To add a remote branch in Git, you can use the `git push` command followed by the `-u` flag to set the upstream branch in one step, connecting your local branch to a branch on the remote repository.
Here's the command:
git push -u origin <branch-name>
Replace `<branch-name>` with the name of your local branch that you want to push to the remote repository.
Understanding Git Remote Branches
What is a remote branch?
A remote branch in Git is a branch that exists on a remote repository (like GitHub, GitLab, or Bitbucket) rather than on your local machine. While local branches correspond to your development work and can be modified freely, remote branches reflect the state of the repository on the server. This differentiation allows developers to collaborate efficiently without conflicts over changes.
Why Use Remote Branches?
Remote branches serve as a crucial component for collaboration in teams. They enable multiple developers to work on different features, fixes, or experiments simultaneously without stepping on each other's toes. By using remote branches, you create a structured environment where changes can be reviewed and integrated before applying them to the main codebase.
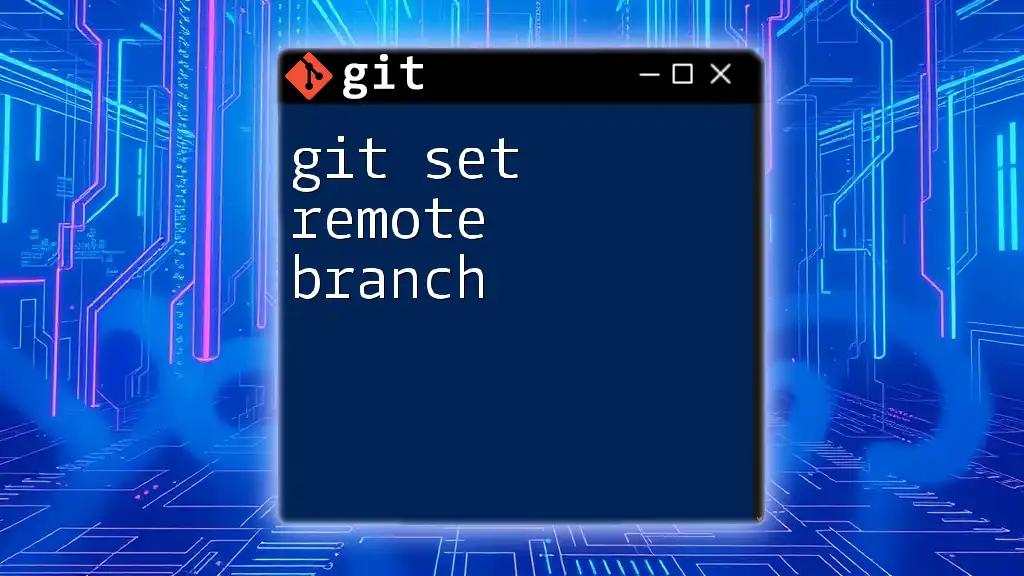
Prerequisites
Basic Git Knowledge
Before diving into adding remote branches, you should have a grasp of basic Git commands. Familiarity with commands like `git commit`, `git push`, and `git fetch` will make this process smoother.
