The `git check-remote-branch` command is used to verify the remote branches available in your repository, allowing you to quickly see which branches are on the remote server.
Here's how you can view the remote branches:
git branch -r
Understanding Remote Branches
What Are Remote Branches?
Remote branches are references to the state of branches in a remote repository. They serve as pointers that keep track of the latest commits that the remote branches contain. By understanding remote branches, developers can effectively collaborate on a shared codebase, ensuring that everyone is aligned with the most current state of the project.
Why Check Remote Branches?
Checking remote branches is crucial for a multitude of reasons. It allows developers to:
- Stay Informed: Understand what changes have been made by collaborators without pushing or pulling updates.
- Merge Effectively: Avoid potential merge conflicts by being aware of changes made in the remote branches before finalizing local changes.
- Maintain Code Quality: Ensure that your commits are cohesive and do not clash with ongoing developments in the remote repo.
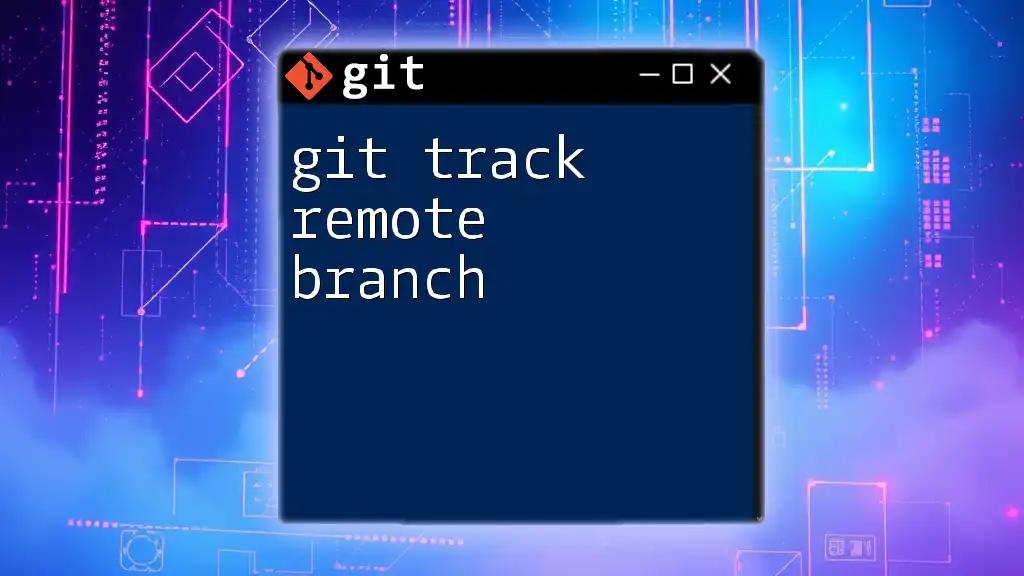
Basic Git Commands for Remote Branches
How to List Remote Branches
To quickly see what branches are available on the remote repository, you can use the `git branch -r` command. This command lists all remote branches that exist.
git branch -r
When executed, you will see output similar to this:
origin/HEAD -> origin/main
origin/main
origin/development
origin/feature-xyz
This output provides a snapshot of all branches stored in the remote repository, helping you identify what collaborative efforts are currently active.
Fetching Remote Branches
Before checking or working with remote branches, it’s often necessary to update your local references to reflect any changes that have occurred on the remote. The `git fetch` command serves this purpose by downloading objects and refs from the remote repository.
git fetch origin
Fetching does not modify your working directory or stage any changes; it merely updates your local tracking branches and is a safe way to ensure that you are viewing the most recent updates.
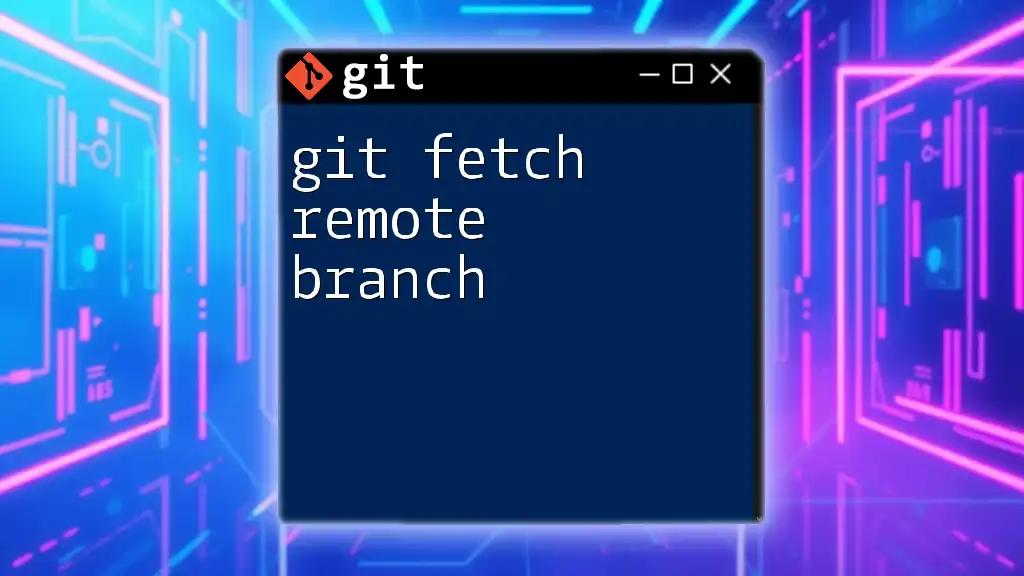
Detailed Guide on Checking Remote Branches
Viewing Remote Branches with Tracking Information
Understanding Tracking Branches
A tracking branch is a local branch that is linked to a remote branch. This linkage means that you can easily synchronize your changes with the remote counterpart, receiving notifications if it's ahead or behind.
