The `git branch` command is used to create, list, or delete branches in a Git repository, enabling parallel development and task management.
git branch <branch-name> # Create a new branch
git branch # List all branches
git branch -d <branch-name> # Delete a branch
Understanding Branches in Git
What is a Branch?
A branch in Git represents a unique line of development. It is a fundamental aspect of source control that allows teams to work simultaneously on different features or fixes without affecting the main codebase. By strategically using branches, you can experiment with new ideas and isolate changes, which can later be merged back into the main branch when they're ready.
The Default Branch
In every Git repository, there typically exists a default branch named either `main` or `master`. This branch serves as the central point for all development and is frequently where the production-ready code resides. Understanding how to branch off from this main line of development is crucial as you navigate through more complex workflows.
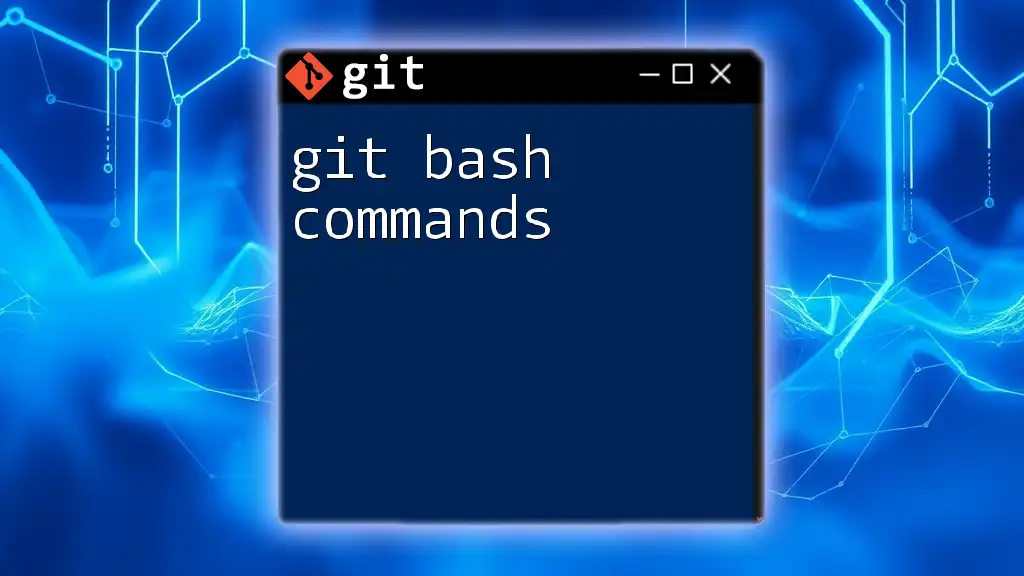
The Git Branch Command
Overview of the `git branch` Command
The `git branch` command is essential for managing branches within your Git repository. It can be used for a variety of tasks, including listing existing branches, creating new ones, and deleting branches as needed. The basic syntax for this command is as follows:
git branch [options] [branch-name]
Listing Branches
To view all existing branches in your repository, execute:
git branch
This command will display a list with the current branch highlighted with an asterisk. Understanding the output helps you identify available branches and ensures you're working within the intended context of your project.
Creating a New Branch
Simple Branch Creation
To create a new branch, use:
git branch branch-name
For example:
git branch feature/new-feature
This creates a new branch named `feature/new-feature`, but keep in mind that you will still be on your current branch until you switch to the new one.
Creating and Checkout in One Command
To streamline your workflow, you can create and switch to a new branch simultaneously with:
git checkout -b branch-name
For instance:
git checkout -b feature/awesome-feature
This command is beneficial because it reduces the number of steps needed to start work on a new feature, saving you valuable time.
