The `git commit -am` command combines staging tracked files and committing changes with a message in one step, allowing you to quickly log changes without needing to manually stage each file first.
git commit -am "Your commit message here"
Understanding Git Commit
What is a Git Commit?
A Git commit is essentially a snapshot of the changes made to your project at a specific point in time. When you commit, you're telling Git to save the current state of your files, so you can revisit that state later if needed. Each commit is associated with a unique identifier (SHA-1 hash), which makes it easy to track the history of the project.
The commit serves not only as a record of changes but also as the foundation for collaboration among multiple developers. With each commit, you can document what you changed through a descriptive commit message, making it clear for anyone reviewing the history what was accomplished.
Importance of Committing Changes
Committing changes regularly is crucial for various reasons:
- Version Control: Each commit provides a historical context. This allows you to revert back to previous versions if something goes wrong.
- Collaboration: When working in teams, clear commits ensure that everyone is on the same page and has a clear understanding of what has been worked on.
- Audit Trail: Commits act as an audit trail for your project, making it easier to track issues or changes over time.
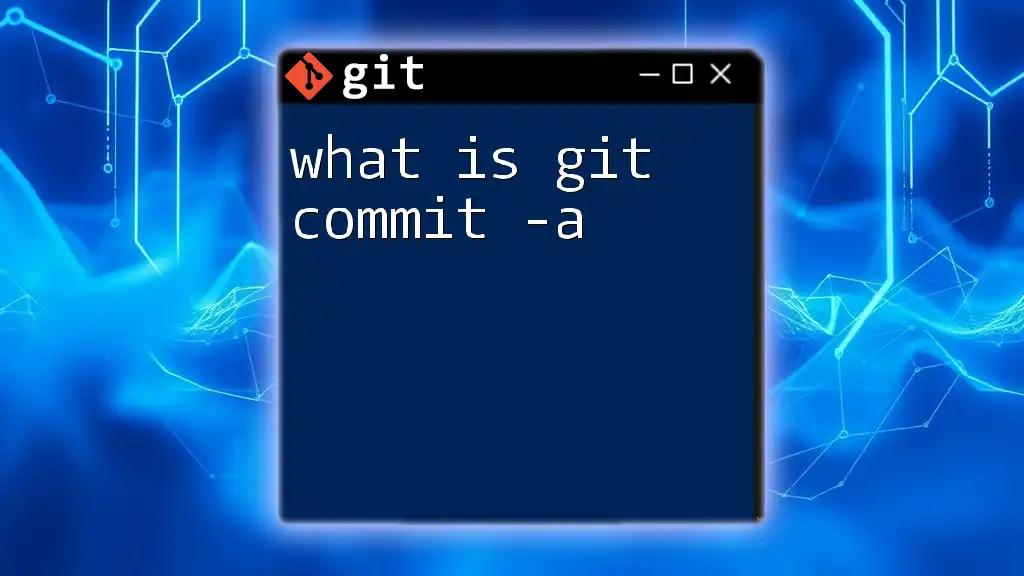
Decoding the Command: `git commit -am`
What Does `-a` Stand For?
The `-a` option in the `git commit -am` command stands for "all." When you use this flag, Git automatically stages all tracked files that have been modified before committing them. This means you don't have to explicitly use `git add` for each modified file.
Tracked files are files that Git has already been made aware of, typically because you have added them to the repository at some point. In contrast, untracked files are new files that have not been added to the repository.
What Does `-m` Stand For?
The `-m` option stands for "message." It allows you to include a commit message directly in the command line, thus saving you from launching the text editor that Git typically uses for commit messages.
Providing a meaningful commit message is vital since it encapsulates the purpose of the commit, enhancing collaboration and making tracking easier for anyone reviewing the commit history.
The Combined Command: `git commit -am`
Using the `git commit -am` command effectively combines both the `-a` and `-m` flags, allowing you to stage all modifications to tracked files and commit them in a single line.
Here's how it generally looks:
git commit -am "Your descriptive commit message here"
This command streamlines the development process, allowing for quicker commits without needing to stage each modified file manually.
