A git command is a specific instruction used to interact with the Git version control system, enabling users to manage and track changes in their code effectively.
Here's an example of a basic git command to initialize a new repository:
git init
Understanding Git
What is Git?
Git is an open-source distributed version control system designed to handle everything from small to very large projects with speed and efficiency. It allows multiple developers to work collaboratively on the same project without interfering with each other’s changes. Originally created by Linus Torvalds in 2005, Git has become the standard for version control used by developers worldwide.
Why Use Git?
The benefits of using Git are manifold. It provides:
- Version History: Every change made is recorded, giving developers access to a complete history of the project.
- Branching and Merging: Git allows for multiple lines of development. Developers can create branches for new features, and merge them back to the main line when completed, ensuring a smooth development flow.
- Collaboration: Tools such as GitHub and GitLab support collaborative work, enabling several developers to contribute simultaneously.
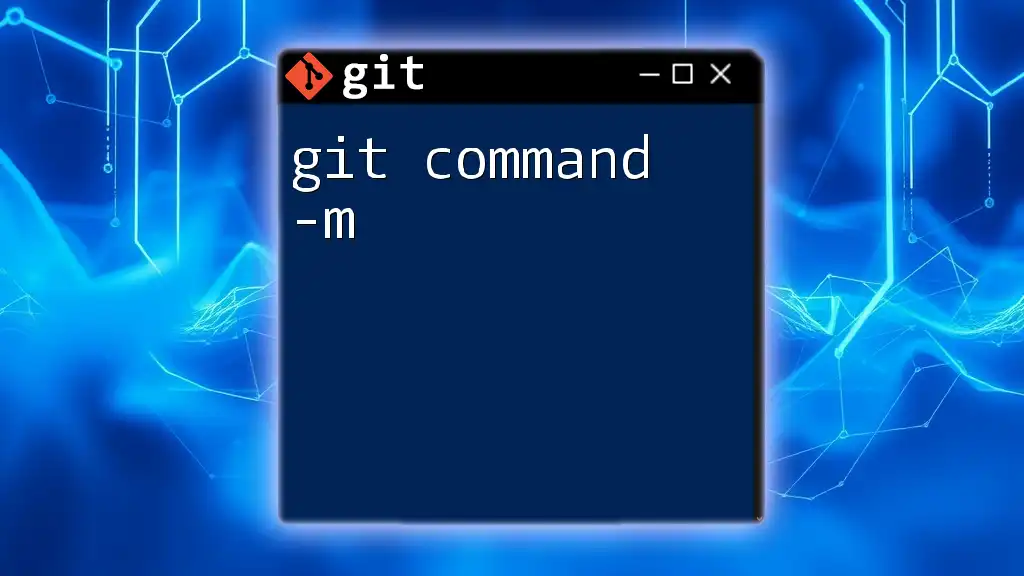
Setting Up Git
Installation
Before you can start using Git commands, you need to install Git on your operating system. Here’s how:
- Windows: Download and install Git from the [official site](https://git-scm.com/download/win). The installer will guide you through the setup process.
- macOS: Use Homebrew and run:
brew install git
- Linux: Depending on your distribution, use either:
sudo apt-get install git # For Debian/Ubuntu sudo yum install git # For CentOS/RHEL
Configuring Git
Initial configuration is essential to set up your Git environment.
Initial Configuration
The first step after installation is to configure your username and email. These will be associated with your commits.
git config --global user.name "Your Name"
git config --global user.email "your-email@example.com"
By using the `--global` option, you set these configurations for all your repositories.
