In Git, when you delete a file and want to stage that change for committing, you can use the `git rm` command followed by the file name.
git rm filename.txt
Understanding Deleted Files in Git
What Happens When You Delete a File?
When you delete a file from your working directory in a Git repository, that action does not immediately remove it from the Git history. Instead, Git simply marks the file as deleted in the next commit. This is a crucial aspect of Git's version control system—it allows you to keep track of changes, including deletions. Understanding this can help you recover lost files easily and manage your repository more effectively.
Types of Deletions
Tracked Files vs. Untracked Files
A tracked file is one that Git is aware of—it's been staged or committed at some point. On the other hand, an untracked file has never been staged or committed. Deleting an untracked file does not have implications for the version history, but deleting a tracked file affects the version history and can be recovered if necessary.
Soft Deletes vs. Hard Deletes
In Git, a soft delete refers to removing a file only from the working directory, making it unavailable in the current commit, but still recoverable. A hard delete occurs when you remove a file permanently so that it cannot be found in any commit history. Understanding these types is vital to carry out deletions safely without losing important data.
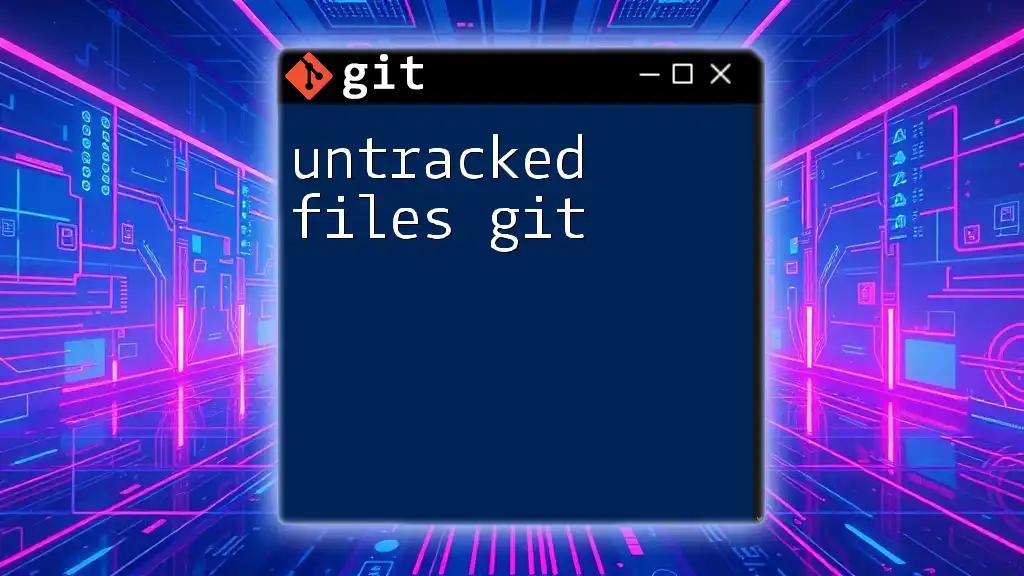
How to Recover Deleted Files
Recovering with `git checkout`
The `git checkout` command allows you to restore a deleted file from a previous commit. For instance, if you accidentally deleted a file but have not yet committed that change, you can easily recover it with the following command:
git checkout HEAD -- path/to/deleted-file.txt
In this command:
- `HEAD` refers to the most recent commit.
- The path directs Git to the specific file you want to recover.
This command restores the file to the state it was in at the last commit, allowing you to move forward without losing any work.
Using `git restore` (Git 2.23+)
With Git versions 2.23 and newer, the `git restore` command offers a more straightforward way to recover deleted files. This command is specifically designed for restoring files and has a simpler syntax. You can recover a deleted file using:
git restore path/to/deleted-file.txt
This command restores the deleted file from the last committed state or the index, making it easy to use.
Recovering from Staging Area
You may also find yourself needing to recover a file that was staged for commit but then deleted. In such cases, the `git reset HEAD` command proves handy. This command will unstage the file while keeping it in your working directory.
git reset HEAD path/to/deleted-file.txt
This lets you recover the file back into your working directory to continue editing or reviewing it before making a new commit.
