To change the remote repository that your local Git repository pushes to, you can use the `git remote set-url` command followed by the name of the remote (usually `origin`) and the new repository URL.
git remote set-url origin https://github.com/username/new-repo.git
What is a Remote Repository?
A remote repository in Git is essentially a version of your project that is hosted on the internet or a network. It serves as a central hub where developers can share their contributions, collaborate on code, and manage different project versions. Common hosting platforms for remote repositories include GitHub, GitLab, and Bitbucket.
Understanding remote repositories is crucial, especially for collaborative development, as it enables multiple team members to work on the same project seamlessly.
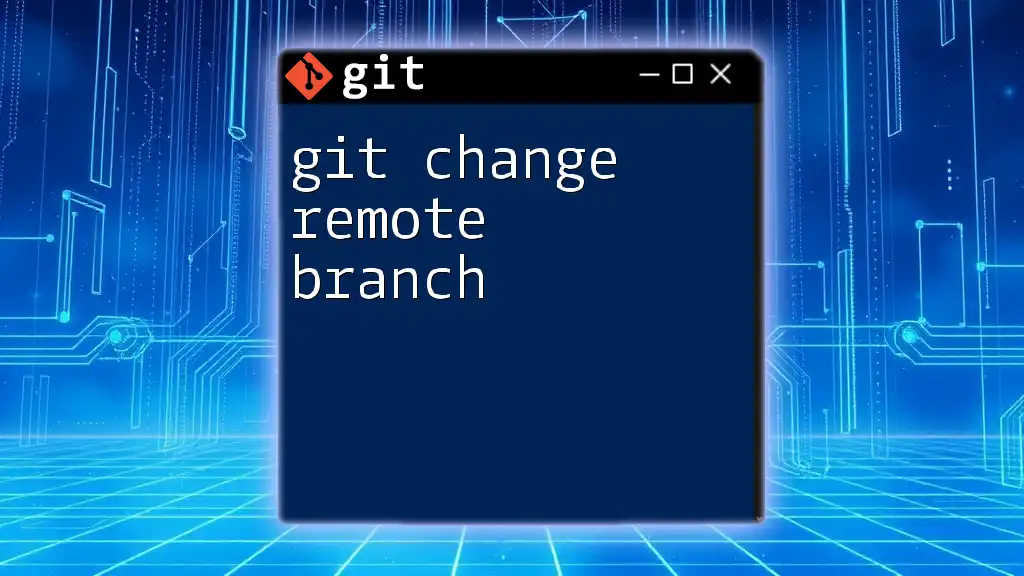
Understanding Git Push
The `git push` command is a fundamental operation in Git. It allows you to upload your local repository changes to a remote repository. When executing this command, Git transfers commits from your local repository to the specified remote branch.
A simple example of using `git push` would be:
git push origin main
In this example, `origin` represents the remote repository you’re pushing to, and `main` is the branch where the commits will be pushed. It’s essential to understand how `git push` works to leverage its functionality effectively.
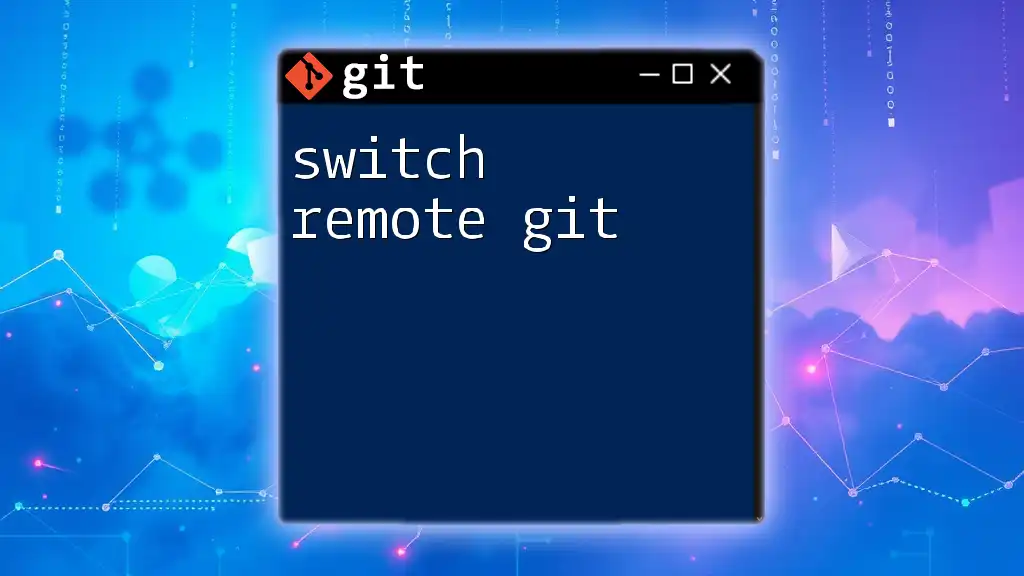
Why Change the Remote for Git Push?
