To clone a specific remote branch from a Git repository, use the following command that specifies the branch and the repository URL.
git clone -b branch-name --single-branch https://github.com/username/repository.git
Understanding Git Cloning
What is Cloning?
Cloning in Git refers to creating a local copy of a remote repository. When you clone a repository, you are not only getting the files but also the entire version history. This is crucial for collaboration, as it allows multiple developers to work on the same codebase. Unlike forking, which is a more isolated copy used mainly for contributors to propose changes, cloning provides full read-write access to the repository.
Why Clone a Remote Branch?
There are several key reasons for cloning a remote branch:
- Access to the Latest Code: Cloning enables you to work with the most current version of the code, including all recent commits and updates.
- Contributing to Ongoing Projects: If you are working in a team, cloning a remote branch allows you to integrate your changes readily and push back your contributions.
- Testing Features from Remote Branches: You can explore and experiment with features that might not yet be merged into the primary codebase, allowing you to provide feedback or simply test new functionalities.
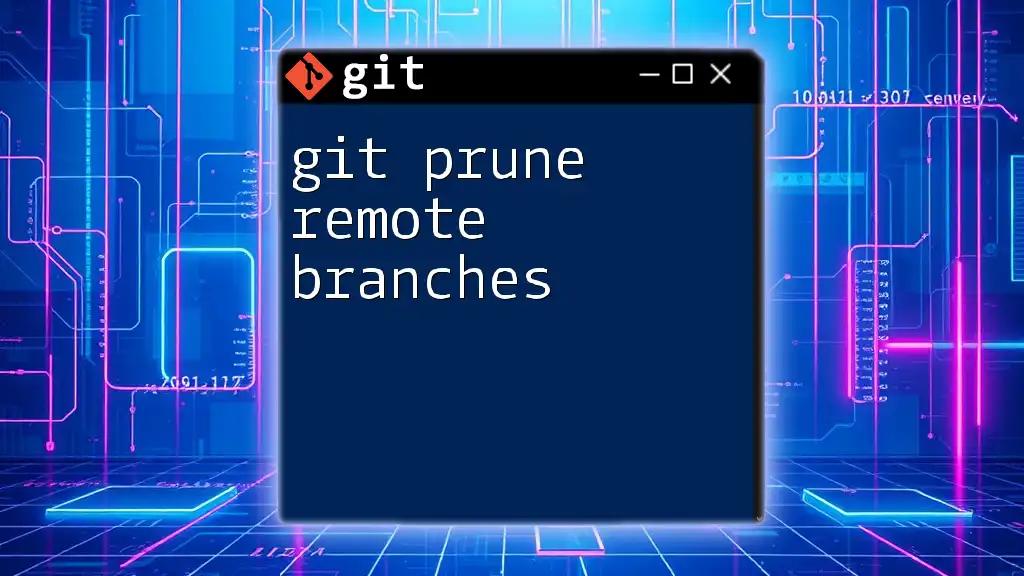
Prerequisites
Basic Git Knowledge
To effectively utilize the `git clone remote branch` functionality, it's essential to have a basic understanding of Git commands and workflows. Familiarity with concepts such as commits, branches, and remotes will facilitate a smoother experience as you navigate through the topic.
Setting Up Your Environment
Before you start using Git, it needs to be installed and configured on your machine. Here’s how:
-
Install Git for your operating system.
- For Windows, you can download the installer from the official Git website.
- For macOS, use Homebrew:
brew install git
- For Linux, you can use your package manager, e.g.,
sudo apt-get install git
-
Configuring Git User Settings is necessary to commit changes with your identity:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
Proper configuration ensures that your commits are attributed correctly.
