To get the current branch name in a Git repository, you can use the following command:
git branch --show-current
Understanding Git Branch Structure
What is a Git Branch?
In Git, a branch is a lightweight movable pointer to a commit. Branches are fundamental to the way Git enables efficient collaboration among teams. They allow multiple developers to work on different features or bug fixes simultaneously without interfering with each other's work. When changes are complete on a branch, they can be merged back into the main project, maintaining a clean history and making it easy to manage different aspects of development.
The Concept of the Current Branch
The current branch in Git refers to the branch you’re actively working on. Understanding which branch you’re currently on is crucial because any commits or changes you make will affect that branch. Not knowing your current branch can lead to issues like unintentional commits being made to the wrong branch, which can complicate collaboration and project management.
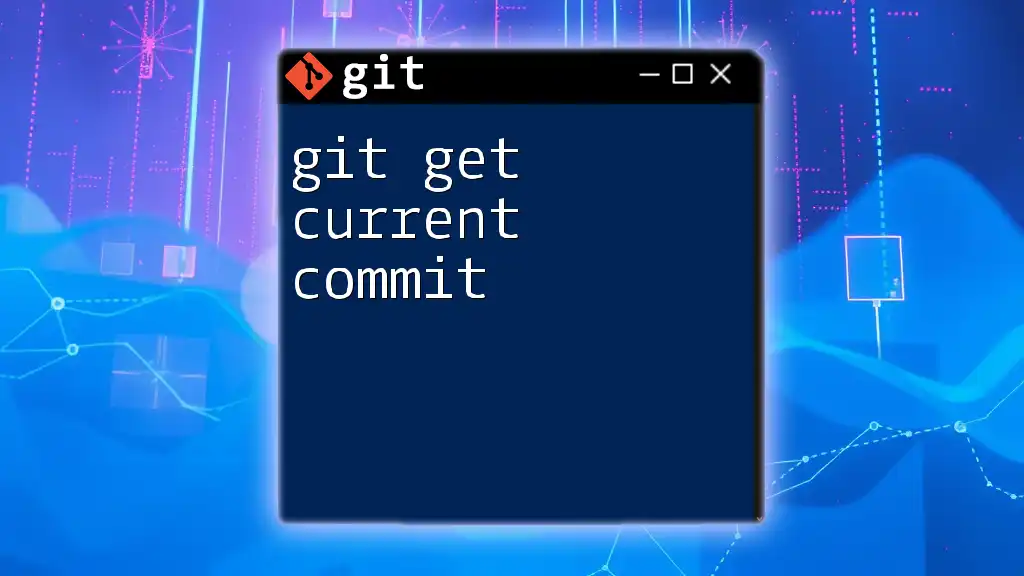
Checking the Current Branch in Git
Using the `git branch` Command
One of the simplest ways to check your current branch is by using the `git branch` command. When executed, this command will list all branches in your repository:
git branch
In the output, the current branch will be denoted with an asterisk (*). For example:
feature-branch
* main
bugfix-branch
This indicates that you are currently on the main branch.
Using the `git rev-parse` Command
For a more concise output, the command `git rev-parse --abbrev-ref HEAD` is a great choice. This command solely returns the name of the current branch, making it very straightforward and useful for scripting.
git rev-parse --abbrev-ref HEAD
Running this command will simply return:
main
This output indicates that you are on the `main` branch, without any additional information from other branches.
Using the `git status` Command
The `git status` command gives a more comprehensive overview of the state of your repository but also includes your current branch information. Running it provides details about staged, unstaged, and untracked files:
git status
In the output, you will see a line similar to this:
On branch main
Your branch is up to date with 'origin/main'.
This output affirms your current branch as well as its status relative to the remote repository.
