To track a remote branch in Git, you can create a local branch that tracks a remote one using the following command:
git checkout -b local-branch-name origin/remote-branch-name
Understanding Remote Branches
What is a Remote Branch?
A remote branch in Git is a pointer to the state of branches in a remote repository. It serves as a reference to how the code on your local machine compares with the code on the repository hosted remotely, such as GitHub or Bitbucket.
Understanding the difference between local and remote branches is crucial:
- Local Branches: These are branches that exist on your local machine, where you can make changes, commit, and test your code.
- Remote Branches: These are branches that exist on your remote repository. They are often prefixed with the name of the remote (e.g., `origin/branch-name`).
Why Track Remote Branches?
Tracking remote branches is essential for collaborative workflows in Git. When you track a remote branch, you can:
- Automatically stay updated with changes made by others.
- Make and push changes to the specific branch you're monitoring.
- Resolve conflicts more effectively by being aware of incoming changes.
For example, in a team project, if several developers are working on the same branch, tracking that remote branch helps you see what modifications they have made before you push your own.
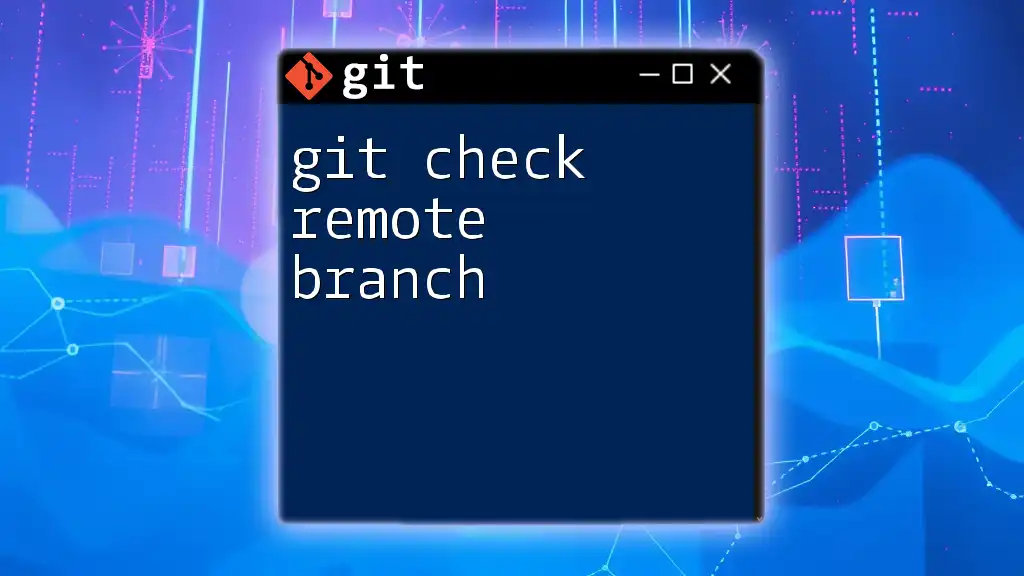
Setting Up Your Local Repository
Cloning a Repository
To begin tracking a remote branch, you first need to set up a local copy of your repository. This is typically done using the `git clone` command, which copies the entire repository to your local machine, including all branches.
git clone https://github.com/username/repo.git
This command creates a directory with the project name and downloads all files and branches from the remote repository, making it easy for you to start working right away.
Viewing Remote Branches
Once you have cloned your repository, you might want to see which remote branches are available. You can use:
git branch -r
This command lists all remote branches. The output will show names like `origin/branch-name`, where `origin` is the default alias for your remote repository. Understanding this naming convention is key to successfully tracking your desired branch.