There are various scenarios where changing the remote for a `git push` operation is necessary.
- Migration: If you decide to switch from one hosting service to another (e.g., GitHub to GitLab), you will need to update the remote URL.
- Collaboration: If you’re working on different projects or teaming up with different developers, you may need to push to a new remote entirely.
Changing the remote URL offers seamless collaboration, making it easy to direct your `git push` commands to the right repository.
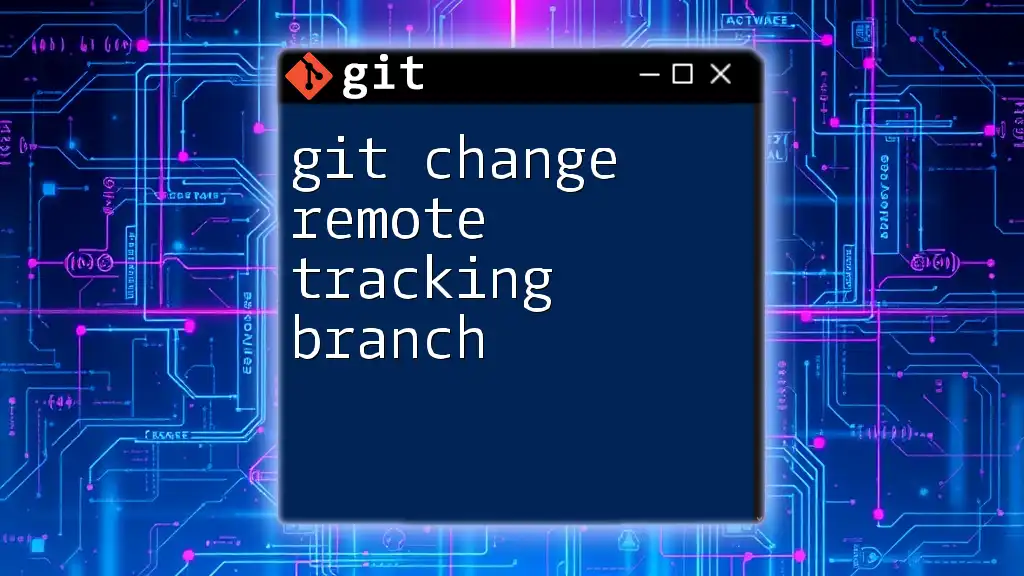
How to Check Current Remote Configuration
Before changing a remote, it’s crucial to have a clear view of your current remote setup. You can do this by running:
git remote -v
The output will display all configured remotes:
origin https://github.com/username/repo.git (fetch)
origin https://github.com/username/repo.git (push)
This command lists the remotes associated with your local repository for both fetch and push operations.