Deleting a Branch
Deletion of Local Branch
Once you no longer need a local branch, you can delete it using:
git branch -d branch-name
For example:
git branch -d feature/new-feature
This command will delete the specified branch, but only if it has already been merged into another branch. If the branch is not merged and you wish to force deletion, use:
git branch -D branch-name
Deleting a Remote Branch
When you want to delete a branch from a remote repository, the command is:
git push origin --delete branch-name
For instance:
git push origin --delete feature/new-feature
Understanding when and why to remove remote branches is essential for maintaining a clean project environment, particularly in collaborative settings.
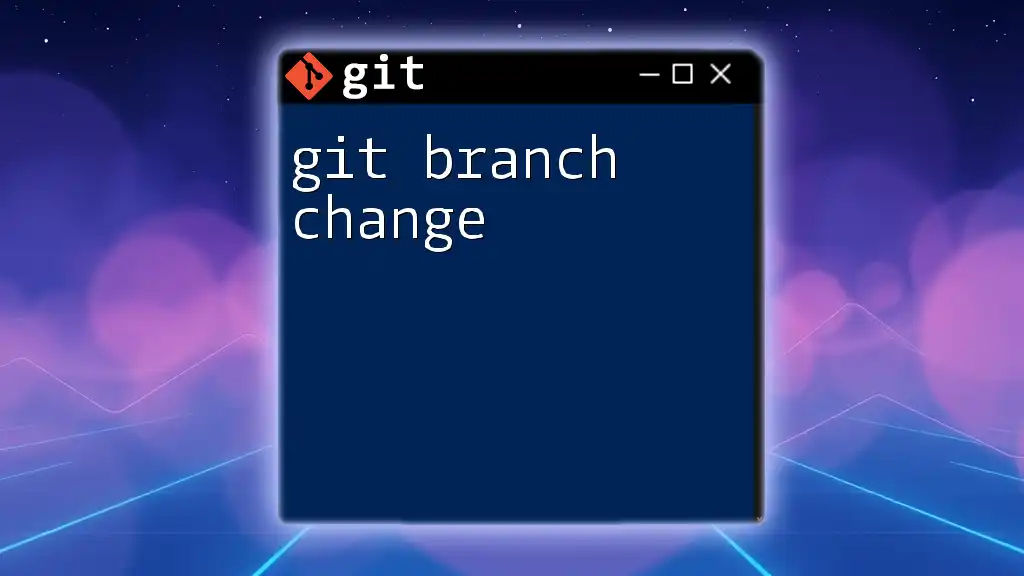
Renaming a Branch
Renaming the Current Branch
If you're looking to rename your current branch, utilize:
git branch -m new-branch-name
For example:
git branch -m feature/renamed-feature
Clear naming is critical for team understanding and collaboration, as it helps convey the purpose of the branch.
Renaming a Different Branch
To rename a branch that you are not currently on, use:
git branch -m old-branch-name new-branch-name
Example:
git branch -m old-feature new-feature
Keep in mind that adequate naming conventions improve project organization and team communication.
