To copy a branch in Git, you can use the following command to create a new branch based on an existing one:
git checkout -b new-branch-name existing-branch-name
Understanding Git Branches
What is a Branch?
A branch in Git is essentially a parallel version of your project. It's a lightweight movable pointer to a commit in your project history. Branches allow developers to work on different features or bug fixes independently without interfering with each other’s work. This capability is fundamental to collaborative programming and code maintenance.
Why Copy a Branch?
Copying a branch can serve multiple purposes. It is particularly useful in scenarios where:
- You wish to create a new feature based on an existing feature's work.
- You want to start a new line of development without losing the previous branch's context.
- You’re maintaining an experimental branch while still keeping your main branch stable.
Benefits include simplifying collaboration, improving organization, and preserving your workflow's integrity.
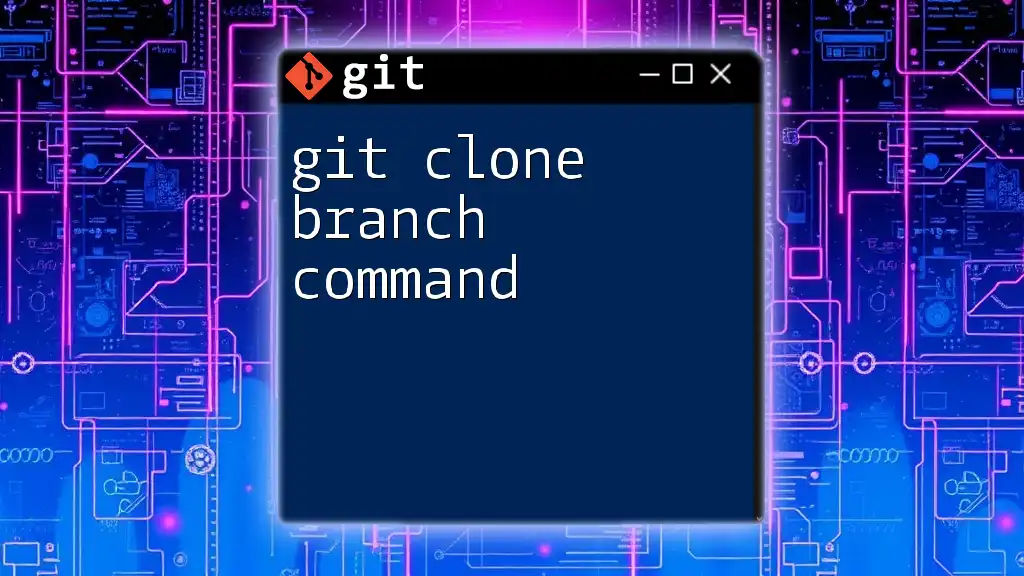
Methods for Copying a Branch
Creating a New Branch from an Existing One
To create a new branch from an existing one, you can use the `git branch` command. This command enables you to designate a new branch based on where the existing branch is currently pointing.
Code Snippet:
git branch new-branch-name existing-branch-name
In this command, replace `new-branch-name` with your desired name for the new branch and `existing-branch-name` with the branch from which you want to copy.
Example Scenario: Imagine you have a feature branch called `feature/login` and you want to create a new branch for `feature/login-test`. Using the command mentioned above, you can accomplish this, allowing you to handle tests independently while preserving the original feature branch.
Checking Out and Creating a New Branch
If you wish to create a new branch and switch to it simultaneously, the `git checkout -b` command is the way to go. This command is a shortcut that simplifies both tasks in one go.
Code Snippet:
git checkout -b new-branch-name existing-branch-name
Here, `new-branch-name` is the name of your new branch, and `existing-branch-name` is the source branch.
Step-by-step Breakdown:
- `git checkout -b` initiates a new branch.
- It then automatically checks out to that new branch, placing you in a position to start making your changes right away.
Example Scenario: You could use this command if you're starting on a new feature, say `feature/logout`, while ensuring you're working off the most recent updates from `main`.
Copying the Latest Commit
Using `git cherry-pick`
If you want to incorporate specific changes from one branch to another, `git cherry-pick` is the command to use. Instead of copying the entire branch, you can selectively apply commits, which is especially beneficial when dealing with bug fixes or minor changes.
Code Snippet:
git checkout new-branch-name
git cherry-pick commit-hash
Explanation:
- First, check out your new branch.
- Then use `git cherry-pick` followed by the specific commit hash (found by using `git log`) that you want to copy over.
When to Use This Method: This is ideal if only specific changes from `existing-branch-name` are required—such as a bug fix that needs to be integrated into the `main` branch without carrying over all of the other changes.