Checking Configuration
To confirm that your settings are correct, you can list your configuration with:
git config --list
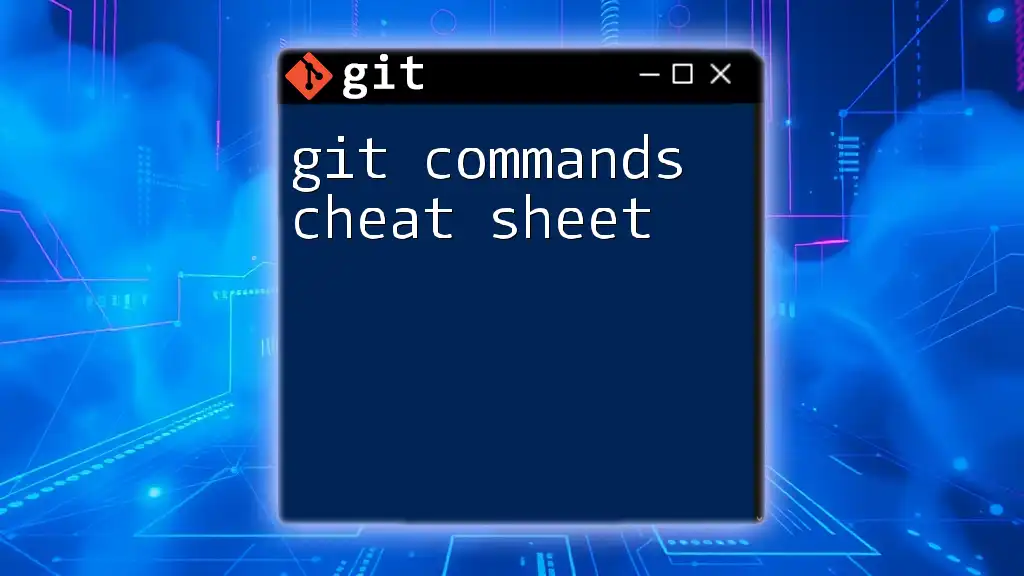
Basic Git Commands
Starting a New Repository
Creating a new Git repository is straightforward. In your terminal, navigate to your project folder and run:
git init my-repo
This initializes a new Git repository in a folder named "my-repo".
Cloning a Repository
If you want to work on an existing project, you'll often clone a remote repository. This can be done with:
git clone https://github.com/username/repo.git
You can also clone using SSH for security:
git clone git@github.com:username/repo.git
Staging Changes
Understanding the Staging Area
The staging area in Git is where you prepare your changes before committing them to the repository. It's like a preview of what will go into your next commit.
Adding Changes
To stage changes, use the `git add` command:
git add file.txt
Alternatively, if you want to stage all your changes, you can execute:
git add .
Committing Changes
Once your changes are staged, they need to be committed.
git commit -m "Your commit message"
When writing commit messages, it’s best to be concise yet descriptive about what changes were made and why.
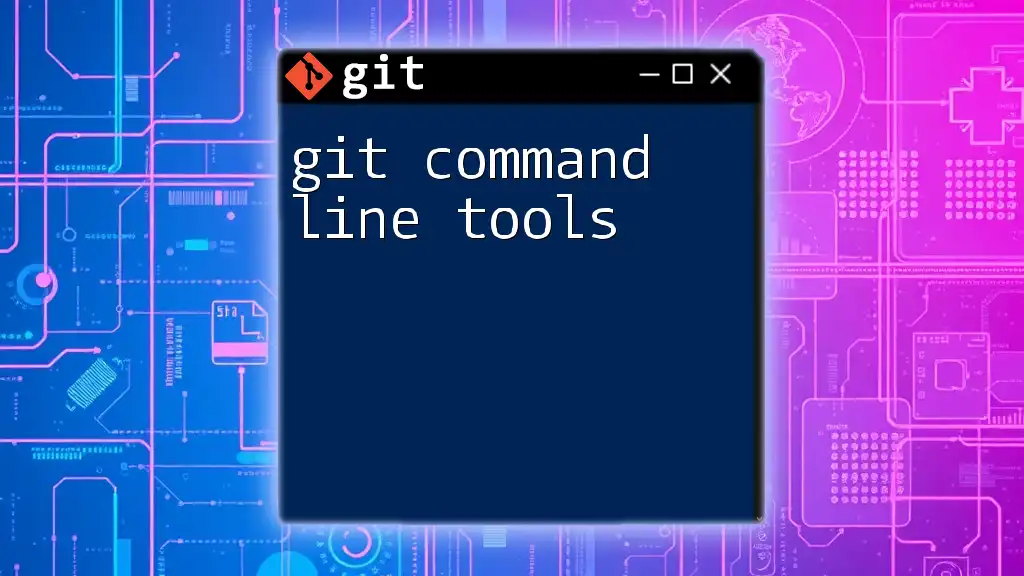
Intermediate Git Commands
Viewing Commit History
Basics of Viewing History
To see a history of your commits, use:
git log
This command reveals the commit ID, author, date, and commit message for each commit.
