To fetch and list all remote branches in your Git repository, use the following command:
git fetch --all && git branch -r
Understanding Remote Branches
What Are Remote Branches?
In Git, remote branches are branches that exist on a remote repository, often shared among multiple developers. They are different from local branches, which you create and manipulate on your own machine. Remote branches track the state of your repository on a remote server, allowing you to see what others are working on and collaborate effectively.
Why Retrieve Remote Branches?
Retrieving remote branches is crucial for various reasons. For instance, if you're working in a team setting, you might want to review your collaborators' work or merge changes they’ve made into your own local branch. By retrieving remote branches, you can keep your local repository in sync with ongoing development, ensuring that you're working on the most current version of the project.
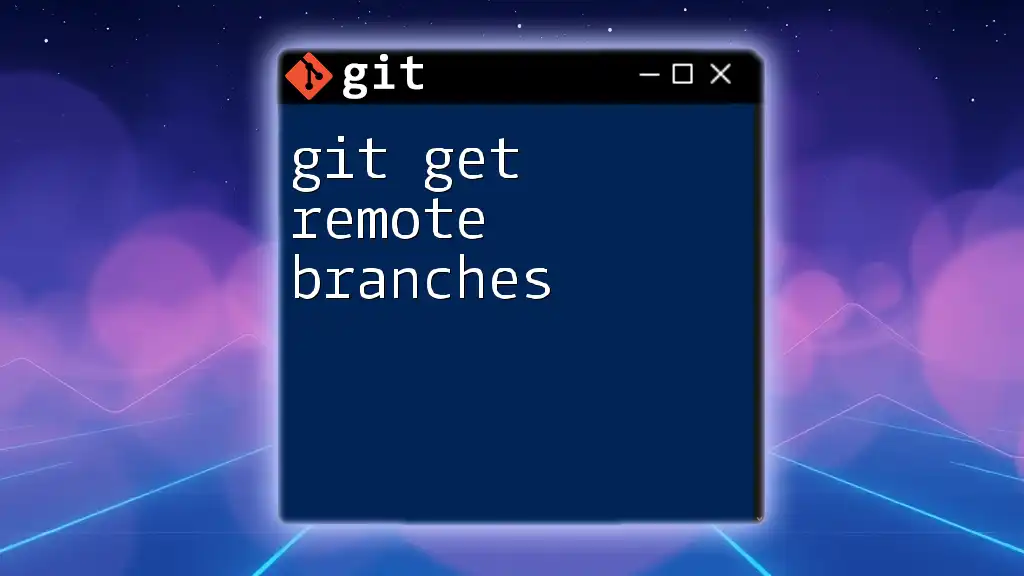
Setting Up Your Local Repository
Cloning a Repository
To get started with any Git project, you typically need to clone the repository. This action not only downloads all the branches but also checks out the default branch—generally `main` or `master`.
To clone a repository, use the following command:
git clone <repository-url>
This command creates a local copy of the remote repository, including all its branches.
Fetching Updates from Remote
Before you can view the remote branches effectively, it’s important to ensure that your local copy is up to date with the remote repository. This is done using the `git fetch` command.
Running this command will update your remote-tracking branches but will not affect your working directory or local branches:
git fetch
By executing `git fetch`, you pull in the latest changes, making it possible to see what branches are available remotely.
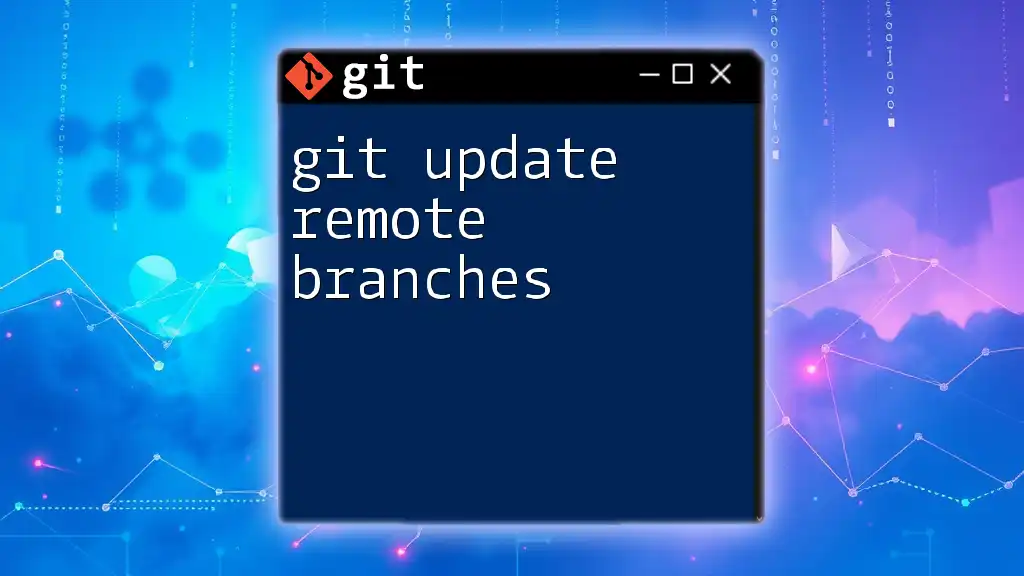
Getting All Remote Branches
Using Git Commands to View Remote Branches
The `git branch` Command
One of the simplest ways to see all the remote branches is by using the `git branch` command with the `-r` option, which lists only remote branches. Simply run:
git branch -r
This command will output a list of all the remote branches. The notation used often appears as `origin/branch-name`, where `origin` is the default name for your remote repository.
