The `git clone` command is used to create a copy of a specific repository or branch from a remote server to your local machine, allowing you to work on the project without affecting the original source.
Here's how you can clone a specific branch:
git clone -b <branch-name> <repository-url>
Replace `<branch-name>` with the name of the branch you want to clone and `<repository-url>` with the URL of the repository.
What is a Branch in Git?
Branches in Git serve as a powerful tool for managing different versions of your project. Each branch represents an independent line of development where you can make changes or add features without impacting the rest of the project. This allows for experimentation and collaboration among multiple developers.
Common Branching Strategies
Different strategies can be used to manage branches effectively, including:
-
Feature Branching: This strategy involves creating a branch for each new feature. Once the feature is complete, the branch is typically merged back into the main branch (often `main` or `master`).
-
Release Branching: In this approach, a new branch is created specifically for preparing a new release. This allows for final testing and fixes while work continues on other features in the main development branch.
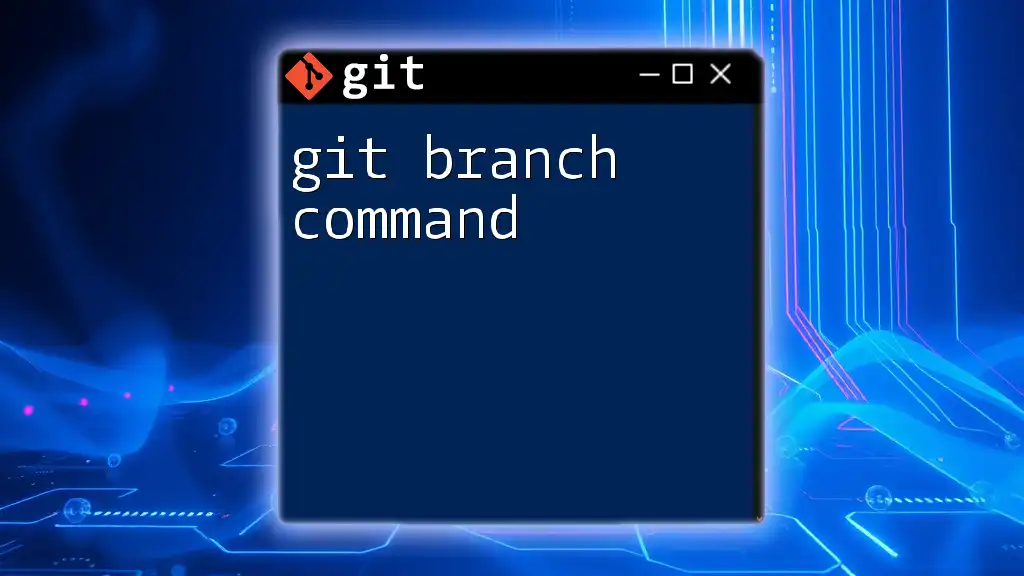
The Git Clone Command
The `git clone` command is fundamental for downloading a copy of a repository from a remote source. The basic syntax for the command is:
git clone [repository URL]
When you execute this command, you create a complete local copy of the repository, including all its files, history, and branches.
Cloning a Repository
To clone a repository, you'll need to have the repository's URL, which can typically be found on the hosting service (like GitHub, GitLab, or Bitbucket).
Example Code:
git clone https://github.com/user/repository.git
This command pulls a complete copy of the repository into your local directory, allowing you to start working immediately.
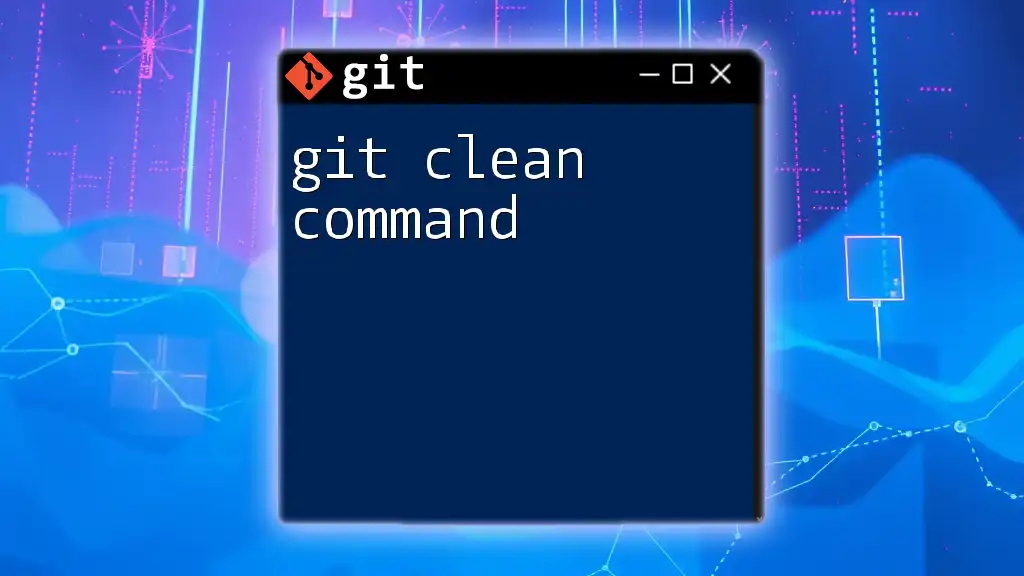
Cloning a Specific Branch
Sometimes you may only be interested in a specific branch, especially if you want to work on a certain feature without downloading the entire repository. This is where the `-b` option comes in handy.
