Git is a distributed version control system that helps manage and track changes in source code during software development, enabling collaboration among multiple developers.
Here’s a simple command to initialize a new Git repository:
git init
What is Git?
Git is a distributed version control system designed to handle everything from small to very large projects with speed and efficiency. Originally developed by Linus Torvalds in 2005 to support the development of the Linux kernel, Git has become the go-to solution for version control in software development and beyond.
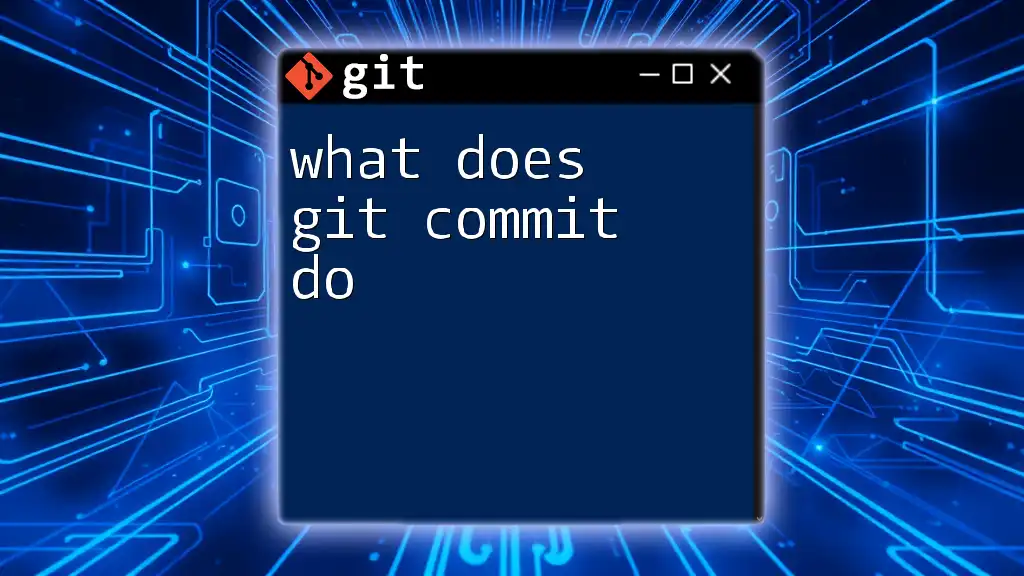
Why Use Git?
Using Git offers significant advantages for developers and teams:
- Collaboration: Multiple developers can work on a project simultaneously without overwriting each other’s work, thanks to Git's branching and merging capabilities.
- History Tracking: Git maintains a historical record of all changes, enabling developers to see who changed what and when. This is crucial for debugging and understanding project evolution.
- Backup and Recovery: With Git, you can revert to previous versions of your files effortlessly, providing a safety net against accidental loss or corruption.
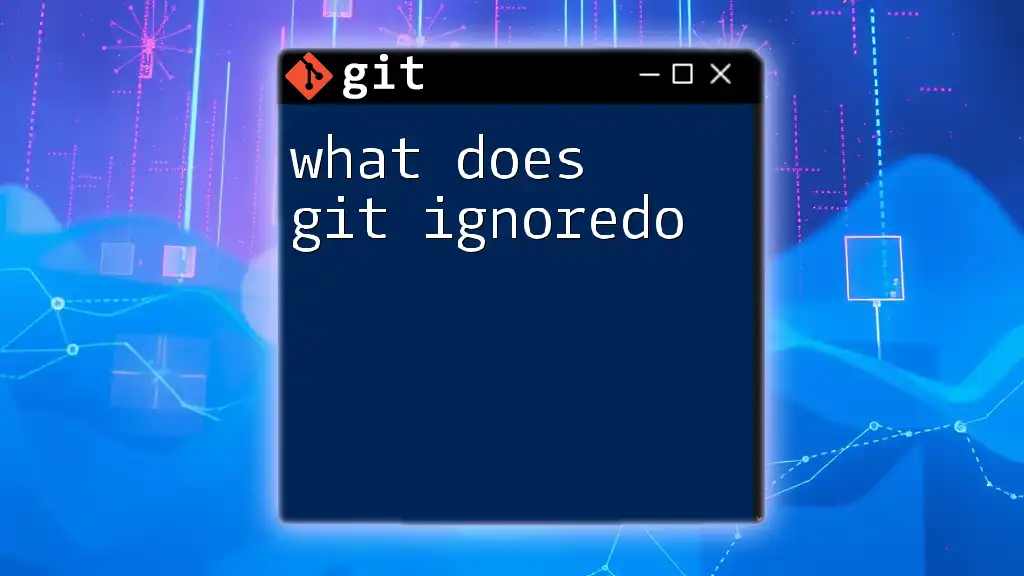
Understanding Version Control
What is Version Control?