Check Tracking Branches
To view your local branches along with the remote ones they are tracking, you can use the `git branch -vv` command.
git branch -vv
This command will display a list not only of your branches but also provide information on the remote tracking branches, indicating which ones are ahead, behind, or up to date.
Comparing Local and Remote Branches
Using `git log` to Compare
To check the differences between your local branch and the remote one, you can utilize `git log`. For instance, if you want to see changes that are present in the `origin/main` branch but not in your local `main`, you would use:
git log origin/main..main
This command will display the commit logs of commits that exist in `main` but do not exist in `origin/main`, letting you identify what new changes or features are present in your branch that aren’t reflected in the remote branch.
Visualizing Differences with `git diff`
Sometimes seeing the actual content differences is essential. By using `git diff`, you can identify the exact changes made in your working files compared to the remote version.
git diff origin/main
This command will reveal differences line-by-line between your local `main` branch and the remote `origin/main` branch, enabling you to review modifications and prepare your code for merging or additional pushes.
Checking Out Remote Branches
How to Checkout a Remote Branch
If you need to transition to a new feature that resides on a remote branch, you can easily do so by checking it out. You'd create a local branch that tracks the remote branch using the following command:
git checkout -b new-feature origin/new-feature
This command creates a new local branch named `new-feature` corresponding to `origin/new-feature`, allowing you to work on this feature locally while keeping it synchronized with the remote branch.
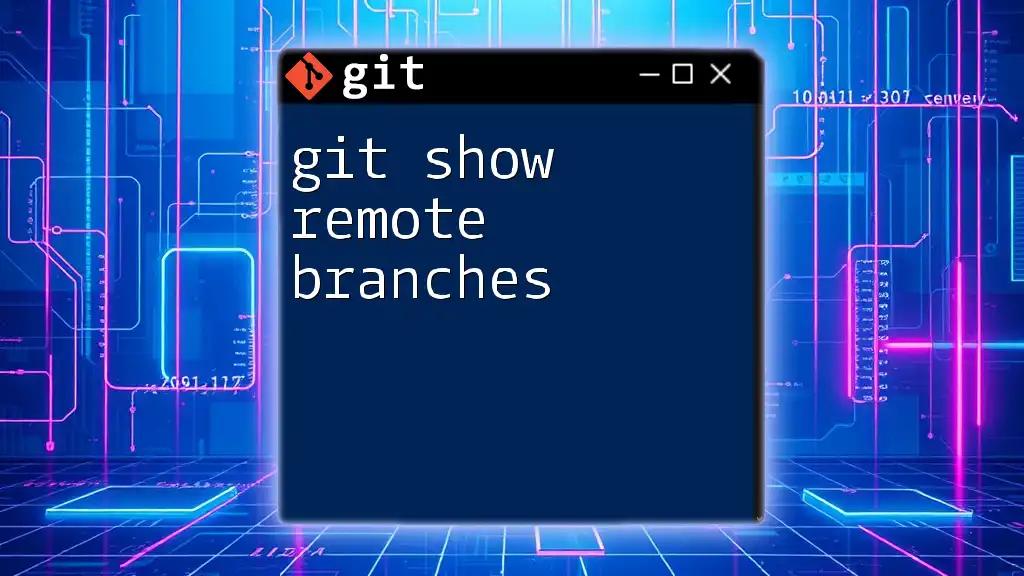
Best Practices for Working with Remote Branches
Regularly Fetching Updates
Frequent fetching ensures that you are aware of the latest changes made by your collaborators. Incorporating a habit of executing `git fetch` before starting new development can save you time and merge conflicts later on.
Periodic Cleanup of Stale Branches
Over time, some remote branches may no longer be needed or may have been deleted from the remote repository. In such cases, it’s useful to clean up your references with the following command:
git remote prune origin
This command removes any remote-tracking branches that have been deleted from the remote, keeping your local repository tidy and relevant.
