To move a tag in Git, you can delete the old tag and then create a new tag at the desired commit location.
git tag -d <old-tag-name> && git tag <new-tag-name> <commit-hash>
Understanding Git Tags
What are Tags?
Tags in Git are critical for marking specific points in the project’s history, often representing major releases or significant milestones. A tag acts like a snapshot of a specific commit, allowing developers to easily reference such points later.
There are two primary types of tags in Git:
-
Lightweight Tags: These are like bookmarks to a specific commit. They do not store additional information, just the commit hash.
-
Annotated Tags: More substantial than lightweight tags, annotated tags are stored as full objects in the Git database. They include the tagger's name, email, date, and an optional tagging message, giving context to the tag.
Why Use Tags?
Using tags is pivotal in version control for several reasons:
-
Versioning: They provide an easy way to track version numbers for software releases.
-
Release Management: Tags serve as references for deployments, enabling easy rollbacks to stable versions if issues arise.
-
Milestone Identification: Tags can mark important milestones in a project, providing clear demarcation of progress.
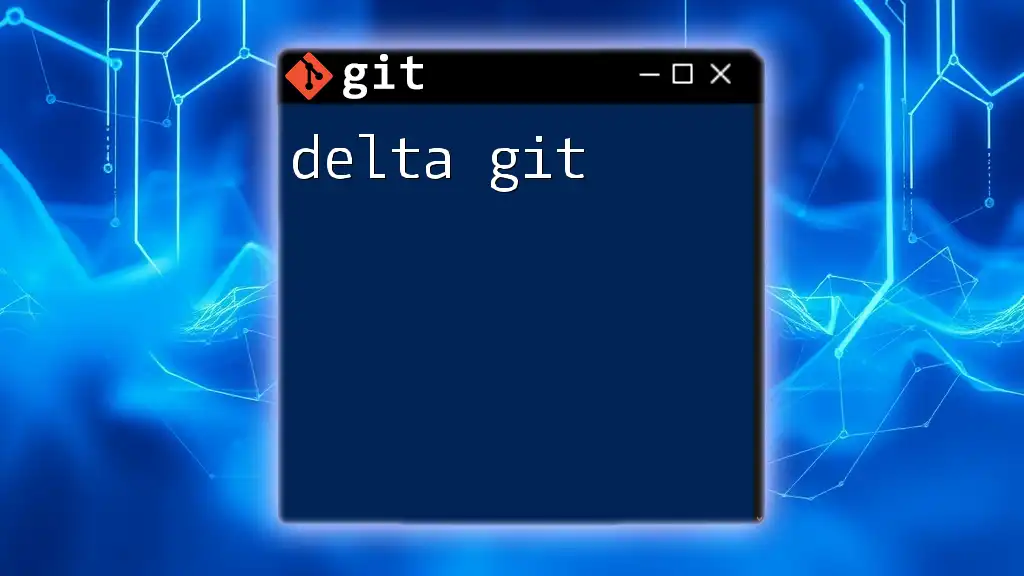
Moving a Tag in Git
When to Move a Tag
Moving a tag may be necessary in various scenarios. Common situations include pointing a tag to the correct commit if it was mistakenly tagged, or after a rebase or merge when the earlier tagged commit is no longer relevant. It is essential to recognize when a tag needs adjustment to maintain the integrity of your project history.
Moving a Local Tag
Command to Move a Tag
To move a tag to a new commit locally, you utilize the `git tag` command with the force option. The syntax for this command is:
git tag -f <tag_name> <new_commit_hash>
In this command:
- `-f` stands for force, indicating that you want to overwrite the existing tag.
- `<tag_name>` should be replaced with the name of the tag you want to move.
- `<new_commit_hash>` is the commit hash where you want the tag to point.
Verifying the Move
After moving the tag, it’s vital to confirm the changes. You can check the tag's new position with:
git show <tag_name>
The output will display details of the commit the tag now points to, confirming the move was successful.
Moving a Remote Tag
Deleting the Old Remote Tag
Once you’ve moved the local tag, you need to update the remote repository. The first step is to delete the old tag from the remote:
git push --delete origin <tag_name>
This command is crucial as it ensures that the remote repository reflects the changes you’ve made locally.
