To delete a Git branch, use the command `git branch -d branch_name` for a safe delete or `git branch -D branch_name` for a force delete.
git branch -d branch_name
or
git branch -D branch_name
Understanding Git Branches
What is a Git Branch?
In Git, a branch is a fundamental concept that represents an independent line of development. It allows you to work on different features, fix bugs, or experiment, all while keeping your main codebase stable. Essentially, branches are a way to isolate your work without affecting the main project. The default branch in a Git repository is typically called `main` or `master`.
Why Delete a Branch?
Deleting branches is essential for maintaining a clean and manageable repository. Here are a few scenarios in which you might want to delete a branch:
- Merged Branches: Once a feature branch has been merged into the main branch, it can often be safely deleted.
- Outdated or Abandoned Branches: Sometimes you may create branches for features or fixes that are no longer relevant. Deleting these can help avoid confusion.
- Reducing Clutter: Keeping a tidy repository is crucial for collaboration and avoiding mistakes, especially when working in teams.

Types of Git Branches
Local vs. Remote Branches
Branches can be categorized as local or remote:
- Local Branches are those created and modified on your own machine. They are only visible to you until shared.
- Remote Branches are the versions of your branches stored on a remote server. They are commonly used for collaboration in a team setting.
Naming Conventions
Establishing a consistent naming convention for your branches can significantly enhance clarity in your project. Common patterns include:
- `feature/feature-name`
- `bugfix/issue-title`
- `hotfix/urgent-fix`
Consistent naming not only aids in communication but also helps team members know the purpose of each branch at a glance.
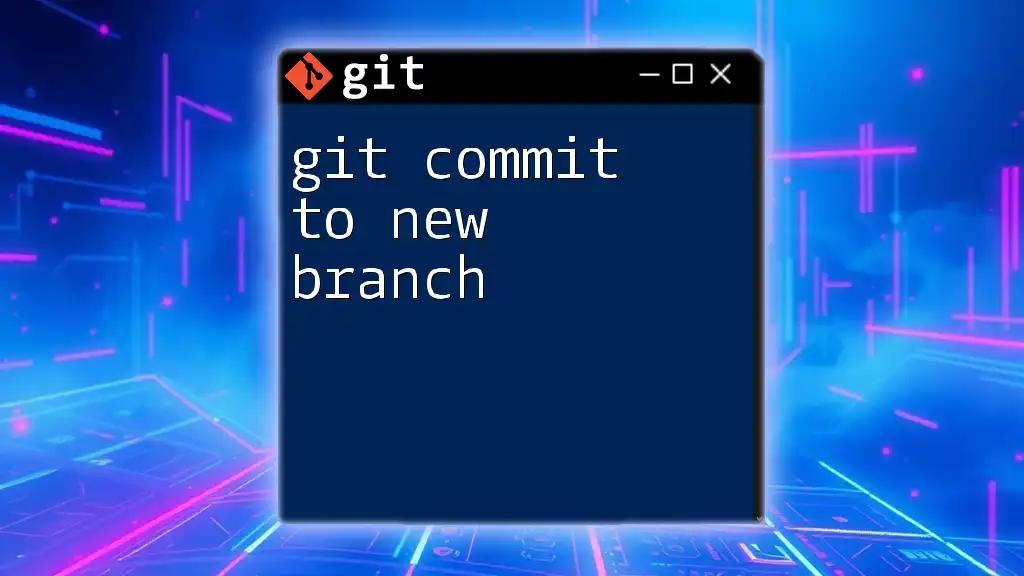
How to Delete a Local Branch
Basic Command to Delete a Local Branch
Removing a branch that is no longer needed is straightforward. To delete a local branch, you can use the command:
git branch -d branch_name
In this command, `-d` stands for delete, and `branch_name` is the name of the branch you want to remove. Note that this command only works if the branch has been fully merged into the current branch.
Force Deleting a Local Branch
If you attempt to delete a branch that hasn't been merged, Git will prevent this action by default. However, in situations where you're certain you want to delete it regardless of its merge status, you can use:
git branch -D branch_name
The `-D` option forces the deletion of the branch, bypassing the merge-check. Use this command with caution, as it may result in the loss of uncommitted changes.
Checking Existing Local Branches Before Deleting
Before proceeding with the deletion, it’s wise to list all existing local branches to ensure you’re deleting the correct one. You can do this with:
git branch
This command will display a list of your local branches, allowing you to double-check your target branch.