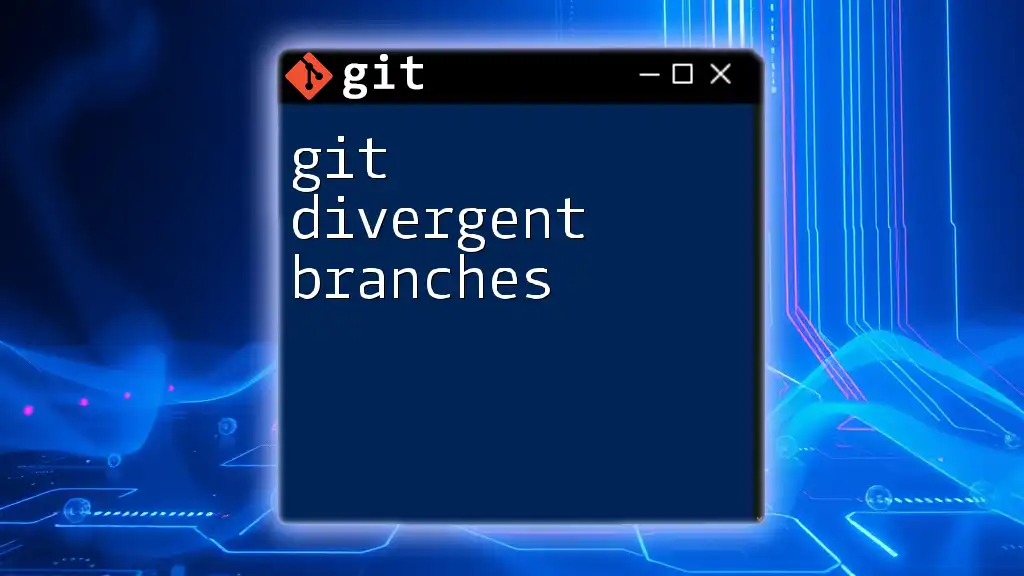
Advanced Ways to Get Current Branch Information
Using Shell Command Substitution
You can further enhance your command line experience by combining commands to store the current branch name in a variable using shell command substitution. This method can be particularly useful in automation scripts.
CURRENT_BRANCH=$(git rev-parse --abbrev-ref HEAD)
echo "You are currently on branch: $CURRENT_BRANCH"
With this snippet, not only do you retrieve the current branch, but you also format your output for clarity.
Scripting for Automation
If you routinely need to check the current branch and perform related actions, creating a simple script might save you time. Here’s an example:
#!/bin/bash
CURRENT_BRANCH=$(git rev-parse --abbrev-ref HEAD)
echo "Current branch is: $CURRENT_BRANCH"
Save this script as `check_branch.sh`, give it executable permission, and run it whenever you need to verify your current branch quickly.
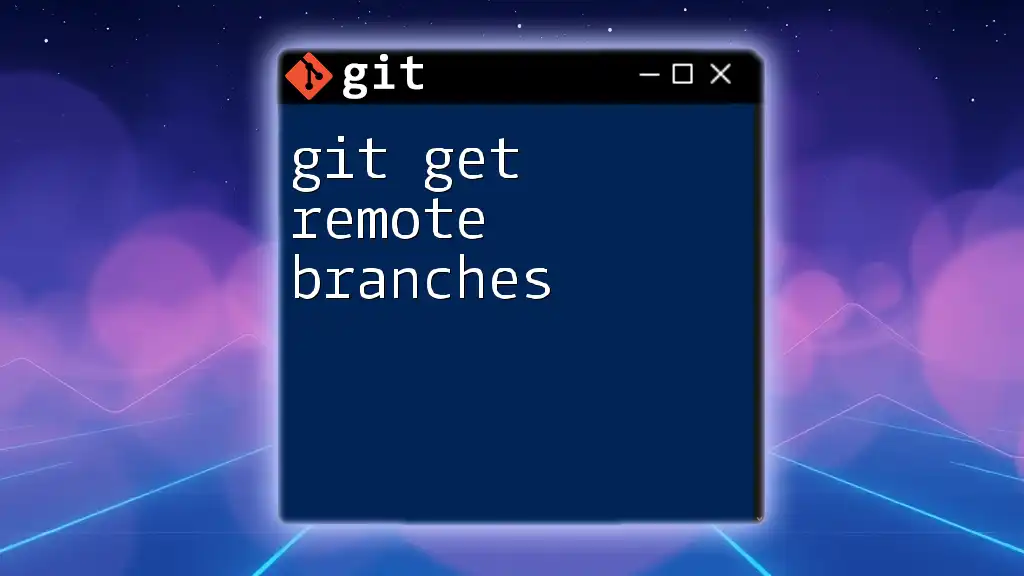
Common Mistakes and Troubleshooting
Issues with Detached HEAD State
A common pitfall for Git users is entering a detached HEAD state, which occurs when Git is not currently on any branch. This usually happens when you checkout a specific commit rather than a branch. In this state, the current branch reference is lost, making it difficult to figure out where you are.
