To remove a branch in Git, you can use the command `git branch -d branch_name` for a safe deletion of a local branch or `git branch -D branch_name` to forcefully delete it, even if it hasn't been merged.
git branch -d branch_name
or, for a forceful delete:
git branch -D branch_name
Understanding Git Branches
What is a Git Branch?
A branch in Git represents an independent line of development. It allows developers to work on different features or fixes without affecting the main project's codebase. The primary benefit of using branches is that they enable experimentation and parallel development. This means you can create, test, and merge features in isolation, reducing the risk of introducing bugs to the production environment.
Why Remove a Branch?
Removing branches that are no longer needed is essential for several reasons:
- Clutter Reduction: Keeping your repository clean helps improve navigation and usability. As branches pile up, it can become challenging to find the active ones.
- Outdated Features: Sometimes, features become irrelevant due to changing requirements or project direction. Deleting these branches prevents confusion later on.
- Completed Tasks: Once a feature branch has been merged back into the main branch, it’s considered complete. Removing it helps maintain clarity in your project’s history.
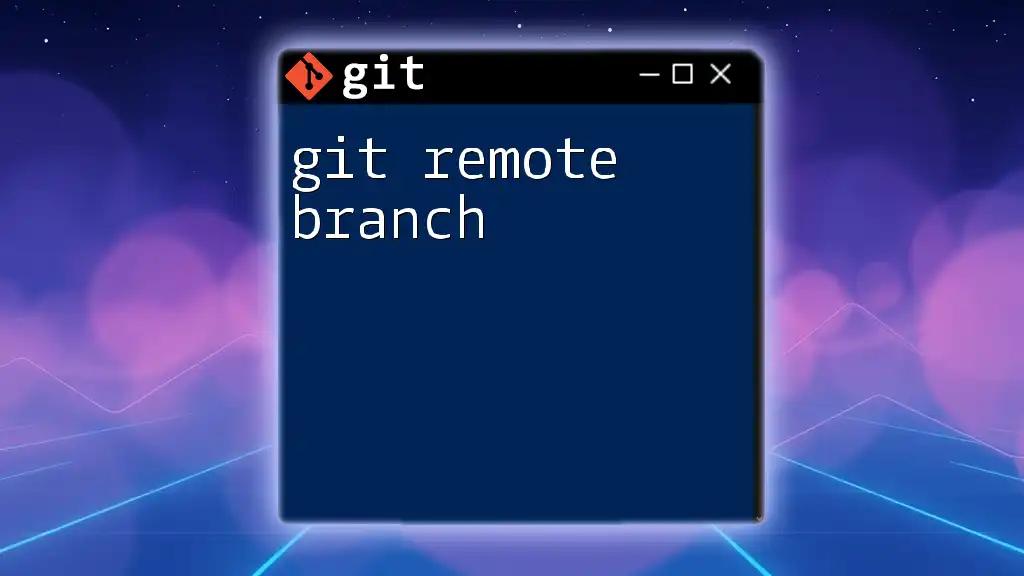
The Basics: `git branch -d` and `git branch -D`
The Command: `git branch -d`
The `git branch -d` command is your go-to for safely deleting branches. This command checks if the branch you want to delete has been merged into the current branch. If it hasn't, Git will stop you with a warning, helping avoid accidental loss of unmerged work.
Syntax:
git branch -d <branch_name>
Example
git branch -d feature-xyz
Here, the command attempts to delete the branch named `feature-xyz`. If `feature-xyz` has not been merged into the current branch, Git will return an error message, informing you that the branch is not fully merged.
The Command: `git branch -D`
For situations where you are certain you want to delete a branch, even if it hasn’t been merged, you can use `git branch -D`. This is a more forceful command that bypasses the safety check.
Syntax:
git branch -D <branch_name>
Example
git branch -D feature-xyz
In this instance, `feature-xyz` will be deleted regardless of its merge status. Exercise caution using this command since it will permanently remove the branch from your local repository.
