To delete a Git branch both locally and remotely, you can use the following commands:
# To delete a local branch
git branch -d branch_name
# To delete a remote branch
git push origin --delete branch_name
Understanding Git Branches
What is a Git Branch?
A Git branch is essentially a pointer to a specific commit in your version history. Think of it as a separate line of development that allows you to work on features, bug fixes, or experiments without affecting the main codebase. Branching enhances collaboration and streamlines workflows, as multiple developers can work in parallel on different aspects of a project without stepping on each other’s toes.
Why Delete a Branch?
Deleting a branch may seem drastic, but it serves an essential purpose in repository management. Over time, especially in collaborative projects, many branches can accumulate. Deleting branches that are no longer needed keeps the repository clean and helps avoid confusion.
A cluttered repository can lead to:
- Difficulty in navigating the branch structure.
- Misunderstandings about which branch is the latest or most relevant.
- Increased risk of errors when merging or switching between branches.
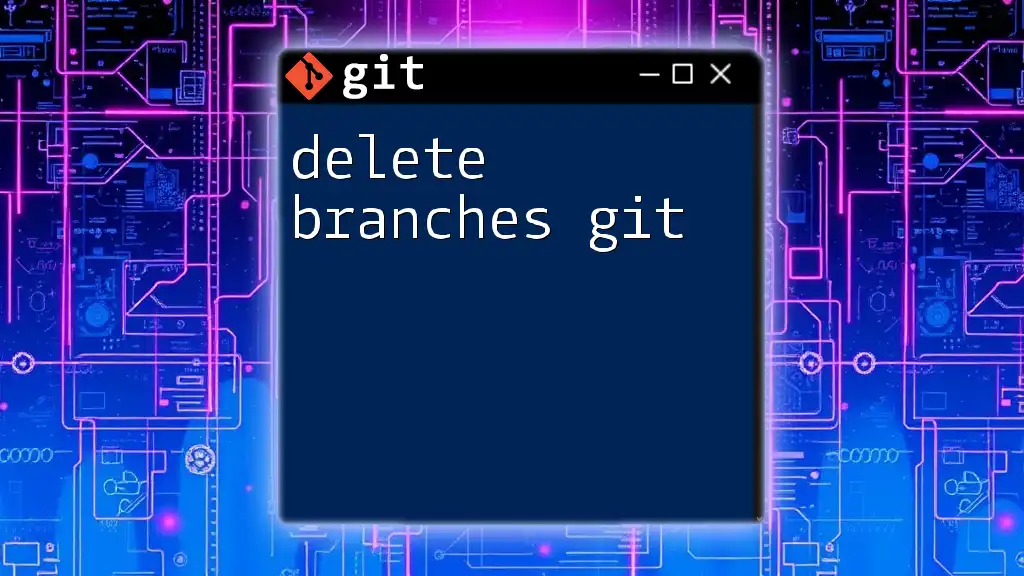
Deleting a Git Branch
Deleting a Local Branch
Local branches are branches that exist on your local machine. You might find yourself in a situation where you've completed a feature or a fix, and the branch is no longer relevant. To delete a local branch, use the following command:
git branch -d branch_name
The `-d` flag stands for delete. This command checks to see if the branch has unmerged changes. If there are unmerged changes, Git will prevent you from deleting the branch to protect any work that might be lost.
Important Consideration: The general rule of thumb is only to delete branches that are fully merged into your main branch (often `main` or `master`). If you're sure that you no longer need a branch, even if it has unmerged changes, you can forcefully delete it using:
git branch -D branch_name
This command removes the branch regardless of its merge status. Use it cautiously, as any unmerged work will be lost.
Deleting a Remote Branch
Remote branches are branches that are stored on a remote server (like GitHub). When you delete a local branch, it does not automatically remove the corresponding remote branch. To clean up your remote repositories, follow these steps:
To delete a remote branch, use this command:
git push origin --delete branch_name
Here, `origin` refers to the default remote name. This command informs the remote server to remove the specified branch.
Why is this important? As with local branches, cleaning up remote branches ensures that your collaborators see only the relevant branches, reducing confusion and potential merge issues.
Checking for Active Remote Branches
Sometimes, before deleting a remote branch, you may want to review which branches are active. To list all remote branches, run:
git branch -r
This command will give you a clear view of the branches available on the remote repository and help you identify the ones that may need to be deleted.
