To delete all Git tags from your repository, you can use the following command:
git tag -d $(git tag)
Understanding Git Tags
What Are Git Tags?
Git tags are essentially bookmarks within your version control system that mark specific points in your project’s history. They’re often used to denote important milestones such as releases or versions. Tags help you quickly return to a specific commit without sifting through your entire history.
There are two types of tags:
- Lightweight Tags: These are simple references to a specific commit. They do not contain any additional information aside from the commit identifier.
- Annotated Tags: These tags are more complex and include a tag message, the tagger's name, and the date. They serve as snapshots of your project with meaningful descriptions, making them incredibly useful in team environments.
Why You Might Want to Delete Tags
There are several valid scenarios to delete all tags in Git:
- Obsolete Tags: If a tag has become irrelevant due to project changes or if it was mistakenly created.
- Renaming: When tags need to be updated or renamed to reflect new practices or conventions.
- Mistakes: In case a tag was created incorrectly, deleting it can help maintain a clean project history.
However, it’s important to understand that deleting tags is irreversible. Therefore, it's prudent to back up your tags before proceeding.
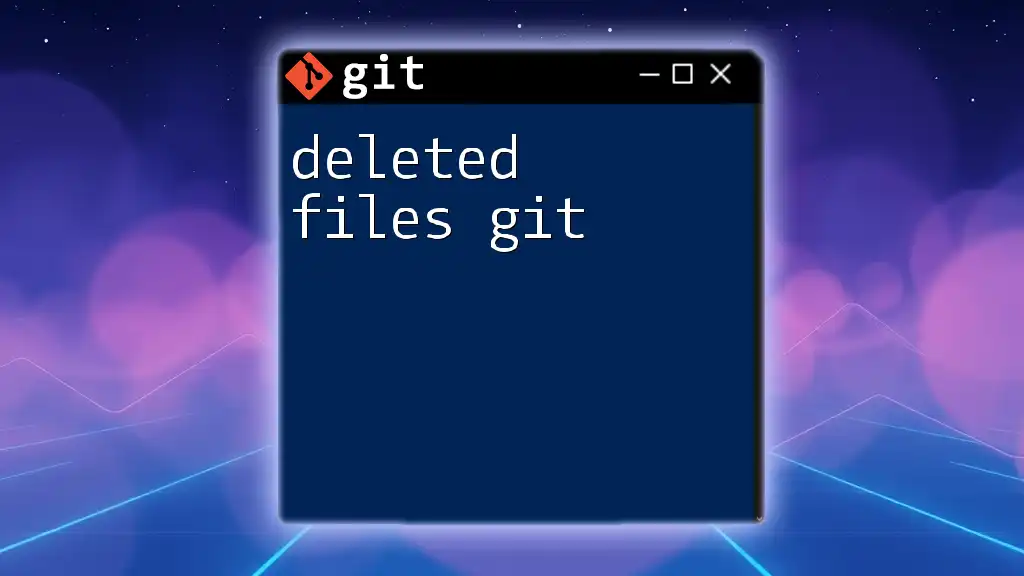
Prerequisites
Basic Git Commands
Before diving into the deletion processes, a brief refresher on essential Git commands will set the stage for efficiency. Familiarity with commands like `git tag`, `git push`, and `git fetch` is essential for managing your repository effectively.
Ensuring Backup
To prevent the loss of valuable history, always back up your tags. You can create a backup by exporting your tags to a file. Here’s how you can do it:
git tag > tags_backup.txt
This will create a text file listing all your tags, ensuring you have a reference if needed later.