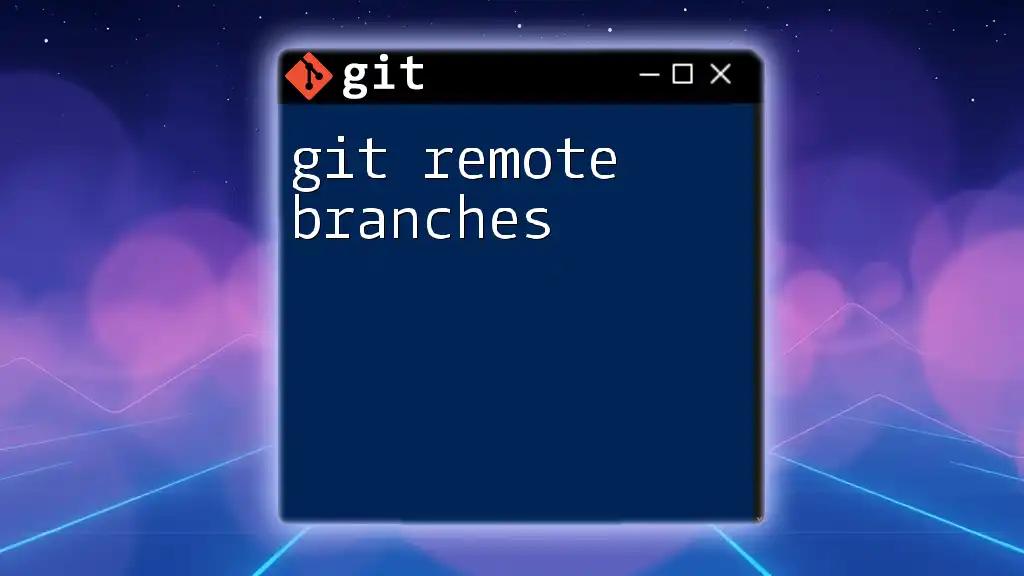
Removing Remote Branches
The Command: `git push --delete`
In addition to managing local branches, you may find it necessary to remove branches from a remote repository. The `git push --delete` command provides a straightforward way to accomplish this.
Syntax:
git push <remote_name> --delete <branch_name>
Example
git push origin --delete feature-xyz
This command deletes the `feature-xyz` branch from the `origin` remote repository. It helps remove branches that are no longer relevant for all team members accessing the remote.
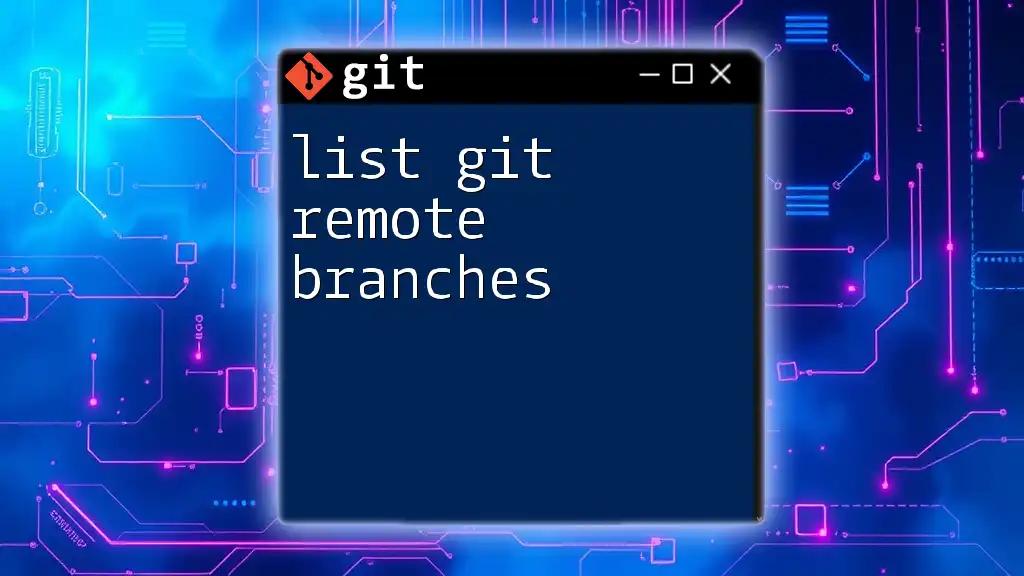
Best Practices for Removing Branches
Keep Your Repository Clean
Regular maintenance and cleanup of your branches can lead to a more organized and efficient workflow. Make it a habit to routinely check your branches and remove any that are stale or no longer in use. This will make the entire version control experience smoother and easier for your team.
Communicate with Team
Establish a protocol for branch removal before deleting any branches that involve team collaboration. This ensures everyone is on the same page, preventing the accidental deletion of branches that someone else may still be working with. Clear communication minimizes disruption in collaborative projects.
Document Merges and Deletions
Keeping track of your branch history, including merges and deletions, is vital. Use descriptive commit messages that explain why a branch was deleted, which will provide context for future reference. Documentation is key to maintaining a clear understanding of your project’s evolution over time.
