Creating a new branch in Git allows you to encapsulate your changes without affecting the main codebase, enabling you to develop features or fix bugs in isolation.
Here's how to create a new branch:
git branch <branch-name>
To switch to the newly created branch, you can use:
git checkout <branch-name>
Or you can combine both commands into one with:
git checkout -b <branch-name>
What is a Git Branch?
A Git branch is essentially a pointer to a specific commit in your code repository. It allows you to diverge from the main line of development, enabling you to work on different features or fixes in isolation. This makes branching a fundamental aspect of Git's design. The branching model facilitates a more organized workflow, enabling experimentation and parallel development without risk to the main codebase.
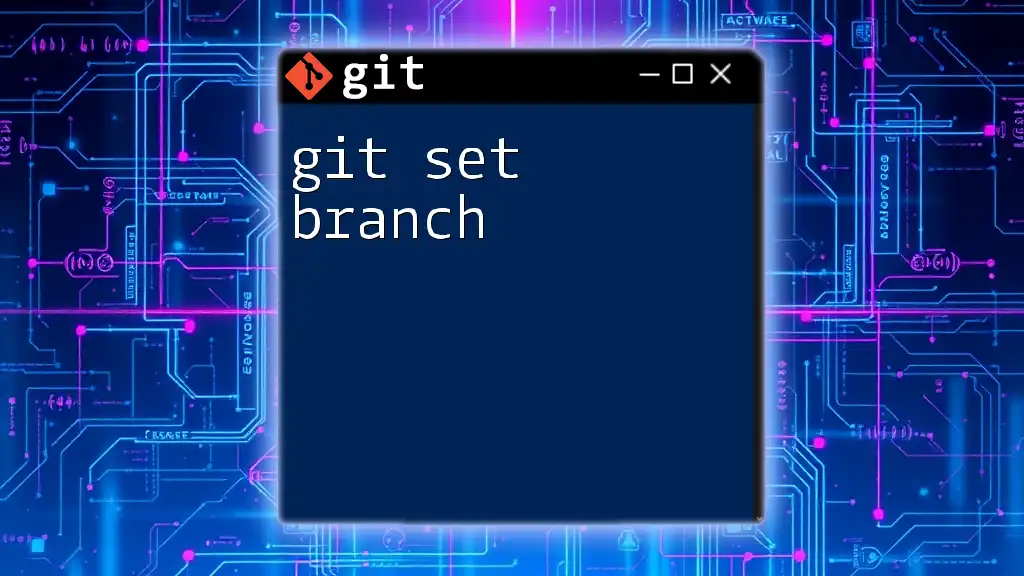
Why Create a New Branch?
Advantages of Using Branches
-
Separation of Features: Creating branches allows developers to work on different features independently. This means one developer can implement a new feature while another fixes bugs, all without conflicts.
-
Facilitates Collaboration: When working in teams, branches enable smoother collaboration. Multiple developers can work on their tasks simultaneously without interfering with each other's changes. Once the work is done, the branches can be merged back into the main branch.
-
Allows for Experimentation: If you're trying out new ideas or methodologies, branching permits you to test changes without affecting the main or production codebase. If the experiment fails, you can simply delete the branch and start over without any consequence.
Common Use Cases
-
Feature Development: When developing a new feature, it's common practice to create a branch specifically for that feature.
-
Bug Fixes: Branches can also be created to tackle specific bugs without disrupting the ongoing development in the main branch.
-
Experimentation: As mentioned, experimentation allows developers to prototype new features without any risk to the main application.