To check the current branch in this state, use:
git branch
Since no branch is checked out, it will return no branch lists. To return to a regular branch, use:
git checkout main
This command switches back to the `main` branch, reestablishing a standard working context.
Misunderstandings with Local vs Remote Branches
It’s also essential to distinguish between local and remote branches. Knowing which branch you are on locally does not always give the full picture regarding the remote state. It’s a good practice to check both using:
git branch -a
This command will list all branches, both local and remote, giving you a clearer view of your repository's structure. To ensure you're aware of the context of the branch you are working on, synchronize it with the remote state regularly.
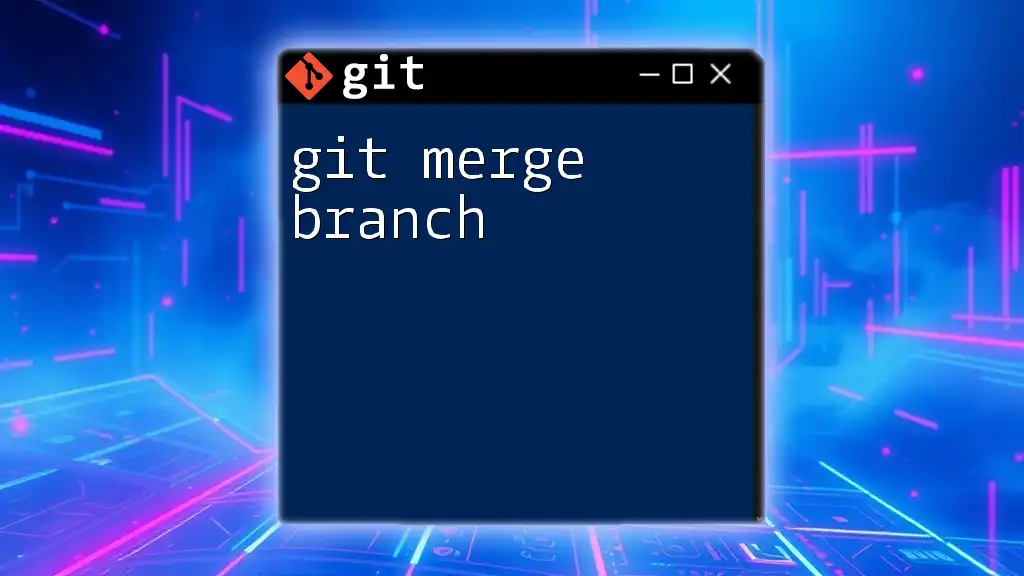
Conclusion
Being aware of your current branch in Git is a vital skill that enhances your workflow and prevents potential mishaps. Whether you check your branch with `git branch`, `git rev-parse`, or `git status`, having this information at your fingertips allows for a more seamless development experience. Practicing these commands and understanding their output will improve your efficiency and effectiveness in using Git.