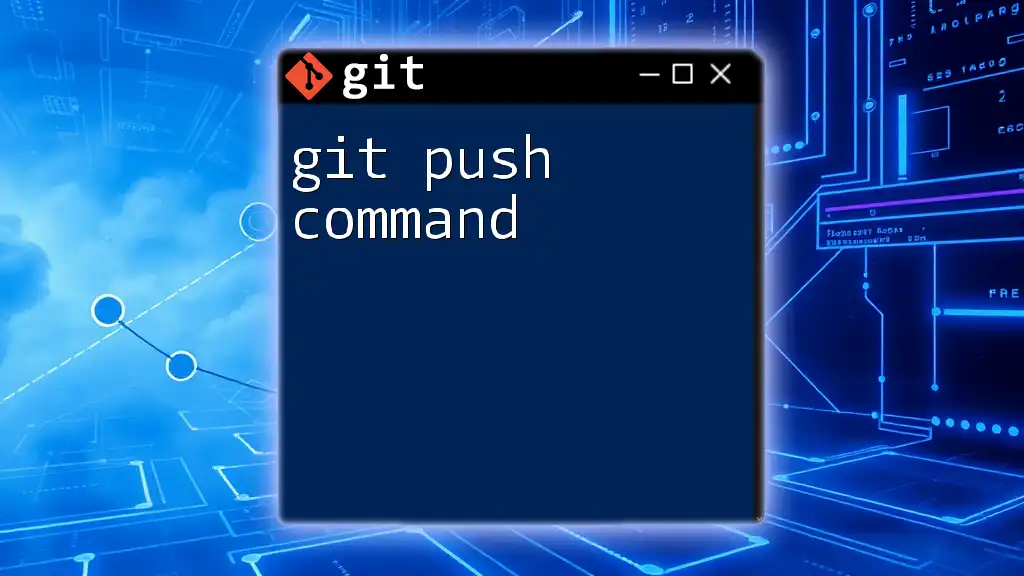
Checking Out Branches
Switching Between Branches
One of the fundamental operations is switching between branches, for which you can use:
git checkout branch-name
Example:
git checkout feature/awesome-feature
The ability to seamlessly switch contexts is vital for any developer balancing multiple features or fixes.
Using `git switch` for Branch Checkout
Git has introduced the `git switch` command, which specifically simplifies branch operations, enhancing usability. To switch to a branch, use:
git switch branch-name
For instance:
git switch feature/awesome-feature
This approach provides a clearer intention and emphasizes usability, making the workflow smoother.
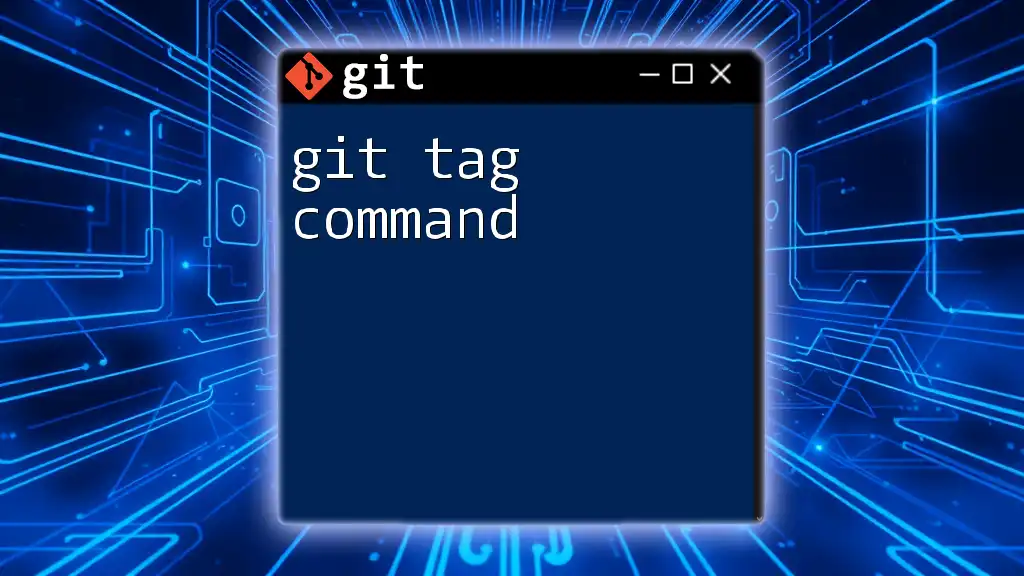
Merging Branches
Merging One Branch into Another
Once a feature is complete, you can merge it back into the main branch using:
git merge branch-name
Example:
git merge feature/awesome-feature
This operation integrates your changes into the target branch. However, be prepared to handle merge conflicts, which can occur if changes overlap.
Fast-Forward and Three-Way Merges
A fast-forward merge occurs when the current branch has not diverged from the target branch. Git simply moves the pointer forward. Conversely, a three-way merge is necessary if both branches have progressed separately. Understanding these different merge types aids in grasping how to manage changes efficiently.