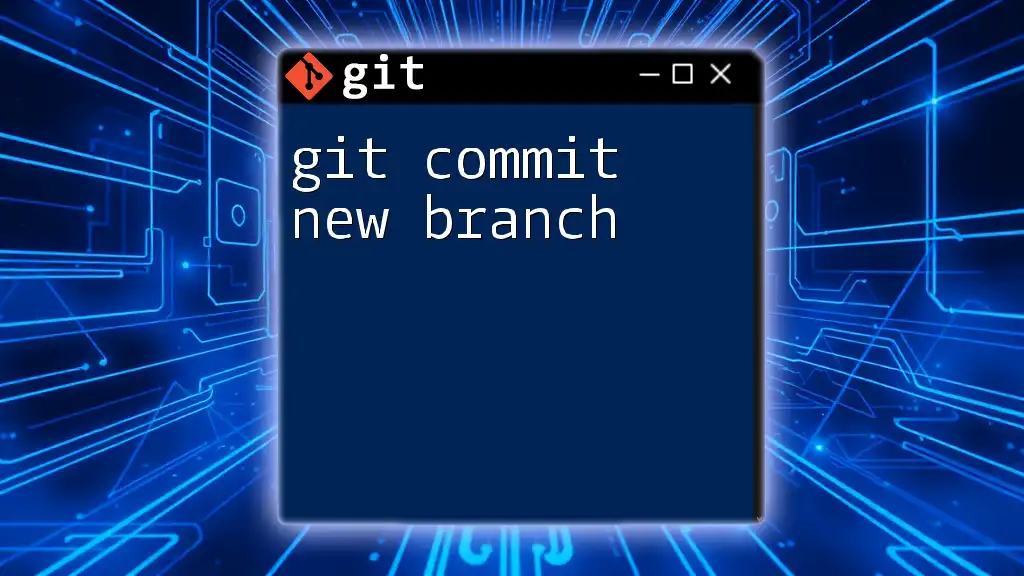
How to Create a New Branch in Git
Prerequisites
Before jumping into creating a new branch, ensure you have a foundational understanding of Git commands. Additionally, make sure that Git is installed on your machine, and you have access to the repository you wish to work on.
Creating a New Branch
Checking the Current Branch
First, it’s prudent to know which branch you are currently on. You can do this by running:
git branch
This command lists all branches in your repository, highlighting the active one.
Creating a Branch
To create a new branch, you can use the following command:
git branch [branch-name]
For example:
git branch feature/new-login
This command creates a new branch called `feature/new-login`. It's important to note that simply creating a branch does not switch you over to it.
Switching to the New Branch
Using `git checkout`
To start working on the newly created branch, you will need to switch to it. You can do this by using the `git checkout` command:
git checkout feature/new-login
This command switches your working directory to the `feature/new-login` branch, enabling you to begin your developments on this branch.
Using `git switch`
In newer versions of Git, you have an alternative command dedicated to switching branches:
git switch feature/new-login
This command essentially does the same thing as `git checkout` for branches but is more intuitive, focusing solely on the action of switching.
Creating and Switching in One Command
For even more efficiency, you can create and switch to a new branch in a single command using:
git checkout -b [branch-name]
For example:
git checkout -b feature/new-login
With this command, you create the `feature/new-login` branch and switch to it in one seamless step.
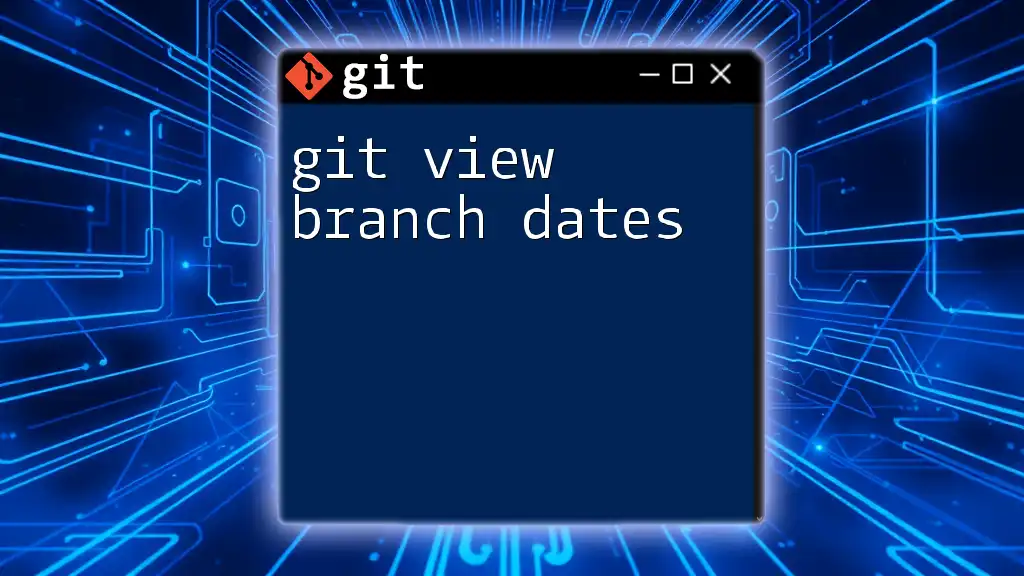
Best Practices for Branch Naming
Proper branch naming conventions can significantly improve transparency and maintainability within your projects.
