The `git clean` command is used to remove untracked files and directories from your working directory, helping to keep your repository clean and organized.
Here's how you can use it:
git clean -f
This will remove all untracked files in the current directory. To also remove untracked directories, you can use:
git clean -fd
Understanding `git clean`
What is `git clean`?
The `git clean` command is a powerful tool that helps you maintain a tidy working directory in your Git repository. It focuses on untracked files, which are files that are not being tracked by Git (i.e., files that have not been staged or committed). By using `git clean`, you can remove these untracked files and directories swiftly, making it an important command for developers who want to keep their projects organized and free of clutter.
Why Use `git clean`?
There are several scenarios where using `git clean` proves particularly useful:
-
After a Merge or Rebase: If your merge or rebase introduces new changes and leftover files from previous changes remain untracked, you may want to clean up these remnants.
-
Switching Between Branches: When jumping between branches, untracked files from one branch may no longer be relevant. Cleaning these up can streamline your workflow.
-
Before Starting a New Feature: If you're about to embark on a fresh feature, ensuring that your working directory is clear of distractions can increase focus and reduce potential conflicts.
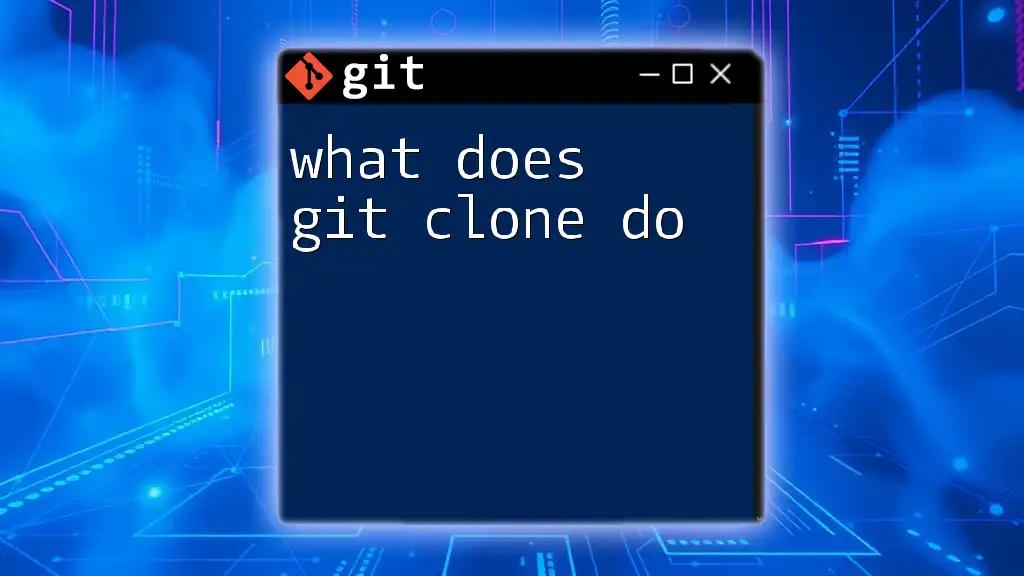
How to Use `git clean`
Basic Syntax
To utilize `git clean`, you'll use the following basic syntax:
git clean [options]
This command won't do anything unless combined with specific options.
Common Options
-d
Using the `-d` option allows you to remove not only untracked files but also untracked directories. This is particularly useful if you've created temporary directories during the development process that are now no longer needed.
Example:
git clean -d
-f
The `-f` option is critical: it forces the clean operation. Git will not delete untracked files or directories the default way for safety reasons, so you need to specify this option to execute the command.
Example:
git clean -f
-n
The `-n` option enables a dry run, which displays a list of files that would be removed without actually deleting them. This safeguard is extremely beneficial as it gives you a chance to review which files will be affected before going ahead with the operation.
Example:
git clean -n
-x
Using the `-x` option removes not only untracked files but also files that are ignored by Git (listed in .gitignore). This is particularly useful if your working directory is cluttered with ignored files that you no longer want.
Example:
git clean -f -x
-X
Conversely, the `-X` option performs the opposite of `-x` by removing only the ignored files while keeping untracked files intact. This selective clean-up can be useful if you want to retain certain untracked files while ensuring ignored files do not linger.