The `git ls-remote` Command
Another effective way to view all remote branches is with the `git ls-remote` command, which allows you to display references in a remote repository. To retrieve all the branches, you can use:
git ls-remote --heads <repository-url>
This command will list all remote branches and their corresponding commit IDs. It's handy for seeing the exact state of the branches without needing to clone the repository.
Additional Command Options
Yet another option to gather information about remote branches is:
git remote show origin
This command provides a summary of the remote repository, including a list of all remote branches, their status, and which tracking branches are configured on your local repository. This is an extremely useful command to get a comprehensive overview of the remote repository's structure.
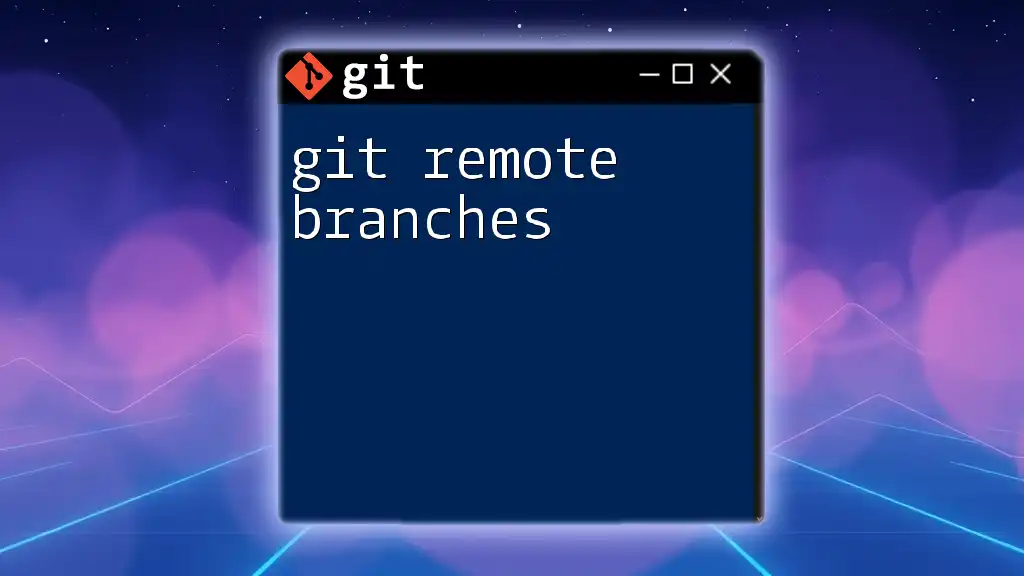
Working with Remote Branches
Checking Out Remote Branches
Once you've identified the remote branches you want to work with, you can check out any of them. To do so, simply run:
git checkout <remote-branch-name>
This command switches your working directory to the specified remote branch. It's essential to note that if you don’t have a local copy of the branch, this command will create a local branch that tracks the remote one.
Creating a Local Tracking Branch
If you intend to make changes to a remote branch, you should create a local tracking branch. This allows you to work on the branch locally while keeping it synchronized with the remote branch. You can set this up using:
git checkout -b <local-branch-name> <remote-branch-name>
This command creates a new local branch that tracks the specified remote branch. Tracking branches make it easier to push and pull changes back and forth with the remote repository.
