To remove a tag in Git, use the command `git tag -d <tagname>` to delete a local tag and `git push --delete origin <tagname>` to remove it from the remote repository.
git tag -d <tagname> # Remove local tag
git push --delete origin <tagname> # Remove tag from remote
Understanding Git Tags
What are Git Tags?
Git tags are a powerful feature in Git that allow you to mark specific points in your repository's history. Tags can be meaningful checkpoints in your project's development, commonly used for marking release points (like v1.0, v2.0, etc.). There are two main types of tags:
-
Lightweight Tags: These are essentially bookmarks to a specific commit. They do not include additional metadata, making them simple and quick to create.
-
Annotated Tags: These are more comprehensive and include information such as the tagger's name, email, date, and can also contain a tagging message. Annotated tags are stored as full objects in the Git database.
Git tags are especially valuable when you want to label a version of your software that you may want to refer back to later, such as for releases.
When to Remove a Git Tag
There are several situations where you might want to remove a tag:
- Incorrect Tags: If a tag was applied erroneously to the wrong commit, it makes sense to remove it.
- Repository Reset: You may need to delete tags if you’re performing a reset or if your project undergoes significant changes.
- Tag Redundancy: Over time, you might find that certain tags are no longer relevant, requiring cleanup for clarity.
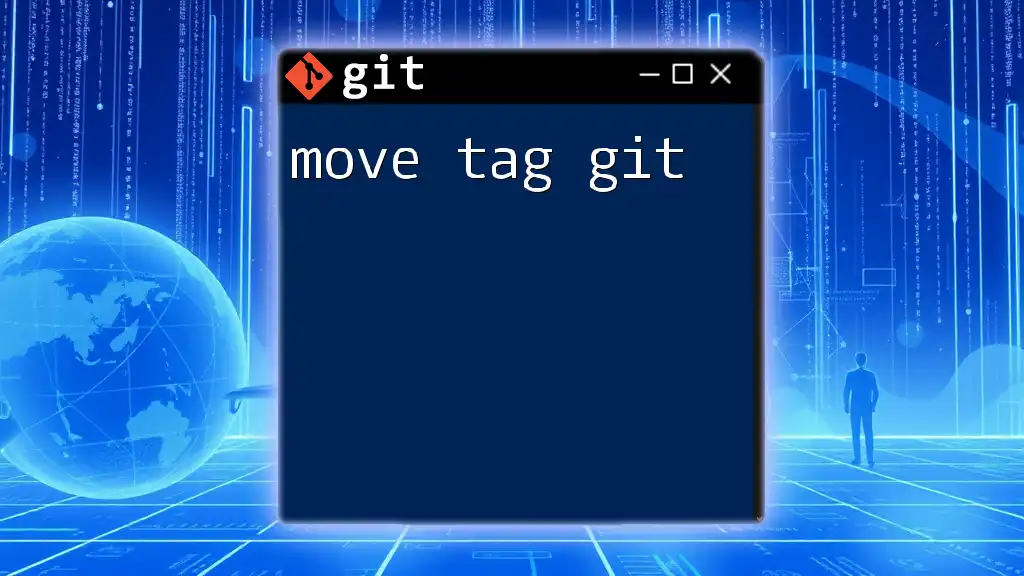
How to Remove a Local Git Tag
Using Git Command Line
Removing a tag in your local repository is straightforward. You will use the `git tag -d <tag_name>` command.
Command:
git tag -d <tag_name>
Example:
git tag -d v1.0
In this example, the tag `v1.0` is being deleted from your local Git repository.
Confirming the Removal of the Local Tag
To ensure the tag has been successfully removed, you can list all the tags with the following command:
Command:
git tag
After executing this command, you should see a list of all remaining tags. If `v1.0` is no longer listed, it has been successfully removed.