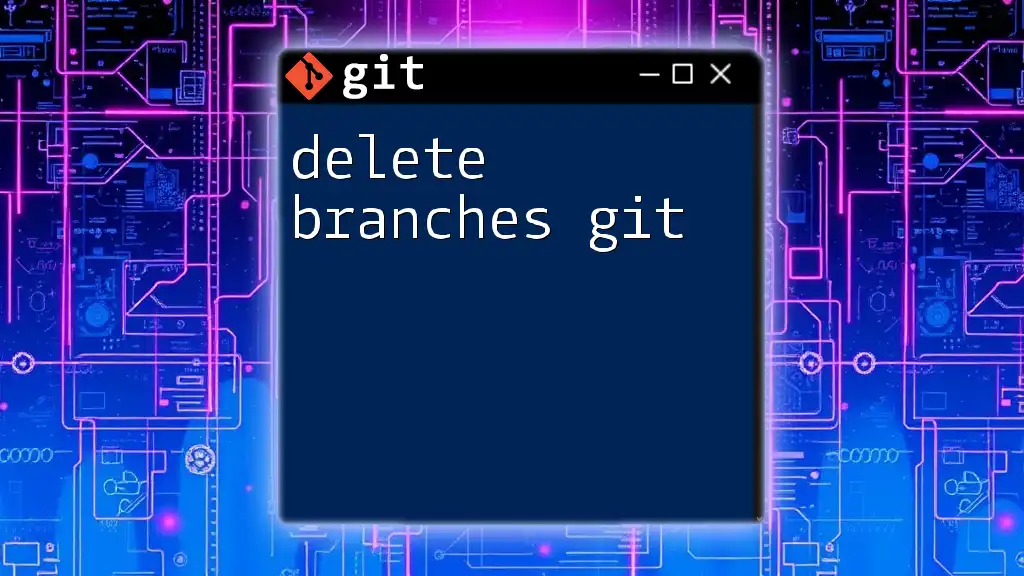
How to Delete All Tags in Git
Deleting Local Tags
Using the Git Command Line
To delete tags locally, you can use the following syntax:
git tag -d <tagname>
For instance, if you want to delete a specific tag named `v1.0`, you would execute:
git tag -d v1.0
This command removes the specified tag from your local repository.
If your goal is to delete all local tags efficiently, you can leverage a command that combines listing tags and passing them to the delete command:
git tag | xargs git tag -d
This command first lists all tags and then pipes them to the `git tag -d` command, deleting each one in a single operation.
Deleting Remote Tags
Pushing Deletions to Remote Repository
In many cases, you also need to remove these tags from your remote repository. The syntax to delete a tag from the remote is:
git push --delete origin <tagname>
For example, to delete the tag `v1.0` from the remote repository, execute:
git push --delete origin v1.0
To delete all remote tags, you can use the following command:
git tag | xargs -n 1 git push --delete origin
This command, similar to the local tag deletion, fetches all tags and successively deletes them from the remote.
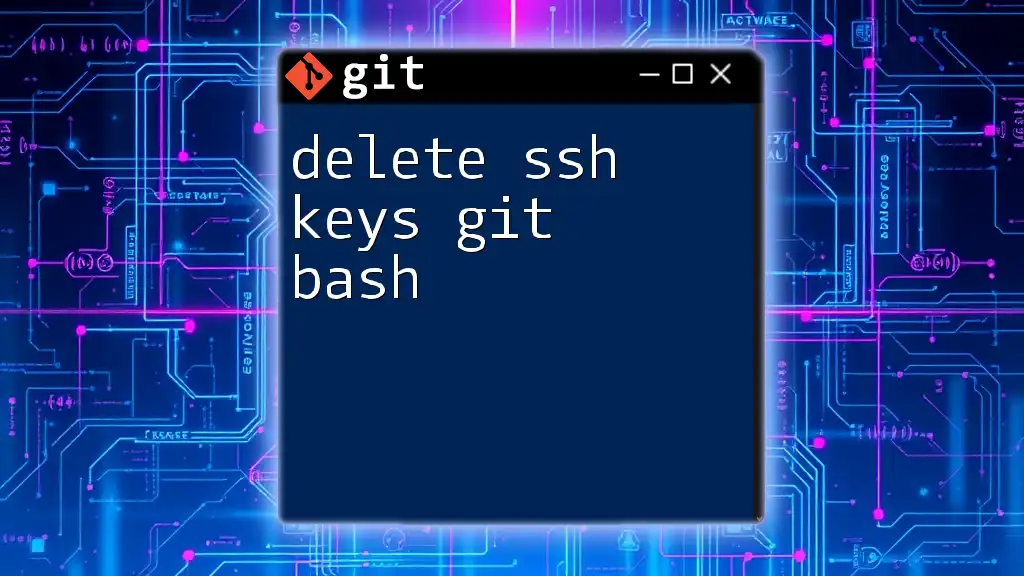
Verifying Tag Deletions
Checking Local Tags
After deleting tags, it’s crucial to verify that the local deletions were successful. You can check your remaining local tags by running:
git tag
If all has gone well, this command should return an empty list.
Checking Remote Tags
To confirm the deletion of tags from the remote repository, use:
git ls-remote --tags origin
This command will list all existing tags in the remote repository, allowing you to validate that the unwanted tags have been properly removed.