Version control systems (VCS) like Git allow developers to manage changes to source code over time. A VCS keeps track of modifications, enabling teams to collaborate more effectively.
Difference Between Centralized and Distributed Version Control
- Centralized Version Control: In centralized systems, there is a single central repository, and all users commit their changes to this single source.
- Distributed Version Control: In distributed systems like Git, every user has their complete copy of the repository, including its history. This allows for greater flexibility and resilience.
Why is Version Control Important?
Version control is crucial because it facilitates collaboration among multiple developers, protects against data loss, and helps maintain a reliable and organized project history. Working on a shared codebase without version control can lead to chaos, miscommunication, and lost progress.
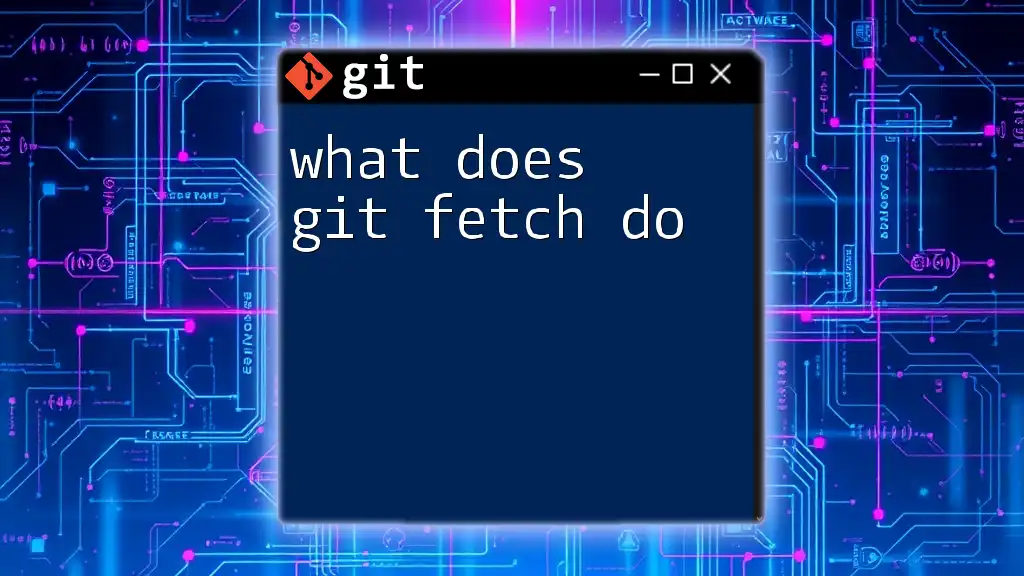
Key Features of Git
Tracking Changes in Files
One of Git's core functionalities is its ability to track changes in files. When modifications are made, Git records these changes in a commit. Each commit acts like a snapshot of the project at a specific point in time, allowing you to revert changes if necessary.
Branching and Merging
Branching in Git allows you to diverge from the main line of development. Creating a new branch is straightforward:
git branch <branch-name>
Once you make your changes, you can merge these branches back into the main line (typically called `main` or `master`):
git merge <branch-name>
This feature encourages experimentation and parallel development, enabling teams to work in isolation until they’re ready to integrate their changes.
Staging Area vs. Repository
The staging area (also called the index) is where you prepare files to be committed. Changes made to files are first added to the staging area using `git add` before committing them to the repository. This two-step process provides control over what changes are included in each commit.