Customizing Commit Logs
You might want a more compact view of the commit history. You can do so with:
git log --oneline --graph --decorate
This command simplifies the output, showing a graphical representation of branches and a brief description of each commit.
Branching in Git
What is a Branch?
Branches are incredibly powerful. They allow you to create an isolated environment for testing new features without affecting the main codebase.
Creating and Switching Branches
To create a new branch, use:
git branch new-branch
To switch to that branch, execute:
git checkout new-branch
Alternatively, you can create and switch in one command:
git checkout -b new-branch
Merging Branches
Once your work on a branch is complete, it’s time to merge it back into your main branch. Switch back to the main branch and execute:
git checkout main
git merge new-branch
Be mindful of merge conflicts, which occur when changes in different branches clash. If this happens, Git will notify you so you can manually resolve the issues.
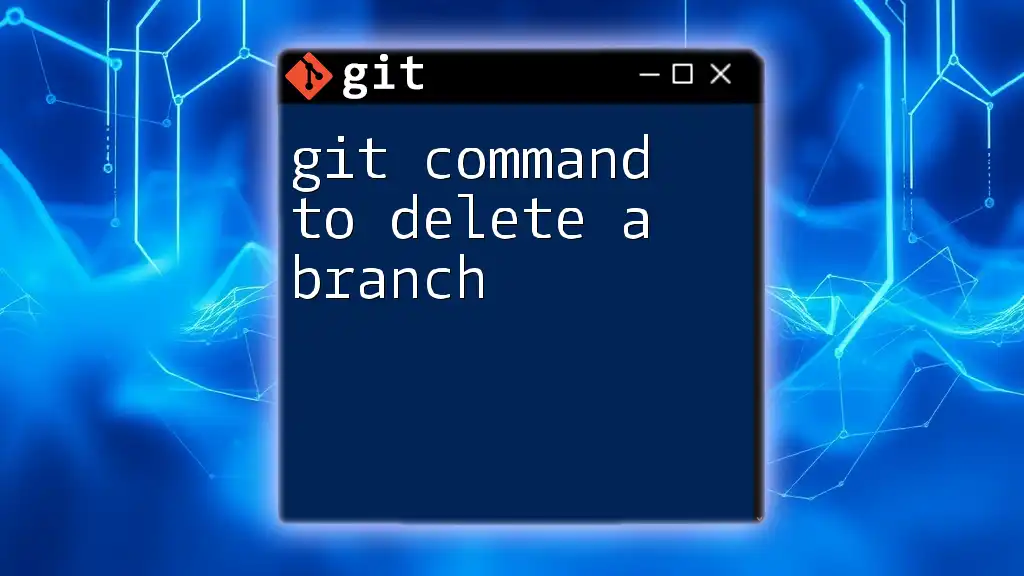
Advanced Git Commands
Rebasing
Rebasing is another way to integrate changes from one branch into another. It re-applies commits on top of another base tip. This results in a cleaner project history.
git rebase main
While rebasing provides a linear history, use it with caution—especially when collaborating with others, as it can rewrite commit history.
Cherry-Picking
Cherry-picking allows you to apply a single commit from one branch to another. This is useful when you want to implement a specific change without merging entire branches.
git cherry-pick <commit-hash>
Cherry-picking can be extremely powerful; however, it should generally be used sparingly to maintain a clean project history.
Undoing Changes
Undoing Commits
If you need to revert changes made in a commit, you can use the revert command:
git revert <commit-hash>
Resetting Changes
If you realize you need to reset your workspace to an earlier commit, use:
git reset --hard HEAD^
Be careful with reset, especially with the `--hard` option, as it will remove all uncommitted changes.