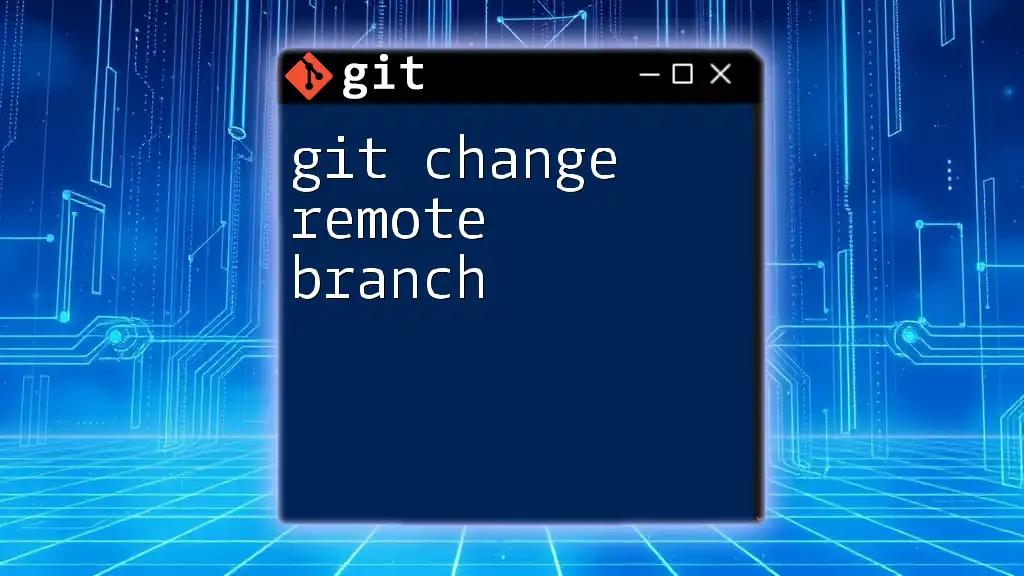
Cloning a Remote Repository
Cloning the Entire Repository
To clone a repository, you’ll use the `git clone` command. This command will retrieve all the files, along with the entire history of commits and branches. The basic syntax is as follows:
git clone <repository-url>
For example:
git clone https://github.com/user/repository.git
By executing this command, you’ll create a local copy of the entire repository in a directory named after the repository.
Cloning a Specific Branch
Understanding Branches in Git
Branches are used in Git to create separate lines of development. They allow developers to work on different features without interfering with the main codebase. Cloning a specific branch can save time and keep your environment clean, especially if the repository has multiple branches, but you only need one.
Syntax for Cloning a Specific Branch
To clone a specific branch rather than the entire repository, you can use the `-b` or `--branch` option. The following syntax will let you clone just the branch you intend to work on:
git clone -b <branch-name> <repository-url>
For example:
git clone -b feature-branch https://github.com/user/repository.git
By using this command, you will only clone the specified branch along with its commit history, allowing for a more focused development experience.
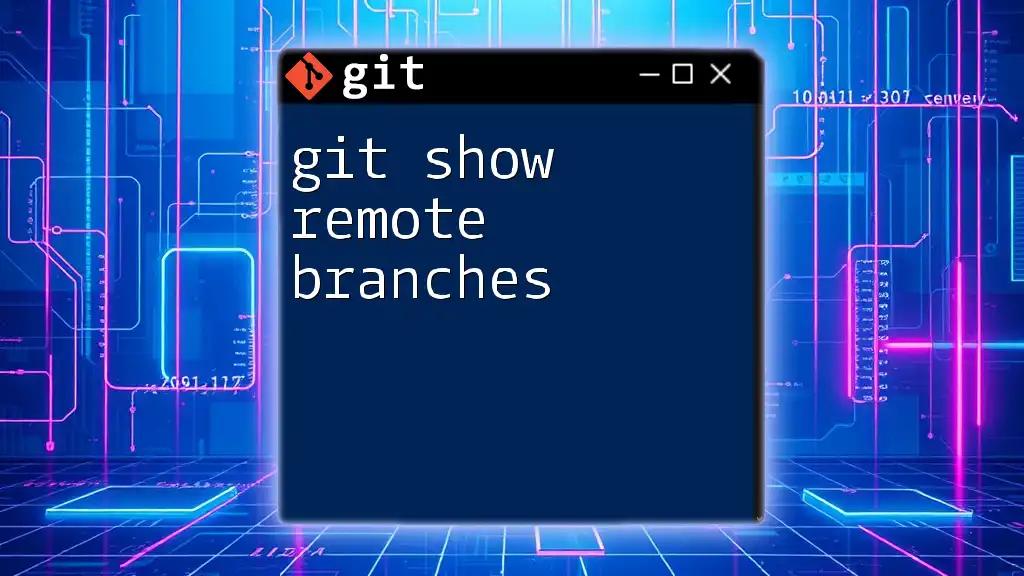
Working with Cloned Branches
Verifying Your Clone
After cloning a remote branch, it’s essential to verify that the process was successful. You can do this by checking the list of branches:
git branch -a
This command will show all local and remote branches, helping you confirm which branch you are currently on.
Making Changes and Committing
Once you’ve verified your cloned branch, you’re ready to make changes. Here’s a basic workflow:
- Make changes to the files you need.
- Stage the changes using:
git add <file-name>
- Commit the changes with a message:
git commit -m "Your commit message"
These three steps form the core of Git workflow—modifying files, preparing them for commit, and then committing them to save the changes.