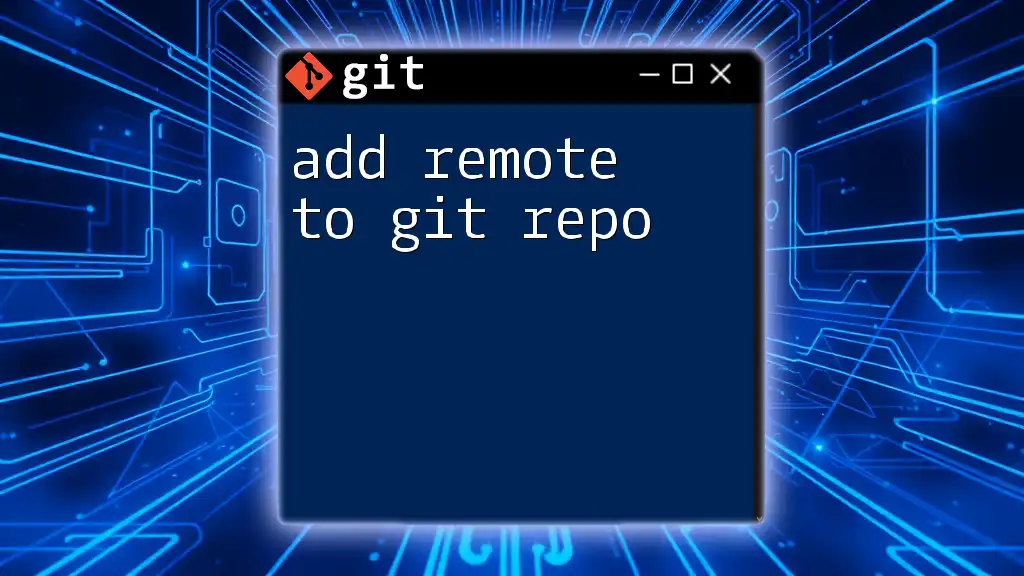
How to Remove a Remote Git Tag
Understanding Remote Repositories
When working with Git, it's crucial to understand the distinction between local and remote repositories. Local tags only exist in your local copy of the repository, while remote tags exist on a shared server, such as GitHub or GitLab.
Steps to Remove a Remote Tag
To remove a tag from a remote repository, you use the command:
Command:
git push --delete <remote_name> <tag_name>
Example:
git push origin --delete v1.0
This command deletes the `v1.0` tag from the `origin` remote repository.
Confirming the Removal of the Remote Tag
To ensure the tag has been deleted from the remote, you can list the tags available on the remote repository:
Command:
git ls-remote --tags <remote_name>
Example:
git ls-remote --tags origin
After executing this command, verify that `v1.0` is no longer listed among the tags. This confirms that the tag has been successfully removed.
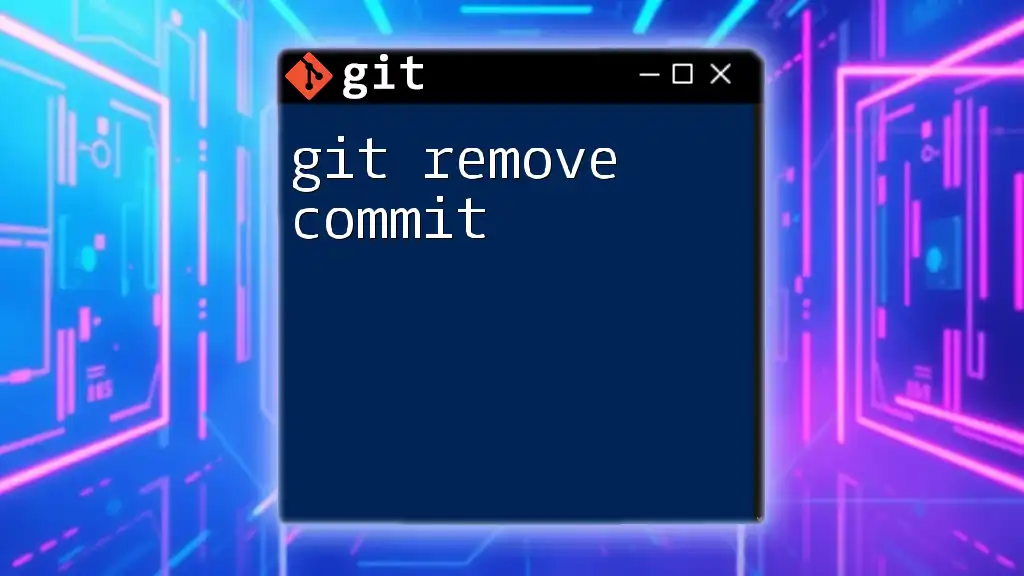
Best Practices for Tag Management
Naming Conventions for Tags
Using a consistent naming convention for your tags can greatly enhance understandability and maintainability. A few best practices include:
- Start with a version number (e.g., `v1.0`, `v1.1`, etc.).
- Use semantic versioning to indicate significant changes (e.g., `v1.0.0` for a major release).
- Avoid special characters that might confuse others interpreting the tags.