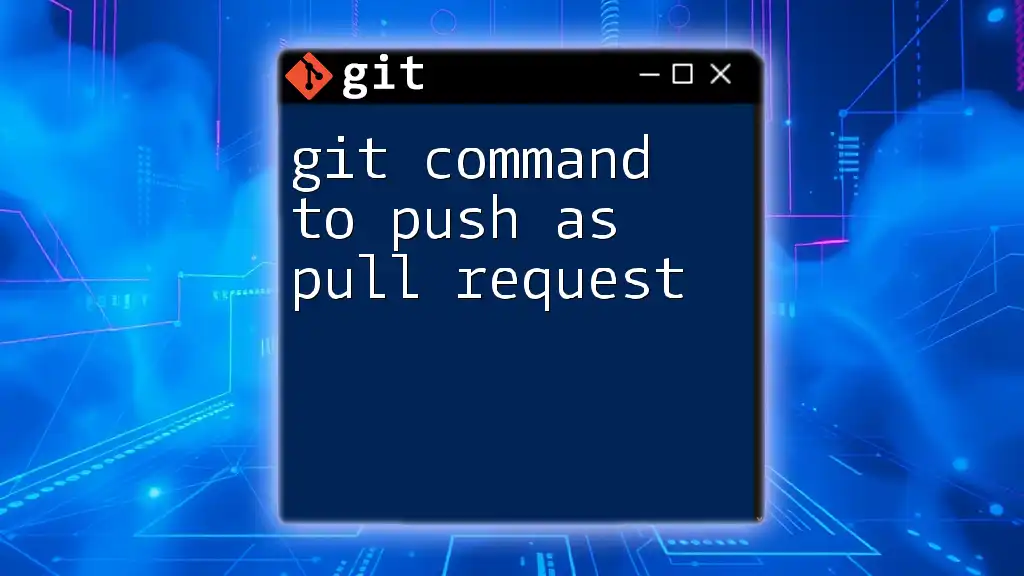
Collaboration with Git
Working with Remotes
Remote repositories are hosted versions of your project, allowing collaboration across different locations.
Pushing and Pulling Changes
To share your changes with others, you push your commits to a remote repository:
git push origin main
To pull changes made by others to your local repository, use:
git pull origin main
Both commands are crucial for collaborative development, ensuring that all team members are on the same page.
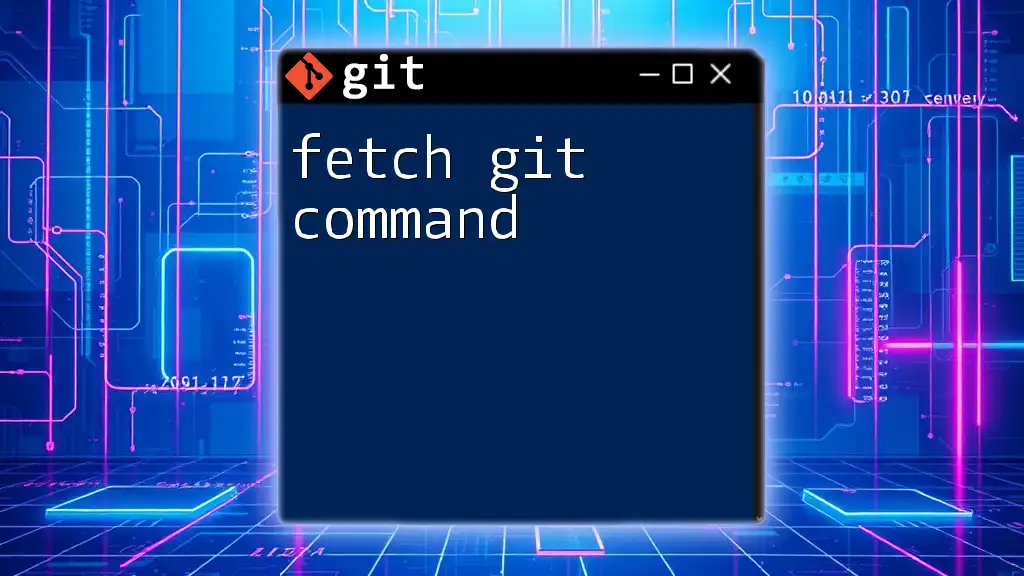
Git Best Practices
Commit Often
Committing frequently helps in isolating changes logically. You can track progress and revert to previous states more efficiently.
Write Clear Commit Messages
Commit messages should be clear and concise. A good message explains what and why the change was made, which aids in project maintenance.
Branching Strategies
Different branching strategies can help maintain order in development. Common strategies include Feature Branching, where each new feature is developed in its branch, and Git Flow, which uses multiple branches for development, releases, and hotfixes.