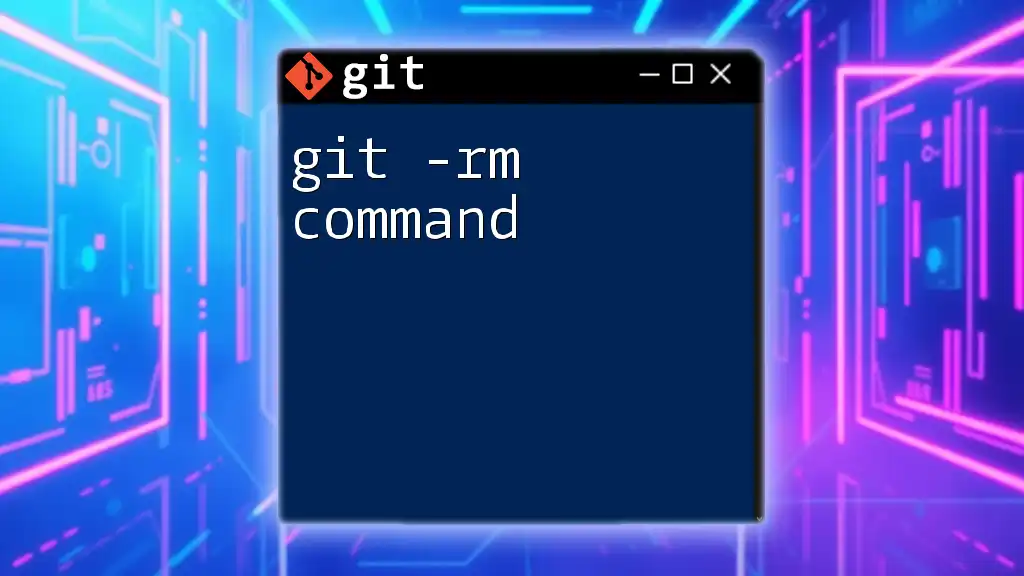
Best Practices for Branching
Naming Conventions
Adopting clear naming conventions for branches is imperative. For instance, prefacing branch names with tags like `feature/`, `bugfix/`, or `hotfix/` can provide context at a glance, ensuring team members understand the purpose of each branch.
Keeping Branches Up to Date
Regularly integrating changes from the main branch into your feature branch helps keep your working codebase current. Use `git fetch` followed by `git merge` or `git rebase` to maintain an updated branch, reducing the complexity of merging later.
Deleting Merged Branches
After successfully merging a branch, it is good practice to delete it. Regular cleanup prevents confusion and keeps the repository organized. A simple command like `git branch -d branch-name` after merging will ensure that only active branches remain.
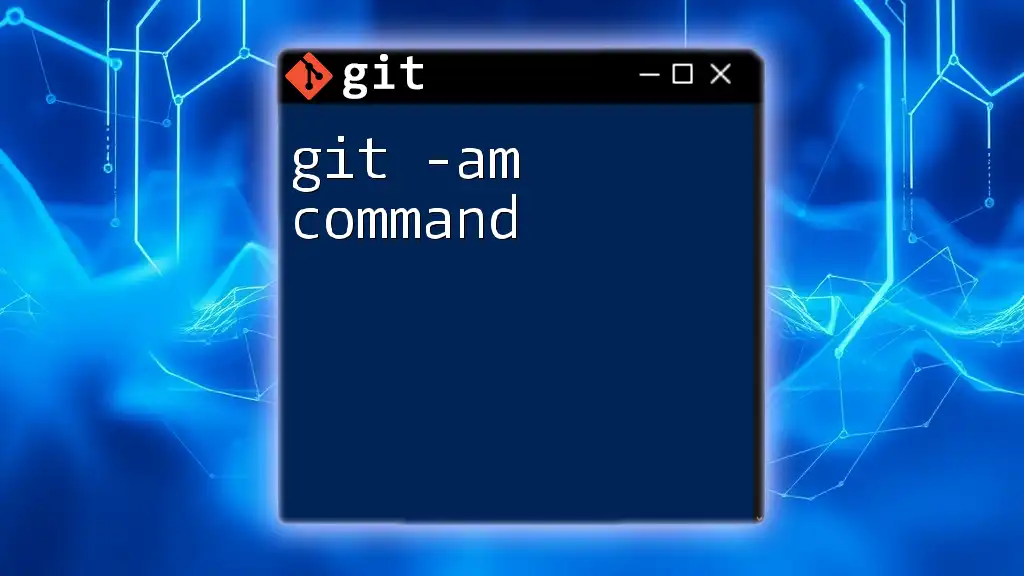
Troubleshooting Common Branch Issues
Resolving Merge Conflicts
Merge conflicts can be daunting, but understanding their resolution is vital. When a conflict arises, Git will inform you about the conflicting files. You need to manually edit these files and then mark them as resolved with:
git add conflicted-file
Follow this by completing the merge with `git commit`.