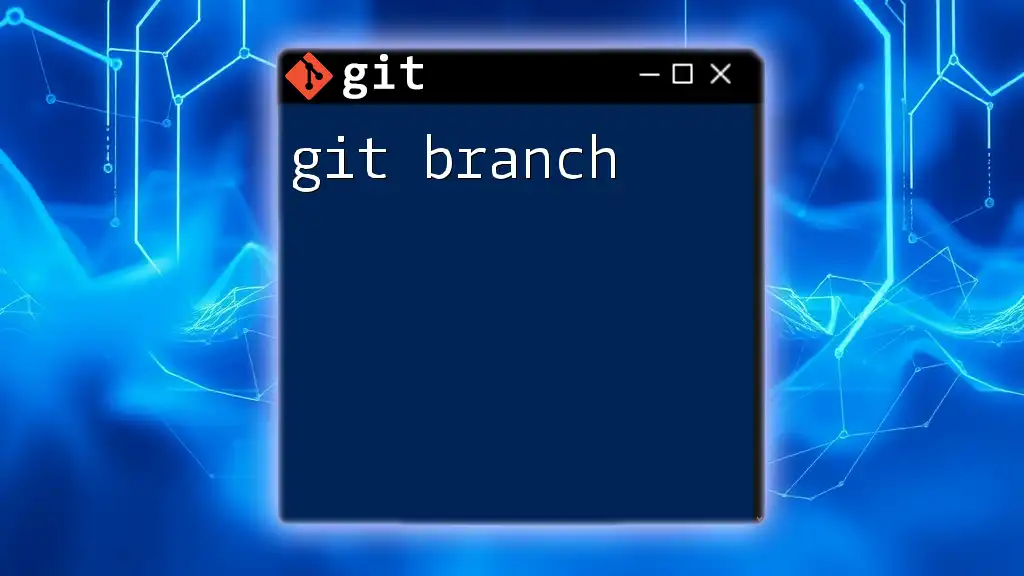
Working with Remote Branches
Copying a Remote Branch Locally
When you’re collaborating on a team, you'll often deal with remote branches. To create a local copy of a remote branch, you first need to update your local repository with changes from the remote.
Code Snippet:
git fetch origin
git checkout -b new-branch-name origin/existing-branch-name
Explanation:
- The `git fetch origin` command updates your remote-tracking branches.
- The `checkout` command then creates a new branch based on the remote branch you specified.
Importance: Being able to copy remote branches ensures that you keep your local environment in sync with your team's work, ultimately improving collaboration.
Pushing a Newly Created Branch to Remote
Once you've developed your new branch and are ready to share your progress, you’ll want to push it back to the remote repository.
Code Snippet:
git push origin new-branch-name
This command uploads your local branch to the remote repository, making it accessible for other team members.
Importance of Synchronization: This step is crucial as it keeps everyone on the same page, ensuring all changes are readily available.
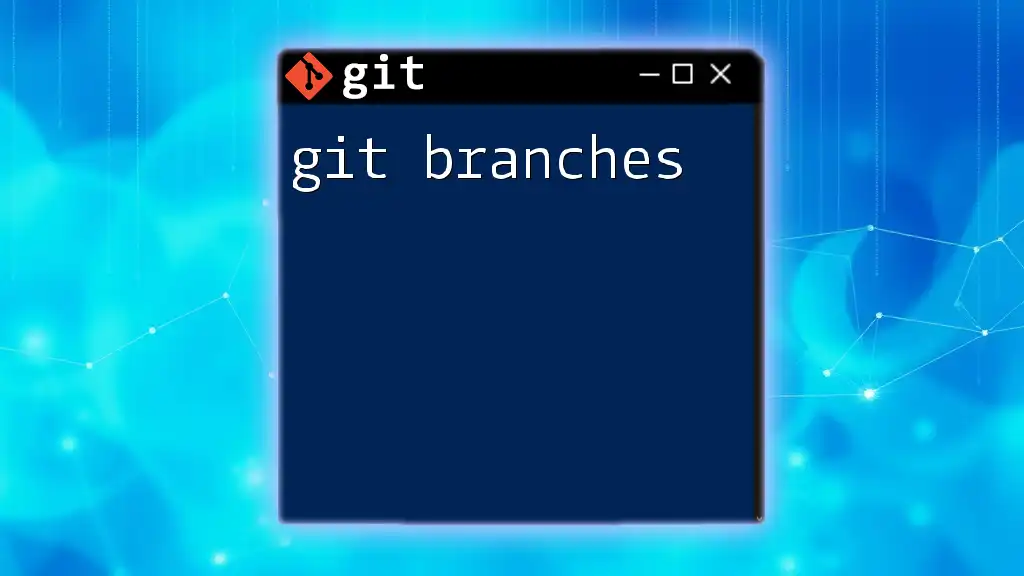
Best Practices for Branch Management
Naming Conventions
Keeping a consistent and clear naming convention for your branches will save time and confusion. Names should be descriptive enough to convey the purpose of the branch, for example, using prefixes such as `feature/`, `bugfix/`, and `hotfix/`.
Regular Merging and Clean-Up
Frequent merging of branches helps keep the integration process smooth. It’s often recommended to merge branches back to the `main` branch as soon as new features or bug fixes have been verified. Additionally, regularly deleting branches that are no longer needed keeps your workflow clean and manageable.