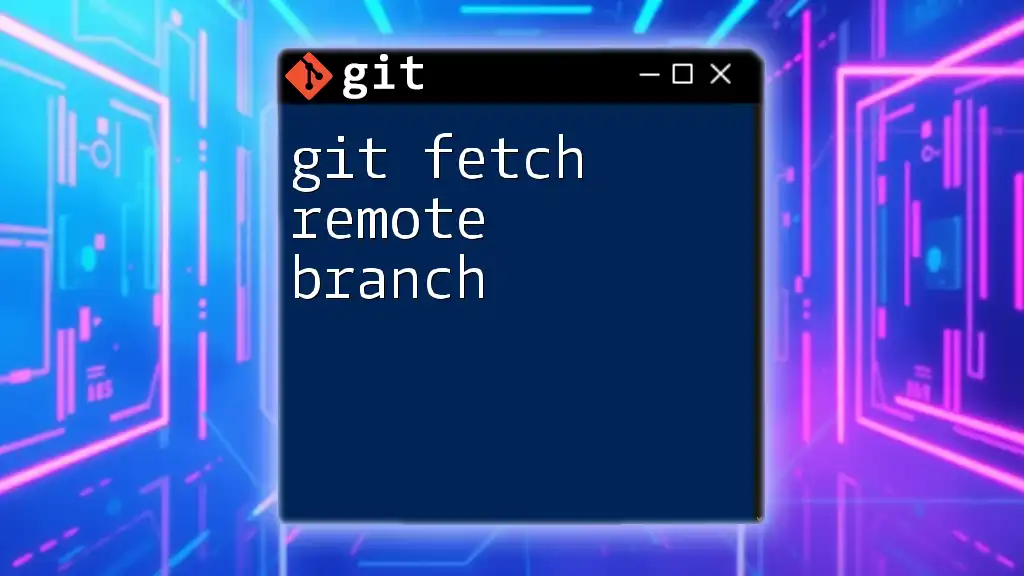
Common Issues and Troubleshooting
Remote Branch Not Found
Sometimes, you might encounter an error stating that a remote branch cannot be found. This usually occurs if the branch has been renamed, deleted, or if there has been an issue with the repository URL. Double-check the remote URL and ensure that the branch exists on the remote repository.
Catching Up with Remote Branches
To keep your local branch updated with changes made on the remote branch, it's crucial to perform a pull operation regularly. This can be done with:
git pull origin <remote-branch-name>
This command fetches the latest changes from the specified remote branch and merges them into your local branch. Be cautious of potential merge conflicts that might need resolving manually.
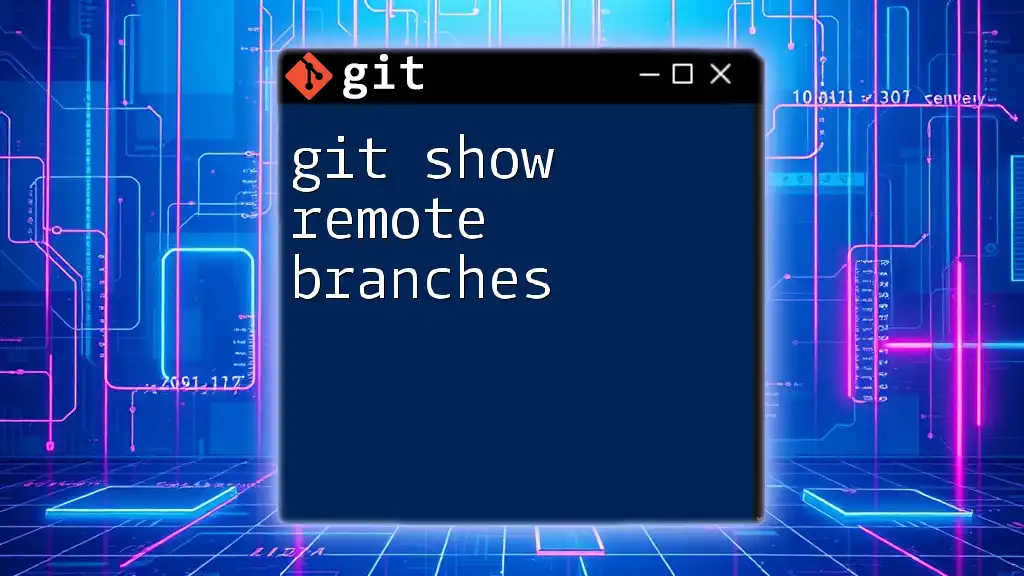
Best Practices for Managing Remote Branches
Regularly Fetching Changes
Consistency is key in collaborative environments. Make it a habit to use `git fetch` to bring your local repository up to date with the remote. This practice ensures that you are always aware of the most recent changes made by your teammates.
Naming Conventions for Branches
Adopting a naming convention for your branches can significantly enhance project organization and clarity. Informative names, such as `feature/add-login` or `bugfix/fix-login-issue`, make it easier to understand the purpose of each branch at a glance.
