Git command line tools are essential utilities that allow users to efficiently manage and track changes in their code repositories through concise commands.
git status
Understanding Git Command Line Tools
Git command line tools are essential for developers looking to effectively manage their code. These tools allow you to perform a myriad of tasks related to version control directly from the terminal, giving you greater control and flexibility compared to graphical user interfaces (GUIs).
Getting Started with Git Command Line
Installing Git is the first step to harnessing the power of Git command line tools. Depending on your operating system, the installation process may vary:
- Windows: Download the installer from the [Git website](https://git-scm.com) and follow the setup instructions.
- macOS: You can install Git using Homebrew with the command:
brew install git
- Linux: Most distributions allow installation via package managers like apt:
sudo apt-get install git
Once Git is installed, it’s important to configure Git to set your user information. Use the following commands to specify your name and email, which will be associated with your commits:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
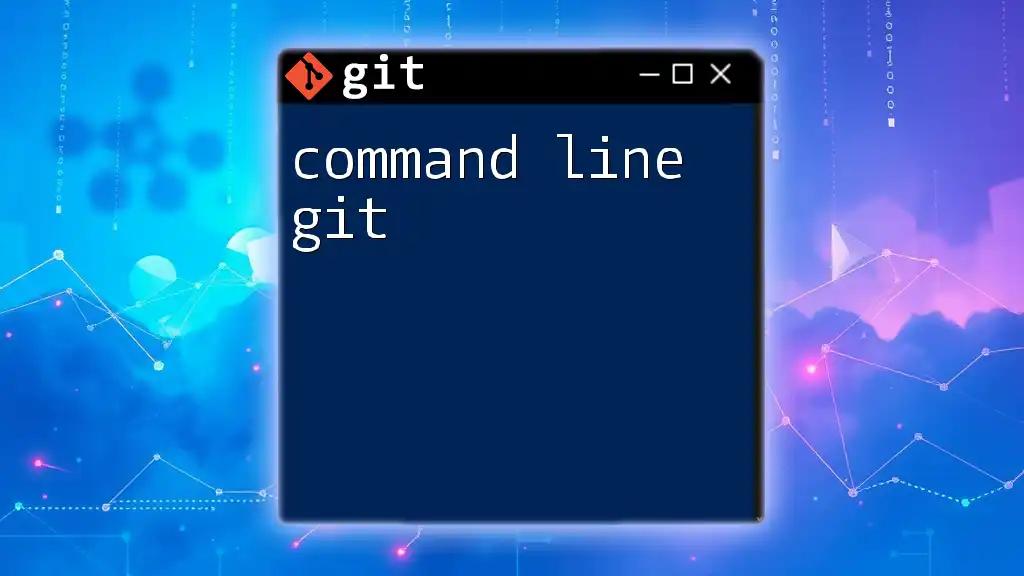
Essential Git Command Line Commands
Creating a New Repository
To kickstart a project with Git, you'll need to create a new repository. This can be achieved using the `git init` command. For example:
git init my-repo
Executing this command will set up a new Git repository in a folder called my-repo, allowing you to start tracking your files.
Cloning an Existing Repository
If you need to work on an existing project, you can use the `git clone` command to download it onto your local machine. Here’s how to do it:
git clone https://github.com/user/repo.git
This command creates a copy of the specified remote repository in your current directory, enabling you to work on it locally.
Working with Changes
Staging Changes
The staging area is a crucial part of the Git workflow, as it allows you to prepare changes before committing them. To stage files, use the `git add` command. You can stage a specific file:
git add filename.txt
Or stage all changes in the current directory with:
git add .
Committing Changes
Once you’ve staged your changes, the next step is to commit them. This creates a snapshot of the project at that moment. Use descriptive commit messages to document what changes were made, which is beneficial for collaboration and project tracking:
git commit -m "Add feature x"
A clear commit message communicates changes effectively to your team members and helps maintain project clarity.
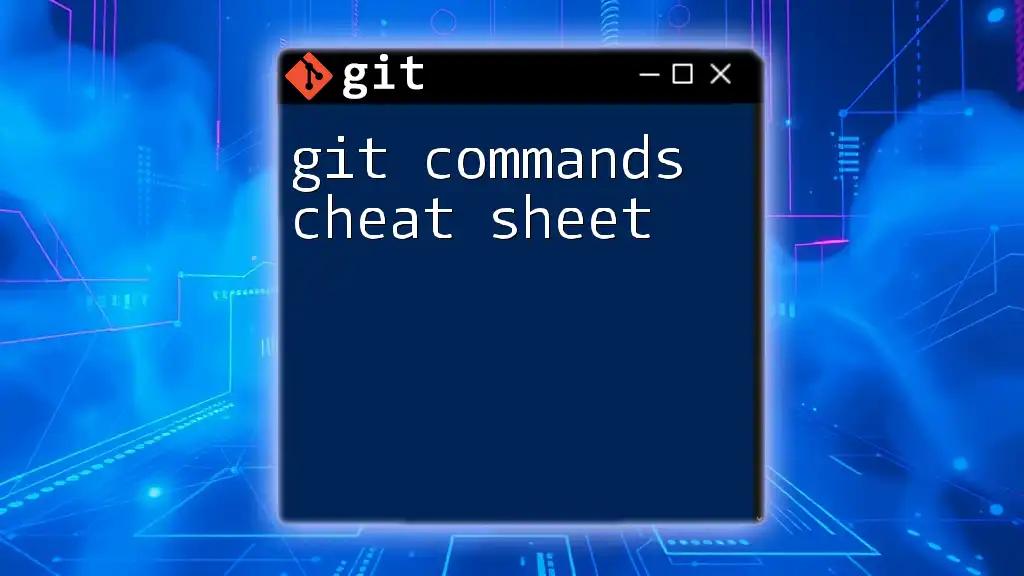
Branching and Merging
Understanding Branches
Branches are integral to Git, allowing you to diverge from the main codebase and work on features or fixes without affecting the existing code. This isolation promotes clean and efficient development workflows.