Viewing Deleted Files in the Reflog
The Reflog is a powerful tool in Git that tracks updates to your HEAD. If you accidentally delete a file, you might be able to find it through the reflog. Use the command:
git reflog
This shows a history of where HEAD has pointed over time. By reviewing this log, you can locate the commit before the deletion took place and recover the file from that point.
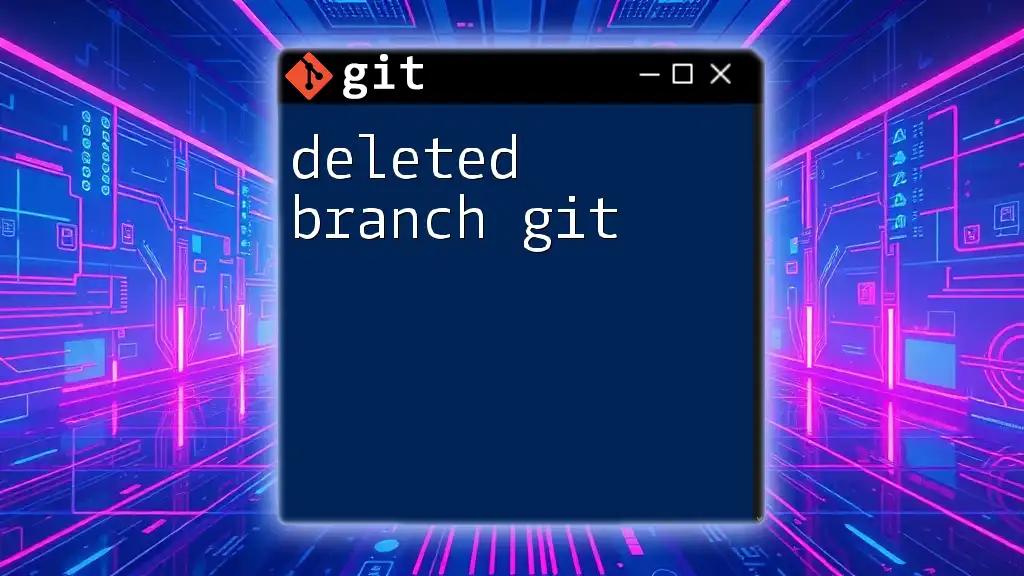
Permanently Deleting Files
The Impact of Hard Deletes
Using the command `git rm` allows you to remove files from the repository, but it permanently deletes the files from the working directory as well. This can be problematic if you didn't intend to remove the file entirely. Here’s how you might use this command:
git rm path/to/deleted-file.txt
Once you execute this command and commit the change, the file is deemed permanently deleted from the repository history, making recovery impossible unless a backup exists.
Unrecoverable Deletions
Certain situations lead to files becoming unrecoverable. If you delete a file with `git rm` and then commit that change, you generally cannot recover that file unless you navigate back through the history using tools like `git reflog`. It emphasizes the importance of cautious actions while working with Git, particularly when deleting files.
