To update your local remote branch to reflect the latest changes from the corresponding remote branch, you can use the following command:
git fetch origin && git merge origin/branch-name
Replace `branch-name` with the name of the remote branch you wish to update.
Understanding Remote Branches
What Are Remote Branches?
Remote branches in Git are essentially versions of your repository hosted on a server. They serve as a point of reference for multiple collaborators working on a project. While local branches exist on your machine, remote branches provide a collaborative framework to ensure everyone on your team can see and communicate changes effectively.
Key Concepts
It's crucial to differentiate between remote and local branches. The remote is the repository that exists on services like GitHub or Bitbucket, while your branch is a parallel line of development. To effectively manage remote branches, several commands are vital, including `git fetch`, which updates your local copy of the repository without merging any changes, and `git pull`, which does both fetching and merging in one go.
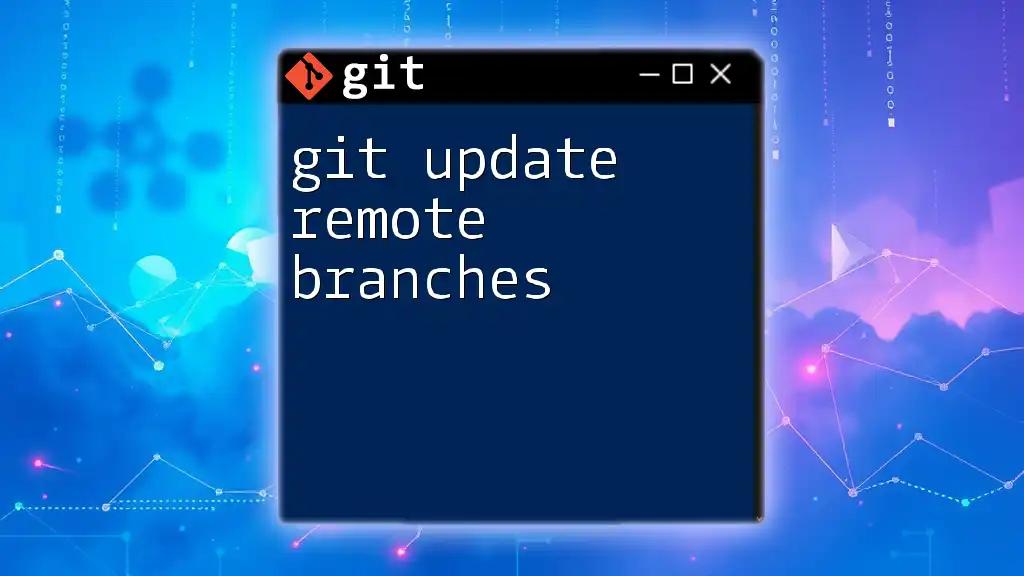
Why Update Remote Branches?
Importance of Synchronization
Keeping your remote branches updated fosters an undistracted workflow. When team members regularly synchronize their changes, everyone stays aligned, reducing the potential for conflicts. Imagine a scenario where several developers update the same file in different ways; without regular updates, these differences can create major headaches when it comes time to merge.
Scenarios for Remote Updates
You may often find yourself needing to update remote branches during collaboration. Whether implementing new features or fixing bugs, regularly updating your branches ensures that you always work with the most current codebase. Additionally, reviewing pull requests will be seamless if your branches are up-to-date, as you’ll be checking against the latest commits.