Pushing the New Tag
With the old tag deleted, you can now push the newly moved tag to the remote repository using:
git push origin <tag_name>
This updates the remote repository with the correct reference, solidifying the move of the tag across both local and remote environments.
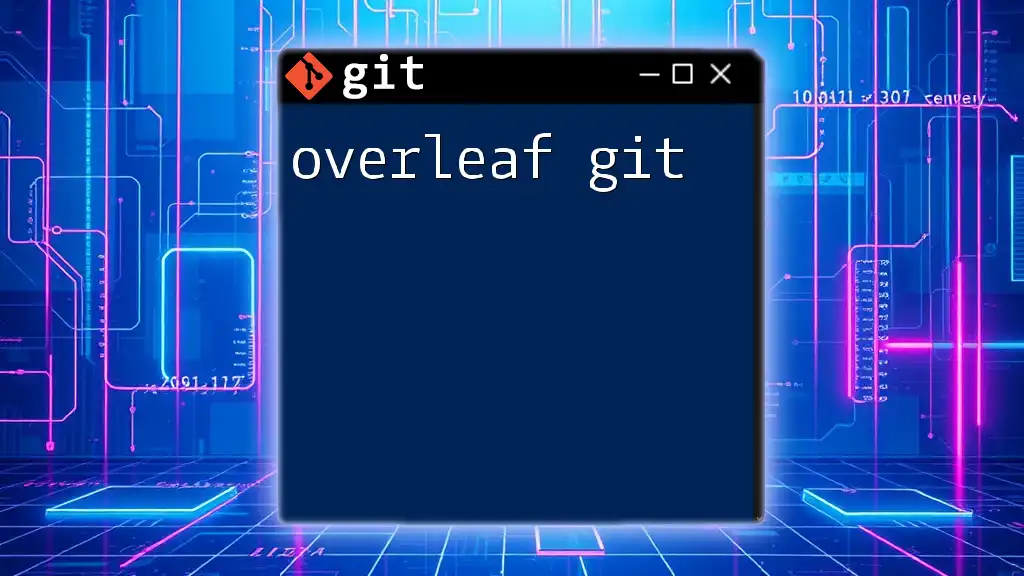
Practical Examples
Example Scenario: Moving a Release Tag
Consider a scenario where a tag named `v1.0` was mistakenly placed on the wrong commit. You need to point it to the correct commit:
-
Identify the commit hash for the correct commit.
-
Move the tag locally with the following command:
git tag -f v1.0 <correct_commit_hash>
-
Remove the erroneous tag from the remote:
git push --delete origin v1.0
-
Push the corrected tag to the remote:
git push origin v1.0
Following these steps ensures that your tagging system remains accurate and useful.
Example Scenario: Correcting a Typo in Tag Name
Sometimes, you might need to correct a typo in a tag name, for instance, changing `v1.0` to `v1.0-beta`.
-
Move the tag to the desired commit:
git tag -f v1.0-beta <commit_hash>
-
Delete the old tag from the remote:
git push --delete origin v1.0
-
Push the corrected tag to the remote:
git push origin v1.0-beta
These steps ensure that your tagging convention remains clear and recognizable.