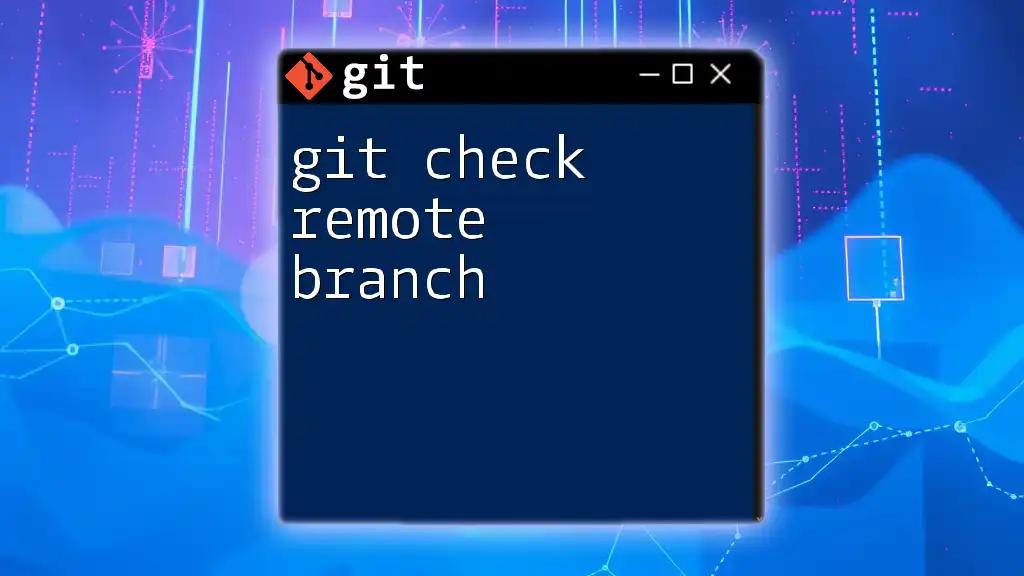
Common Issues and Troubleshooting
Unable to Clone Repository
Sometimes you might face errors while trying to clone a repository. Common issues include permission errors, incorrect repository URLs, or network connection problems. When these errors occur, pay close attention to the message displayed, as it often contains hints on how to resolve the issue.
Working with Detached HEAD
After cloning a specific branch, you might find yourself in a detached HEAD state. This happens when you check out a commit that's not at the tip of a branch. If you attempt to make changes in this state, your commits won’t belong to any branch. To avoid or resolve this:
- Always ensure that you’re on a branch before making changes:
git checkout <branch-name>
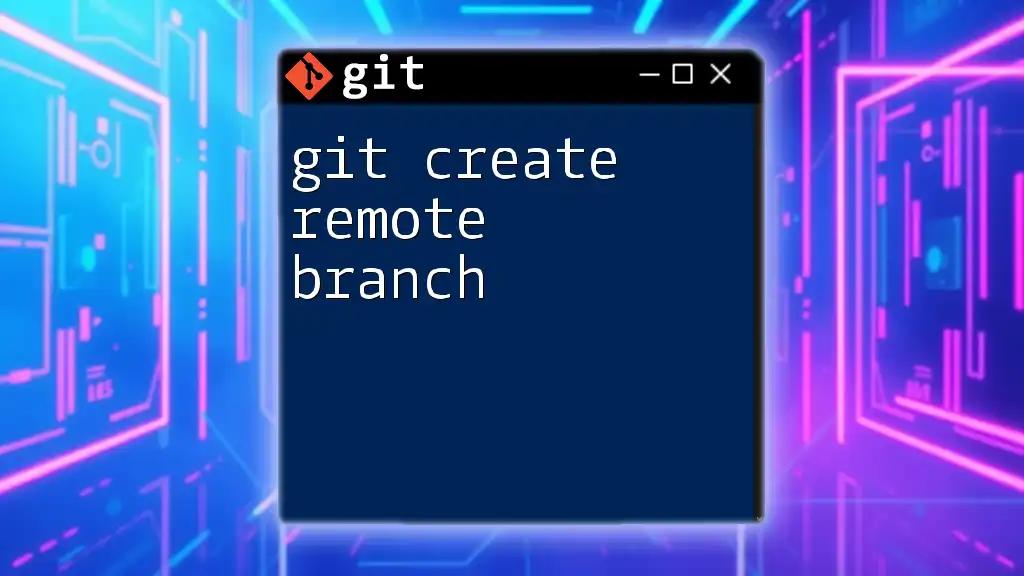
Best Practices for Cloning Remote Branches
Choosing the Right Branch to Clone
Before cloning, consider the following tips:
- Evaluate the project’s branching model: Understand which branches are stable or intended for feature development.
- Communicate with your team: Make sure you are cloning branches that align with your workflow and contribution plans.