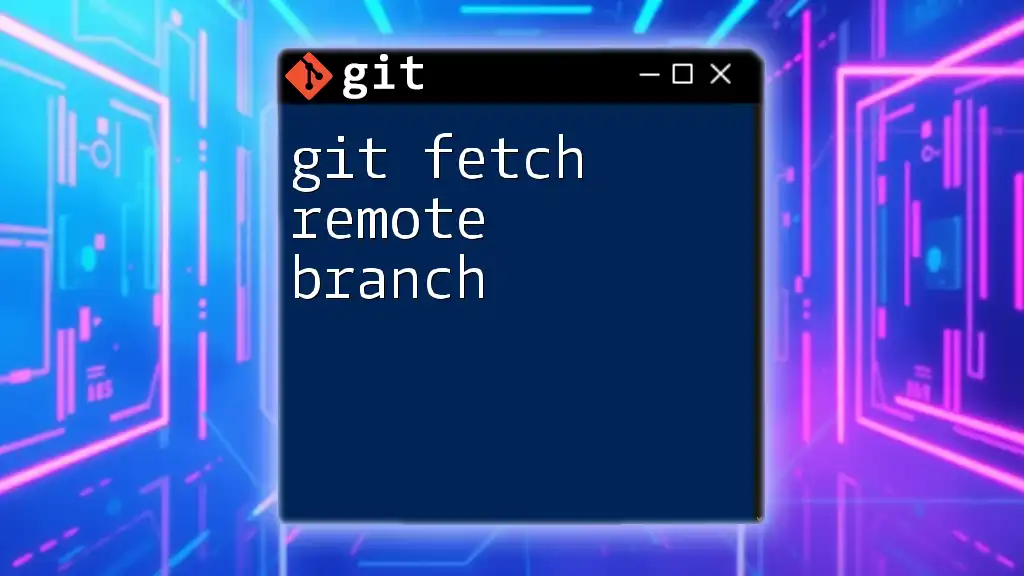
Tracking a Remote Branch
How to Track a Remote Branch
To track a remote branch, you’ll need to create a local branch that is linked to it. You can do this using the `git checkout` command with the `-b` flag.
git checkout -b local-branch-name origin/remote-branch-name
In this example, `local-branch-name` will be your new branch name on your local machine, while `origin/remote-branch-name` indicates the remote branch you’re tracking. This command sets up your local branch to track the specified remote branch, so you'll automatically fetch changes from it.
Verifying Tracking Connection
After creating your tracking branch, you should verify that everything is set correctly. You can use:
git branch -vv
The output will show you the local branches alongside the corresponding remote branches they track. If you see the correct remote branch associated with your local branch, you’re all set to begin working!
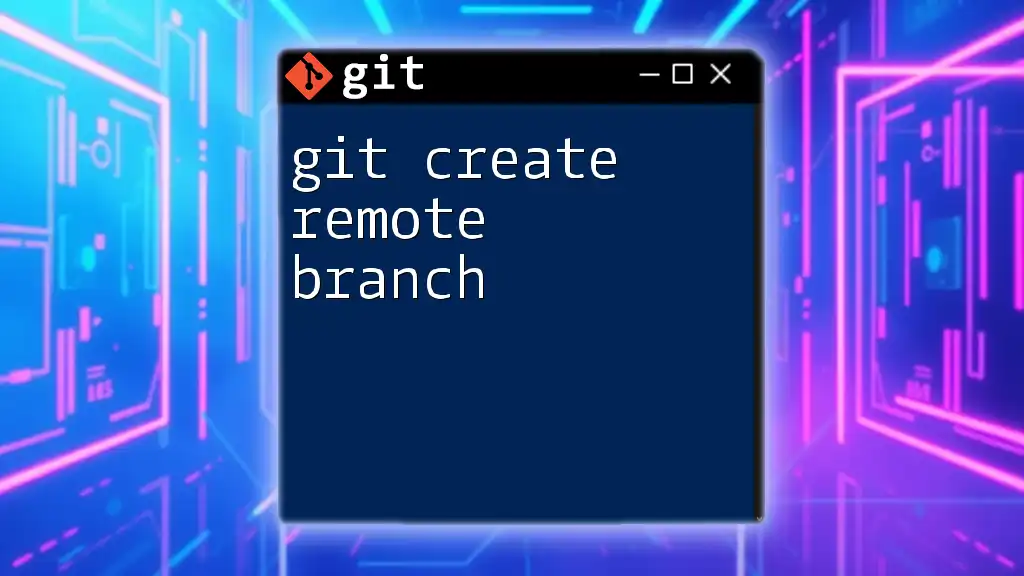
Working with a Tracked Remote Branch
Pulling Changes from the Remote Branch
To stay updated with the latest changes in the remote branch, use the `git pull` command. This fetches any changes from the remote branch and merges them into your local tracking branch.
git pull origin remote-branch-name
Regularly using `git pull` helps prevent conflicts and ensures everyone on the team is synchronized. It's a best practice to pull changes often, especially before starting new work.