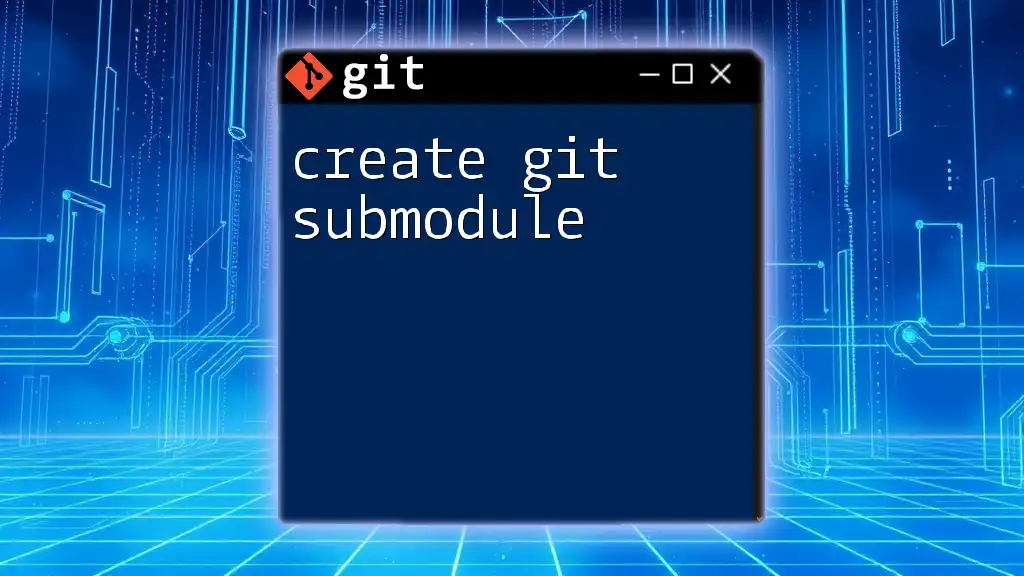
Changing the Remote URL
Updating the Existing Remote
If you need to change the URL of an existing remote (e.g., due to migration), you can do so using the `git remote set-url` command:
git remote set-url origin https://new-url.com/username/repo.git
In this command, `origin` is the name of the existing remote, and the second parameter is the new URL you want to set. This command updates the URL without needing to delete the remote.
Adding a New Remote
Alternatively, you may find it beneficial to add a new remote instead of changing the existing one. This approach is useful if you want to keep multiple remotes for different purposes. You can do this with the `git remote add` command:
git remote add new-origin https://new-url.com/username/repo.git
Here, `new-origin` is the name you’re assigning to your new remote. This allows you to easily push to multiple remotes if needed.
Verifying the Remote Change
After updating or adding a remote, it's essential to verify that the changes have been made correctly. Again, use:
git remote -v
The output should reflect the new URL you have set, ensuring that your configuration is accurate:
origin https://new-url.com/username/repo.git (fetch)
origin https://new-url.com/username/repo.git (push)
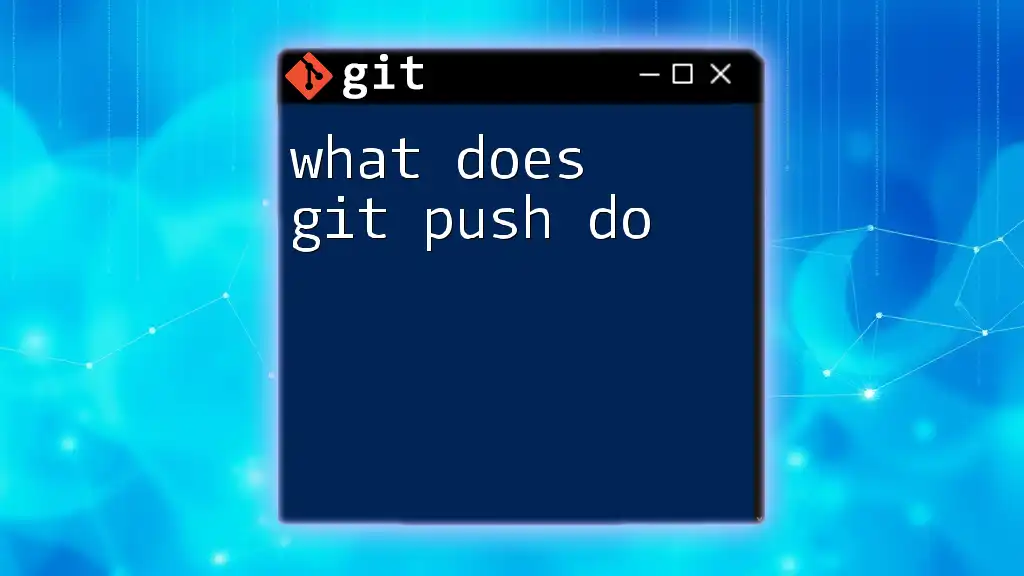
Pushing to the New Remote
Once you have updated the remote, you can proceed to push your changes. If you have set a new remote, use:
git push new-origin main
This command specifies that you want to push your changes to the `new-origin` remote’s `main` branch. It’s crucial to specify which remote you’re using to avoid any confusion, especially when managing multiple remotes.