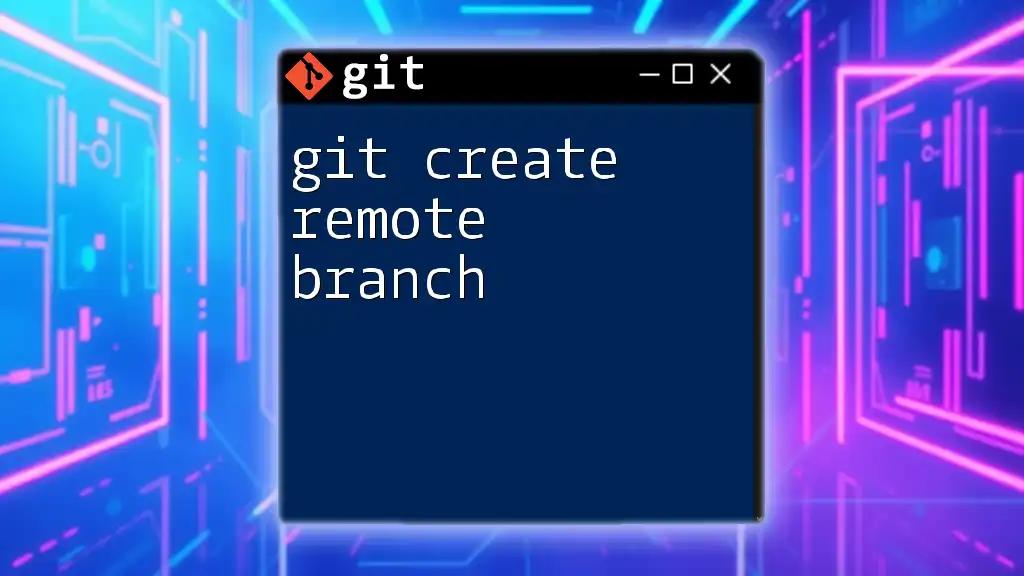
How to Delete a Remote Branch
Basic Command to Delete a Remote Branch
If you're ready to clean up remote branches as well, the command to remove a remote branch is:
git push origin --delete branch_name
Here, `origin` is the default name for your remote repository, and `--delete` specifies that you want to remove the branch.
Checking Existing Remote Branches Before Deleting
Before deleting a remote branch, it is essential to verify its existence. You can list all remote branches using:
git branch -r
This command will show you all the branches on the remote, ensuring you are targeting the correct one for deletion.
Understanding Remote Branch Deletion Impact
Deleting a remote branch can affect other team members who may be relying on that branch. It’s important to communicate with your team regarding any deletions, especially if someone has pending work or is unaware of the deletion. Doing so can prevent potential confusion or loss of work.
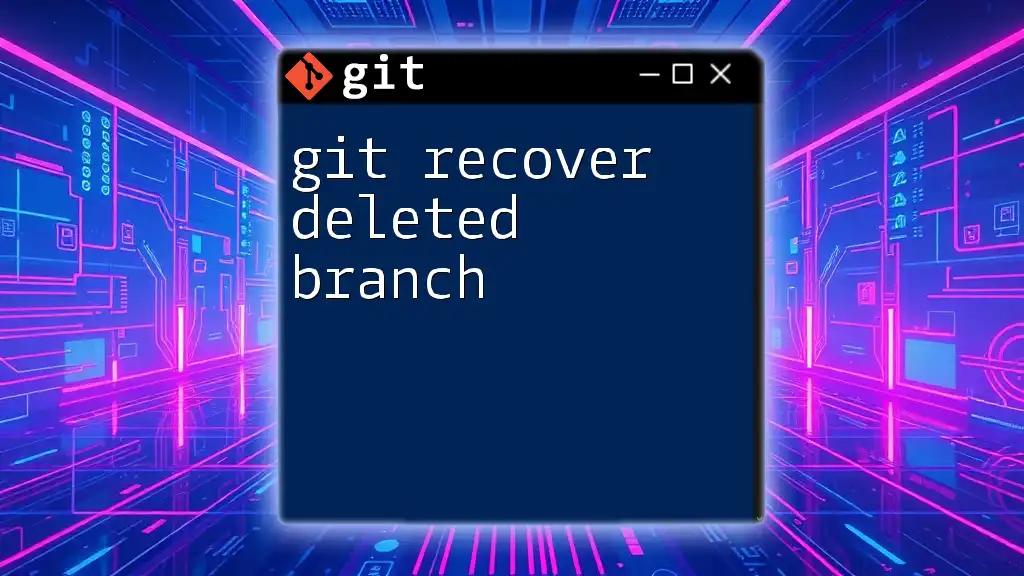
Best Practices for Branch Management
Regular Maintenance of Branches
To maintain an organized repository, regularly review your branches. This involves assessing which branches are active, which have been merged, and which are no longer needed. Performing periodic clean-ups will help keep your project streamlined.