Pushing Local Changes to the Remote Branch
Once you've made changes locally, you may want to push them to the remote branch. Use the following command to do so:
git push origin local-branch-name
This pushes your local changes to the corresponding remote branch. Remember, it's essential to pull changes before pushing to avoid potential merge issues.
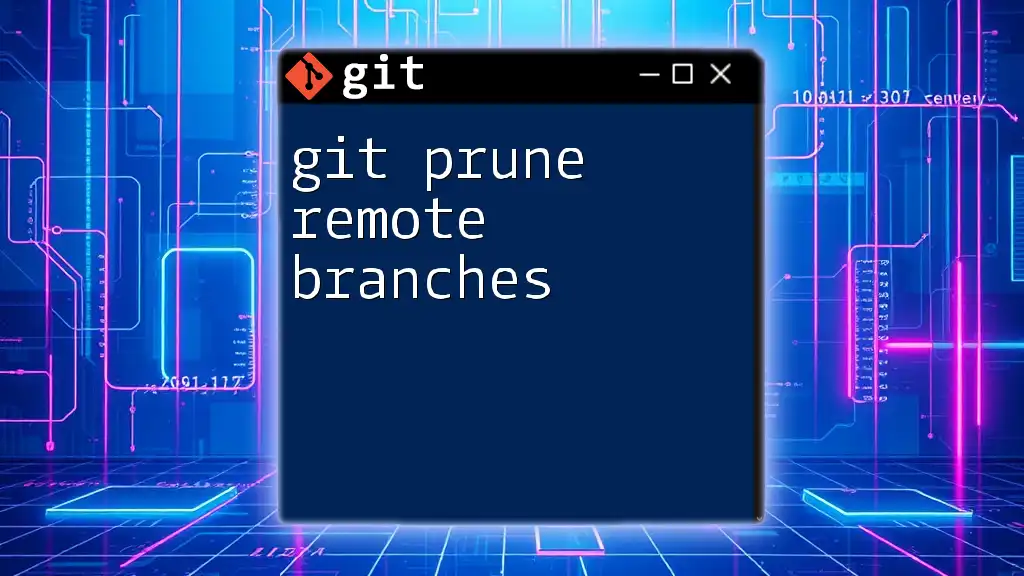
Advanced Tracking Techniques
Switching Tracking Branches
If you need to switch which remote branch you’re tracking, Git allows you to do that too. You can change the upstream branch using:
git branch --set-upstream-to=origin/new-remote-branch local-branch-name
This command is helpful if you initially set your local branch to track a different remote branch or if the development focus shifts.
Deleting Remote Branches
As development progresses, you may find that certain remote branches become obsolete. You can delete branches both locally and remotely.
To delete a local branch that is no longer needed, you would use:
git branch -d local-branch-name
And to remove the branch from the remote repository, use:
git push origin --delete remote-branch-name
Cleaning up branches helps keep your repository organized and reduces confusion about which branches are active.
