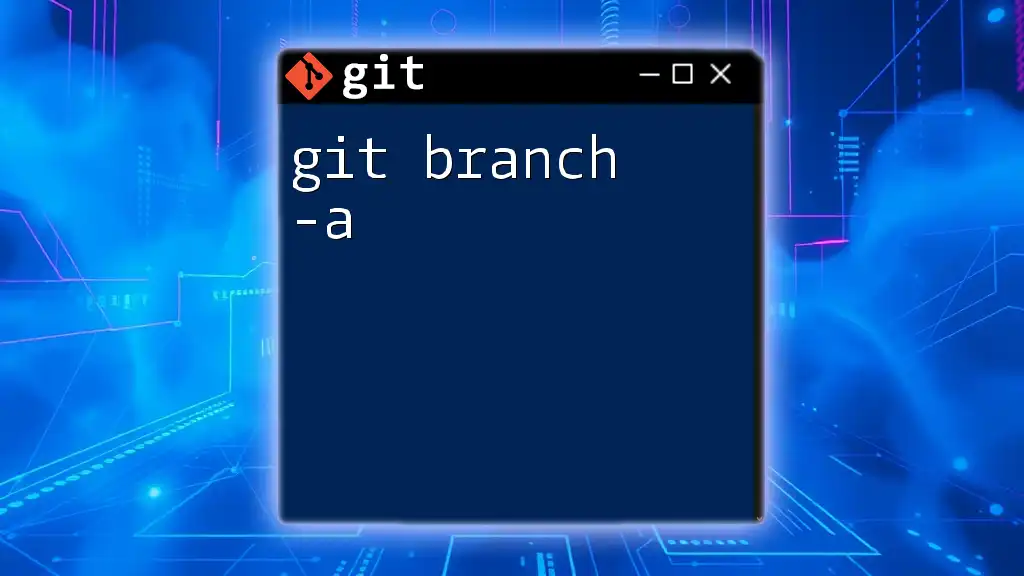
Branching Strategies
Feature Branching
Feature branching is an effective strategy where developers create a separate branch for each new feature or task. This isolation provides a clean way to develop without impacting the main branch, and once the feature is complete, it can be merged back into the main branch.
Git Flow
The Git Flow model introduces a more structured branching system involving multiple types of branches such as:
- Main: Represents production-ready code.
- Develop: Holds all completed features prepared for the next release.
- Feature Branches: For ongoing development.
This method enhances team collaboration and ensures clarity throughout the project lifecycle.
Trunk Based Development
Trunk Based Development emphasizes frequent integration of small changes directly into the main branch. This strategy accelerates feedback loops and promotes continuous delivery while simplifying the branching structure.
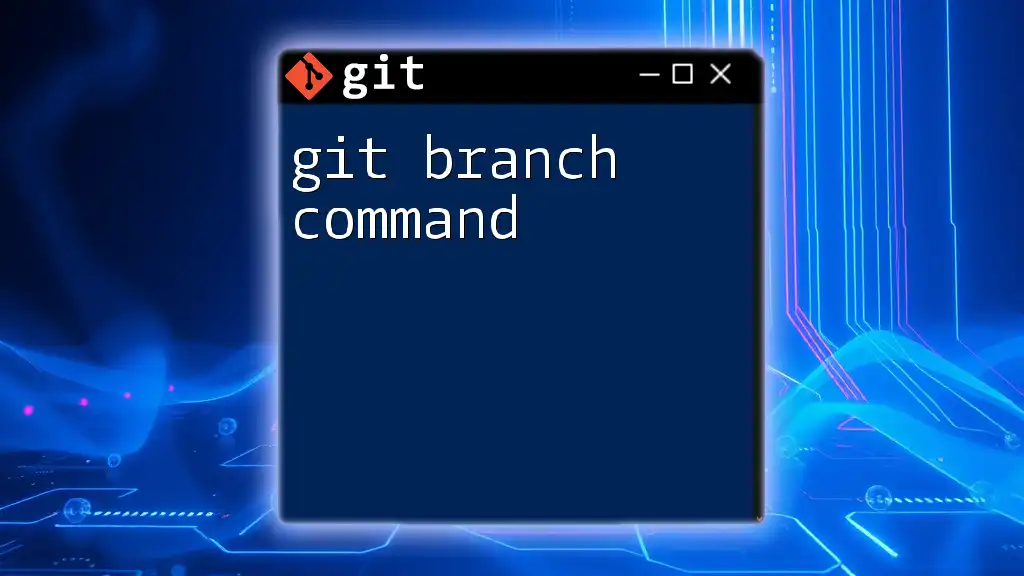
Best Practices for Branching in Git
- Naming Conventions: Use descriptive names that summarize branch purpose, making it easy to identify the branch's function.