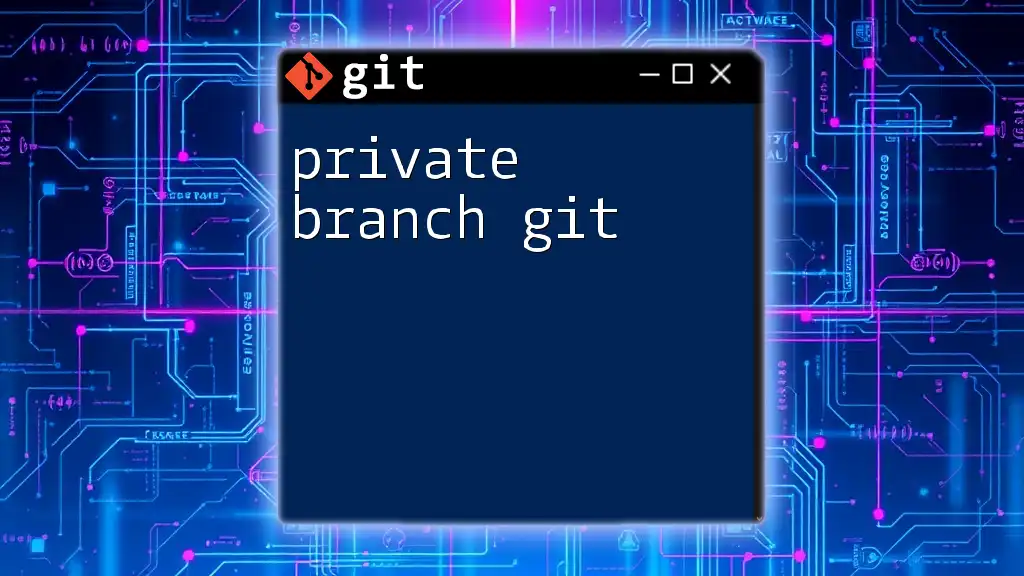
Recovering Deleted Branches
Can You Recover a Deleted Branch?
Once a branch is deleted using the commands mentioned above, it’s not entirely gone. Git maintains a history of commits, which can be accessed through the reflog. The reflog is a log of where your HEAD has been, allowing you to track commits even after their corresponding branches have been deleted.
Step-by-Step: Recovering a Deleted Branch
If you realize you need a deleted branch, recovery is possible. Start by checking the reflog with:
git reflog
This command will display a list of your recent commits along with their associated commit hashes. Find the commit hash corresponding to the deleted branch you want to recover.
Next, you can recreate the deleted branch at that commit:
git checkout -b branch_name commit_hash
This command creates a new branch from the specified commit, effectively restoring your work.
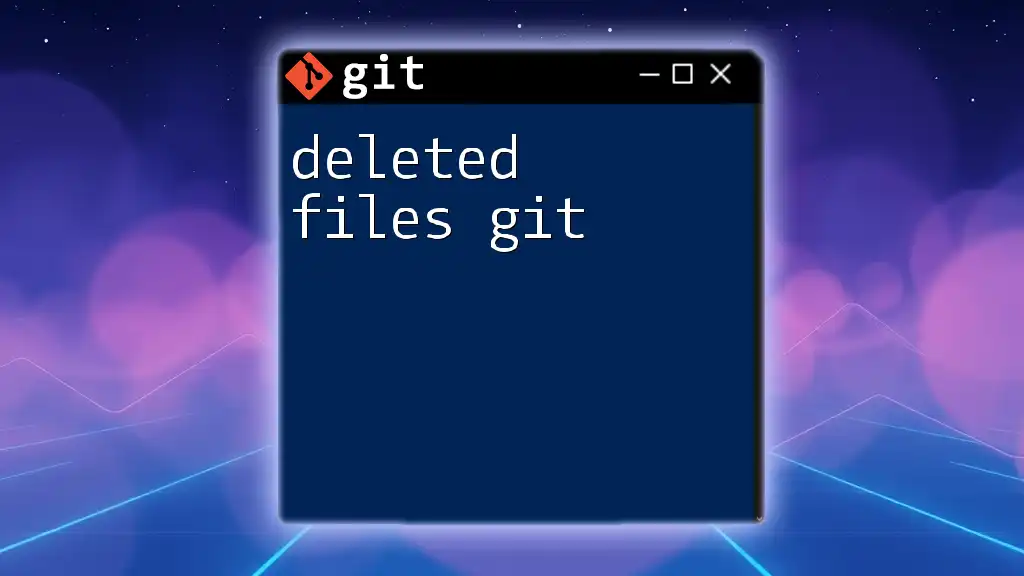
Best Practices for Branch Management
Naming Conventions for Branches
Establishing a clear naming convention for your branches can significantly ease navigation through your repository. Consider using prefixes that indicate the type of work being done, such as:
- `feature/` for new features (e.g. `feature/user-authentication`)
- `bugfix/` for bug fixes (e.g. `bugfix/fix-crash-on-start`)
- `hotfix/` for urgent fixes (e.g. `hotfix/urgent-security_patch`)
Regular Maintenance for Git Repositories
It’s crucial to periodically review your branches for unnecessary clutter. Regular maintenance helps to ensure that your repository remains well-organized. Automate this process with scripts or tools that can identify or prune stale branches, especially in collaborative environments where branches may linger after their purpose has been served.