Why Clone Only a Branch?
Cloning only a specific branch can save time and storage, as you're not pulling an entire repository with multiple branches that you may not need. For instance, if you're tasked with enhancing a feature that exists only on `feature-branch`, cloning just that branch focuses your resources.
Using the `-b` Option
The `-b` flag allows you to specify which branch you want to clone directly during the cloning process.
Syntax for Cloning a Specific Branch
To clone a specific branch, the command format is:
git clone -b [branch-name] [repository URL]
Example of Cloning a Specific Branch
If you want to clone a branch named `feature-branch`, you would execute:
git clone -b feature-branch https://github.com/user/repository.git
This command will create a local copy of the repository but will check out `feature-branch` right away.
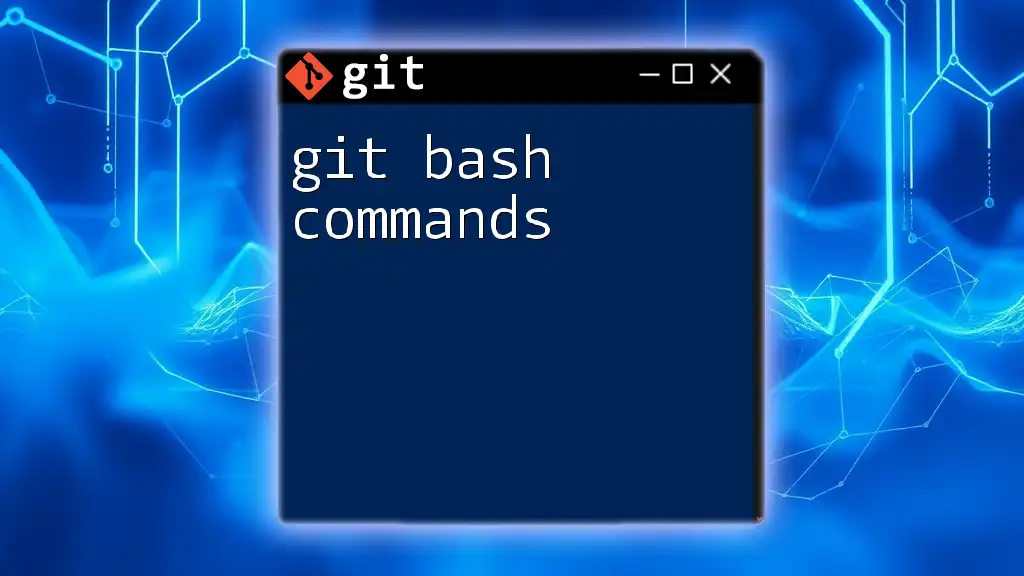
Verifying the Cloned Branch
Navigating into the Cloned Repository
After cloning, you'll want to navigate into your working directory. This can be done using the `cd` command:
cd repository
Checking the Active Branch
To confirm that you've successfully cloned the intended branch, you can use:
git branch
This will display the currently active branch, allowing you to ensure you're exactly where you need to be before making any changes.