Conventions for Branch Names
-
Be Descriptive: When naming your branches, ensure they are descriptive enough that others (or you in the future) can understand the purpose of the branch just by looking at its name.
-
Keep It Short, but Informative: While you want your names to be descriptive, there’s also value in brevity. Aim for clarity without excessive length.
-
Use Consistent Naming Conventions: Establish a standard format for branch names. For instance, use prefixes like `feature/`, `bugfix/`, or `hotfix/` to categorize purposes.
Examples of Good Branch Names
-
`feature/add-search-bar`: This branch name immediately suggests that it is meant to add a search bar functionality.
-
`bugfix/fix-login-error`: Clear and concise, this branch indicates a fix for an existing login error.
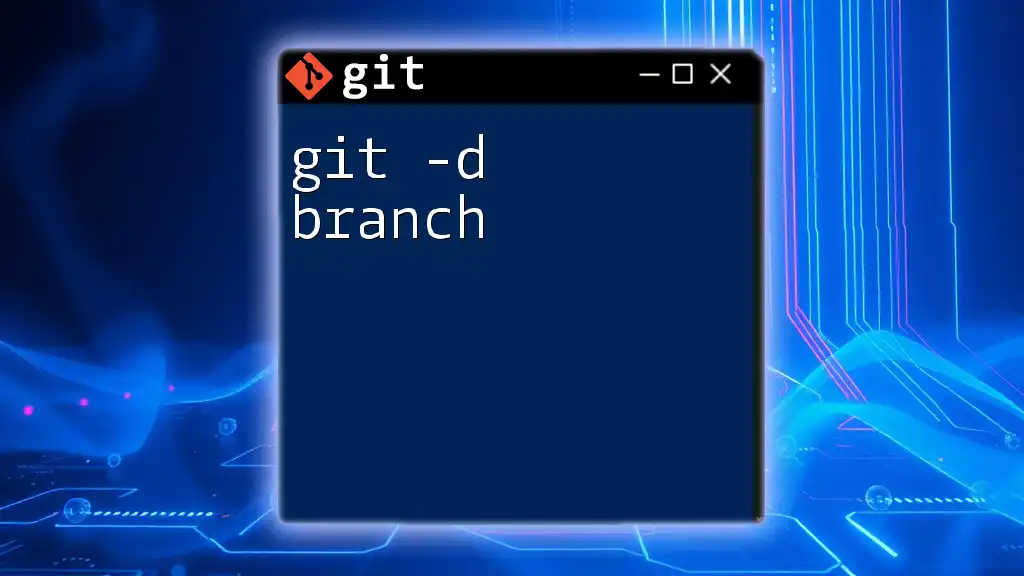
Working with Multiple Branches
Viewing All Branches
To see all the branches available in your repository, simply use the command:
git branch
This will show your current branch along with all other branches, making it easier to manage your workflow.
Deleting a Branch
When to Delete a Branch
Once you have merged a feature or completed your work, it may be appropriate to delete a branch that is no longer needed. This helps keep your repository clean and manageable.
Deleting a Local Branch
To delete a branch locally, you can use the following command:
git branch -d [branch-name]
For example:
git branch -d feature/new-login
This command will delete the `feature/new-login` branch, provided that it has been merged into the main branch.