Creating and Switching Branches
Creating a new branch is simple with the `git branch` command. After that, you can switch between branches using either `git checkout` or `git switch`:
git branch feature-branch
git checkout feature-branch
Alternatively, you can create and switch in one command:
git switch -b feature-branch
Merging Branches
Once you complete the work on a branch and are satisfied with your changes, you can integrate those changes back into the main branch. This is done using the `git merge` command:
git merge feature-branch
Effective merging is vital to ensure that collaborative efforts are streamlined and the main branch is always stable.
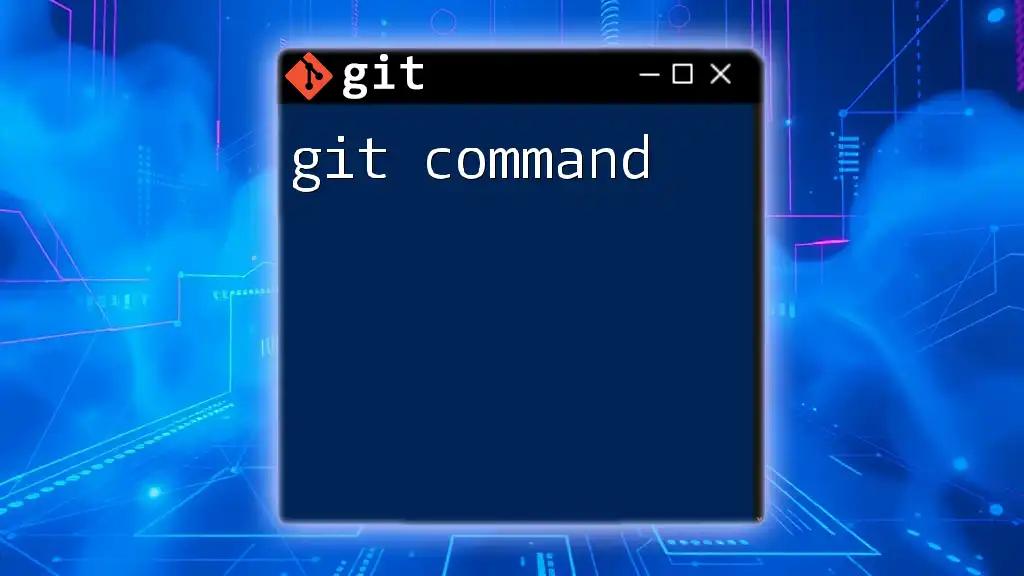
Advanced Git Command Line Tools
Viewing Project History
To keep track of the history of your project, the `git log` command is indispensable. It displays a list of all commits made in the repository. You can customize how the log is displayed:
git log --oneline --graph --all
This command will show a concise view of the commit history, making it easier to review changes across branches.
Undoing Changes
Mistakes happen; knowing how to undo changes is essential. Use `git reset` to remove commits from your branch history:
git reset HEAD~1 # Undo last commit
Alternatively, if you want to revert a specific commit while keeping the history intact, you can use `git revert`:
git revert <commit_id>
This way, you can undo changes without losing the context of your project's development.
Collaborating with Others
Fetching and Pulling Changes
When working in teams, it's crucial to stay updated with the latest changes from the remote repository. The `git fetch` and `git pull` commands are key to this process.
- Fetching changes retrieves the latest commits from the remote without altering your local branches:
git fetch origin
- Pulling changes integrates fetched changes into your current branch:
git pull origin main
Using these commands ensures that you are always aware of other collaborators' updates.
Pushing Changes
After committing your changes locally, you will want to push them to share with others. This is done with the `git push` command:
git push origin feature-branch
This command sends your changes and updates the remote repository, allowing your team to see the new features or fixes you've implemented.
Managing Remote Repositories
Adding a Remote Repository
When starting a project, you might want to link a local repository to a remote repository. Use the `git remote` command to achieve this:
git remote add origin https://github.com/user/repo.git
This command sets up a connection between your local repository and the specified remote path.