Staying Updated After Cloning
Once you have cloned a repository and are working in it, it's important to stay updated with the latest changes from the remote branch. You can do so using:
git pull origin <branch-name>
Regularly pulling updates ensures that your local instance reflects the most recent developments, reducing the likelihood of conflicts when pushing your changes.
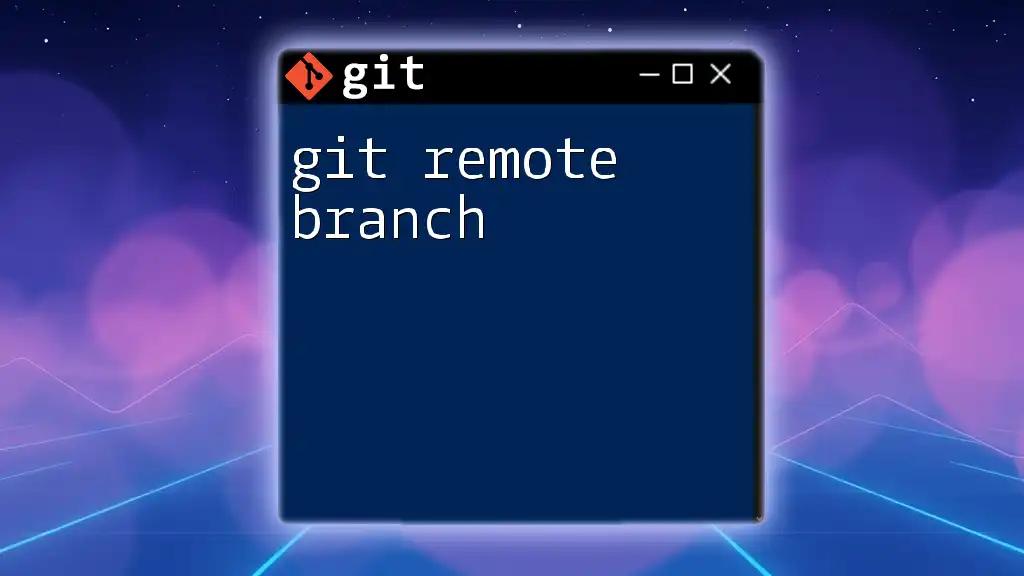
Conclusion
In this guide, we've explored the essential aspects of git clone remote branch. From understanding the cloning process and best practices to troubleshooting common issues, you’re now equipped with the knowledge to use Git effectively. Commit to practicing these commands and workflows; you’ll develop your skills and confidence over time. If you have questions or feedback, feel free to reach out!
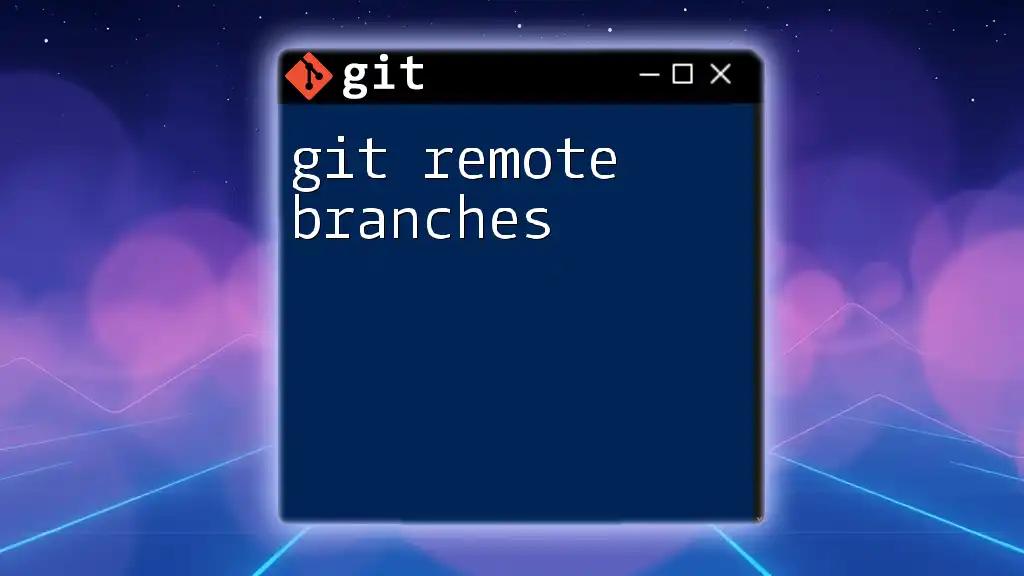
Additional Resources
For further reading and exploration, consider checking the following resources:
- [Official Git Documentation](https://git-scm.com/doc)
- Recommended Git tutorials and courses that deepen your understanding and mastery.