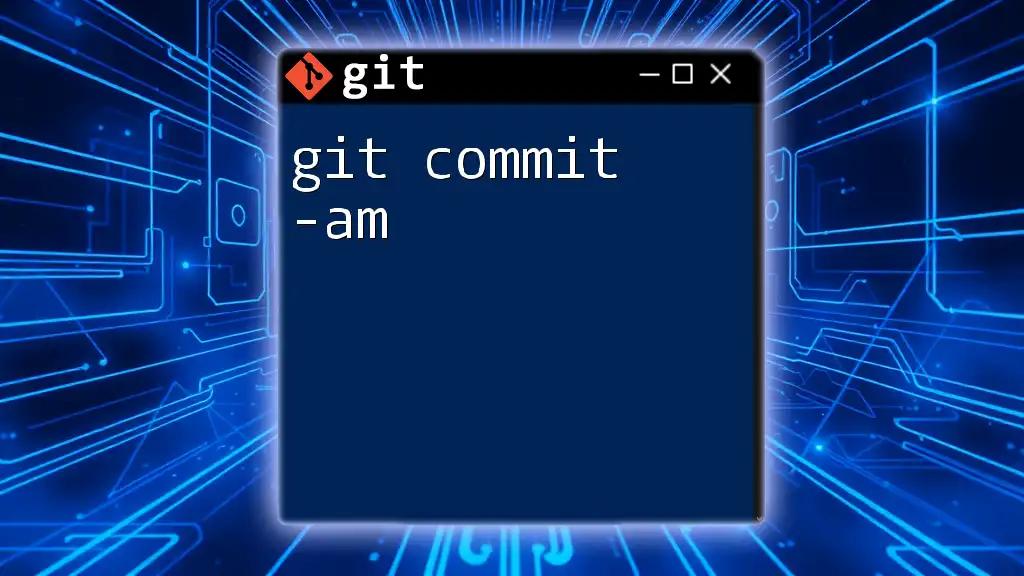
Practical Examples
Example 1: Making a Quick Commit
Suppose you've just fixed a bug in your application. After making the necessary changes in your code, you can quickly commit your work using:
git commit -am "Fixed a bug in the login feature"
In this case, the command stages all modified tracked files and commits them, which makes your workflow more efficient.
Example 2: Committing After Making Changes
Imagine you've made several edits to your project files. To commit these changes, simply:
- Modify a file (e.g., `index.html`).
- Run:
git commit -am "Updated the header in index.html"
This command automatically stages `index.html` if it's a tracked file and commits it with the provided message.
Example 3: What Happens with Untracked Files?
If you have untracked files in your repository (files that Git hasn't added to be tracked yet), the `-a` flag will not stage these files for commit. To see untracked files, use:
git status
This will show you which files are untracked. If you try to commit with `git commit -am "Message"` when there are untracked files, Git will not include them in the commit. This highlights the importance of managing your file states carefully.
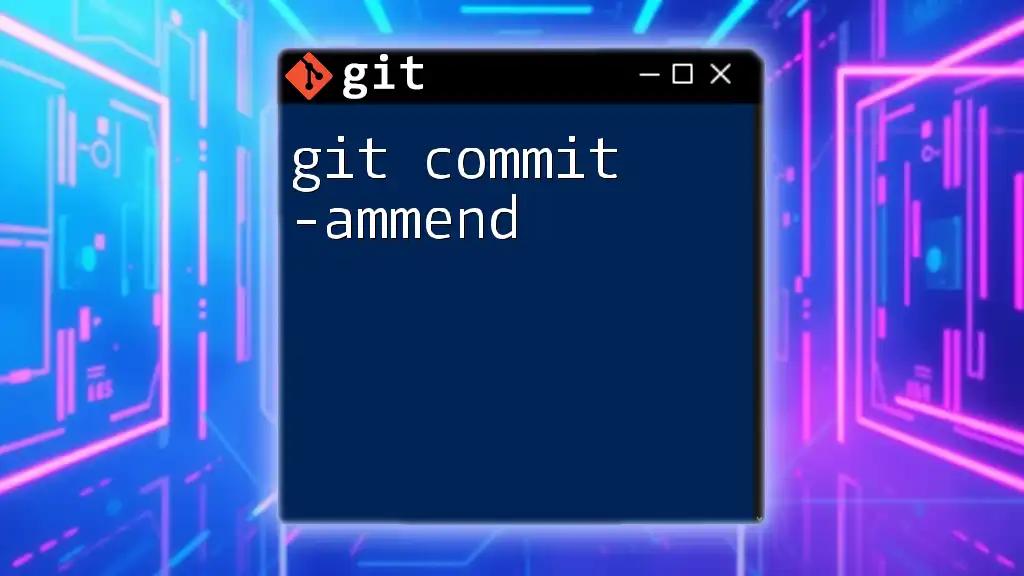
Common Use Cases for `git commit -am`
Quick Fixes
The `git commit -am` command shines in scenarios where rapid changes are necessary, such as urgent bug fixes or minor updates. The ability to quickly stage and commit changes enables developers to maintain momentum and productivity.