Branch Not Found Errors
Sometimes, you might encounter a "branch not found" error. This often happens due to typos or trying to access remote branches that haven't been fetched yet. Use `git branch -a` to list all branches, including remote ones, ensuring you’re targeting the correct branch.
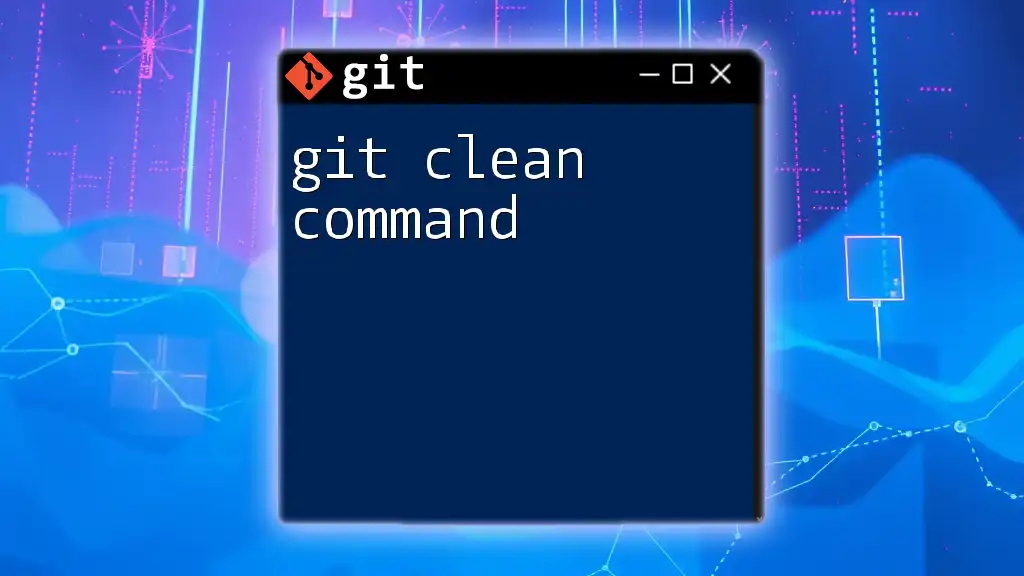
Conclusion
Branching in Git is a powerful feature that facilitates effective project management and collaboration. Understanding and mastering the `git branch command` can significantly improve your workflow, leading to cleaner code and less friction in team environments.