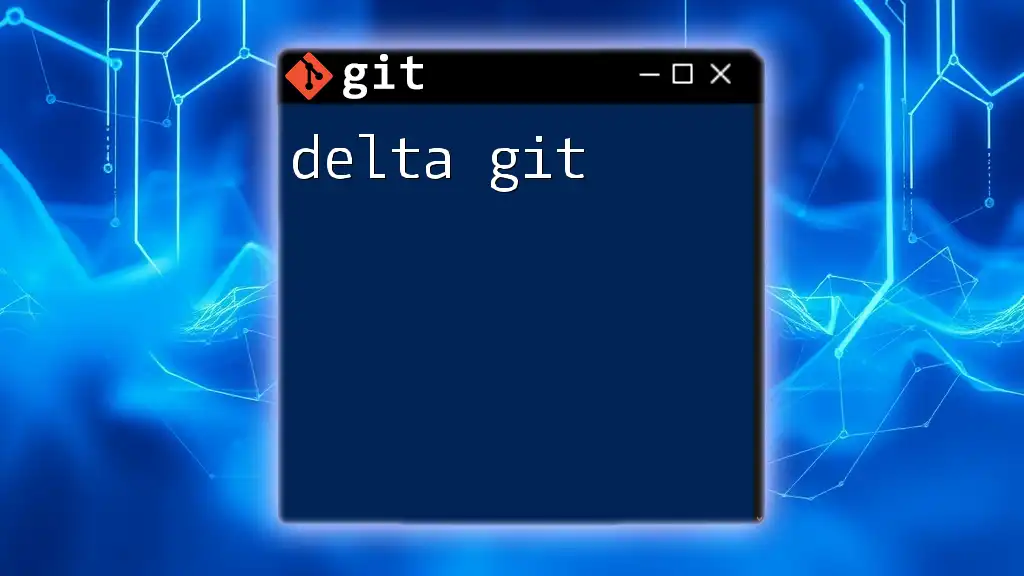
Alternative Approaches to Tag Management
Using GUI Tools
For those less comfortable with command-line interfaces, GUI tools like GitKraken and Sourcetree can simplify tag management. These tools provide a visual interface that lets you easily add, edit, and delete tags. However, keep in mind that while these can be user-friendly, they may not always offer the same power or flexibility as command-line operations.
Scripting for Advanced Users
For more advanced users, scripting can automate the tag deletion process. Here is a sample bash script that deletes all local and remote tags:
#!/bin/bash
git tag | xargs git tag -d
git tag | xargs -n 1 git push --delete origin
This script can save time and effort, especially in repositories with numerous tags. Automating tag management can ease the administrative overhead in larger projects.
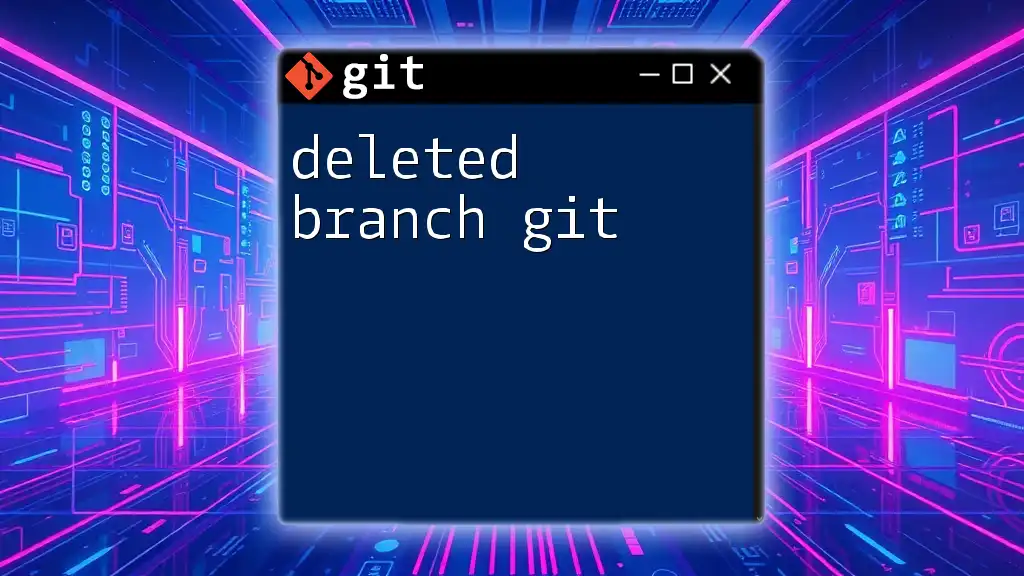
Conclusion
In summary, managing your Git tags effectively is crucial for maintaining a tidy version history. Deleting all tags in Git can be accomplished both locally and on remote repositories through straightforward commands. Regularly audit your tags to ensure they reflect the current state of your project effectively.