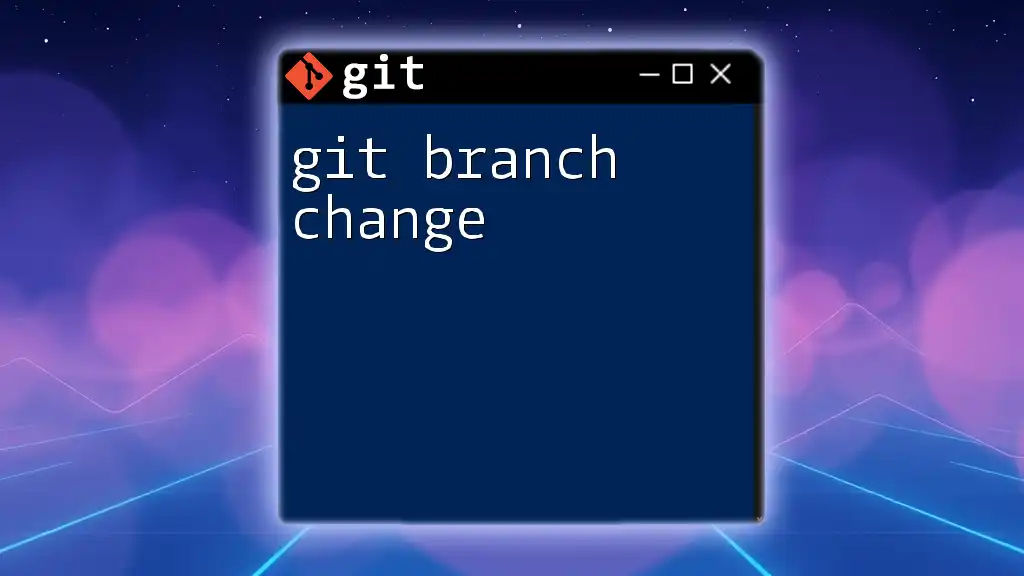
Understanding the Clone Operation
What Gets Cloned?
When executing the `git clone` command, you're not just downloading files; you're also pulling in the complete history of the repository. This includes all commits and branches, making it easy to navigate through past changes.
Remote Tracking Branches
Cloning a repository creates remote-tracking branches that keep track of branches in the remote repository. For example, if you clone a repository with two branches, `main` and `feature-branch`, you will have local references (e.g., `origin/main` and `origin/feature-branch`) that correspond to those branches.
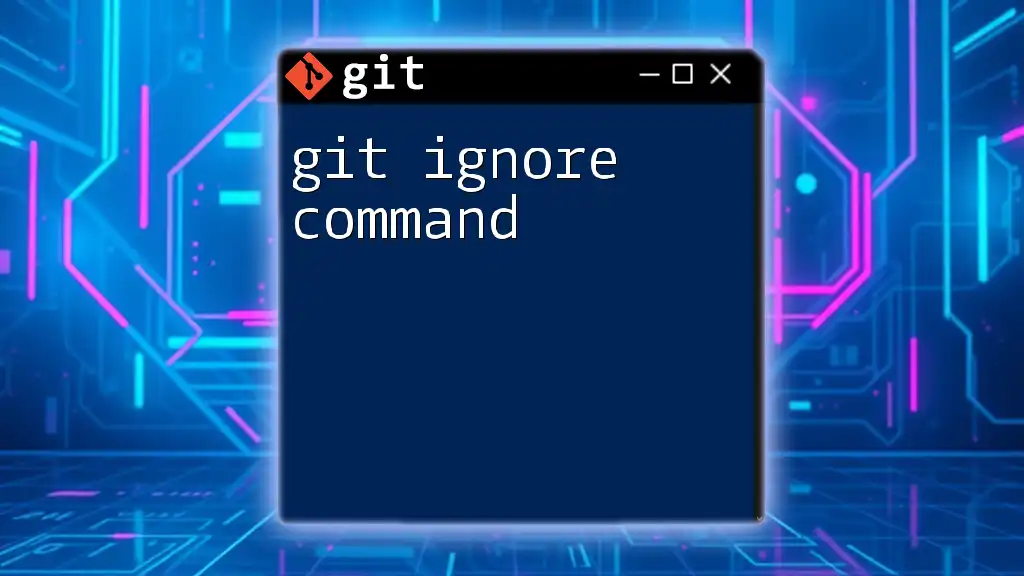
Troubleshooting Common Issues
Common Errors When Cloning
You may encounter common error messages when cloning a repository. The most frequent of these is "repository not found." This often occurs due to an incorrect URL or network issue.
Using SSH vs HTTPS for Cloning
You can choose between two protocols for cloning: SSH and HTTPS. While HTTPS is more straightforward as it doesn’t require any setup, SSH is beneficial for authenticated access and allows for secure connections, especially if you’ll be pushing changes back to the repository frequently.