Keeping Your Repository Clean
Maintaining a clean repository is essential for long-term project success. Regularly check for unused or obsolete tags and remove them to reduce clutter. Automating tag management tasks or using scripts can help streamline this process.
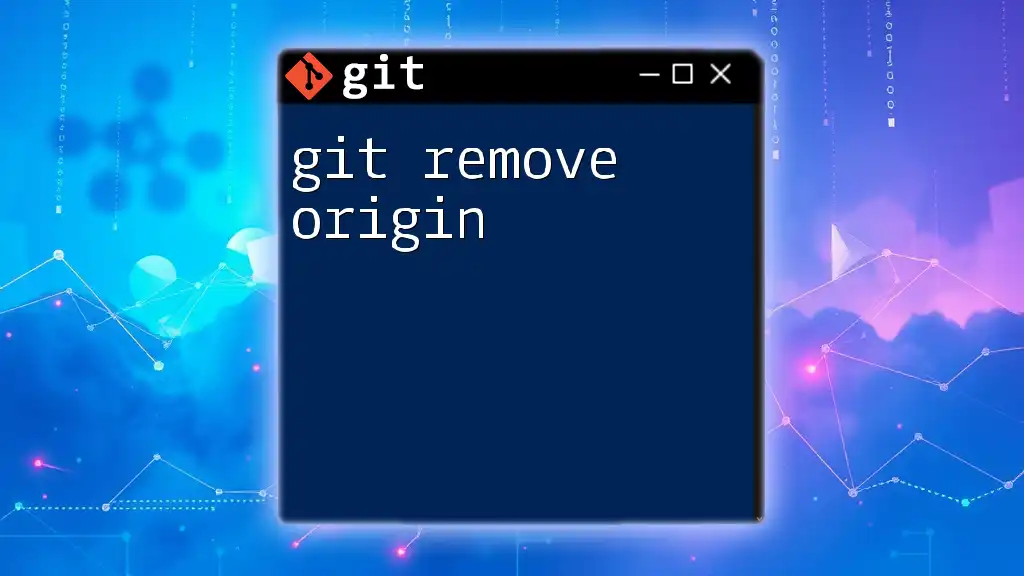
Troubleshooting Common Issues
Can't Find the Tag to Delete
If you are unable to locate a tag you intend to delete, you can list all tags in your repository using:
Command:
git tag -l
This command lists all tags, helping you find the tag's exact name for deletion.
Issues with Remote Tag Deletion
If you encounter issues when removing a tag from a remote repository, here are steps to troubleshoot:
- Ensure you are connected to the correct remote repository.
- Double-check that you have the necessary permissions to delete tags.
- Verify the tag name and syntax in your command for any mistakes.
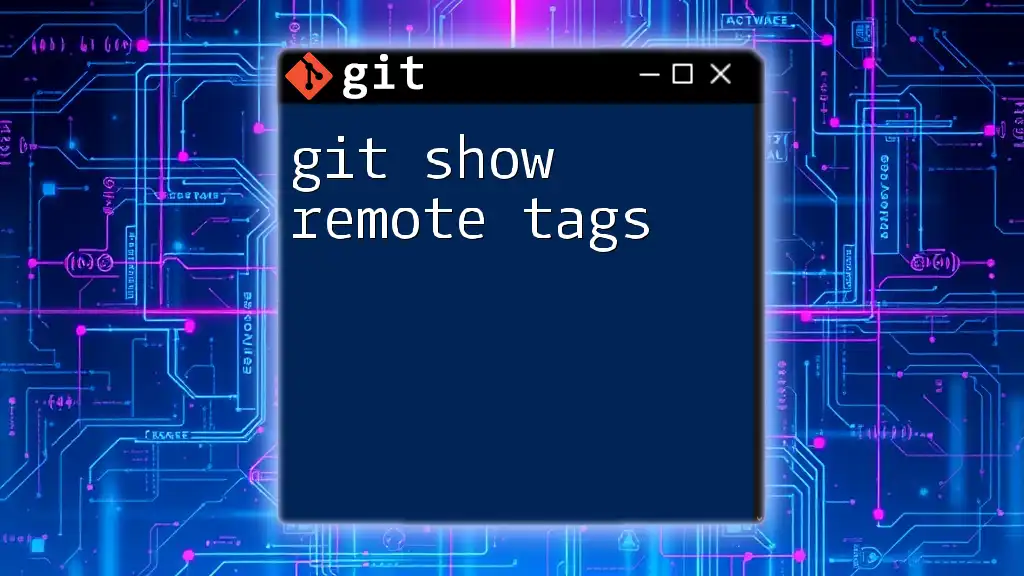
Conclusion
Understanding how to manage and remove tags effectively is an important skill for any Git user. By following the guidelines outlined in this article, you can confidently handle the tagging process, ensuring your versioning system remains clear and efficient.