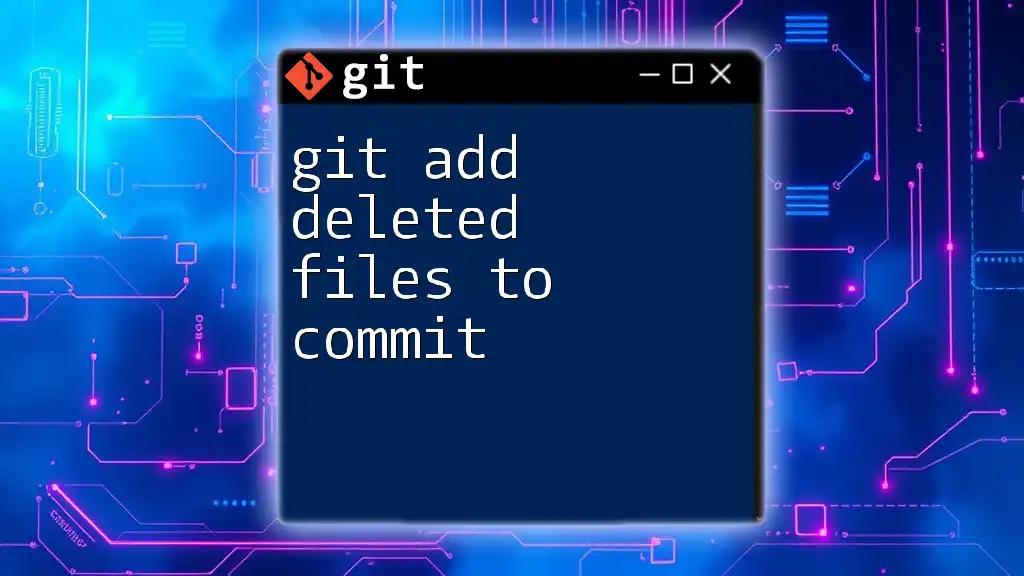
Best Practices for Managing Deleted Files
Regular Commits
One of the best practices in Git is to commit changes regularly. Committing often not only minimizes data loss risks but also provides more recovery points. The more often you commit, the more states you can revert to if a deletion or error occurs.
Understanding .gitignore
Using `.gitignore` effectively helps in managing files you don't want Git to track. Files listed in `.gitignore` will not be added to Git's version control, reinforcing the importance of knowing which files belong in your repository. This avoids accidental deletions or conflicts when you're not concerned with certain files.
Leveraging Branches
Creating branches is an excellent strategy for protecting your main working directory. By developing features or experimentation in separate branches, you reduce the likelihood of unintended deletions affecting your main codebase. Use the following command to create a new branch:
git checkout -b feature-branch
With branches, you can confidently make changes, test different ideas, and only merge them into the main branch when you're sure they are successful.
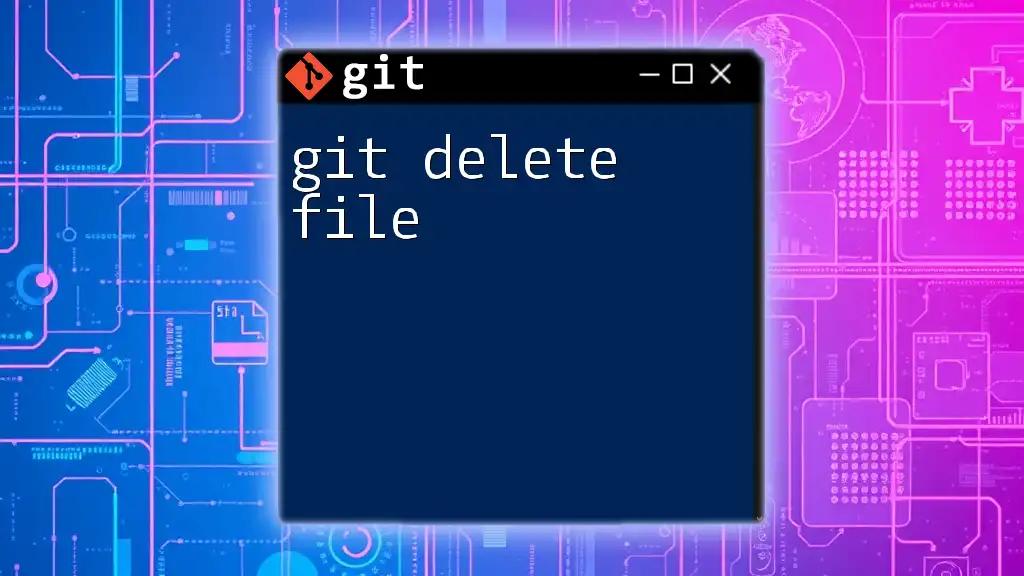
Conclusion
Understanding how to manage deleted files in Git is essential for any developer. By familiarizing yourself with the various commands available for restoring these files, you enhance your ability to navigate around potential issues. Regular commits, effective use of `.gitignore`, and maintaining clear branching strategies are vital practices to prevent data loss. Embrace these principles, practice recovery techniques, and ensure a smoother workflow in your projects.