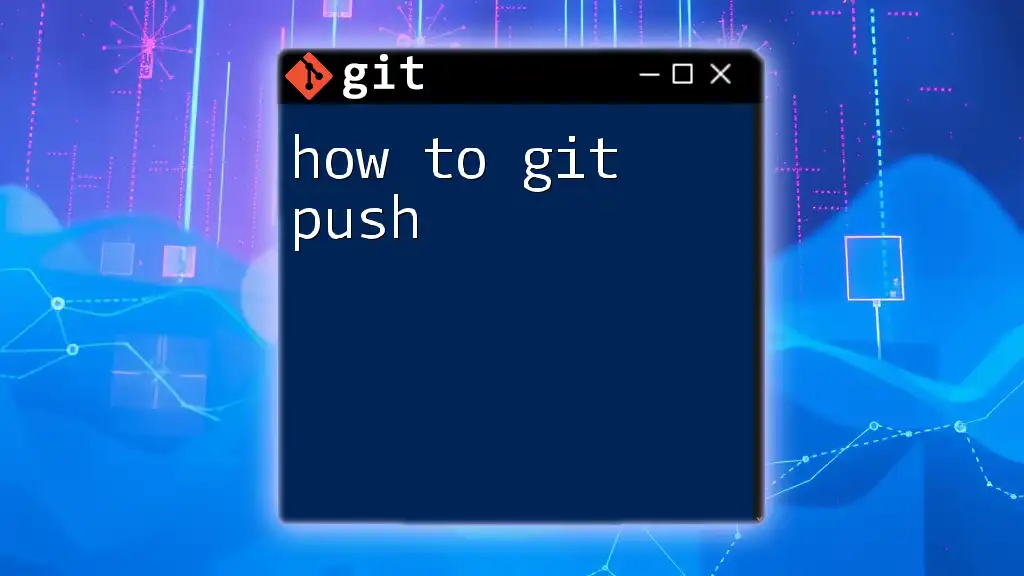
Handling Authentication Issues
When changing remotes, you might encounter authentication issues, especially if the new repository requires different credentials.
- For HTTPS remotes, ensure your credentials are updated in your credential manager or prompt.
- For SSH remotes, verify that your SSH key is appropriately set up and added to the new remote hosting account.
In case you experience permission issues, double-check that you have the correct access rights for the new remote repository.
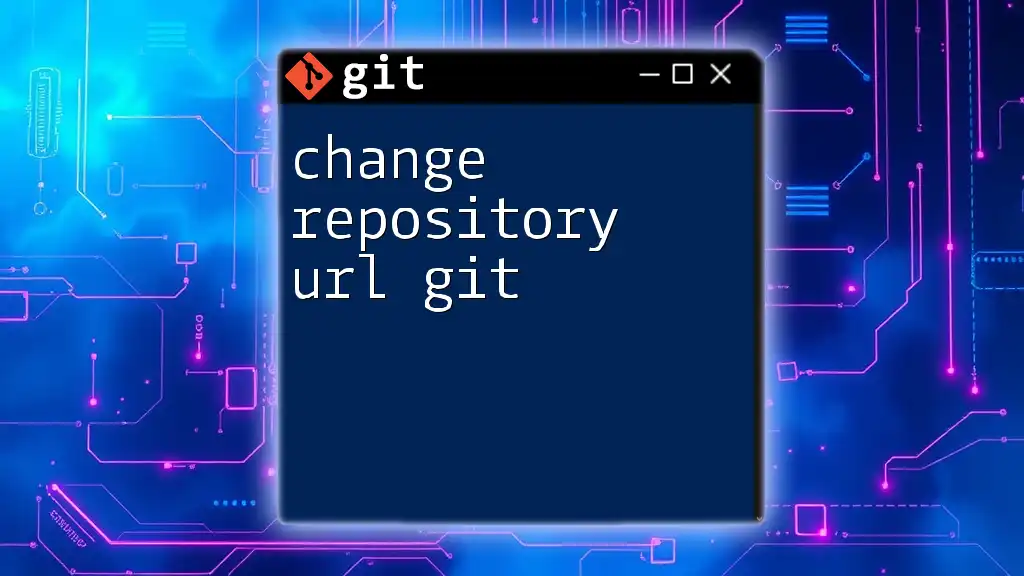
Best Practices for Managing Remotes
Managing remotes effectively is essential for a smooth workflow. Here are a few best practices:
- Use descriptive names for your remotes (e.g., `upstream`, `test`, `prod`) to indicate their purpose.
- Regularly update your remote URLs if changes occur in your hosting services.
- Keep a documentation file that outlines your remote configurations, making it easier for team members to understand the setup.