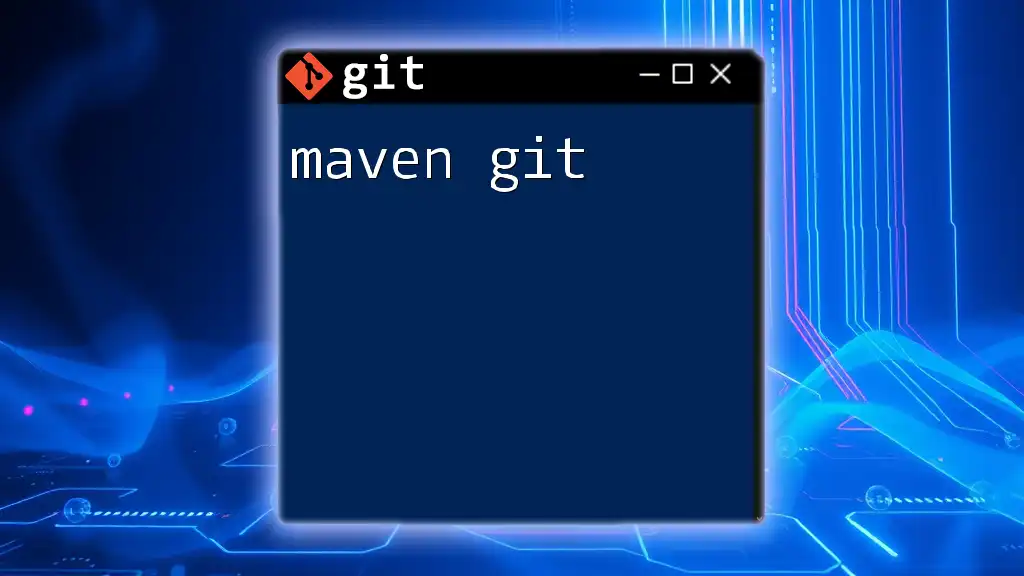
Tips and Best Practices
Best Practices for Tagging in Git
To maintain an efficient tagging system, update documentation regularly to outline the significance of each tag. Additionally, establish a consistent naming convention to avoid confusion in the future.
Managing Tags Effectively
Adopt tagging strategies such as semantic versioning (e.g., v1.0.0, v1.0.1) to help categorize the nature of changes (major, minor, patch). This practice aids others in understanding the evolution of the project intuitively.
Cautionary Notes
Before moving tags, it’s crucial to communicate with your team. Ensure that others are aware of the changes, as tags can often be relied upon in collaborative environments. Be cautious when using the `-f` option; it carries a risk of confusion if not properly documented.
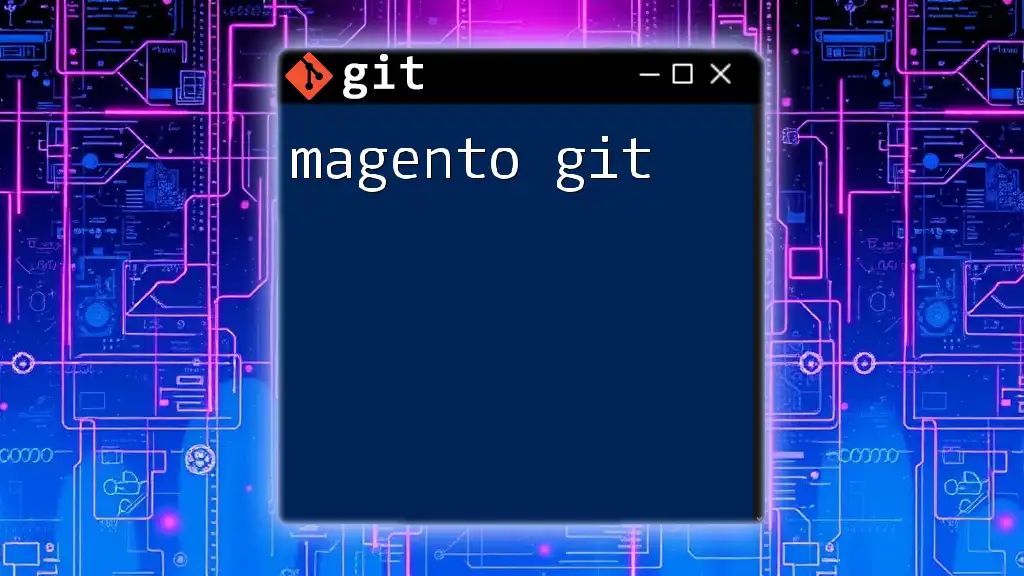
Conclusion
Understanding how to move a tag in Git enhances your version control capabilities. By keeping your tags accurate and reflective of your project history, you foster a more robust development environment. With careful management and regular practice, you can master the art of tagging in Git and improve your overall workflow efficiency.