Acquiring mastery over these commands will empower you to manage your repository efficiently, ensuring that your version control system remains organized and streamlined.
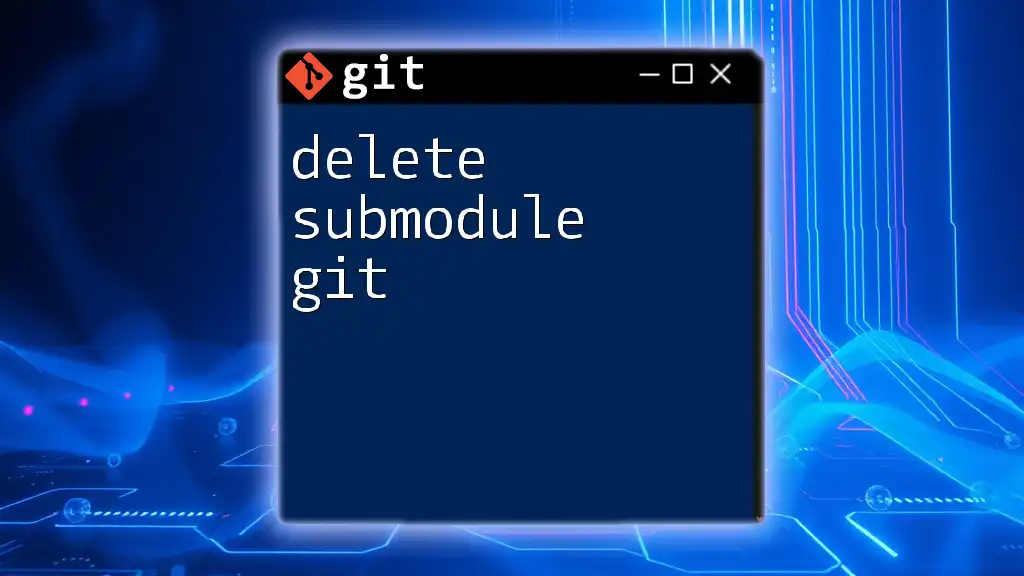
Call to Action
If you found this guide helpful, consider subscribing for more quick and concise tutorials on Git commands. Sharing your experiences, tips, or questions about tag management in the comments section would greatly enrich our community discussions!
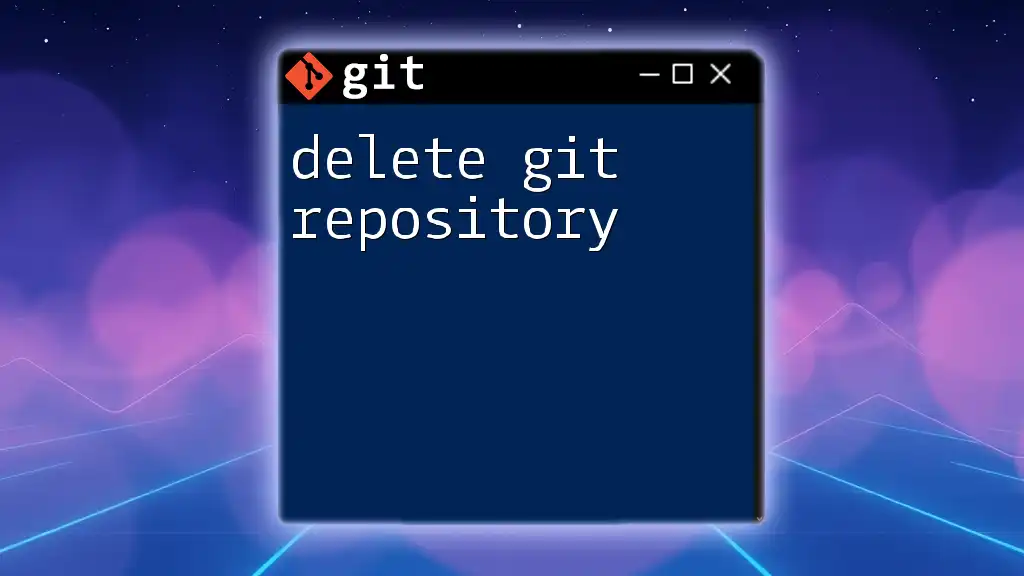
Additional Resources
For further reading on Git tags, consider exploring best practices in version control. Enhance your skills with recommended tools designed for effective project management.