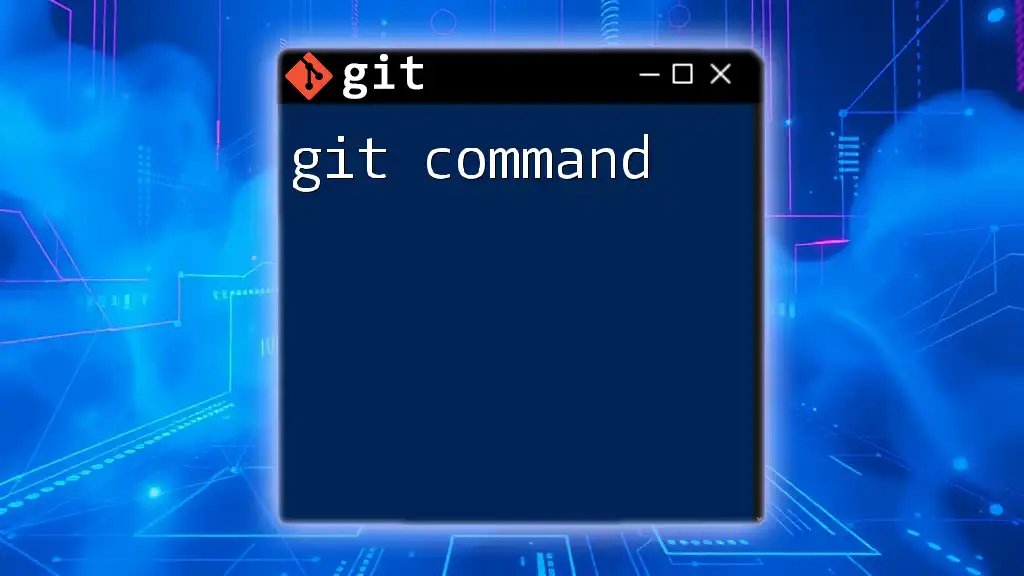
Further Reading and Resources
To delve deeper into Git functionalities, consider exploring Git's official documentation, engaging with recommended books, or diving into online courses that cover Git commands in greater detail. Also, keep handy a Git cheat sheet for quick reference.
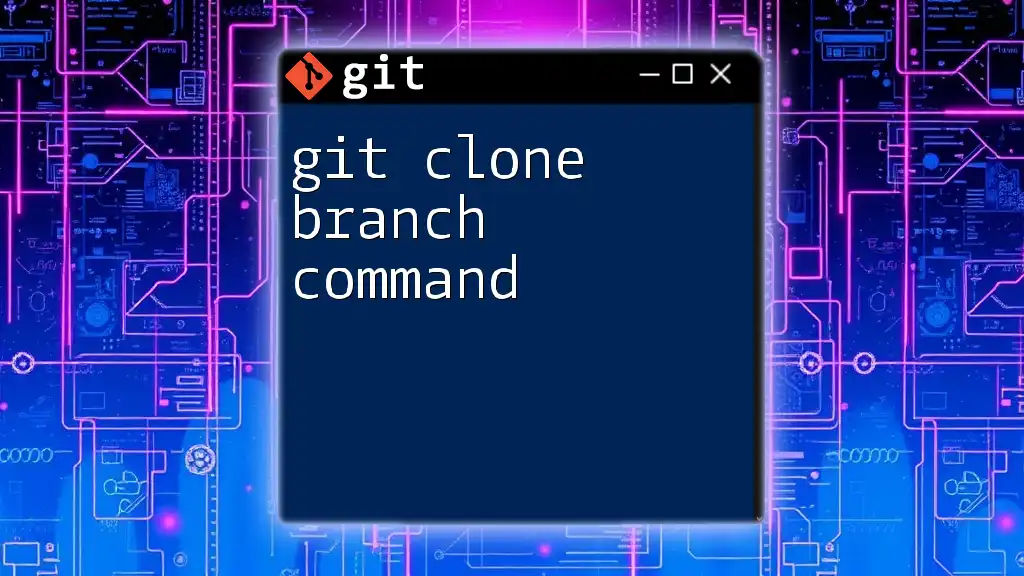
Call to Action
Stay tuned for more guides and insights into Git commands. If you're looking to elevate your skills, subscribe to our updates or download our free resource on Git commands for beginners!