Setting Up Git
To begin, ensure that Git is installed on your system and that you have a local repository set up. You can initialize a repository using:
git init
Alternatively, if you want to clone an existing repository, use:
git clone [repository-url]
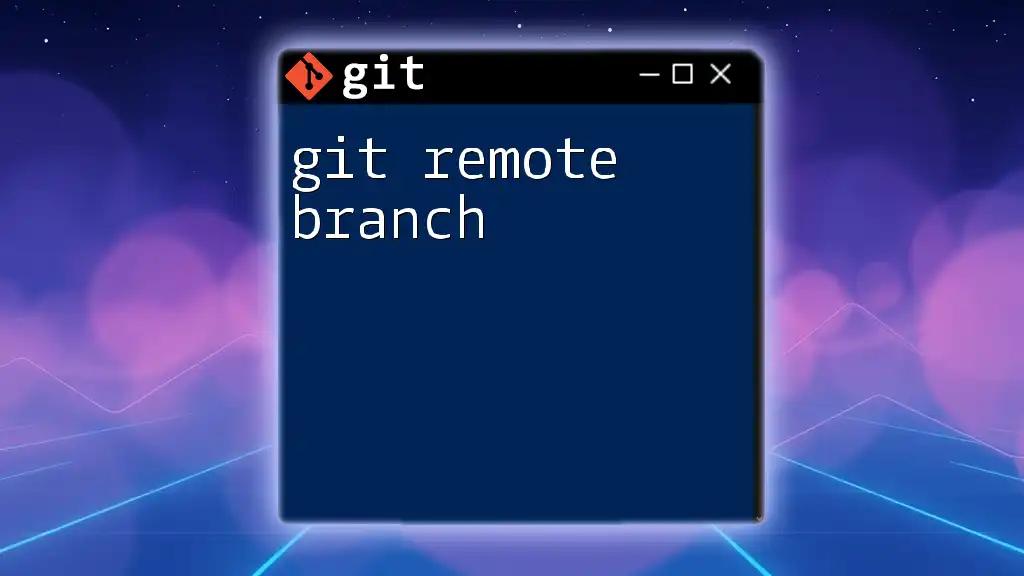
Step-by-Step Guide to Adding a Remote Branch
Step 1: Verify Your Remote Repository
Before adding a remote branch, it's essential to check what remote repositories you are currently connected to. You can do so by executing:
git remote -v
This command will display the names and URLs of the remotes you've configured. For example, you might see:
origin https://github.com/username/repo.git (fetch)
origin https://github.com/username/repo.git (push)
Step 2: Fetching Remote Branches
To ensure you have the latest branches from the remote repository, you should fetch them. This can be achieved with:
git fetch
This command updates your local copy of the repository's branches without merging any changes into your local branches, allowing you to see what has changed on the remote repository.
Step 3: Adding a New Remote Branch
Command: `git branch --track`
Once you know the name of the remote branch you want to track, you can create a corresponding local branch that tracks it. This is done with the following command:
git branch --track [local-branch-name] [remote-name/remote-branch-name]
For example, if you want to track a remote branch called `feature-branch` from the `origin` remote, you would run:
git branch --track feature-branch origin/feature-branch
The `--track` option is beneficial because it sets up your local branch to pull from the specified remote branch seamlessly. This means that when you perform `git pull`, it knows where to fetch the changes from.
Alternative Method: Creating the Local Branch
If you prefer to create a local branch directly while setting it to track the corresponding remote branch, you can use the following command:
git checkout -b [local-branch-name] [remote-name/remote-branch-name]
For instance:
git checkout -b feature-branch origin/feature-branch
This command creates a new branch called `feature-branch` and immediately checks it out.
Step 4: Pushing Your Local Changes to the Remote Branch
Once you've made your changes and are ready to share them with the remote, you need to push them to the remote branch. Use the following command:
git push -u [remote-name] [local-branch-name]
For example, to push your local `feature-branch` to the remote repository, execute:
git push -u origin feature-branch
The `-u` flag is significant as it sets the upstream tracking relationship, allowing you to simply run `git push` or `git pull` in the future without needing to specify the branch again.
