To create a new branch in Git, use the following command to ensure isolation for your changes.
git branch <branch-name>
Replace `<branch-name>` with your desired name for the new branch.
Understanding Git Branching
What is a Git Branch?
A branch in Git is essentially a pointer to a specific commit in your project’s history. While the default branch (usually `main` or `master`) represents the base of your code, branches allow you to diverge from that point and develop independently.
Using branches is crucial when teams are collaborating on a single codebase. It enables developers to work on different tasks simultaneously without interfering with one another's code until the changes are ready to be merged back into the main line.
Why Use Branches?
Branches are essential for several reasons:
- Feature Development: Each new feature can be developed in its own branch, ensuring that the main codebase remains stable while new code is being tested.
- Bug Fixes: You can create a branch specifically for fixing bugs, making it easier to isolate the changes until they are confirmed to work.
- Experimentation: If you want to try out a new idea without any impact on your main project, creating a new branch allows you to do that freely.
In essence, branches provide an isolated environment for your work, which can simplify collaboration and version management.

Getting Started with Branches
Prerequisites
Before you can create a new branch in Git, you need to have the following prerequisites in place:
- Git Installed: Ensure you have Git installed on your device. You can check this by running `git --version` in your terminal.
- Basic Knowledge of Git Commands: Familiarity with commands such as `git commit` and `git push` will be beneficial.
- Access to a Git Repository: You should have the repository cloned to your local machine, or you can create a new one.
Initial Setup
To start working with Git branches in an existing repository, you'll need to clone it, if you haven’t already:
git clone <repository-url>
After cloning, navigate to the newly created directory:
cd <repository-name>
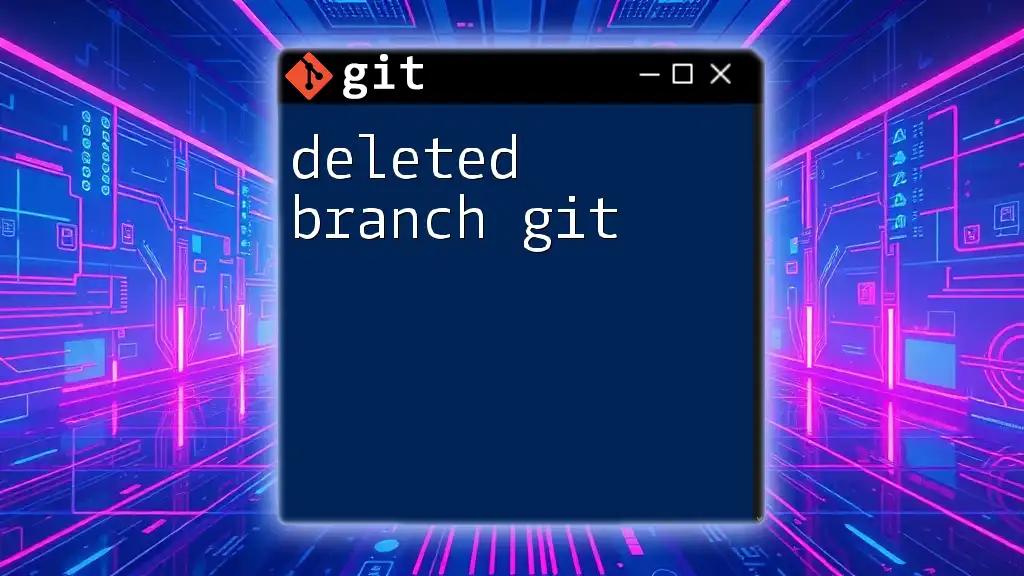
Creating a New Branch
Basic Command to Create a Branch
To create a new branch, you’ll use the `git branch` command followed by the name you want to give your branch. This is the syntax:
git branch <branch-name>
Here, `<branch-name>` should be descriptive of the task or feature you are working on. Good naming conventions can offer better clarity in version control.
Switching to the New Branch
Once your branch is created, you need to switch to it using the `git checkout` command:
git checkout <branch-name>
Alternatively, if you want to create the new branch and switch to it in one step, you can use the combined command:
git checkout -b <branch-name>
This can save time and streamline your workflow, allowing you to start working immediately on your new branch.