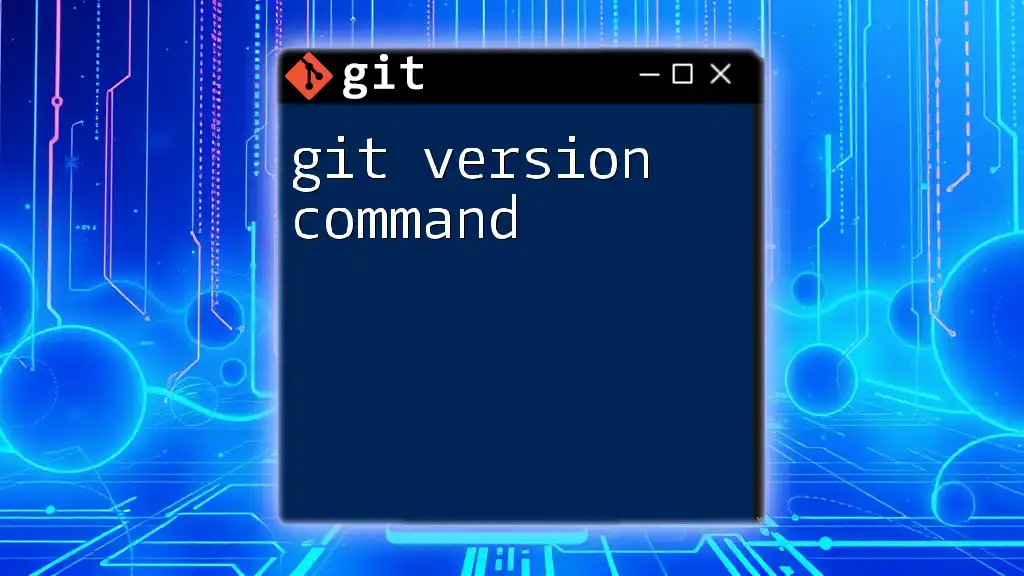
Best Practices for Cloning
Minimizing Repository Size
When working with large or complex repositories, be mindful of cloning only the branches you need. Consider alternatives like `git sparse-checkout` for cases where you only require specific directories within a repository.
Keeping Your Branches Clean
It's essential to keep your local repository organized. Regularly prune merged or outdated branches and consider using a clear naming convention for your feature branches. A tidy workspace can significantly improve productivity and ease collaboration.
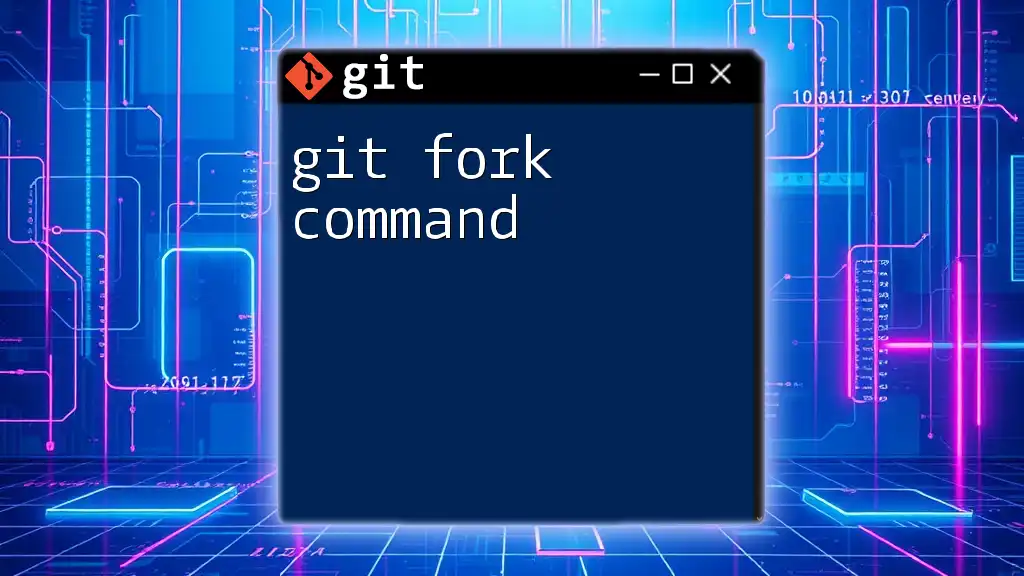
Conclusion
The git clone branch command is a powerful feature that enables you to work efficiently within Git repositories. By following the guidelines outlined in this article, you'll be able to clone specific branches effectively, troubleshoot common issues, and implement best practices to streamline your development process.