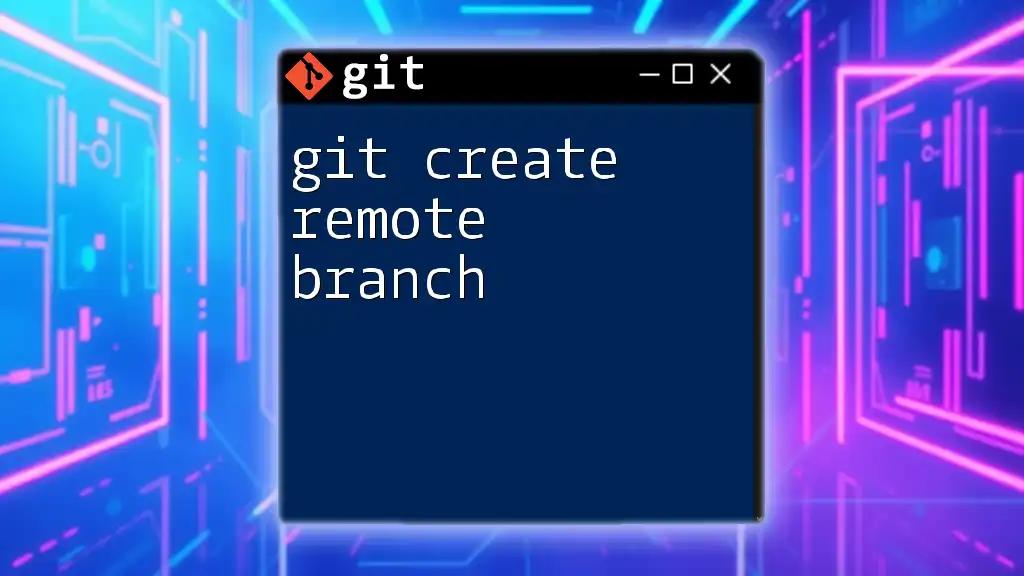
How to Update a Remote Branch
Basic Steps Overview
To efficiently update a remote branch, you need to follow these three basic steps:
- Fetch changes from the remote repository.
- Merge the fetched changes into your local branch.
- Push your updated local branch back to the remote.
Step 1: Fetching Changes
The first step in updating your remote branch is to retrieve any changes made by your collaborators. You can do this by running:
git fetch origin
Using `git fetch` will pull down the latest changes from the remote repository, updating your local references without altering your working directory. For example, imagine you’re collaborating on a team project; running this command allows you to see any new features or bug fixes others have added.
Step 2: Merging Changes
After fetching the changes, the next step is to merge those changes into your local branch. This can be accomplished with the following command:
git merge origin/<branch-name>
For example, if you have fetched changes from the main branch, you would execute:
git merge origin/main
This command takes the updates you just fetched and integrates them into your current local branch. Note that you may run into merge conflicts if changes clash with local edits, which brings you to the next section on handling conflicts.
Step 3: Pushing Changes to Remote
After successfully merging any updates, it’s important to push your changes back to the remote repository. This ensures that everyone else on your team can access your latest work. You can do this by running:
git push origin <branch-name>
For example, to push your local changes on a feature branch called `feature-xyz`, you would execute:
git push origin feature-xyz
This command synchronizes your local work with the remote repository, thus updating the branch for all collaborators to see.
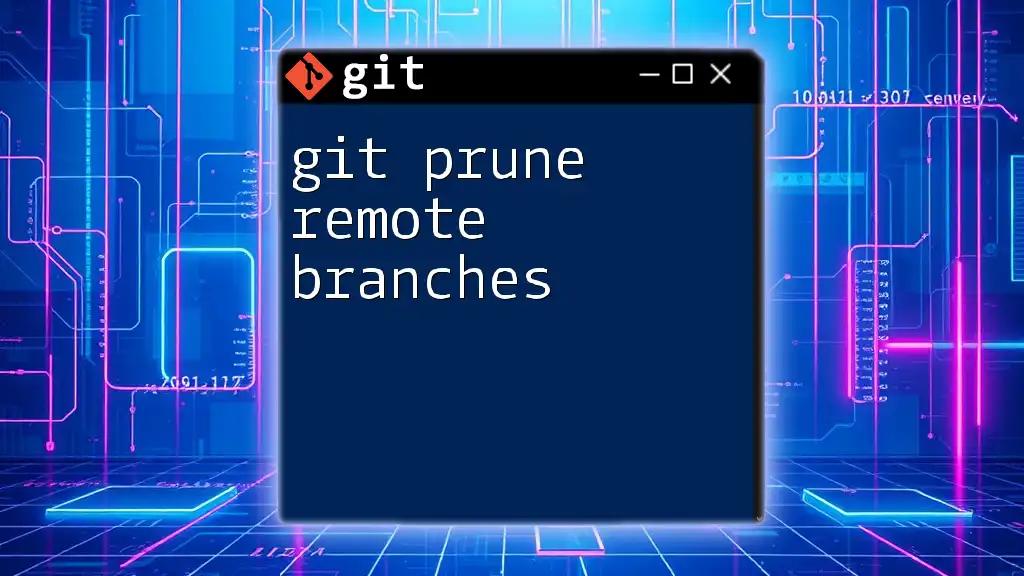
Advanced Techniques for Updating Remote Branches
Using Git Pull
If you prefer a more streamlined approach, you could utilize the `git pull` command, which fetches and merges in a single step:
git pull origin <branch-name>
For instance, if you wanted to update your local version of the main branch, you would type:
git pull origin main
This method is quicker but may make it harder to identify the source of issues if conflicts arise.