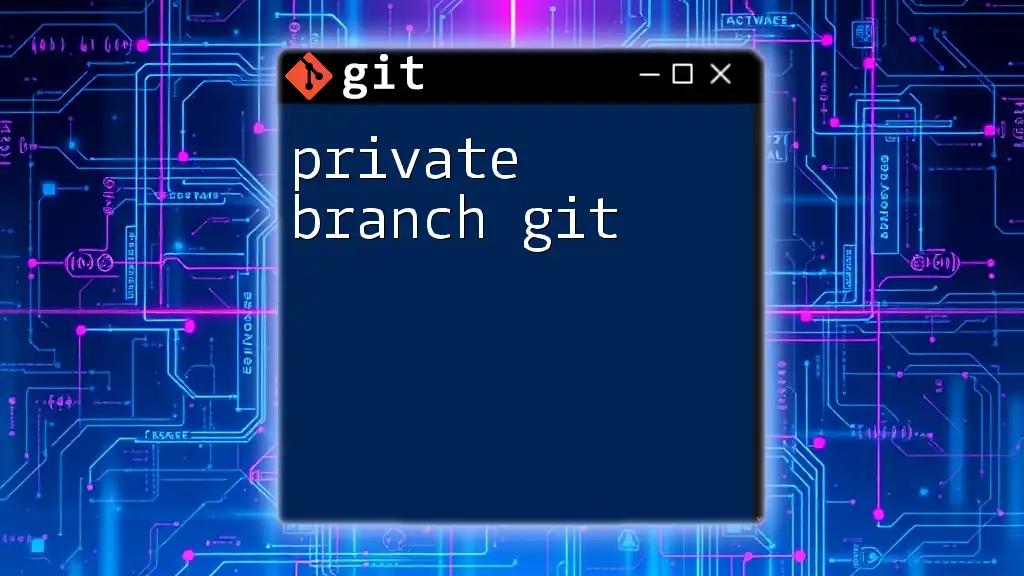
Best Practices for Branch Naming
Naming Conventions
Using a consistent naming strategy will help maintain an organized workflow. Here are some suggested conventions:
- Feature Branches: Prefix with `feature/` (e.g., `feature/user-authentication`)
- Bug Fixes: Prefix with `bugfix/` (e.g., `bugfix/login-error`)
- Hotfixes: Prefix with `hotfix/` for urgent fixes (e.g., `hotfix/crash-on-load`)
Examples of Good Branch Names
- For New Features: `feature/shopping-cart`
- For Bug Fixes: `bugfix/typo-in-header`
- For Hotfixes: `hotfix/payment-gateway-failure`
This clear naming can help team members quickly understand the purpose of each branch at a glance.
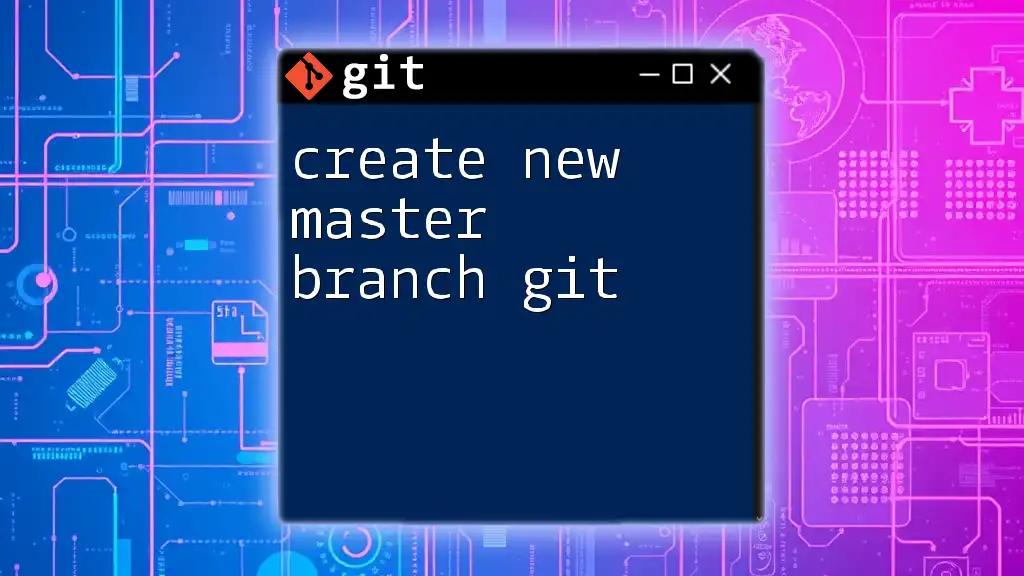
Working with Branches
Viewing Branches
To see a list of all the branches in your repository, including which one you are currently on, simply run:
git branch
The branch you are currently on will be indicated by an asterisk (`*`). This is helpful for knowing where you stand in development and which branch you need to switch to for a specific task.
Deleting a Branch
Once you have completed the work on a branch and merged changes back into the main branch, you may want to delete the unnecessary branch to keep your repository clean. To delete a branch, use:
git branch -d <branch-name>
Keep in mind that Git will prevent you from deleting branches that haven’t been merged to avoid losing work unintentionally. If you are sure and want to force delete, you can use:
git branch -D <branch-name>
This will delete the branch regardless of its merge status, so use it with caution.