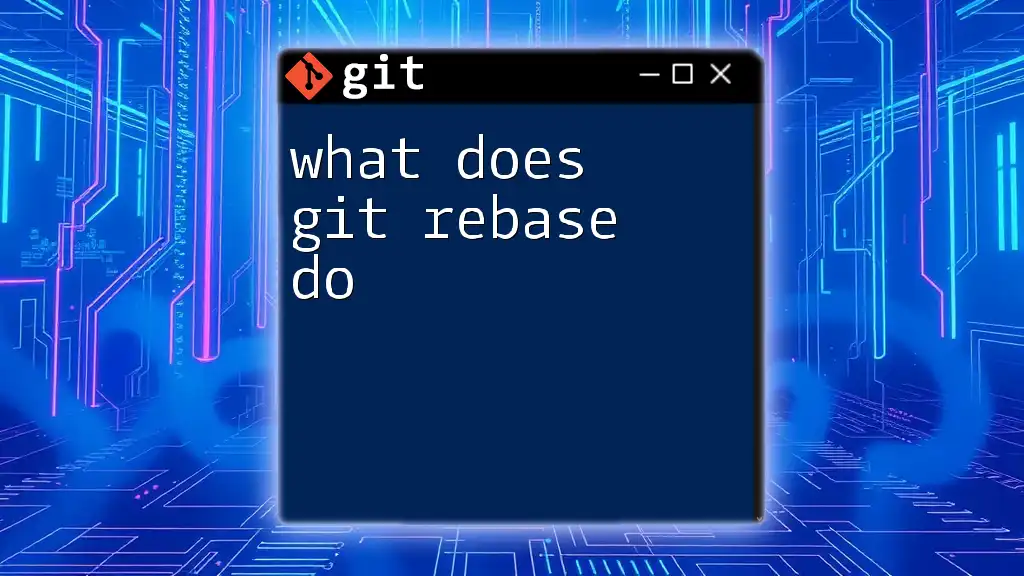
Basic Git Commands and Their Functions
git init
To create a new Git repository, you use the `git init` command. This initializes a new Git repository in the current directory:
git init
This command sets up the hidden `.git` folder that tracks all your version control data.
git add
The `git add` command stages modifications. This is how it works:
- To add a specific file:
git add <file-name>
- To add all modified files in the current directory:
git add .
Using this command frequently ensures you’re keeping your repository prepared for committing.
git commit
Committing captures a snapshot of your staged changes. You should always add a meaningful message to document your changes:
git commit -m "Your commit message here"
Good commit messages are crucial for maintaining a readable project history.
git status
To check the status of your working directory and staging area, use:
git status
This command provides insight into changes that are staged, unstaged, or untracked, assisting you in managing your workflow effectively.
git push and git pull
Pushing changes to a remote repository allows others to access your work. To push your committed changes, you would use:
git push origin <branch-name>
Conversely, pulling retrieves changes from a remote repository and merges them into your local branch:
git pull origin <branch-name>
These commands are fundamental for collaborating with others in a shared environment.
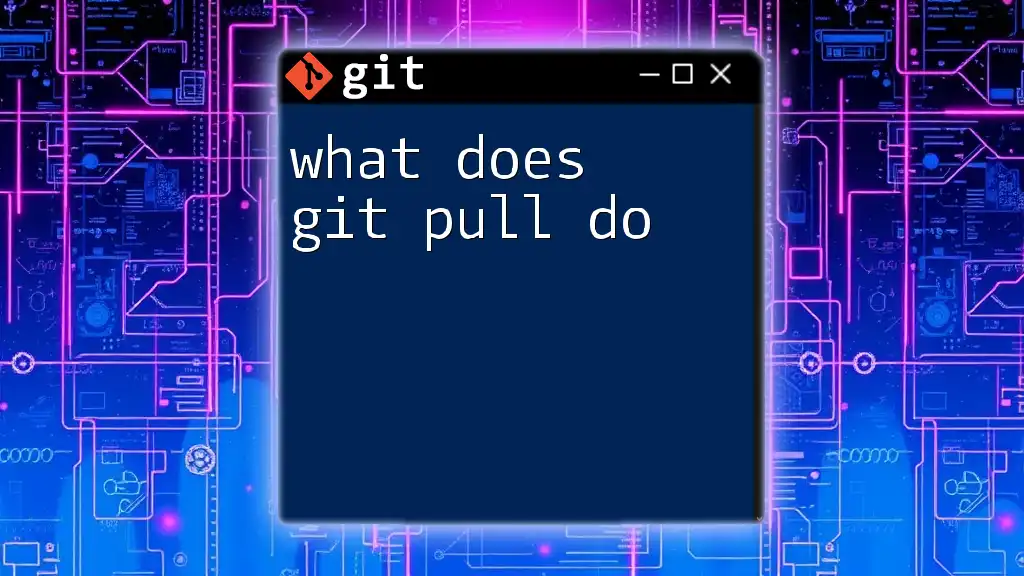
Understanding Remote Repositories
What are Remote Repositories?
A remote repository is a version of your project that is hosted on the internet or another network. It enables distributed collaboration as multiple people can access and contribute concurrently.
How to Connect to a Remote Repository
To copy a remote repository to your local machine, utilize the following command:
git clone <repository-url>
This command creates a local copy of the remote repository, enabling you to start working on it instantly.
Collaboration with Remote Repositories
Using remote repositories, teams can collaborate effectively through Pull Requests (PRs). A PR is a request to merge changes from one branch into another, usually requiring code review:
- Teams can discuss changes within the PR.
- After review, the changes can be merged into the main branch.