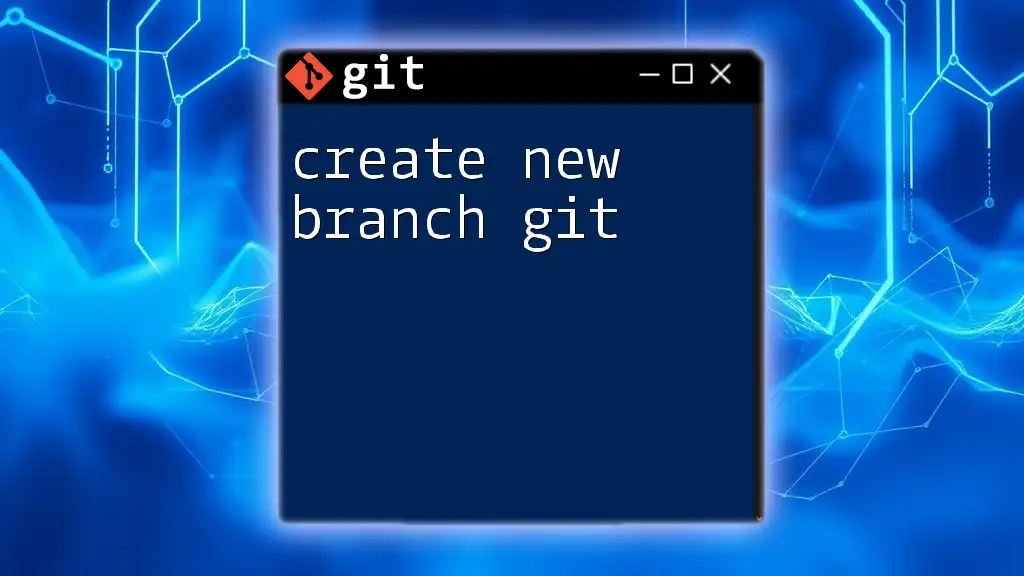
Conclusion
Managing branches in Git, especially regarding deleted branches, is pivotal for maintaining a clean and efficient workflow. Always be mindful of the implications of deleting branches, whether local or remote, and utilize Git's features like the reflog for recovery when needed. Embrace best practices to keep your branches organized, ensuring a seamless collaborative experience for you and your team.
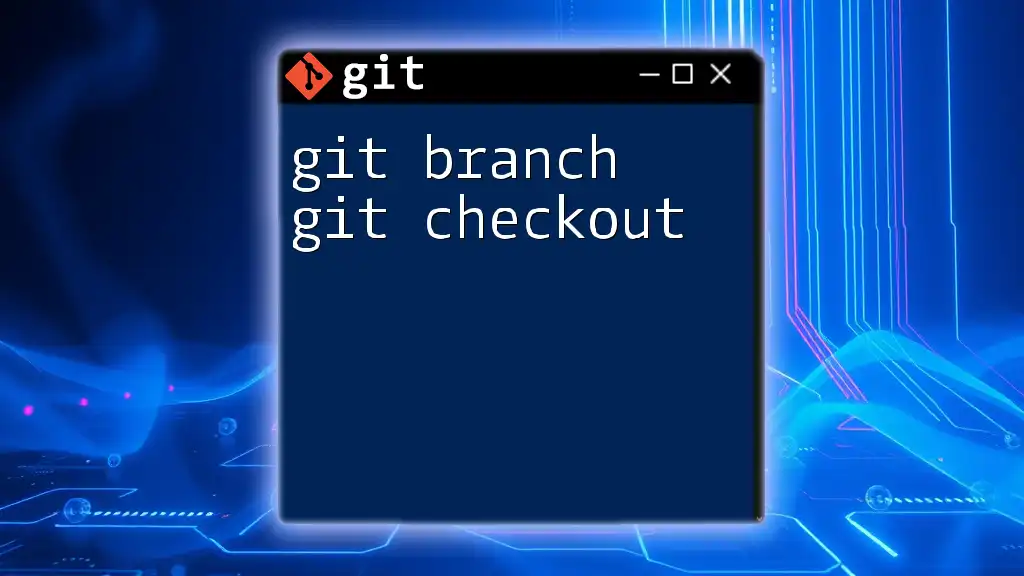
Additional Resources
For further reading on effective Git branch management and command use, explore the official Git documentation or consider tools like GitKraken and SourceTree that offer GUI options for managing branches visually. Remember, practicing these Git commands and techniques will improve your efficiency and confidence in using version control.