Daily Development Processes
In the daily routines of software development, regularly using `git commit -am` allows for a smoother workflow. It helps developers keep their work documented without adding unnecessary steps to their processes.
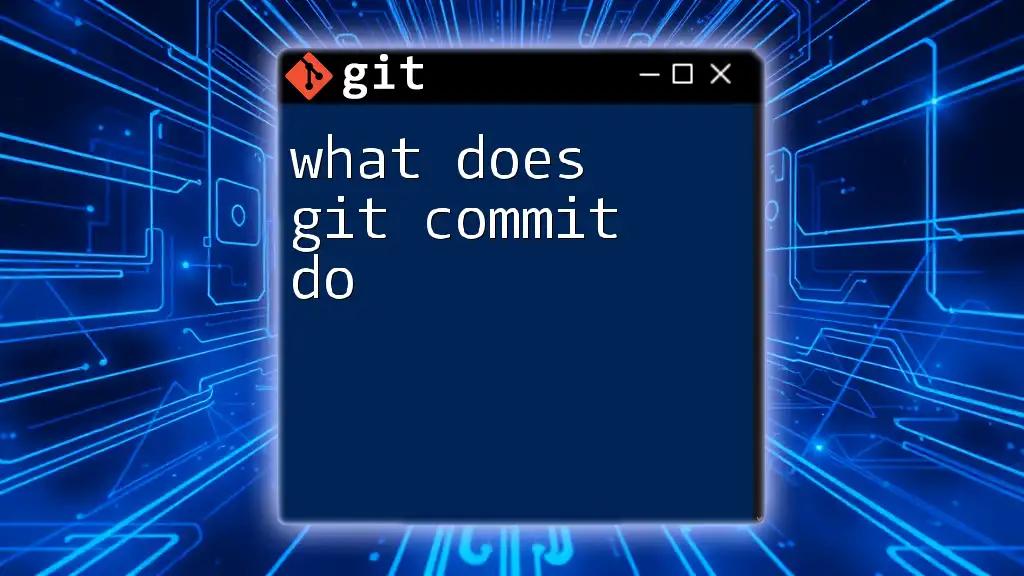
Potential Pitfalls
Overusing `git commit -am`
While this command can speed up your workflow, overusing it can lead to accidental commits of unwanted changes. Since `-a` stages all modified tracked files, you might commit changes you didn’t intend to include. Always run `git status` to verify what will be included in your commit.
Creating Ambiguous Commit Messages
The quality of your commit message is just as important as the codes you commit. A vague message like "Fixed stuff" fails to provide context for future developers or even yourself. Commit messages should be clear and descriptive.