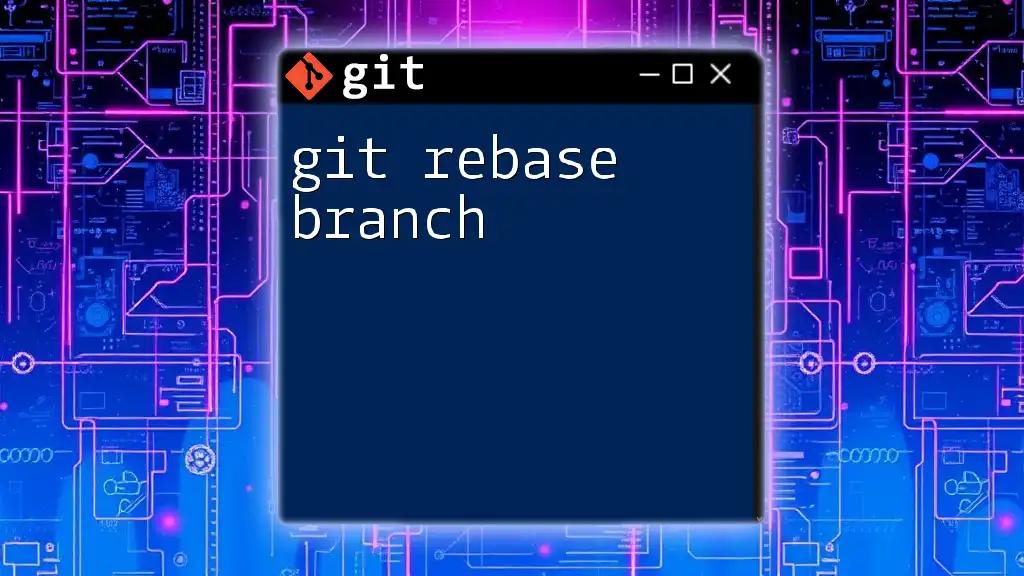
Conclusion
Efficiently managing branches is a critical aspect of using Git. Learning how to effectively `git remove branch` commands can significantly enhance your workflow by keeping the repository organized and relevant. Practice using these commands to understand their functionalities and be prepared for real-life application. For more concise Git tutorials and tips, be sure to follow along with our content as you continue to become proficient in using Git.
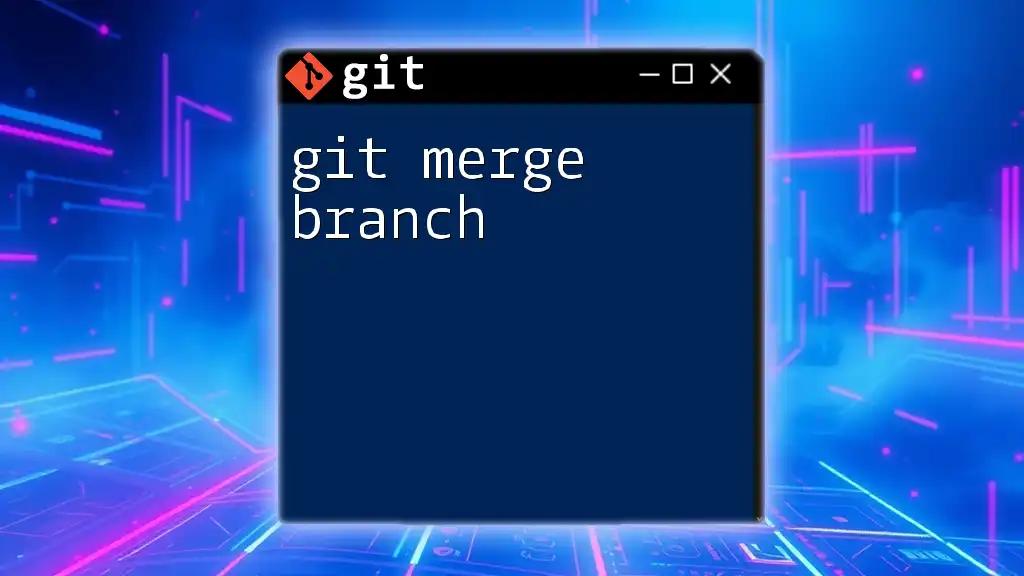
Additional Resources
For those looking to deepen their understanding of Git, consider exploring the official Git documentation or engaging with video tutorials that visually explain the concepts discussed in this article. Keeping up with learning resources will ensure that you stay at the forefront of Git development skills.