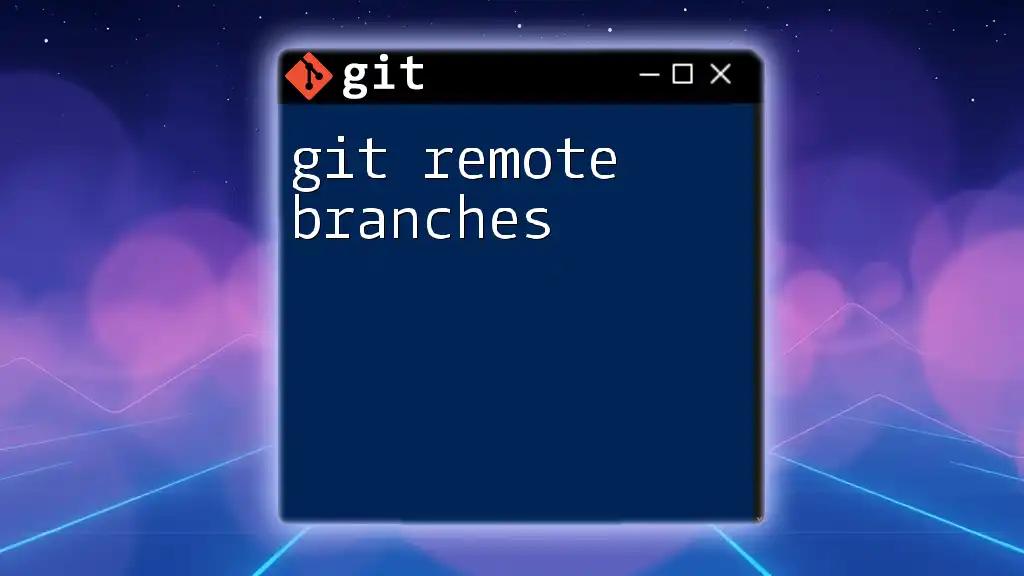
Common Issues and Troubleshooting
Remote Branch Not Found
When trying to add or update a remote branch, you may encounter errors indicating that the branch does not exist. If this occurs, double-check the name of the remote branch using:
git branch -r
This command lists all remote branches to confirm their existence.
Confusion Between Local and Remote Branches
Managing both local and remote branches can be overwhelming, particularly if you're not strict with naming conventions. Employing clear, descriptive names for branches (e.g., `feature/login-page` or `bugfix/header-issue`) helps maintain organization and clarity.
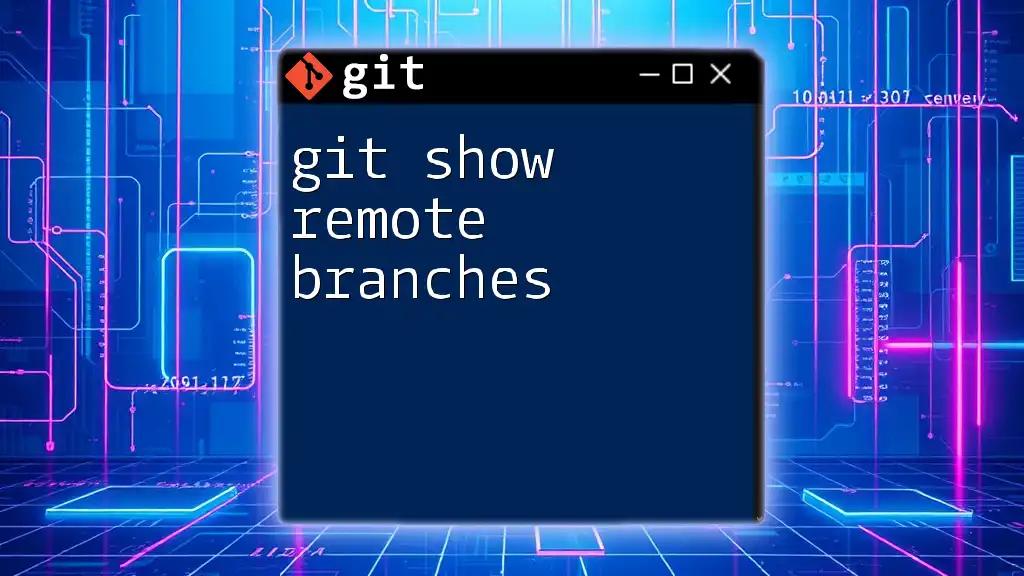
Best Practices for Working with Remote Branches
Regularly Syncing Local and Remote
To prevent merge conflicts and ensure your local work is based on the latest changes, regularly synchronize your work with the remote repository. Use:
git pull
This command brings in any changes from the remote branch into your local branch. However, ensure that you have committed or stashed your changes before pulling to avoid conflicts.
Documenting Branch Purpose
To foster clear communication within your development team, adopt a practice of documenting the purpose of each branch. Use informative commit messages and descriptive branch names, making it easier for your teammates to understand the goals associated with each branch.