- Focused Branches: Keep branches focused on a single task, feature, or bug fix to make collaboration smoother.
- Regular Syncing: Frequently pull changes from the main branch into your feature branch to minimize conflicts during merges.
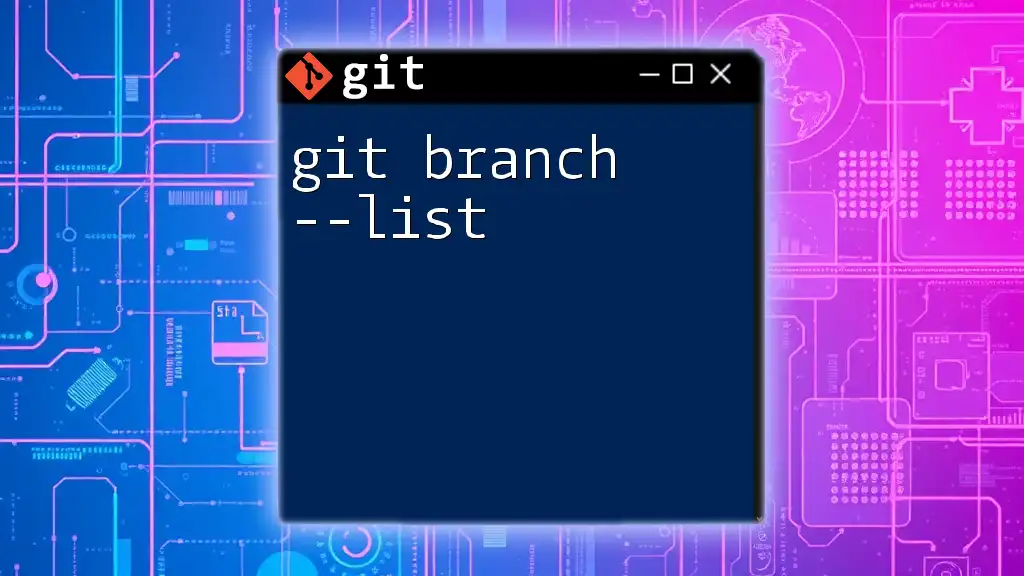
Conclusion
Understanding and effectively using git branch is essential for modern-day development. Branching enables a organized, collaborative environment where multiple developers can work in tandem without stepping on each other's toes. Explore these commands and techniques in your projects to harness the full power of Git.
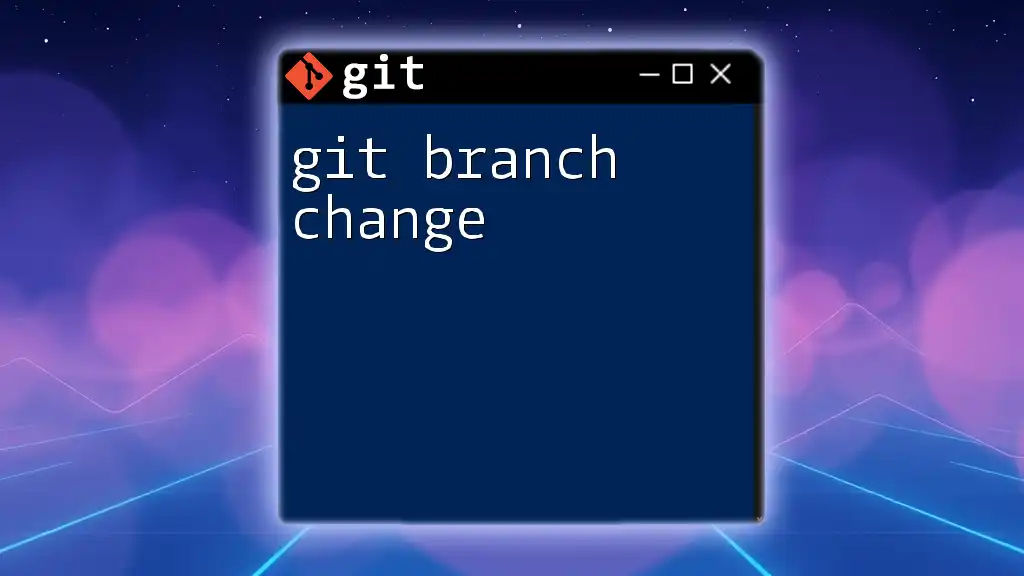
Additional Resources
For further depth on Git branching and its techniques, check out the official Git documentation or explore comprehensive guides and tutorials that can bolster your understanding and efficiency in version control.