To retrieve the remote URL of your Git repository, you can use the following command:
git remote get-url origin
Understanding Remote URLs in Git
What is a Remote URL?
A remote URL refers to the web address that serves as a pointer to a remote Git repository. This URL allows you to interact with repositories hosted on platforms such as GitHub, GitLab, and Bitbucket. Understanding remote URLs is crucial as they are essential for sharing code and facilitating collaboration in a team environment.
Types of Remote URLs
SSH URLs are one of the two primary types of remote URLs. They use Secure Shell (SSH) protocol to provide a secure connection between your local machine and the remote Git server. The key benefits of SSH include:
- Enhanced Security: Communication is encrypted, making it difficult for unauthorized users to intercept data.
- Convenience: Once set up, you can push and pull without having to enter your username and password each time.
For instance, an SSH URL looks like this:
git@github.com:username/repository.git
On the other hand, HTTPS URLs are easier to use for beginners and are particularly popular due to their simplicity. With HTTPS, you authenticate using a username and password (or personal access tokens). The main advantages include:
- User-Friendly: Great for those who might not be familiar with SSH.
- Firewall Compatibility: Works better with restrictive firewall settings.
An example of an HTTPS URL is:
https://github.com/username/repository.git
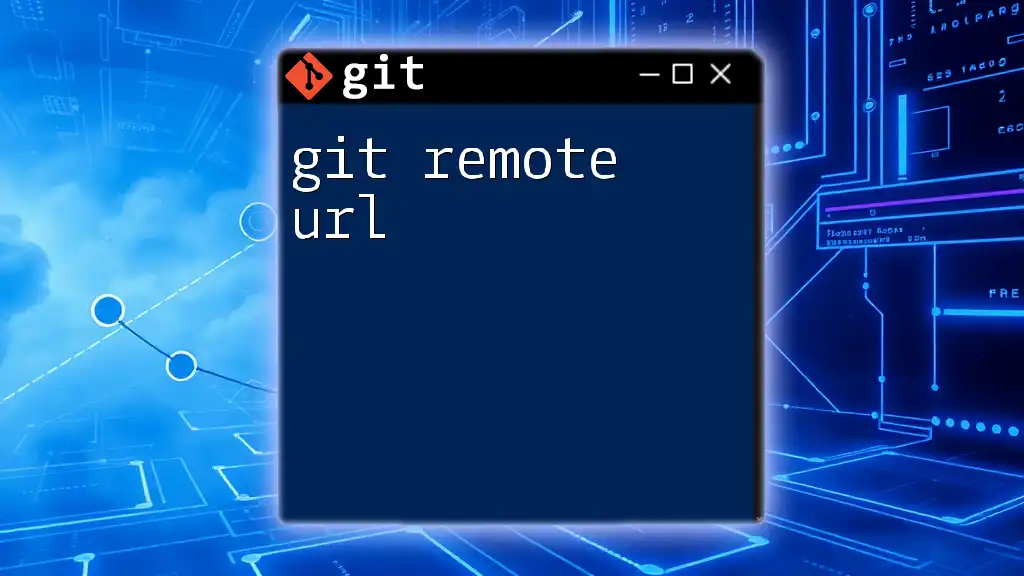
How to Get the Remote URL of a Git Repository
Checking Existing Remote URLs
To check the existing remote URLs associated with your local repository, simply run the following command in your terminal:
git remote -v
This command will provide output indicating the names of remotes (commonly `origin`), along with their fetch and push URLs. For instance:
origin https://github.com/username/repository.git (fetch)
origin https://github.com/username/repository.git (push)
Here, both fetch and push commands will target the same URL, simplifying operations.
Adding a New Remote URL
If you need to add a remote URL, you can do so using the following command:
git remote add origin <remote_url>
Replace `<remote_url>` with the actual URL of your Git repository. For example, adding a GitHub repository would look like this:
git remote add origin https://github.com/username/repository.git
This command establishes a link between your local repository and the specified remote repository, typically with the default name `origin`, which is a convention used in Git.