Example:
git clean -f -X
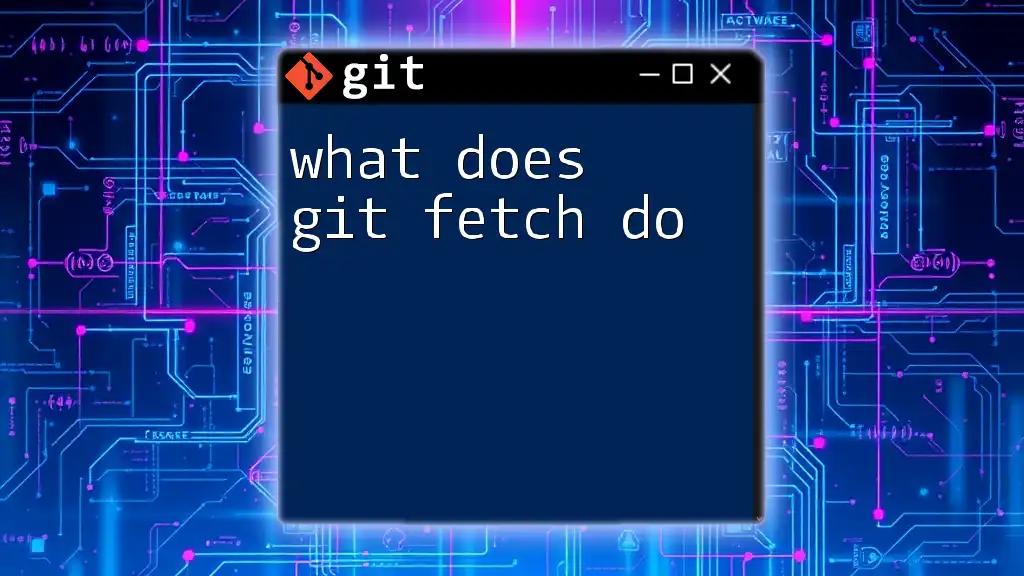
Practical Examples
Using `git clean` in Real Scenarios
Cleaning Up After a Large Merge
After merging changes, your working directory may include untracked files that are no longer necessary. Cleaning these up can ensure that you focus solely on relevant changes.
Example Command:
git clean -fd
This command will remove all untracked files and directories, allowing you to start fresh.
Preparing for a Fresh Start
Before switching to a new feature branch, it is advisable to do a thorough clean-up of untracked files that might interfere with your new work.
Example Command:
git clean -nfd
With this command, you will see a list of what will be removed without applying the changes just yet, allowing for a last-minute confirmation.
Precautionary Measures
Creating Backups
Before diving into any cleanup operations, it's a good practice to create a backup of your current changes. You can accomplish this by using `git stash` to temporarily hold any changes you don't want to lose.
Example:
git stash
This command saves your modifications, freeing you to perform a clean operation without worry.
Using the Dry Run Option
Before executing a clean command, it's always best to utilize the `-n` option. This ensures you are fully aware of the consequences of your actions.
Command Example:
git clean -n
This way, you retain control over the process, preserving any files that shouldn't be removed.
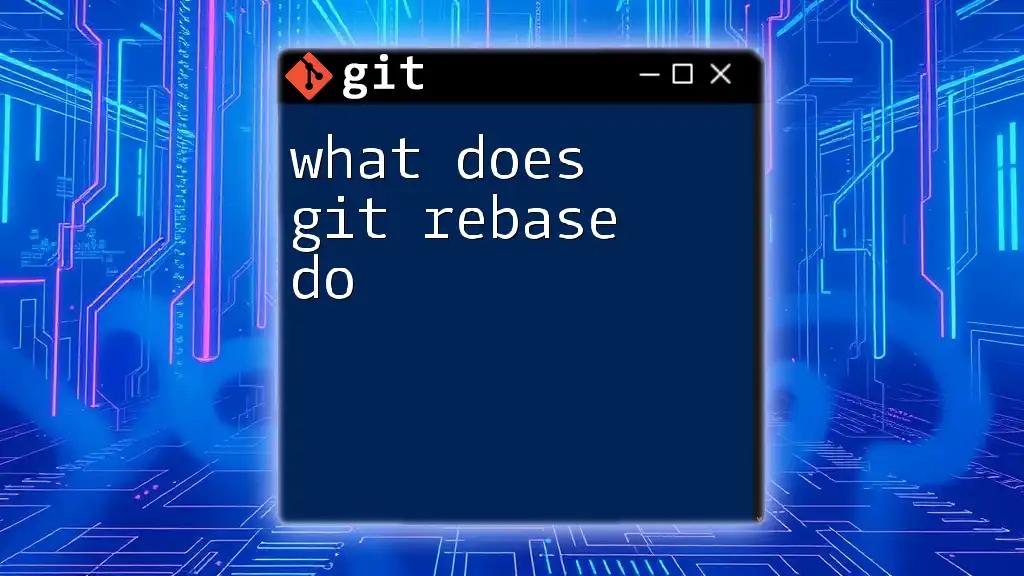
Best Practices
Understanding the Risks
While `git clean` is an incredibly helpful tool for maintaining file organization, it should be used with caution. The -f and -x flags can permanently delete important files if you're not careful. Make it a habit to double-check which files will be affected before executing the command.