Removing a Remote Repository
If you ever need to remove a remote reference, perhaps because you're switching to a different repo, use:
git remote remove origin
This command will effectively delete the reference to the remote repository, but won't affect your local files.
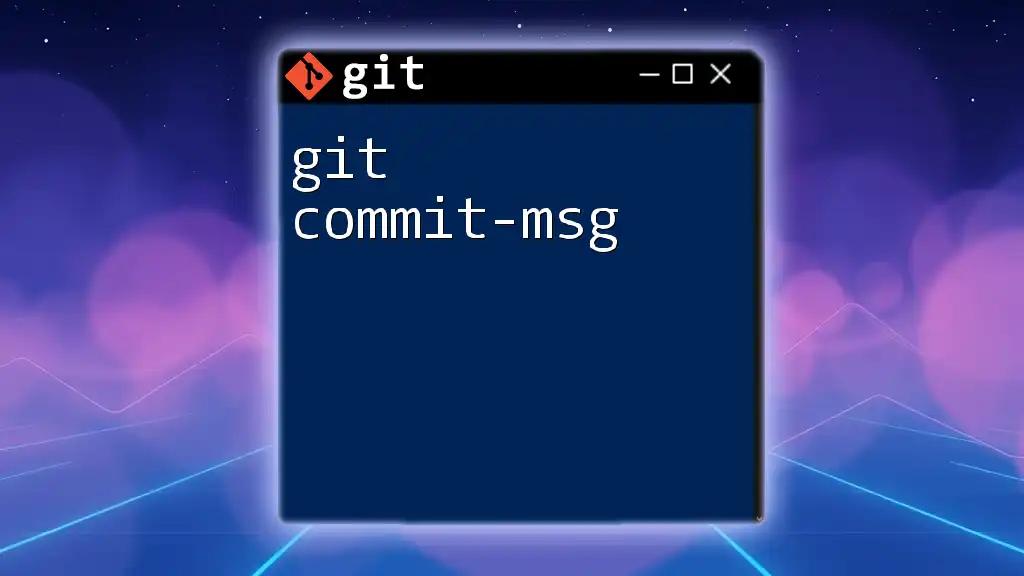
Best Practices for Using Git Command Line Tools
To get the most out of Git command line tools, consider these best practices:
-
Writing Clear Commit Messages: Always use meaningful commit messages that clearly describe the purpose of the changes. This practice aids collaborators and future you in understanding the project's evolution.
-
Using Branch Protection: Protect your main branches from direct modifications. Set up branch protection rules in your remote repository settings to ensure that changes undergo testing and review.
-
Regularly Pulling Changes: Consistently pull updates from the remote to keep your branches up-to-date. This minimizes merge conflicts and keeps your development synchronized with that of your team.
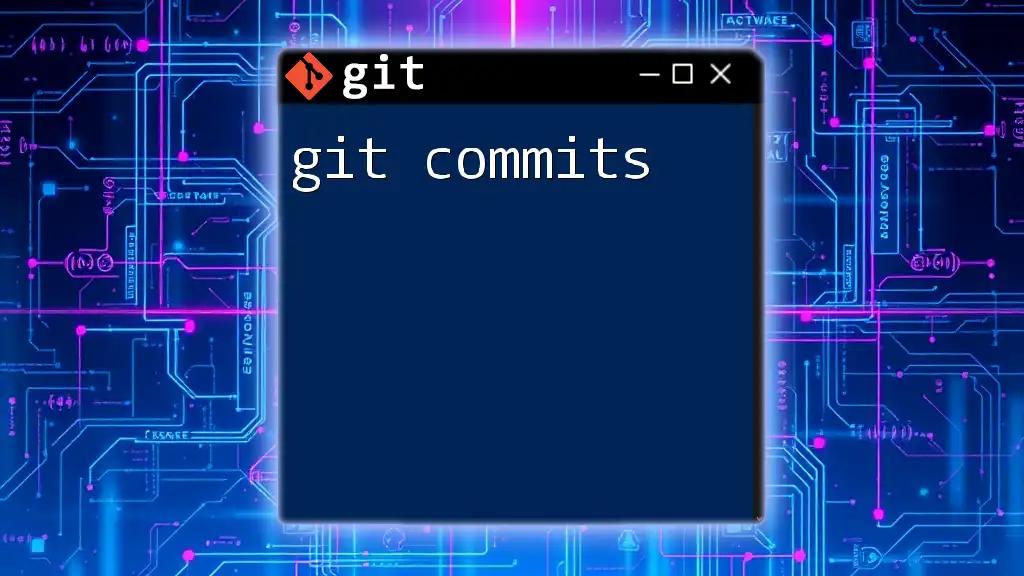
Conclusion
Understanding and effectively utilizing Git command line tools is essential for any developer in today's collaborative environments. With the knowledge of commands for creating repositories, staging changes, managing branches, and collaborating with others, you are well-equipped to handle a wide range of version control tasks.
With continued practice and exploration of more advanced functionalities, you will not only enhance your workflow but also contribute more effectively to your team's projects. Keep learning, stay curious, and harness the full potential of Git command line tools!