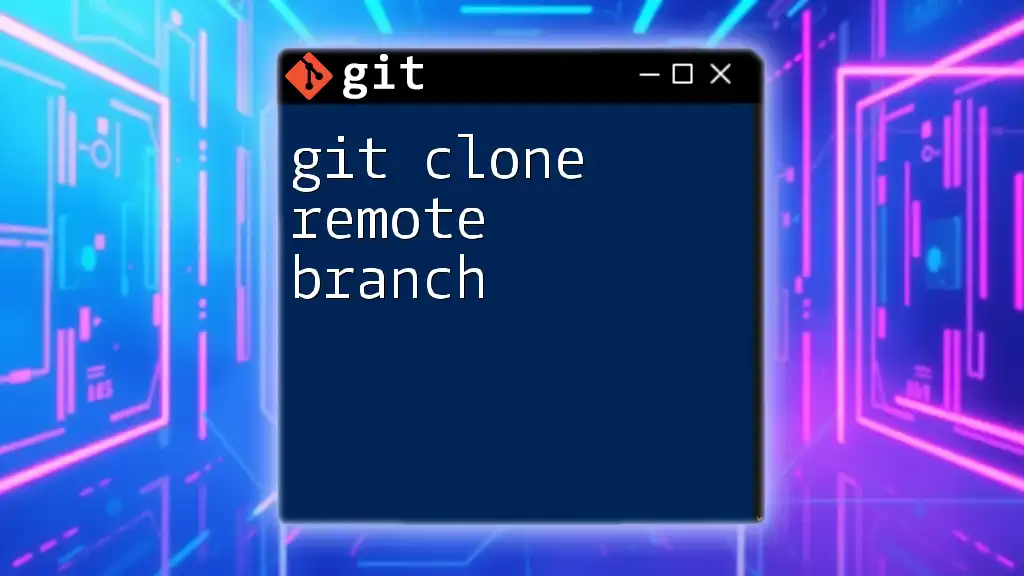
Common Errors and Troubleshooting
When changing a git remote, you may encounter some common issues:
- Remote does not exist: This occurs if you’ve accidentally misspelled the remote name or provided an incorrect URL. Double-check your command and the name.
- Permission denied: This usually happens when your credentials are incorrect or if you lack access. Make sure that you have the right permissions for the new remote.
Understanding these common errors will help you troubleshoot effectively and maintain your workflow.
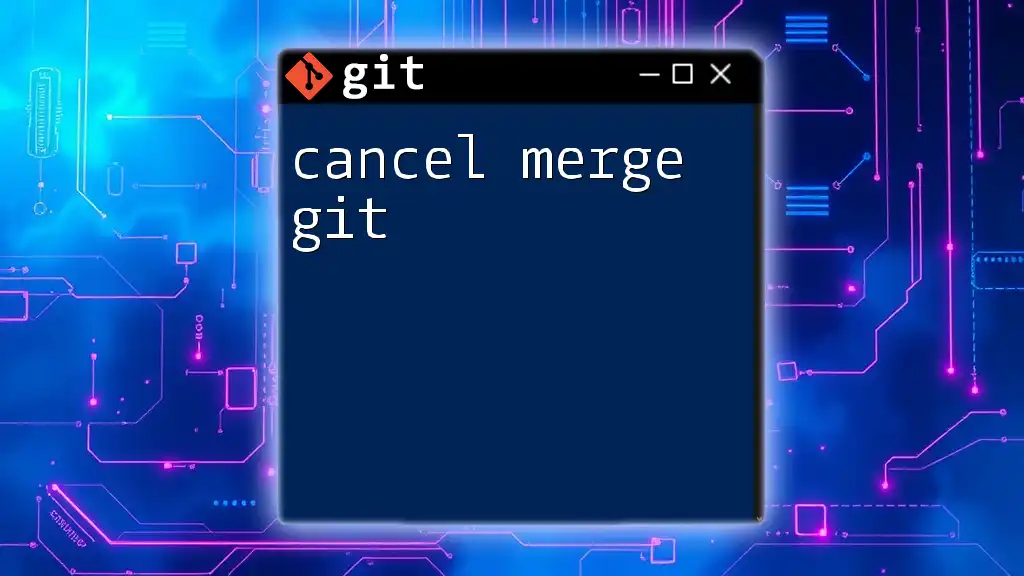
Conclusion
Knowing how to change the remote repository for `git push` operations is an essential skill for any developer. It enables you to adapt to changes quickly and collaborate with teams effectively. Practice the commands and concepts discussed in this article to enhance your Git proficiency.
