To delete a submodule in Git, you need to remove it from the `.gitmodules` file, delete the submodule entry from the Git index, and then remove the submodule's files from your working directory using the following commands:
git config -f .gitmodules --remove-section submodule.<submodule-name> && \
git config -f .git/config --remove-section submodule.<submodule-name> && \
git rm --cached <submodule-name> && \
rm -rf <submodule-name> && \
git commit -m "Removed submodule <submodule-name>"
Understanding Submodules
What is a Git Submodule?
A Git submodule is essentially a repository embedded within another repository. This allows developers to keep their project modular by implementing a separate Git repository as part of their main project. Submodules come in handy when you want to include libraries or components that are developed independently but are needed in your application.
Benefits of Using Submodules
Using submodules offers several advantages:
- Modular Architecture: Submodules allow you to maintain distinct repositories for different components, enhancing project organization.
- Version Control for Dependencies: Each submodule can be version-controlled independently, which provides flexibility in managing dependencies.
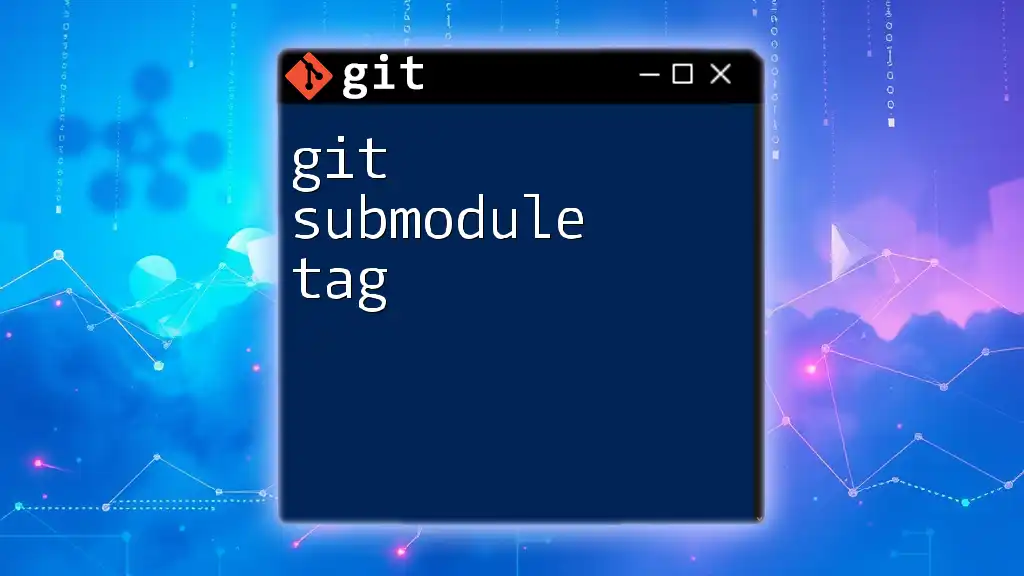
Preparing to Delete a Submodule
Before deleting a submodule, it's crucial to confirm your current setup. This involves checking existing submodules and understanding their configurations.
Confirming Your Current Setup
You can check your submodules by inspecting the `.gitmodules` file, which contains pertinent information about your submodules and their paths. Use the following command to view your submodule configuration:
git config --file .gitmodules --get-regexp path
This will allow you to assess which submodules are currently active.
Understanding Dependencies and Impacts
Before proceeding, consider the potential impacts of removing a submodule. This is particularly important if other parts of your project rely on this submodule. To avoid breaking dependencies, ensure nothing will be adversely affected by the removal.
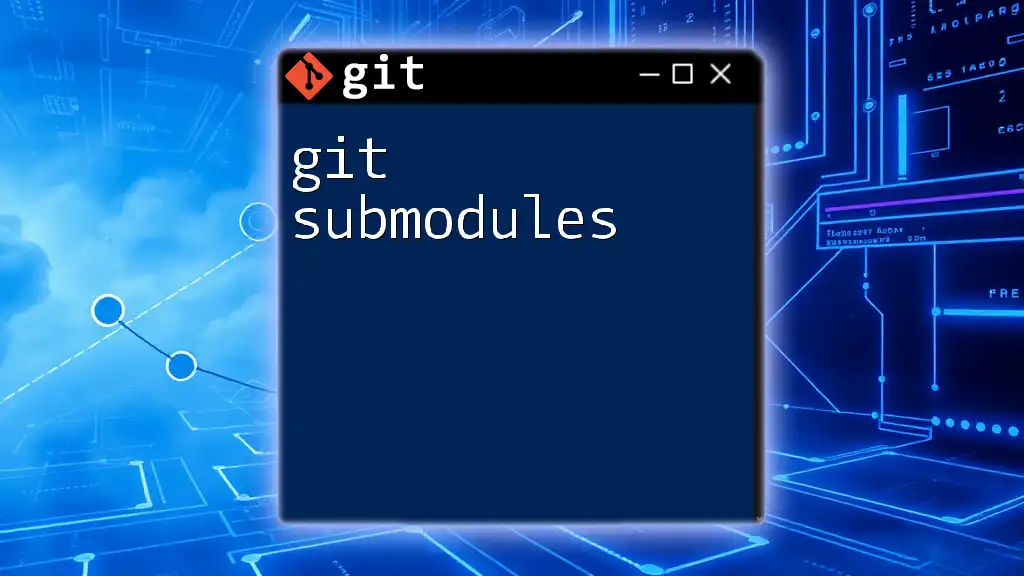
Steps to Safely Delete a Submodule
To effectively delete a submodule, follow these steps to ensure a clean removal without running into issues.
