The `git push` command is used to upload local repository changes to a remote repository, effectively syncing your local commits with the remote branch.
Here’s a code snippet demonstrating a basic usage of `git push`:
git push origin main
What is `git push`?
`git push` is a fundamental command in the Git version control system that allows you to upload your local repository changes to a remote repository. It plays a pivotal role in the overall Git workflow, facilitating collaboration among multiple developers and ensuring that everyone has access to the most recent codebase.
By executing `git push`, you send committed changes in your local branch to the specified remote branch, effectively enabling other team members to see and incorporate your updates. Understanding how `git push` operates is essential for maintaining seamless teamwork in software development.
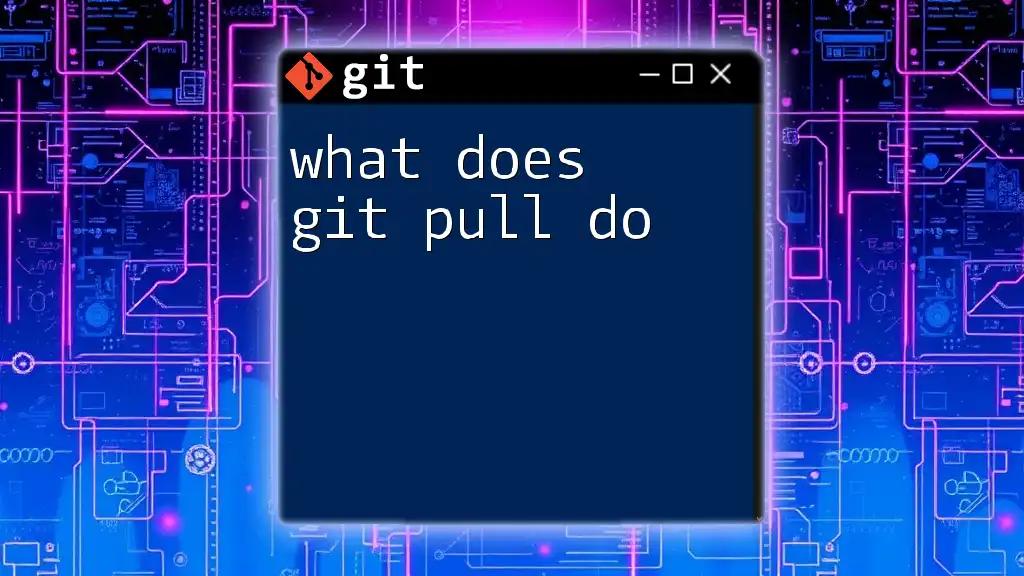
How `git push` Works
The Mechanics of `git push`
The basic syntax of the `git push` command is as follows:
git push [remote] [branch]
- `remote`: This refers to the remote repository you are pushing changes to (such as `origin`, which is the default name for the repository you cloned).
- `branch`: This is the name of the branch containing the changes you want to push.
Here’s a common use case:
git push origin main
In this example, you are pushing the commits from your local `main` branch to the `main` branch on the `origin` remote repository. This command updates the remote repository to reflect the latest commits made locally.
How `git push` Communicates with Remote Repositories
In Git, a Remote Repository refers to a version of your project that is hosted on the internet or a network. When you use `git push`, Git compares your local branch with its counterpart on the remote repository. If there are new commits in your local branch, `git push` sends only those updates to the remote repository, preventing unnecessary data transfers.
Upon successful execution, `git push` may output messages confirming the new commits that were added to the remote branch, making it clear what changes have been shared with your collaborators.
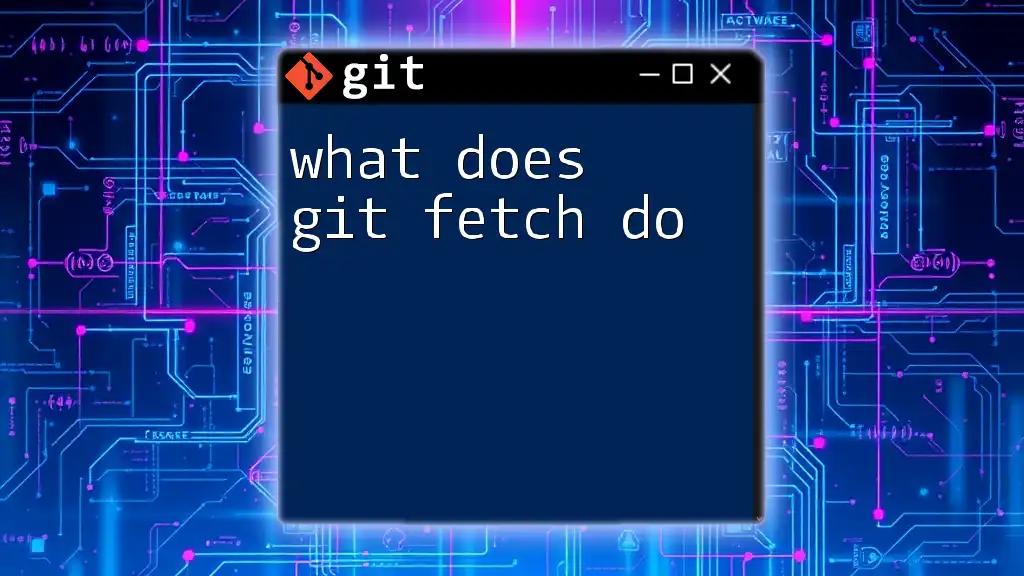
Understanding Different Scenarios of Using `git push`
Pushing to a New Remote Branch
When you create a new branch locally and want to push it to the remote repository for the first time, you can use the following command:
git push -u origin new-feature
The `-u` (or `--set-upstream`) flag establishes a tracking relationship between the local branch (`new-feature`) and the remote branch on the `origin`. This means that subsequent pushes and pulls can be done without having to specify the remote and branch names, making your workflow more streamlined.