Clean Up Unused Remote Branches
Over time, remote branches may become outdated or irrelevant. It’s important to evaluate and delete them as necessary. To remove a stale remote branch, you can execute:
git push origin --delete <remote-branch-name>
Maintaining a clean repository prevents confusion and ensures that the current team members focus on active development.
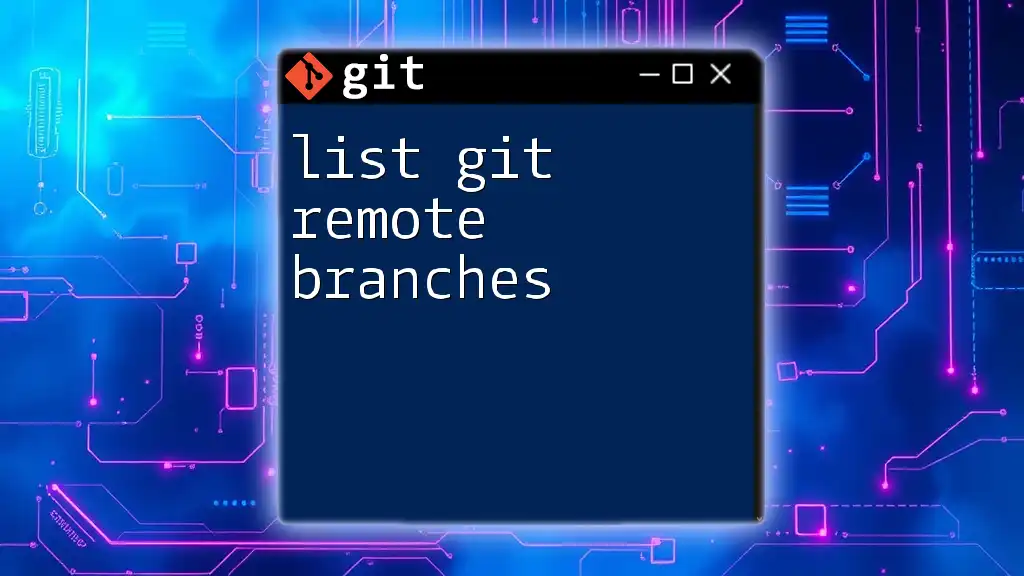
Conclusion
Retrieving and managing remote branches is an essential skill for any developer using Git, especially in a collaborative environment. By learning how to effectively use commands like `git fetch`, `git branch -r`, and `git checkout`, you will position yourself to work more efficiently and productively in team-based projects. Make sure to practice these concepts regularly, and feel free to explore more advanced features of Git as you become more comfortable with the tool.
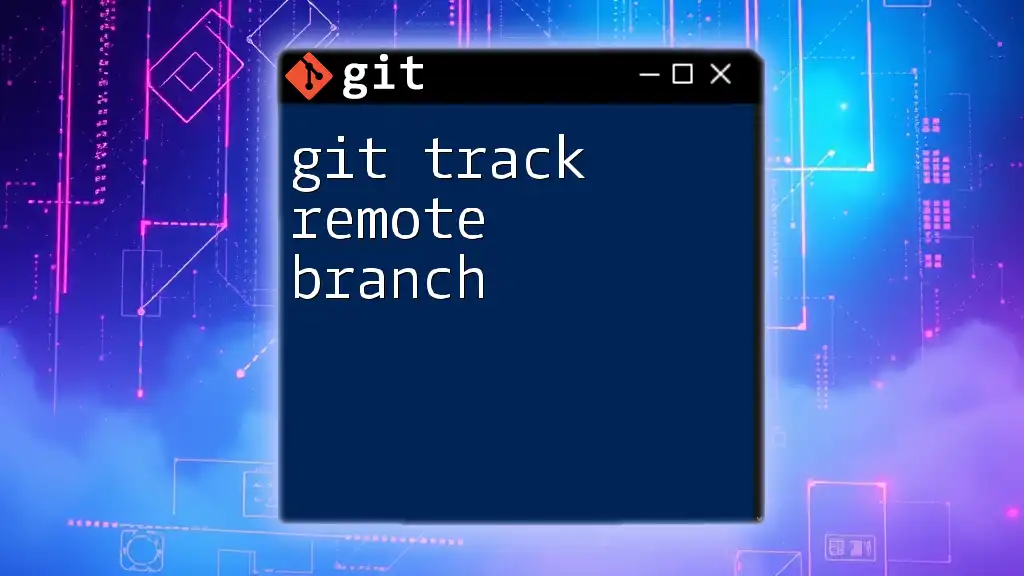
Additional Resources
For further exploration, refer to the official Git documentation to deepen your understanding of command syntax and advanced features. Consider subscribing to resources or platforms that share tips and tutorials to keep enhancing your Git skills. Happy coding!