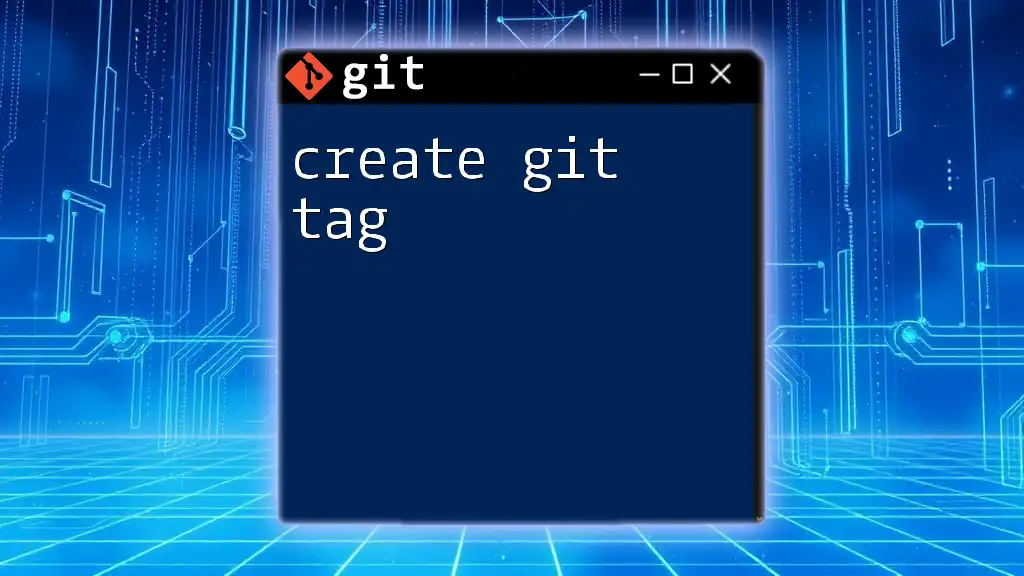
Additional Resources
For further learning, check out the official [Git documentation](https://git-scm.com/doc), explore various online tutorials, and consider joining forums for community support. Staying informed and engaged with the Git community will deepen your understanding and help you navigate any challenges effectively.
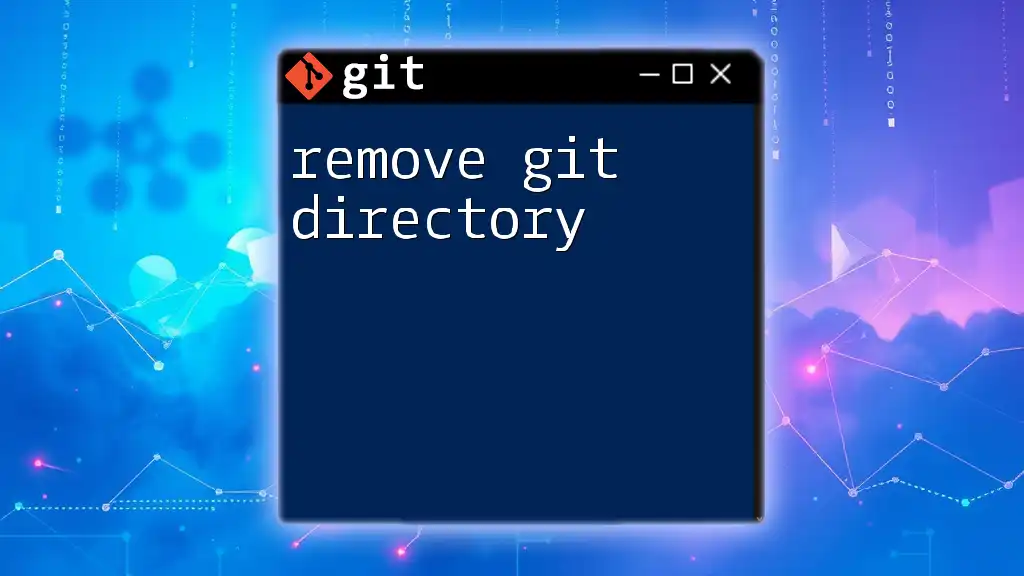
Call to Action
Join our community to receive more tips and concise guides on mastering Git commands efficiently!