Regular Maintenance
Incorporating `git clean` as part of your regular Git workflow is advisable. Consider using it in combination with other commands such as `git status` to ensure you're aware of the state of your files before proceeding with any cleaning operation.
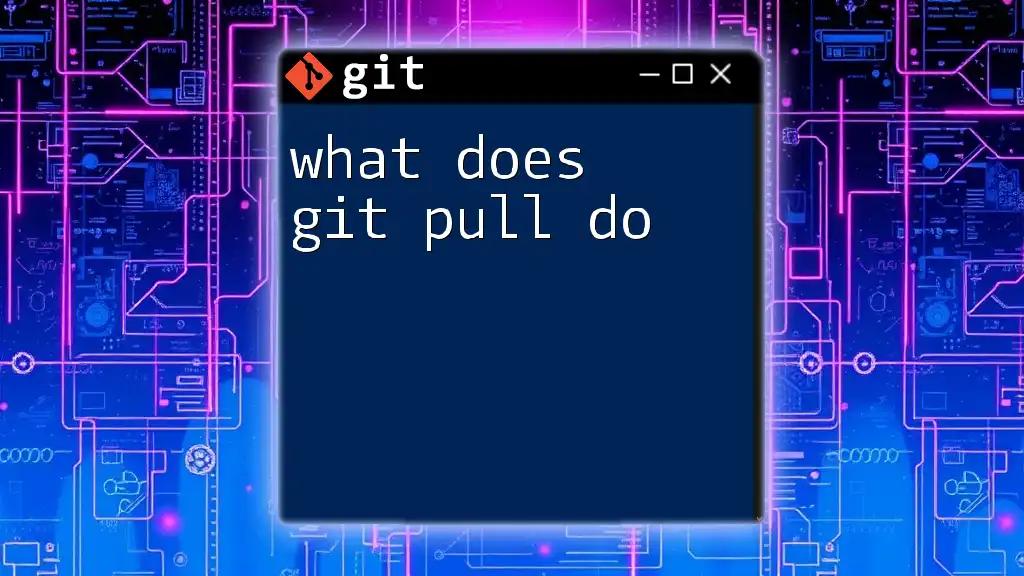
Conclusion
In summary, understanding what does `git clean` do empowers developers to maintain a clean, organized working directory effectively. Whether you are dealing with leftover files after merges or preparing for new features, `git clean` serves as an essential tool in your Git toolkit. Experiment with this command in your projects to experience firsthand the productivity boost of a clutter-free environment. Don't hesitate to reach out with feedback or questions as you embark on your Git learning journey!
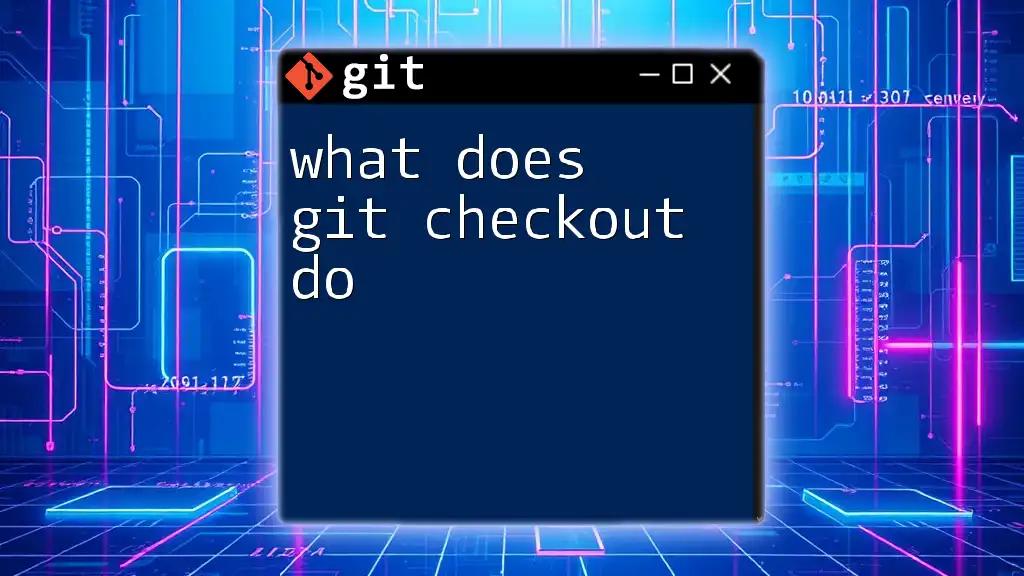
Additional Resources
For further reading and deeper understanding, consider exploring the following helpful resources:
- Official Git documentation: An exhaustive reference for all Git commands.
- Git tutorials: Numerous online platforms feature detailed Git courses.
- Command references: Websites like GitHub or GitLab often provide easy access to command lists and usage guidelines.
The knowledge gained through this article will be invaluable as you navigate your journey in Git and version control.