Encouragement to Practice
To solidify your understanding, practice cloning repositories and branches on your own. Experiment with different configurations and strategies to find what works best for your projects.
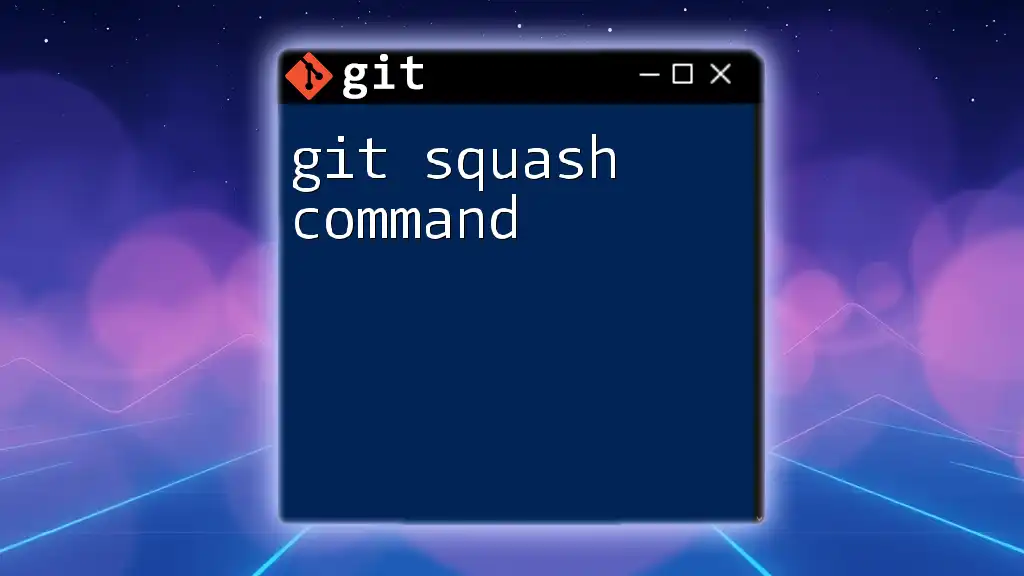
Additional Resources
For further learning, check out the official Git documentation and relevant articles on branching strategies. Engaging with these resources will improve your command of Git and refine your development workflow.
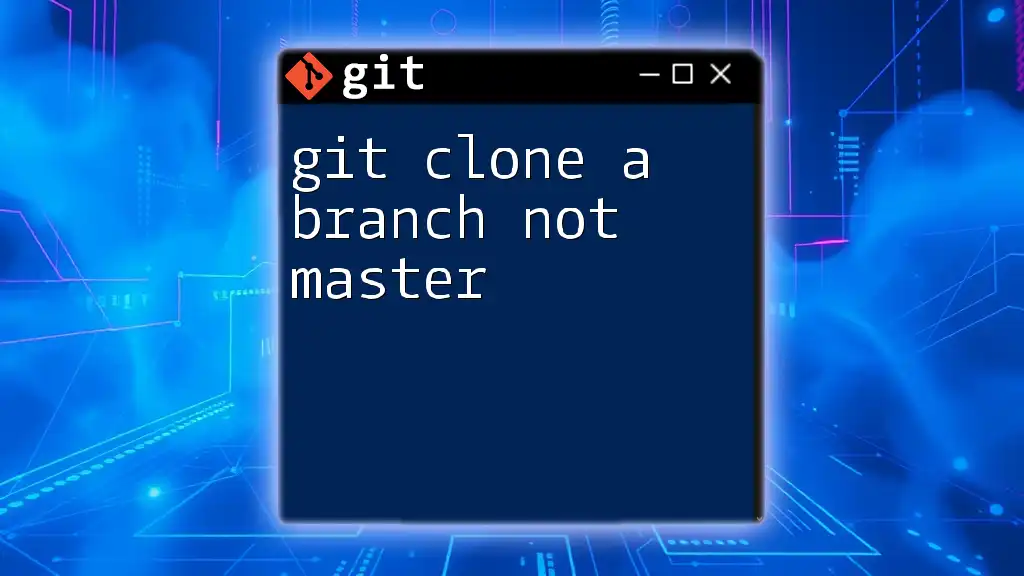
Call to Action
Stay updated with practical tips on Git commands by subscribing to our newsletter or following us on social media. Sharpen your skills and make the most out of your version control experience!