Force Pushing with `git push --force`
Sometimes, you may need to overwrite the state of a remote branch, especially when your local branch includes changes that are not based on the latest commit of the remote branch. In such cases, you might use:
git push --force origin main
Caution: Force pushing can lead to the loss of data in the remote repository by deleting commits that others may rely on. It is usually considered a last resort. Always communicate with your team before using this command to avoid conflicts.
Pushing with Tags
Tags are useful for marking specific points in your code's history, such as release versions. To create and push a tag, you can use the following commands:
git tag v1.0
git push origin v1.0
Here, the first command creates a tag named `v1.0`, and the second command pushes it to the remote repository. Tags help you manage and reference particular versions of your code efficiently.
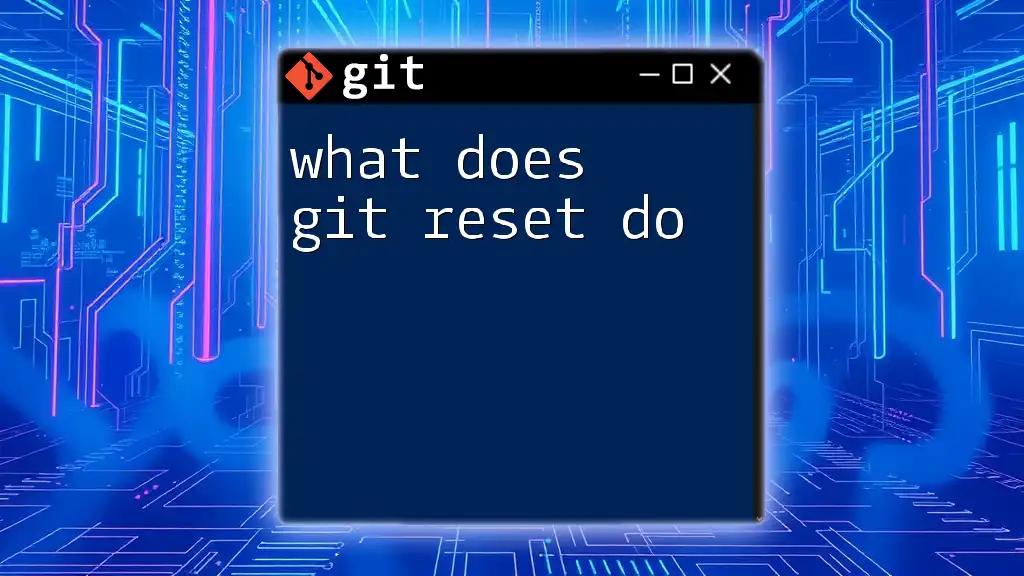
Common Issues and Troubleshooting with `git push`
Authentication Errors
Authentication errors usually occur when Git cannot verify your identity while trying to push changes to a remote repository. This can happen due to improper credentials or an unconfigured SSH key. Make sure to check the following:
- SSH vs. HTTPS: Ensure you are using the correct URL type for your repository. If you're using SSH, confirm that your SSH key is properly set up.
- Credentials: Double-check your username and password if you are using HTTPS.
Non-Fast-Forward Errors
`git push` will fail if the remote branch has commits that your local branch does not have. This is known as a non-fast-forward error. When this happens, Git prevents you from unintentionally overwriting those commits. You can resolve this by first pulling the changes:
git pull origin main
After merging the changes, you should be able to push again. In some scenarios, you may need to use force pushing, but remember this can potentially overwrite essential commits, so proceed with caution.