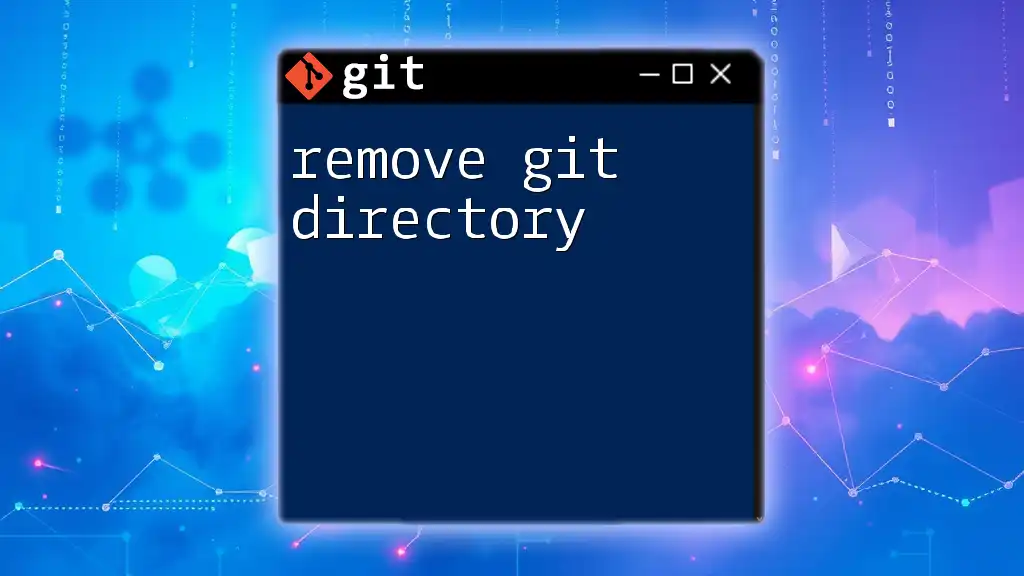
Additional Resources
For further reading, consult the official Git documentation on tags, which can provide deeper insights into tag management. Explore advanced Git usage to refine your skills even more.
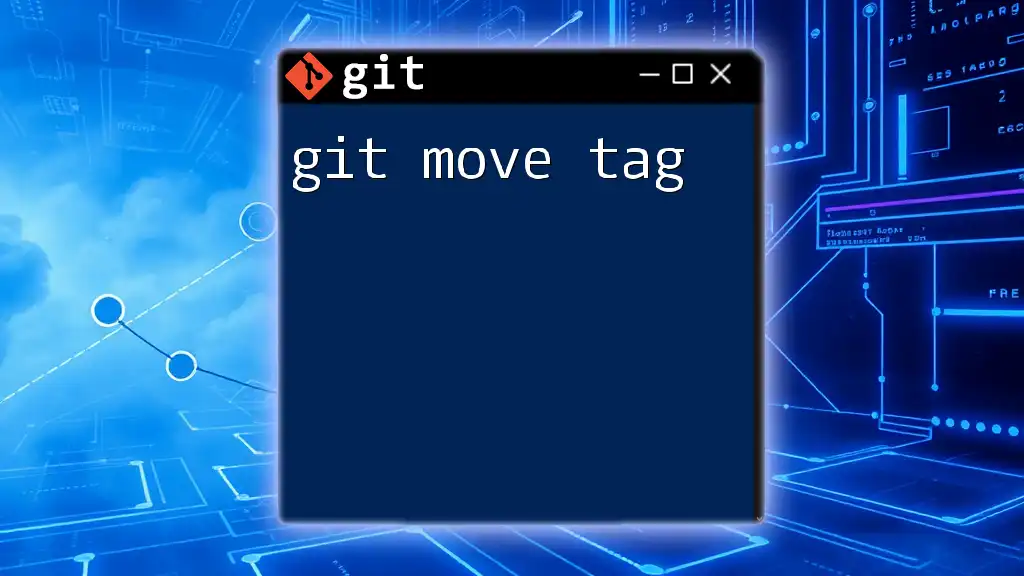
Call to Action
Now that you’re equipped with the knowledge to move tags effectively, try it in your projects. Share your experiences and questions with the community to deepen your understanding and foster collaborative learning.