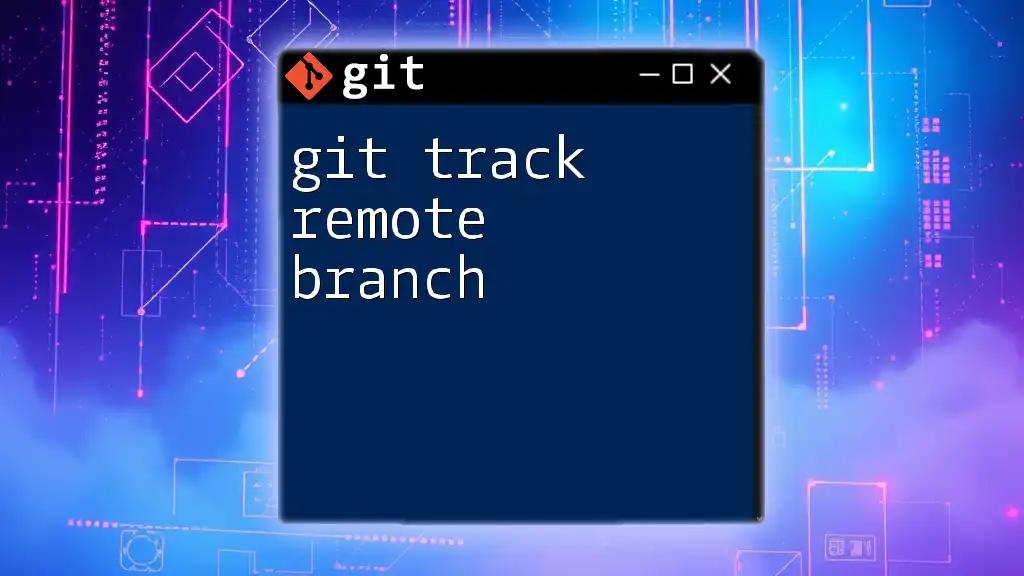
Conclusion
In this guide, you have learned how to effectively add a remote branch using Git. By understanding the different commands and their purposes, you can facilitate collaboration within your team and maintain a structured codebase. Remember to experiment with these commands as you enhance your Git skills!
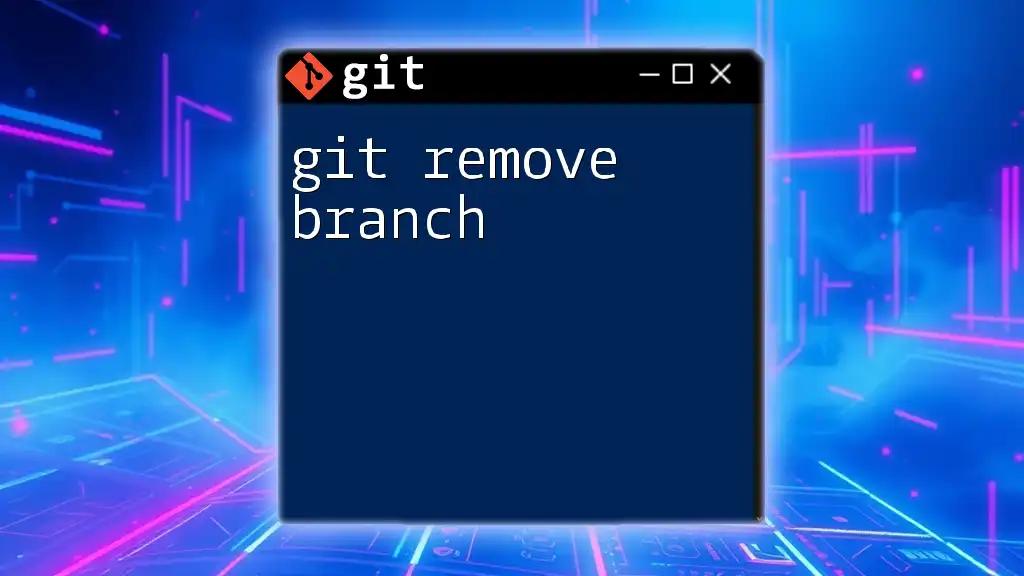
Additional Resources
For further learning, consider exploring the official Git documentation, which provides extensive information on commands and best practices. Engaging with community tutorials and asking questions will help deepen your understanding of Git and remote branches. Don't hesitate to reach out if you have any feedback or queries!