Step 1: Deinitialize the Submodule
The first step in the deletion process is to deinitialize the submodule. Running the following command clears the submodule's cached data and removes it from the working tree:
git submodule deinit <submodule-path>
This command removes the submodule's configuration from your working directory but does not delete any files yet.
Step 2: Remove the Submodule's Entry from the Configuration File
Next, you need to edit the `.gitmodules` file to remove the submodule's entry. Open the file using a text editor:
nano .gitmodules
Inside this file, find and delete the section that corresponds to the submodule you're removing. By doing this, you're ensuring that your repository does not reference a non-existent submodule.
Step 3: Remove the Submodule Directory
Now that the submodule is deinitialized and its configuration removed, you should delete the submodule's folder from your working tree. This can be done using the following command:
rm -rf <submodule-path>
This command permanently removes the submodule's files from your local repository.
Step 4: Remove the Submodule from Git Index
It’s essential to also remove the submodule from Git’s index to ensure it's no longer tracked. You can achieve this using the command:
git rm --cached <submodule-path>
Running this command tells Git to stop tracking the submodule. Note that this does not delete the submodule's files at this point; they're already removed from the working directory.
Step 5: Commit Your Changes
Once you've followed the previous steps, commit your changes to reflect the removal of the submodule in your repository’s history:
git commit -m "Removed submodule <submodule-name>"
Committing these changes is crucial as it ensures that the removal is documented, allowing for better project management and version control.
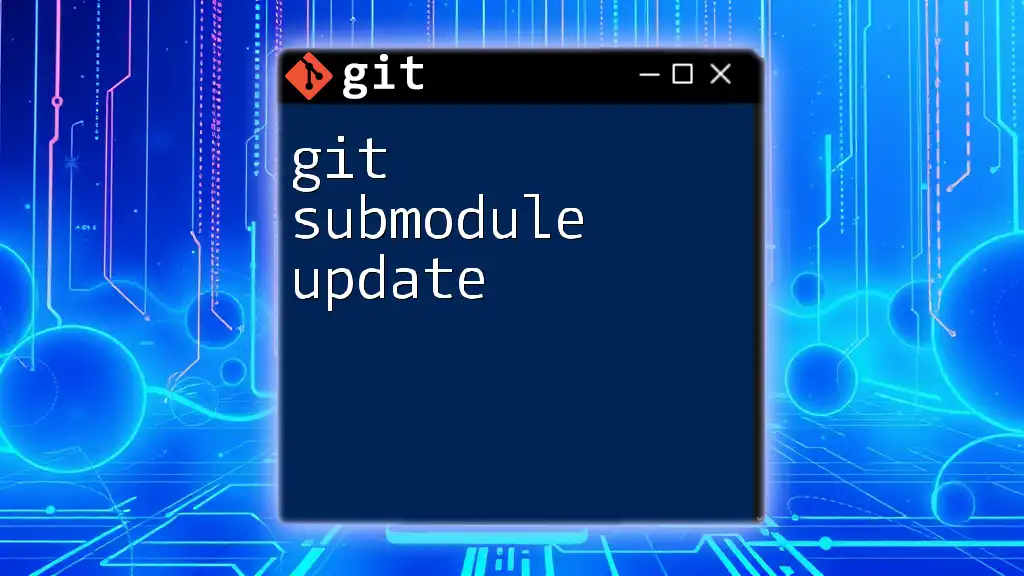
Verifying Removal of the Submodule
To confirm the successful removal of the submodule, run the following command:
git status
This command will help you check for any untracked files or other changes in your repository. Ensure that the submodule path you removed is no longer listed.