Changing an Existing Remote URL
At times, you may need to change an existing remote URL, perhaps because you’ve migrated your repository or switched from HTTPS to SSH. You can update it using the following command:
git remote set-url origin <new_remote_url>
For example, if you switch to using SSH for a repository previously set with HTTPS:
git remote set-url origin git@github.com:username/repository.git
This command allows your existing `origin` to point to a new remote URL, ensuring that all subsequent Git operations use the updated URL.
Removing a Remote URL
In some cases, you might find it necessary to remove a remote URL. This can occur if it is no longer relevant or you want to start fresh. You can remove a remote URL with:
git remote remove origin
This command deletes the remote repository link named `origin`, allowing you to tidy up your project and make sure that irrelevant remotes are not cluttering your settings.
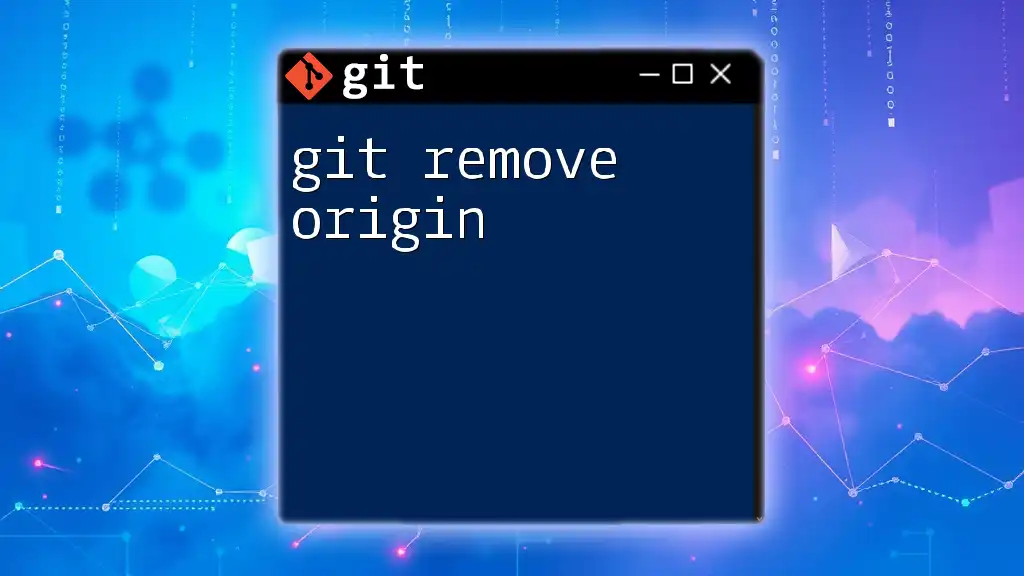
Troubleshooting Common Remote URL Issues
Remote Repository Not Found
Encountering a "repository not found" error usually indicates that the URL is incorrect or that you don’t have access to the repository. Check the spelling of the URL, ensure that you are authorized to access it, and confirm that the repository exists in the specified location.
Authentication Errors
Authentication issues generally arise when your credentials are incorrect. If you’re using HTTPS, double-check that your username and password (or personal access token) are accurate. If using SSH, ensure that your SSH key is correctly set up and added to your Git hosting service, such as GitHub.
Verifying Remote Connections
To verify that your connection to a remote repository is functioning as expected, you can run:
git ls-remote <remote_url>
This command attempts to fetch references from the remote repository. If it fails, it may provide prompts to rectify connectivity issues, allowing you to troubleshoot effectively.