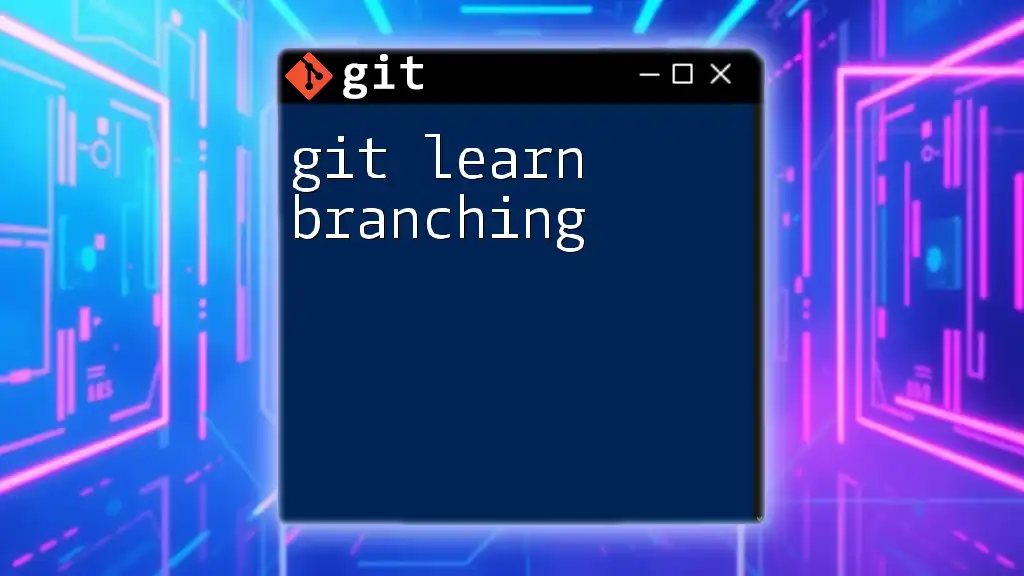
Additional Resources
For further reading and to expand your Git knowledge, consider visiting the official Git documentation or exploring tutorials specifically focused on branching and Git commands.
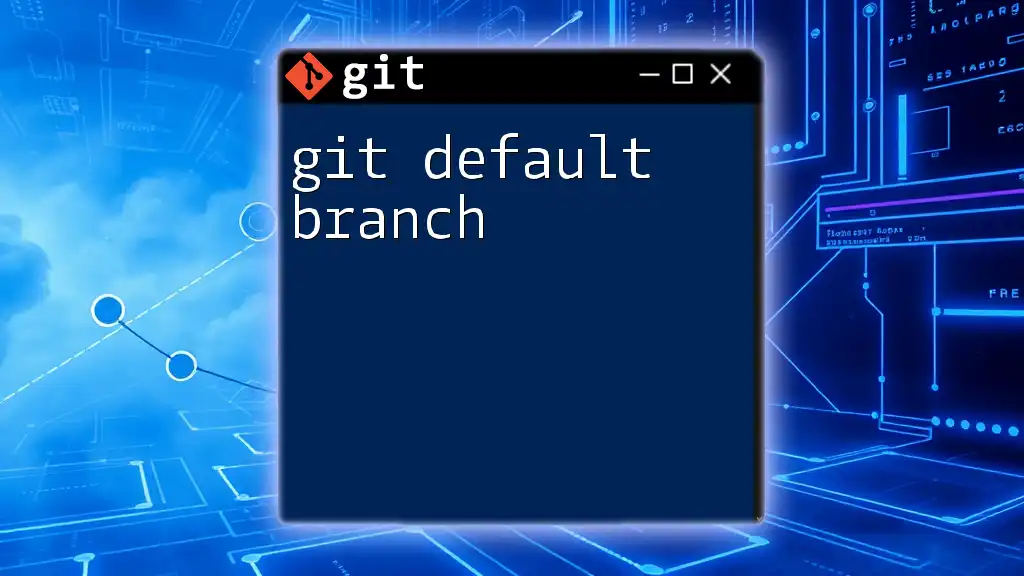
Call to Action
Stay updated and enhance your skills with concise Git command tips. Subscribe to our newsletter for more learning insights and practical advice!