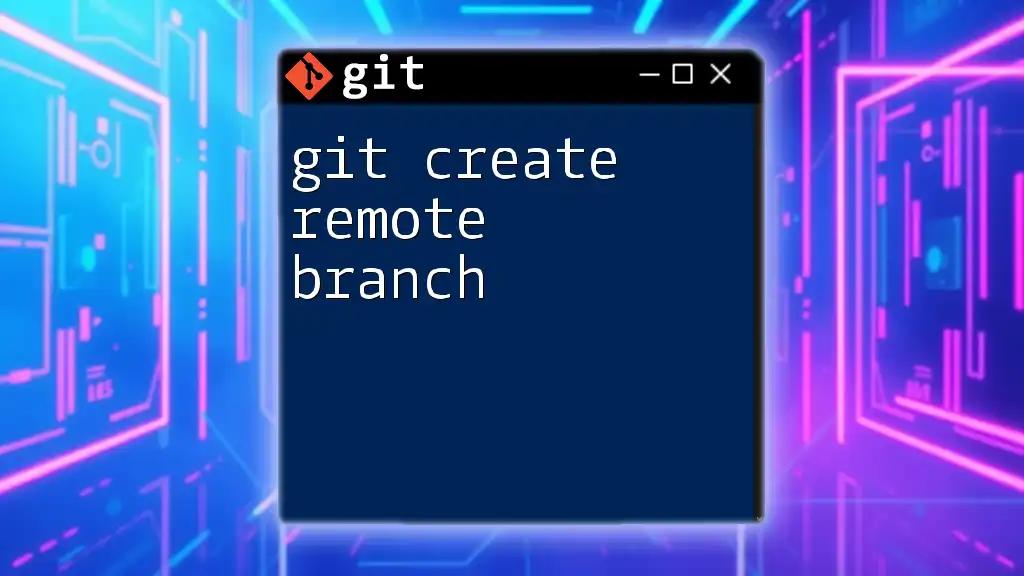
Troubleshooting Common Issues
Resolving Conflicts When Checking Remote Branches
Even the most careful developers can run into conflicts while working with remote branches. These conflicts can arise when you try to merge or rebase changes that are incompatible with what’s currently in the repository.
To mitigate these issues, it’s best to:
- Communicate: Discuss with your colleagues to ensure simultaneous changes don’t overlap.
- Pull Regularly: Update your local branches frequently to incorporate ongoing changes gradually.
- Review Changes: Before performing merge operations, always double-check for differences using `git diff`.
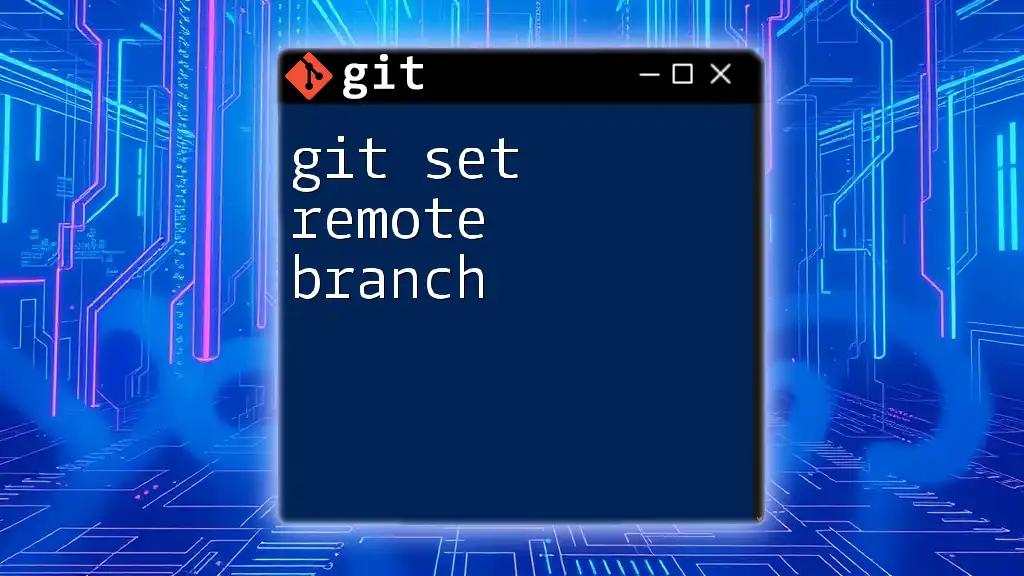
Conclusion
Understanding how to effectively check remote branches using Git commands is vital for maintaining a seamless workflow in team-based projects. By regularly using various commands to list, fetch, and examine remote branches, developers can remain aligned with ongoing work, merge more effectively, and enhance their collaborative coding experience.
For further learning, don’t hesitate to explore official documentation and online resources for a deeper understanding of Git functionality. Happy coding!