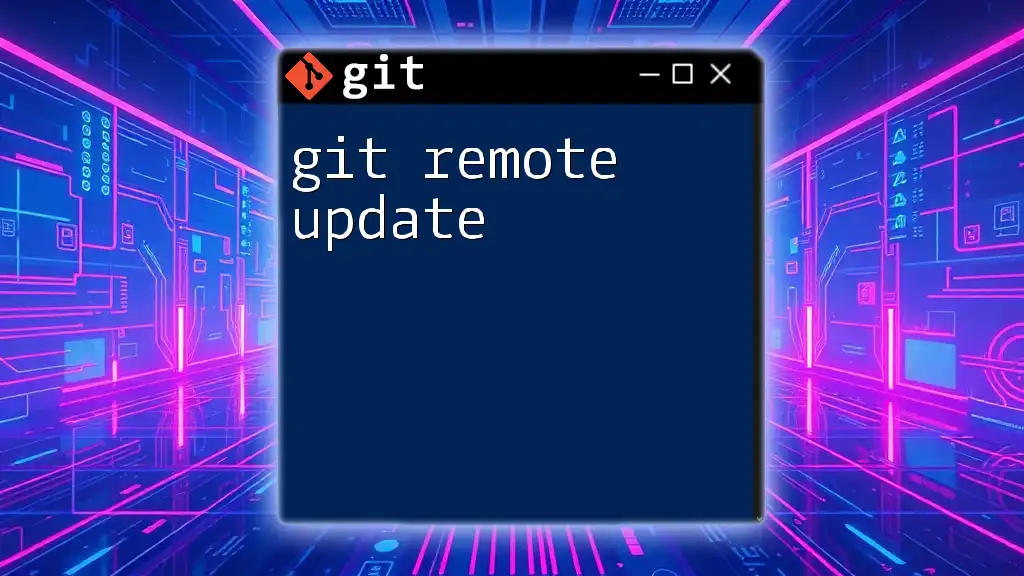
Best Practices for Managing Remote URLs
Use Meaningful Remote Names
When working with multiple remotes, consider naming them descriptively. While `origin` is the default name used for the primary remote repository, you can title additional sources based on their purpose or relationship, such as `upstream` for the original repository of a fork.
Regularly Check and Update Remote URLs
It's a good practice to regularly use `git remote -v` to confirm that your remotes are correctly set up. Keeping URLs updated is essential, especially in environments where repositories might migrate from one host to another or switch between HTTPS and SSH.
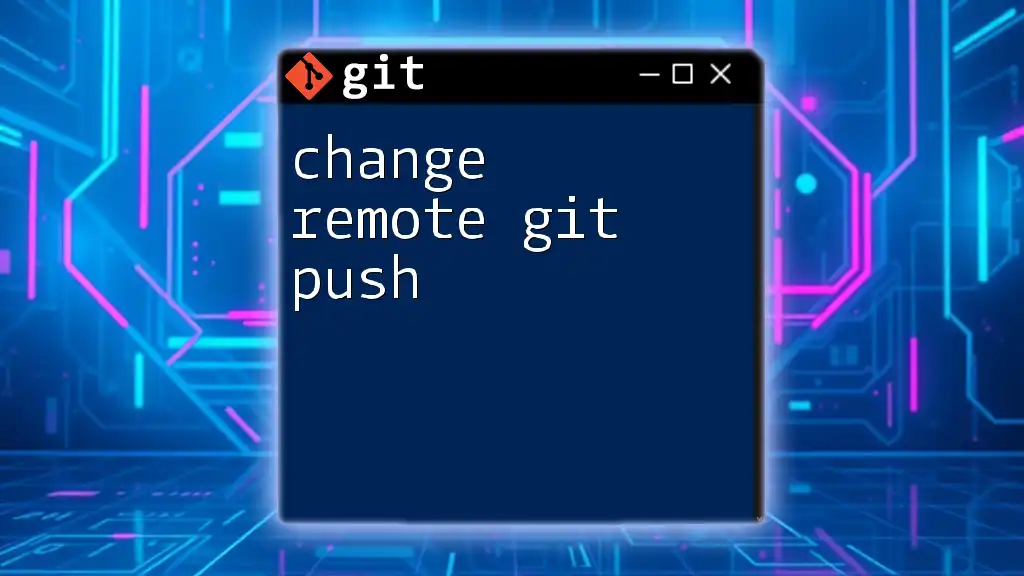
Conclusion
Knowing how to get remote URLs in Git is essential for effective collaboration and management of your projects. By understanding how to check, update, add, and remove remote URLs, you empower yourself to navigate shared repositories efficiently. Practice using the commands provided, and don’t hesitate to explore further as you delve deeper into the world of Git.