Handling Conflicts
When merging or pulling changes, you might encounter conflicts. A conflict occurs when two developers edit the same file differently. Here’s how you can handle it:
-
Identify Conflicting Files: Git will notify you about files that have conflicts.
-
Edit the Files: Open the conflicting files to resolve the issues. You'll see conflict markers that indicate the differing changes.
-
Stage and Commit: Once resolved, stage the changes with:
git add <conflicting-file>
Then, commit your changes:
git commit -m "Resolved merge conflicts."
By carefully reviewing and editing conflicting files, you preserve the integrity of your coding decisions.
Rebase vs Merge
What is Git Rebase?
Rebase is an alternative approach to updating your branch, offering a cleaner project history. Using the command:
git rebase origin/<branch-name>
allows you to move your commits on top of the latest changes from the `origin`. This method re-applies your local changes after the external changes, presenting a linear commit history.
When to Use Rebase
Rebase is particularly useful when you want to maintain a cleaner git history without all the 'merge' commits. For example:
git rebase origin/main
This command will rewrite the history of your current branch, ensuring that your commits effectively follow the latest changes made by others.
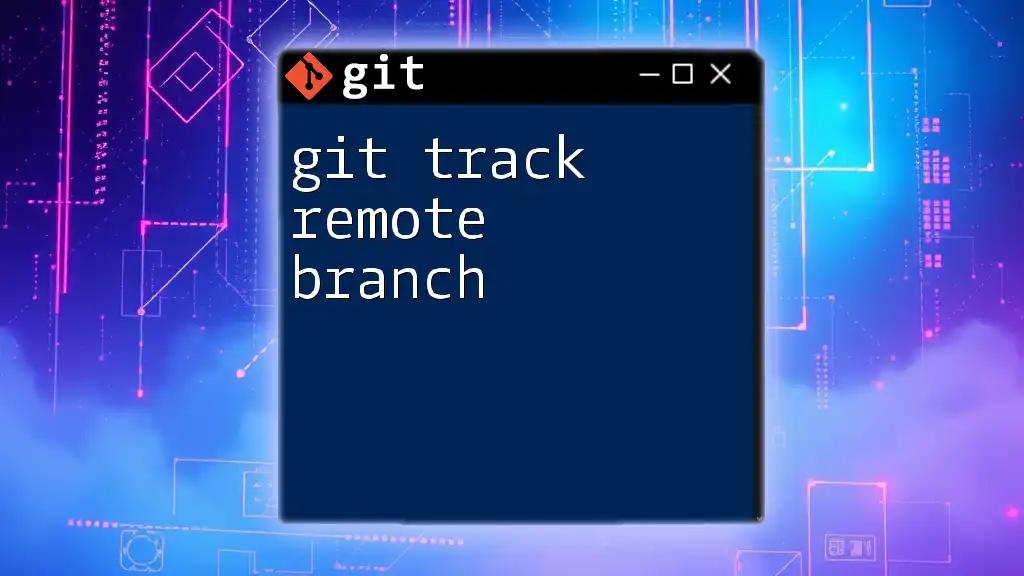
Best Practices for Maintaining Remote Branches
Regularly Update Your Local Branch
To prevent large merge conflicts and lost work, it's advisable to frequently update your local branches. Set a routine—at least daily or per sprint—to ensure you receive the latest updates from your collaborators. This practice fosters communication and allows for smoother collaboration.
Collaborating with Others
Open lines of communication are vital when you're working with a team. Regularly update your teammates on the status of branch changes and commit progress to ensure everyone is on the same page. A centralized communication platform, like Slack or Microsoft Teams, can facilitate this exchange.
Naming Conventions
Establish clear and consistent naming conventions for branches. Descriptive names—like `feature/add-login`, `bugfix/fix-header`—help everyone on the team quickly understand the purpose of each branch. This simple step can significantly enhance collaboration and project management.