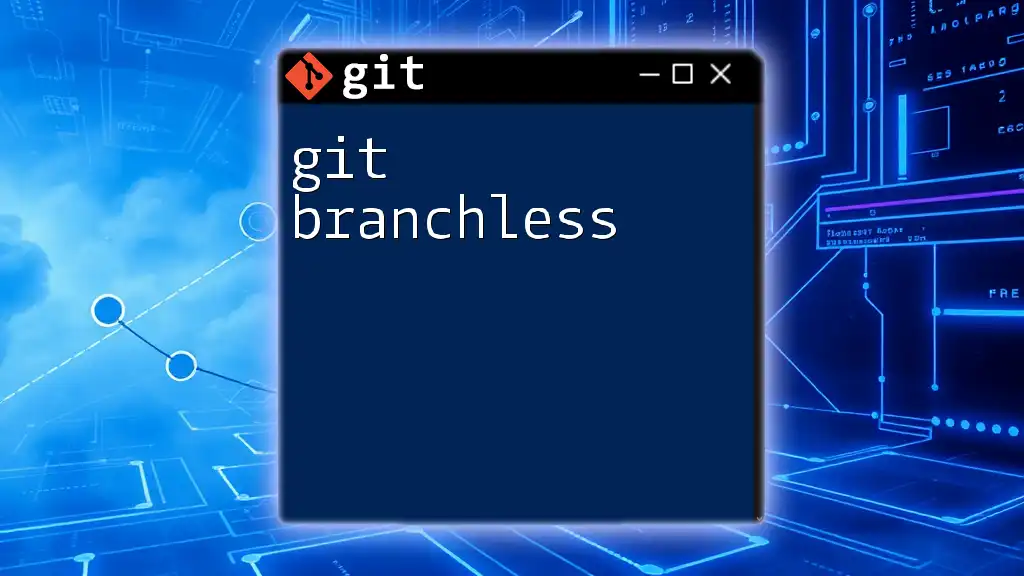
Common Mistakes to Avoid
Forgetting to Push Changes
A common pitfall for developers is neglecting to push changes after creating a new branch. This can lead to confusion when teammates are unaware of new developments. Always remember to check that your changes are reflected on your remote.
Confusing Branch Names
It’s important to avoid using vague or similar names that might confuse team members. The clearer your branch naming scheme, the easier collaboration will be.
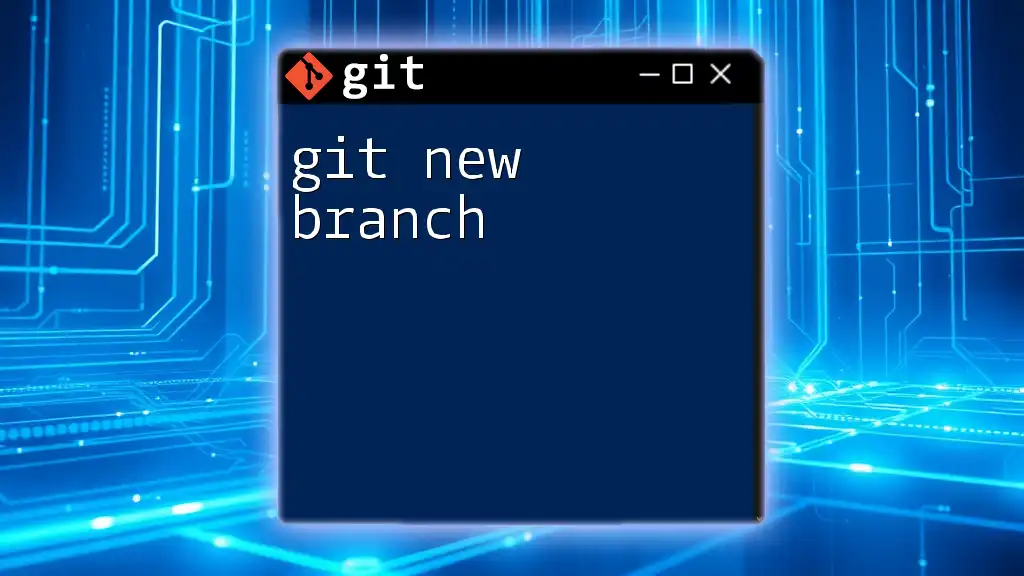
Conclusion
In summary, understanding how to git copy branch effectively is a valuable skill for any developer. It allows for enhanced collaboration, improved project organization, and the ability to revert to previous states if necessary. By practicing these commands and adhering to best practices in branch management, you'll become significantly more proficient with Git.
Additional Resources
For further information, the official Git documentation offers in-depth guidelines on commands, branching, and version control practices, supplementing the knowledge gained from this guide. It’s highly recommended to explore books, tutorials, and online courses to strengthen your understanding of Git for everyday development tasks.