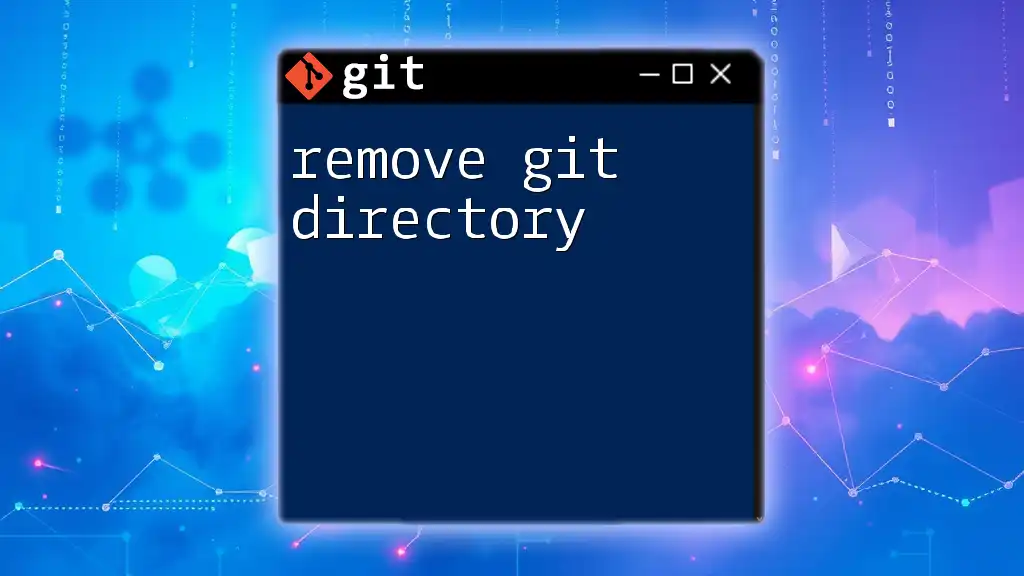
Call to Action
For more tutorials on Git commands and best practices, subscribe to our mailing list. As a bonus, get a free downloadable cheat sheet for managing Git tags to make your version control experience even smoother!
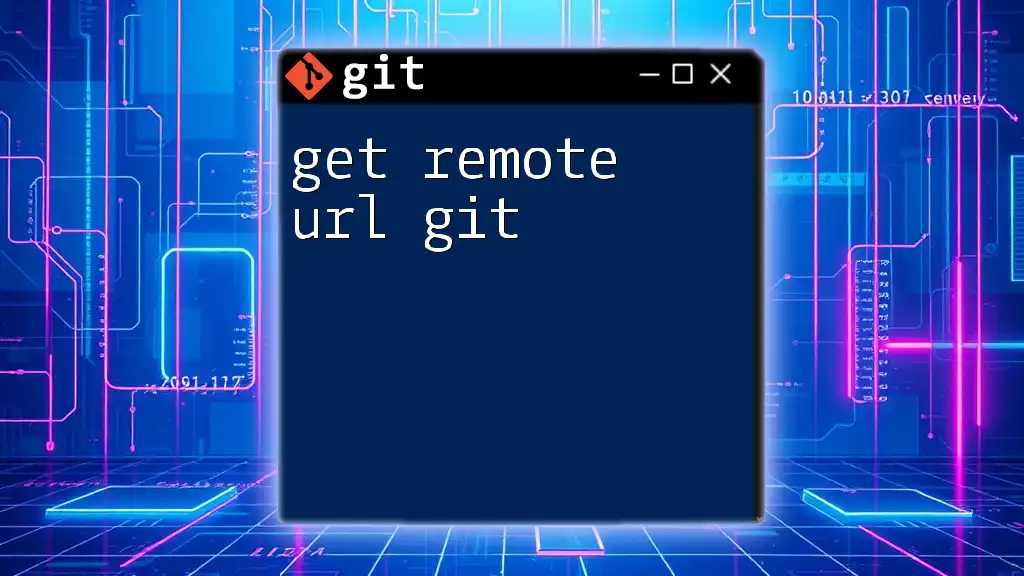
Additional Resources
For further exploration on tags in Git, check out the official Git documentation and suggested articles that dive deeper into Git best practices.