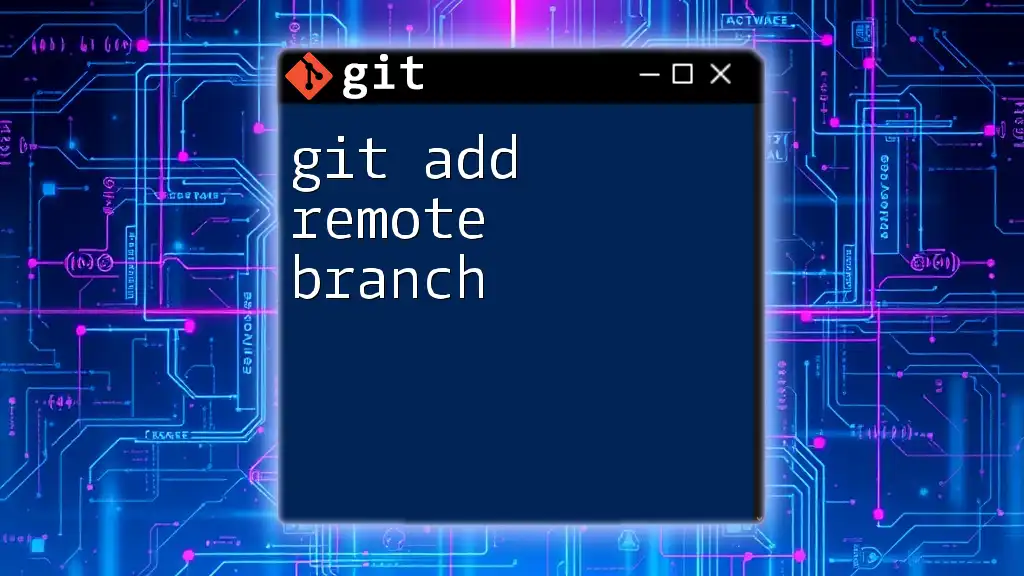
Troubleshooting Common Issues
Conflicts while Pulling
One common issue developers encounter is merge conflicts when pulling changes from a tracked remote branch. Conflicts occur when changes in the remote branch intersect with changes you’ve made locally.
Git will pause the pull process and flag the conflicting files. You will need to:
- Manually resolve the conflicted files.
- Add the resolved files using `git add`.
- Commit your changes to complete the merge.
Tracking Misconfigurations
Sometimes, you might find that a local branch is not properly tracking its remote counterpart. This could result from incorrect setup or manual adjustments in the repository.
You can fix tracking configurations by confirming the upstream branch with:
git branch -vv
If your local branch is not tracking correctly, you can set it again with the `--set-upstream-to` command as shown earlier.
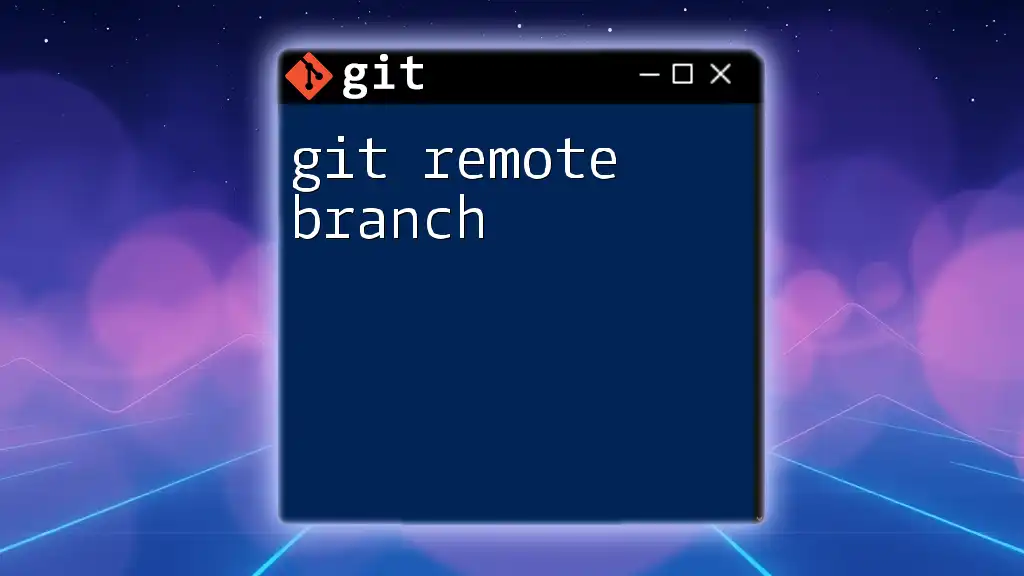
Conclusion
Tracking remote branches in Git enables collaborative workflows, allowing teams to stay synchronized and productive. Mastering the commands to clone, track, pull, and push changes is essential for anyone using Git effectively.
By following the outlined techniques in this guide, you can ensure seamless collaboration with your team and manage your Git repositories efficiently. Happy coding!