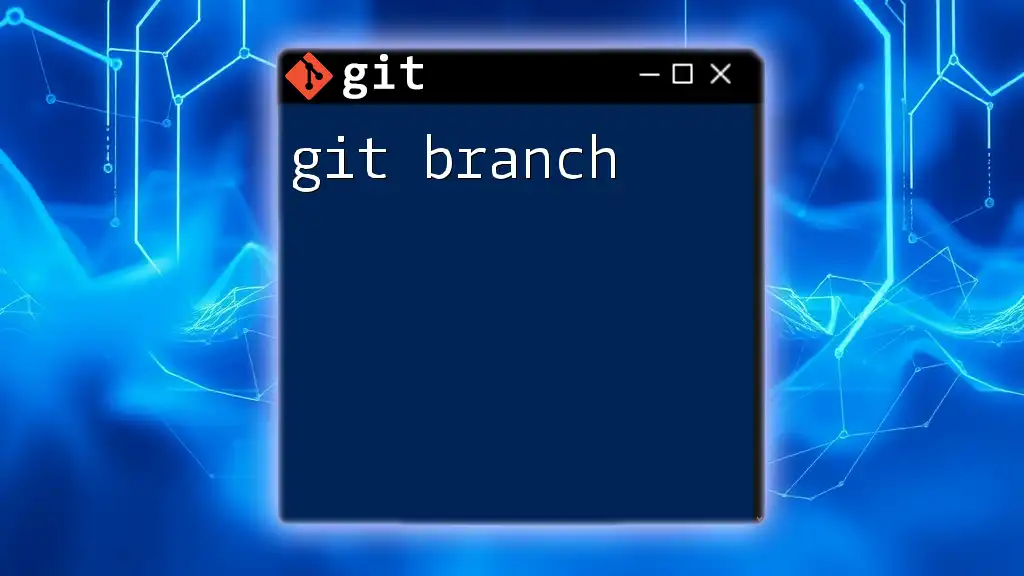
Conclusion
In conclusion, understanding how to create and manage a git new branch is crucial for any developer working with version control. Branches allow you to develop features, fix bugs, and experiment with new ideas while maintaining a clean and organized codebase. Mastering this aspect of Git will not only improve your individual productivity but also enhance your ability to collaborate effectively with your team.
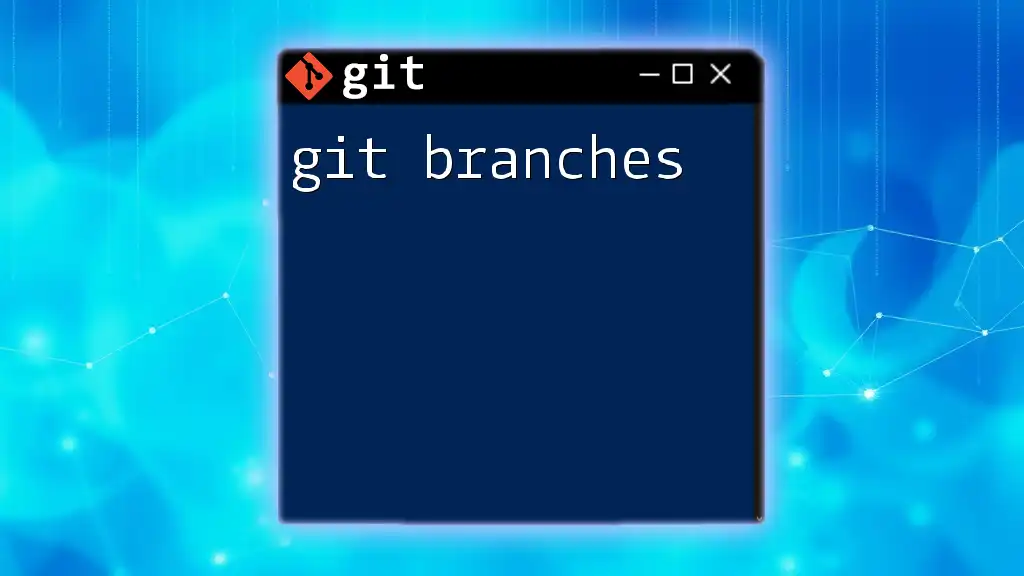
Call to Action
If you’re keen to improve your Git skills further, check out our additional resources on Git commands. We encourage you to practice creating and switching branches in your own projects! If you have any feedback or questions, feel free to leave them in the comments section. Happy branching!