Guidelines for Branch Deletion
When deleting branches, consider the following best practices:
- Ensure you have merged any vital changes before deletion.
- Communicate with your team about upcoming deletions.
- Document deletions for future reference, especially in collaborative environments.
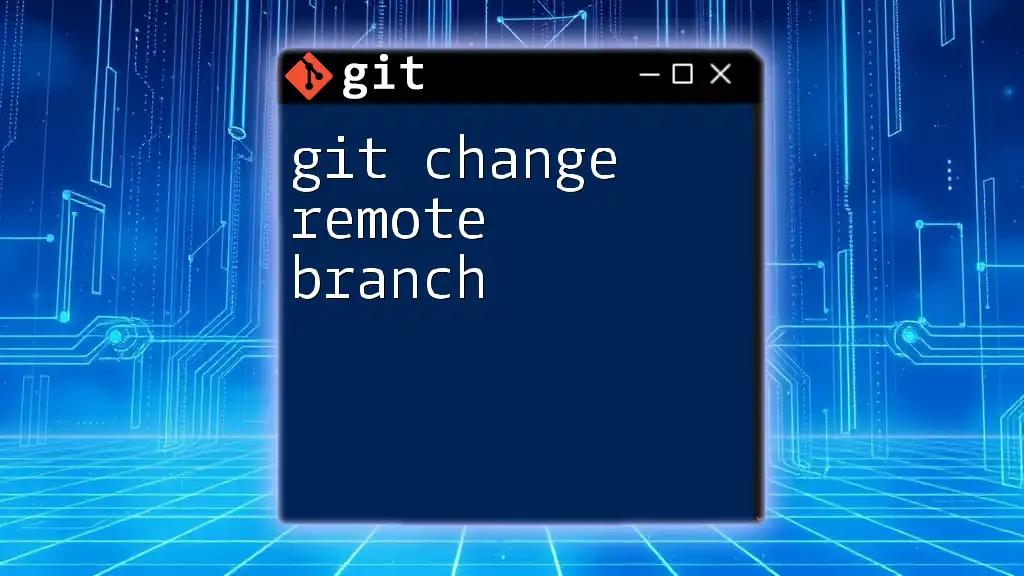
Conclusion
Deleting branches is an integral aspect of Git management. Understanding the appropriate commands and their implications can significantly enhance your workflow. By effectively utilizing the git command to delete a branch, you not only keep your repository clean but also contribute positively to your team's collaboration. Embrace these practices, and evolve your Git skills to be a more proficient version control user.
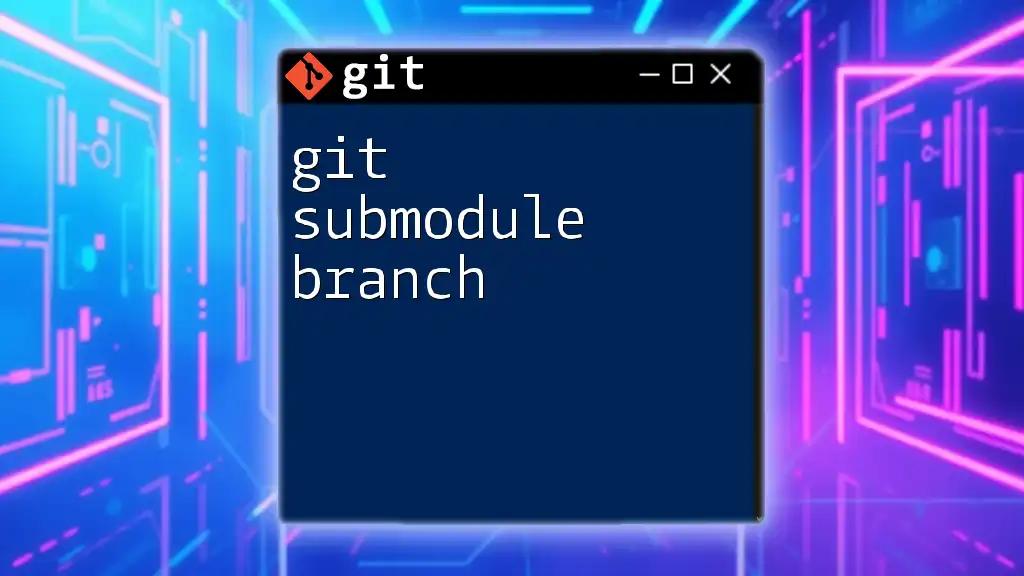
Additional Resources
For further learning, consider exploring the official Git documentation. Engaging with tutorials or Git GUI tools can also enhance your understanding and execution of branch management tasks in Git.