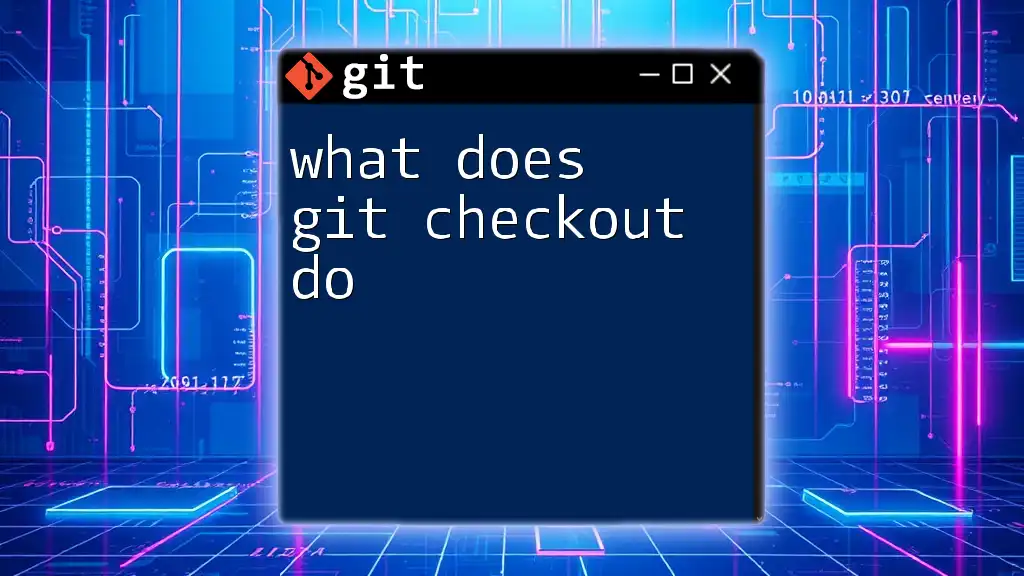
Navigating Git History
Viewing Commit History
To view your project's commit history, use:
git log
This command displays a series of entries about past commits, providing insights into the project's evolution.
Reverting Changes
If you want to revert to a previous commit, you can checkout that commit with:
git checkout <commit-hash>
This allows you to inspect an earlier state of your project. Alternatively, to reverse changes made by a commit, use `git revert`, which creates a new commit that undoes the previous changes.
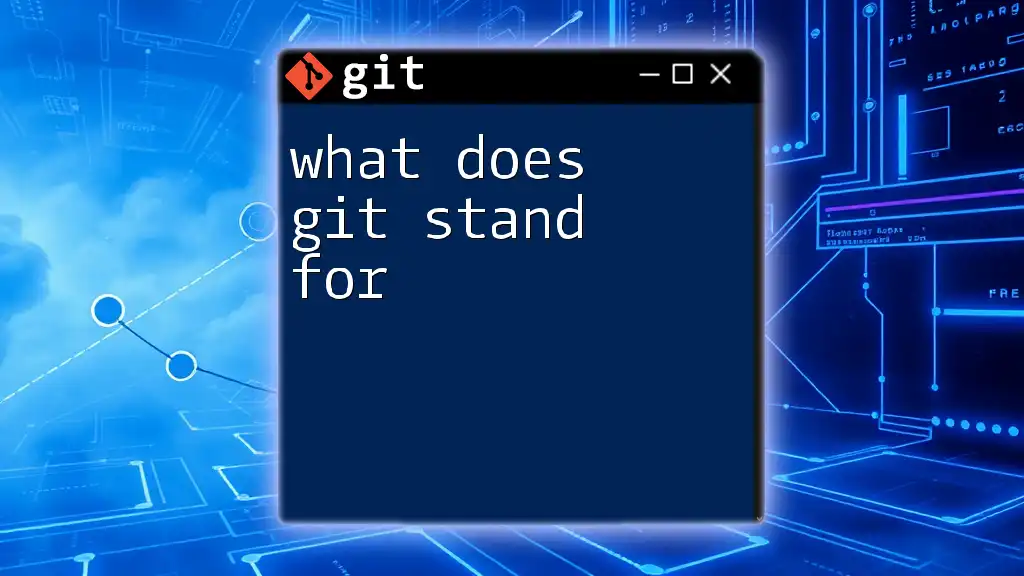
Conclusion
In summary, Git plays a critical role in modern development practices by enabling effective collaboration, change tracking, and project management. Understanding what Git does and how it works empowers developers to manage their code effectively and maintain a reliable workflow.
As you explore Git further, consider leveraging resources such as the official Git documentation, online courses, and books that delve deeper into its powerful capabilities. Familiarizing yourself with Git commands and principles will ultimately enhance your software development skills and contribute to your success in collaborative projects.