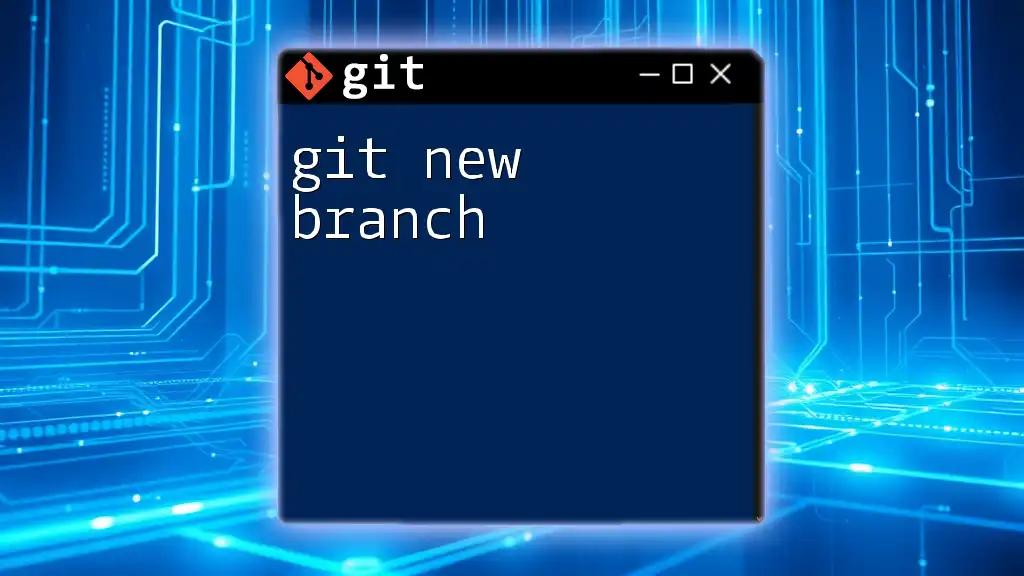
Conclusion
Creating a new branch in Git is a straightforward process that opens up a world of possibilities for managing your code and collaborating with others. With the ability to develop features, fix bugs, and experiment safely, branching is a crucial practice in modern software development. By following the best practices outlined in this article, you can ensure that your branching strategy remains effective and well-organized.
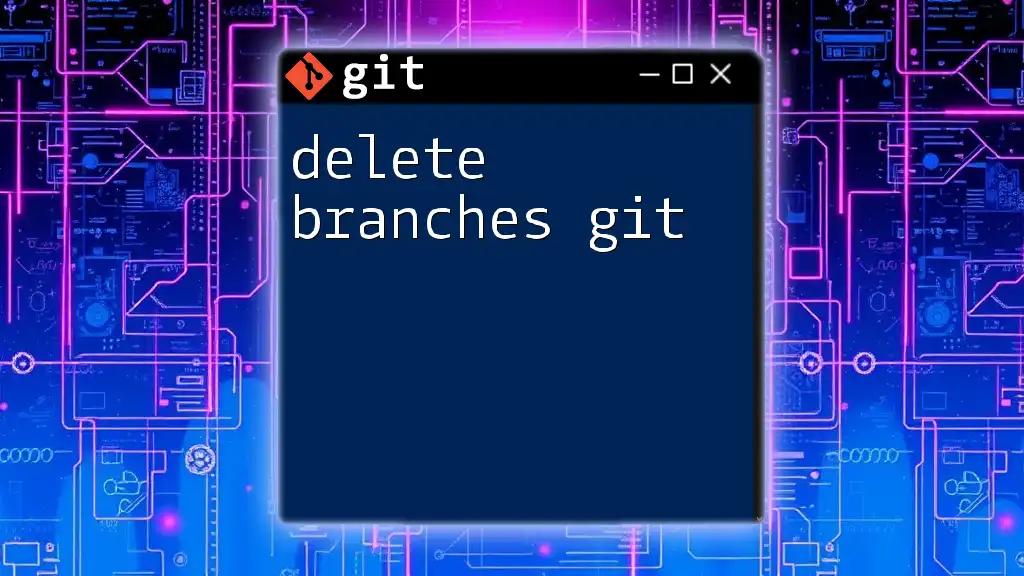
Additional Resources
- For deeper insights into Git functionalities, the official [Git Documentation](https://git-scm.com/doc) can be an invaluable resource.
- You might also consider looking for online tutorials or courses dedicated to mastering Git and version control best practices.
FAQs About Git Branching
-
What if I want to switch back to the main branch? Simply use the command:
git checkout main
-
Can I create a branch from another branch? Yes, simply checkout the branch you want to base your new branch on and then create the new branch.
-
How do I merge my branch back into main? After pushing your branch, you usually \create a pull request\ from your branch to the main branch, or use the command:
git checkout main git merge <branch-name>
With this knowledge, you are now equipped to confidently create new branches in Git and manage your workflows effectively!