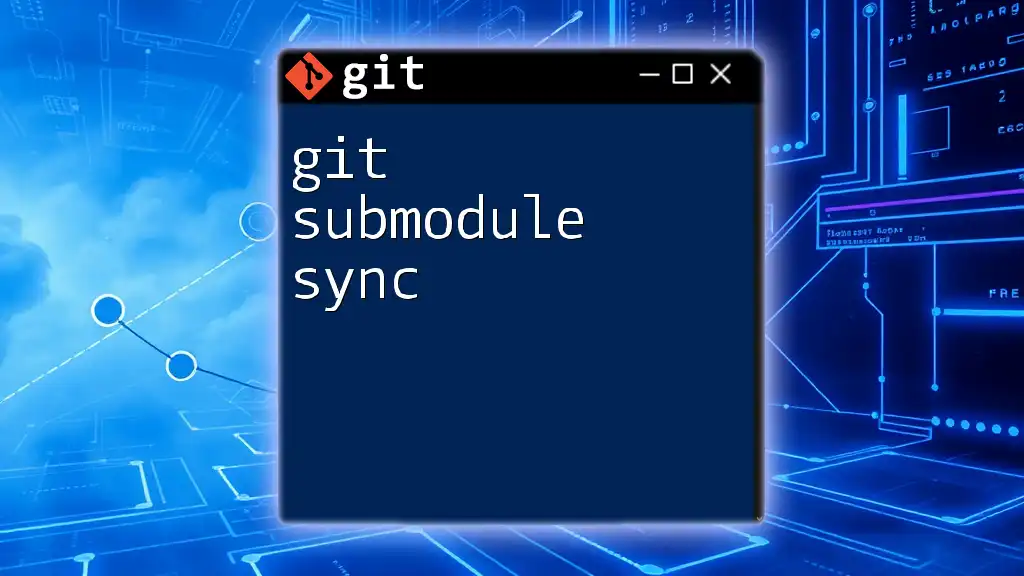
Common Issues When Deleting a Submodule
When deleting a submodule, you may encounter several errors or issues. Here are some common mistakes to watch out for:
- Untracked Files: Sometimes, after removing a submodule, you might find that Git still tracks some files. Always ensure that you remove all cached references.
- Commit Failures: If you forget to commit your `.gitmodules` modifications, it could lead to inconsistencies in your repository.
Always double-check each removal step to prevent these issues.
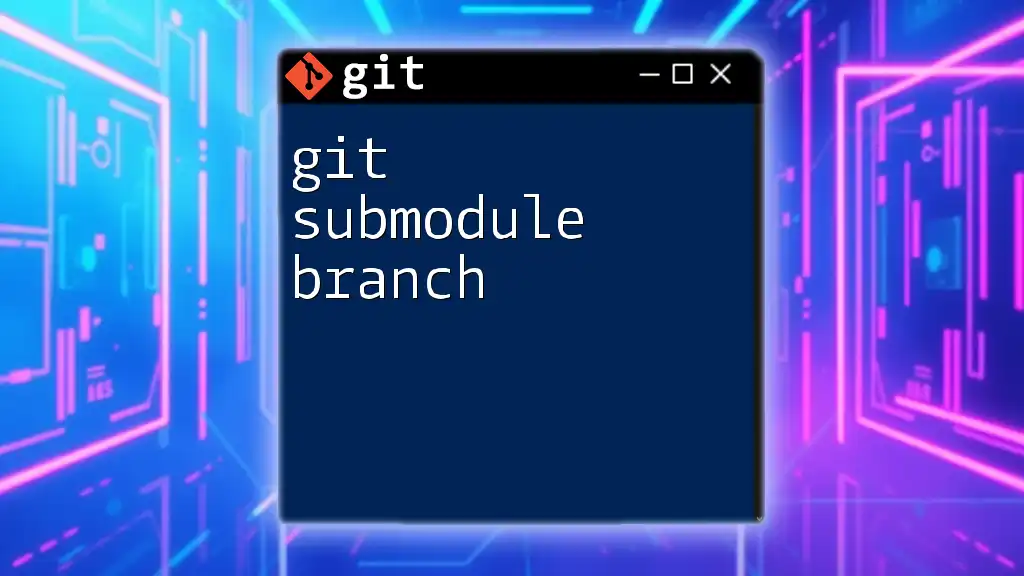
Alternatives to Deleting Submodules
If you're thinking about removing a submodule, consider some alternatives first:
- Temporary Deactivation: Instead of a complete deletion, you could temporarily deactivate the submodule. This allows you to keep the code but not have it included in the project for the time being.