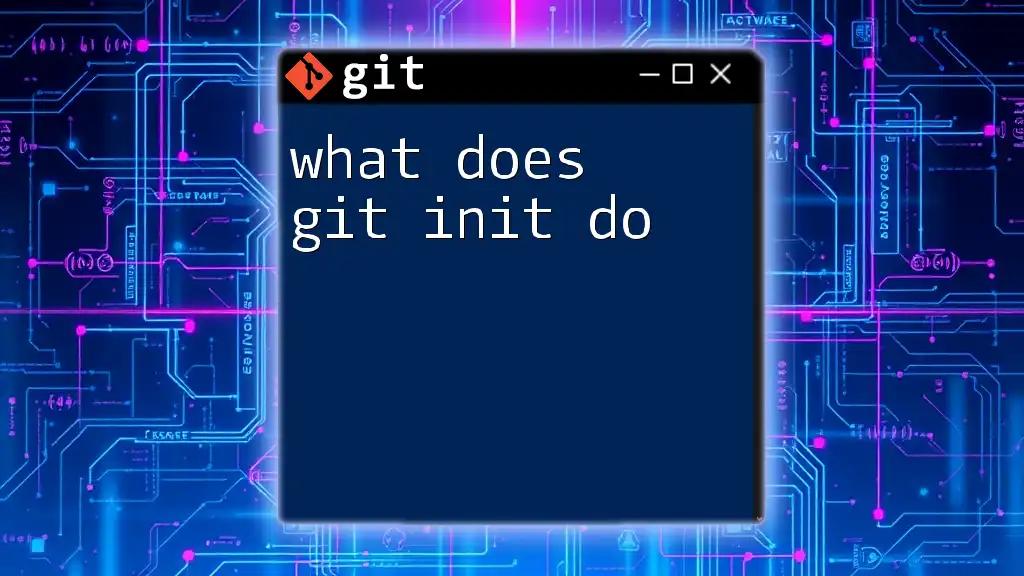
Best Practices for Using `git push`
Commit Frequently Before Pushing
When collaborating with other developers, committing frequently helps in maintaining a clean, understandable project history. Smaller, incremental commits make it easier to review changes and revert if necessary. Here's a streamlined workflow before pushing:
git add .
git commit -m "Clear commit message"
git push origin main
Collaborating with Team Members
Effective collaboration is essential in any software project. Always keep communication lines open with your team regarding changes you plan to push. Regularly pushing your updates ensures that everyone is aligned and working on the latest version of the code, reducing the chances of conflicts.
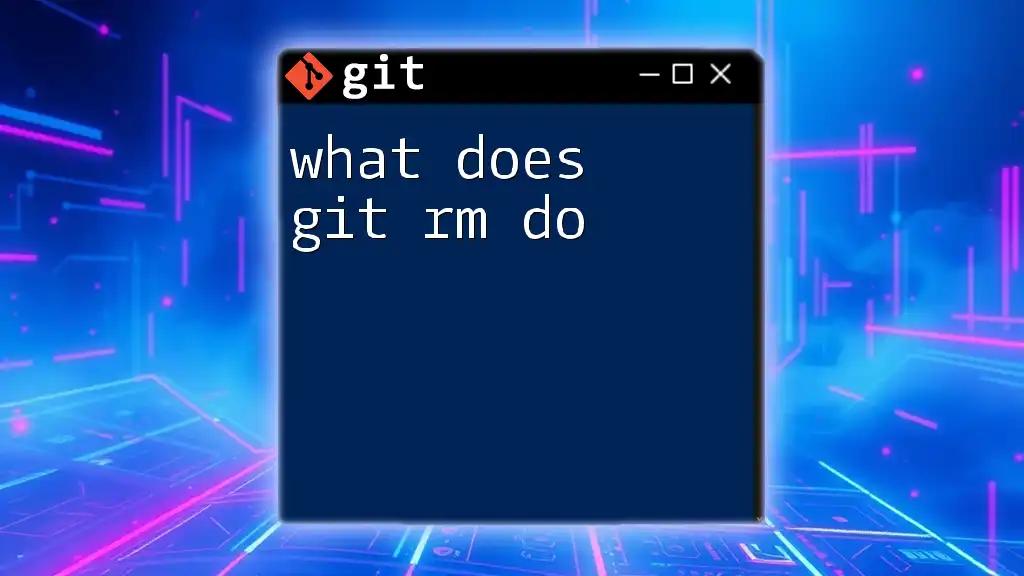
Conclusion
Understanding what `git push` does is vital for effective version control and collaboration in software development. By mastering this command, you're empowering yourself to share your work with others seamlessly while maintaining a tidy project history. As you continue to learn and practice using Git, you will find that consistent application will enhance your workflow and ease your collaborative efforts.
Feel free to reach out for further guidance, tools, and opportunities to deepen your understanding of Git commands and best practices!