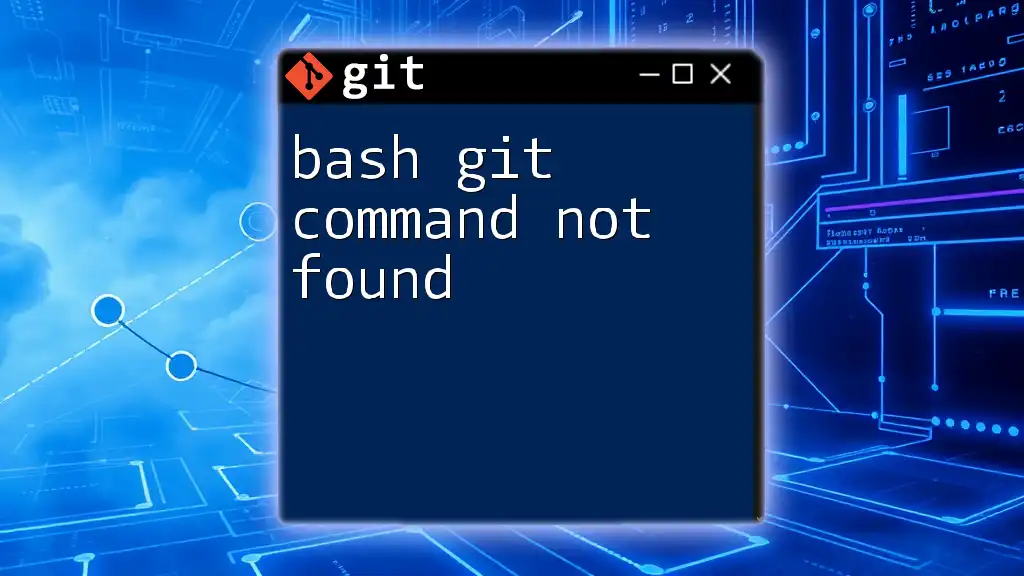
Conclusion
The world of Git commands is vast and powerful, empowering developers to manage projects efficiently and collaboratively. By practicing the commands outlined in this guide, you'll build a solid foundation in version control and become more proficient in your workflows. As you advance, explore additional resources to master more complex functionalities. Happy coding!
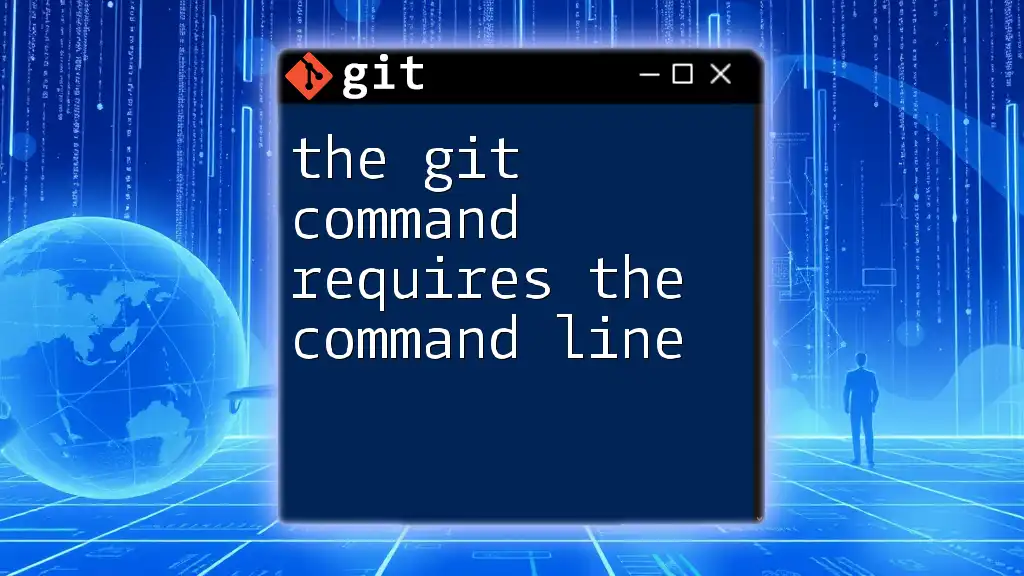
Resources
- Git documentation: [git-scm.com/doc](https://git-scm.com/doc)
- Pro Git book: [Pro Git](https://git-scm.com/book/en/v2)