- Refactoring for Better Dependency Management: Depending on your requirements, you might want to refactor your code to manage dependencies differently. Instead of using a submodule, consider using package managers or other dependency management strategies.
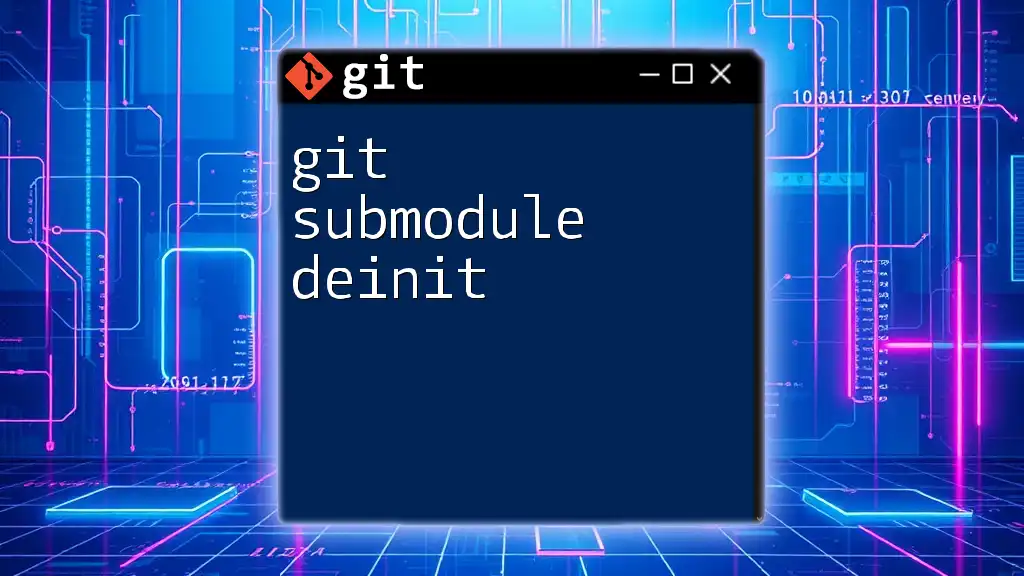
Conclusion
Deleting a Git submodule doesn't have to be a convoluted process. By following the steps outlined above, you can ensure a smooth and clean removal. Remember the importance of backing up code and understanding the impact of your changes. Submodules can add complexity, but being well-versed in their management can help you navigate your projects more effectively. Be encouraged to explore and experiment further with Git as your projects evolve!
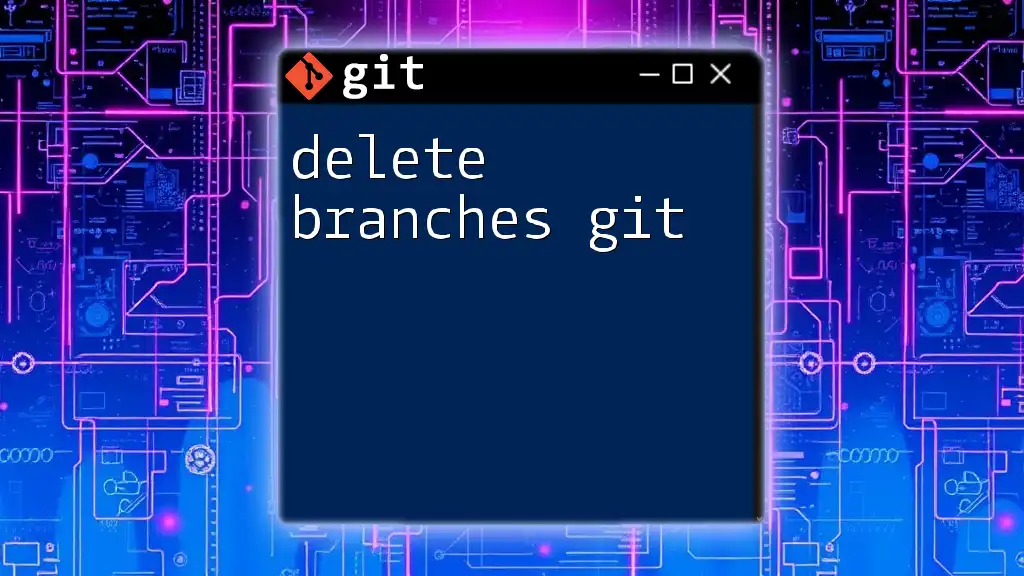
Additional Resources
For more in-depth understanding and examples, consider referring to the official Git documentation, recommended tutorials, or community forums. Having these resources at hand can further boost your proficiency with Git commands.