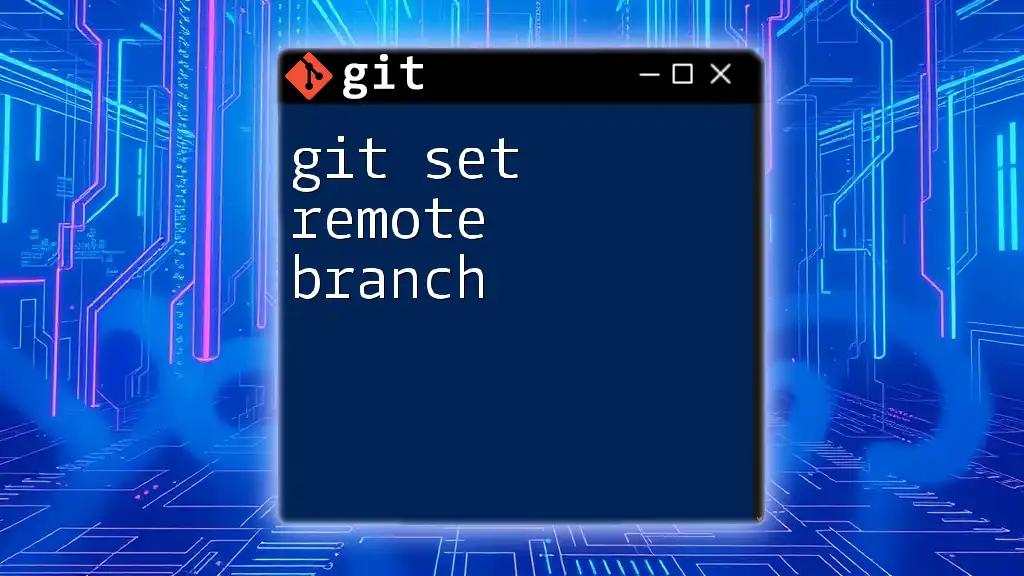
Conclusion
In summary, keeping your remote branches updated is a fundamental skill for any Git user, especially when working in a team. By understanding how to efficiently fetch, merge, and push changes, you foster a productive environment where project development can flourish. Regular practice of these techniques will lead to more cohesive collaboration and fewer conflicts in your projects.
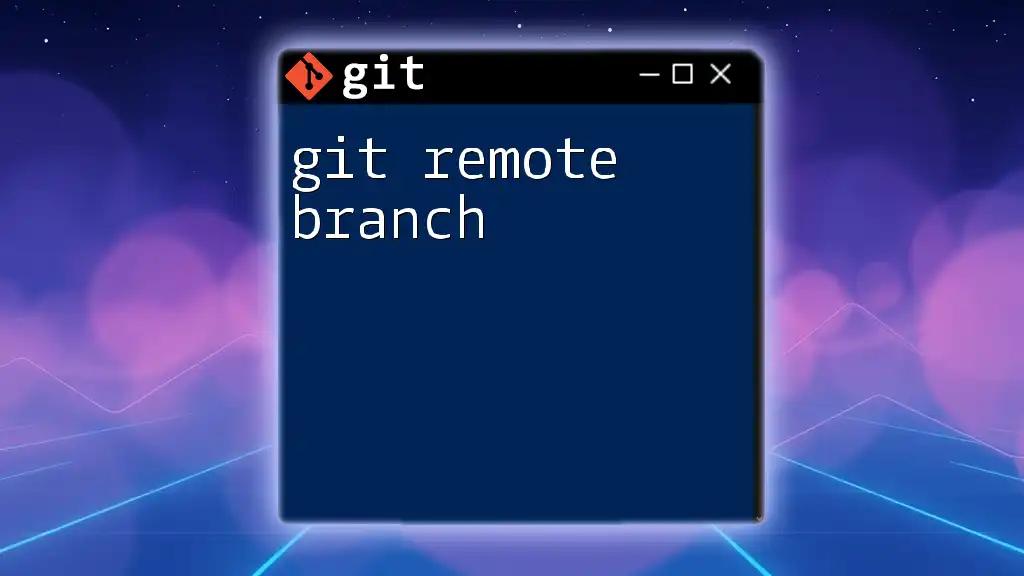
Additional Resources
For further learning, consider diving into the official Git documentation. There you will find comprehensive guides and tutorials that can enrich your understanding. Moreover, exploring courses related to Git can enhance your skills and confidence in using version control effectively.
By mastering the process of updating remote branches, you can significantly improve your workflow and collaboration with your team.