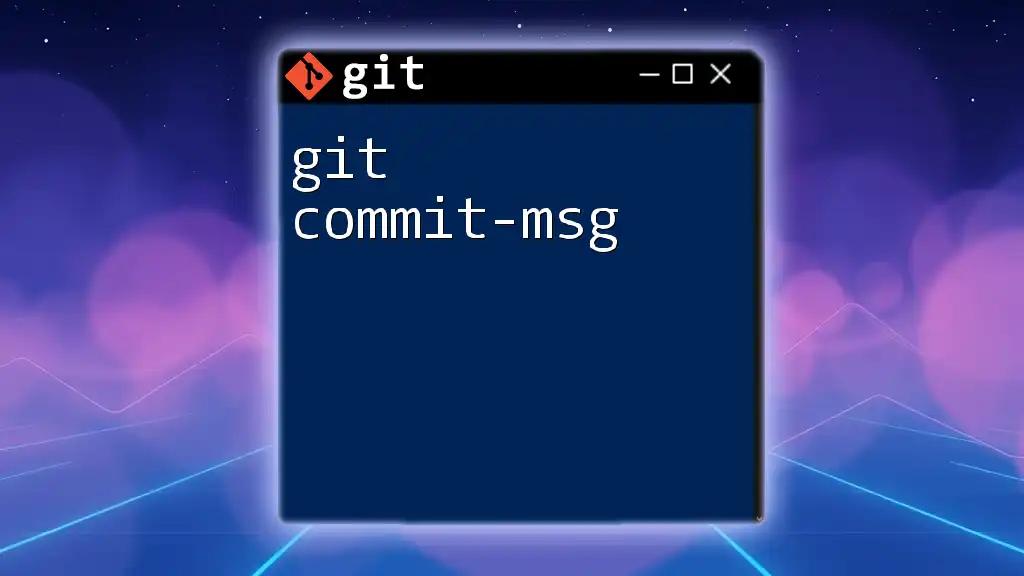
Best Practices for Committing Changes
Creating Meaningful Commit Messages
Good commit messages follow a concise structure:
- Summary Line: A brief overview of the changes. E.g., "Refactored payment processing logic."
- Optional Body: Explain the "why" and "how" of the changes if necessary.
Staging Changes with Care
Fine-tuning your commits involves using `git add` to stage only the most relevant changes. This is particularly helpful when you’re working on multiple features simultaneously but don’t want to embed unrelated changes.
For example:
git add file1.js
git commit -m "Implement feature X"
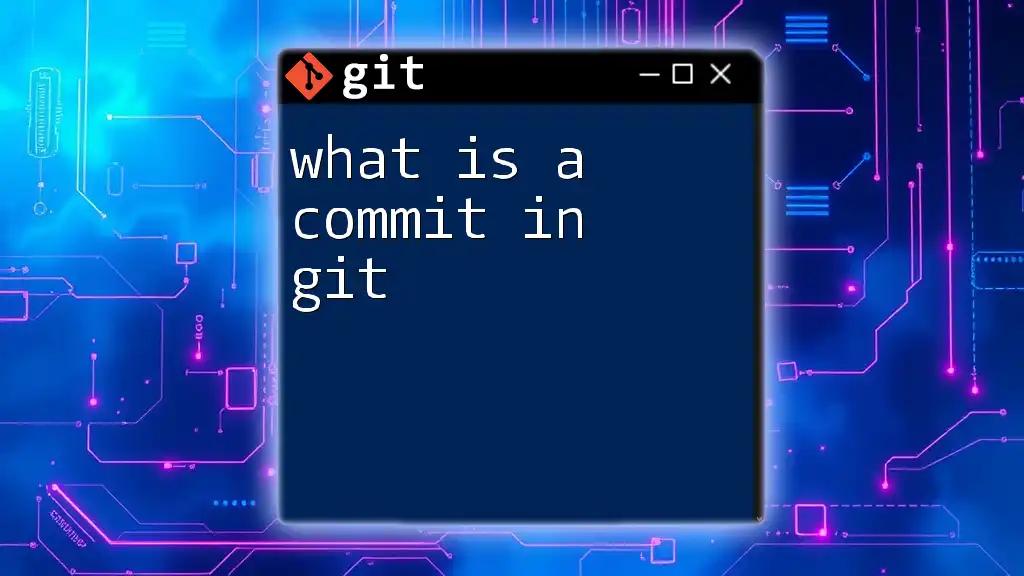
Alternative Commands
Using `git commit` without Options
You can always use the basic `git commit` command for more granular control:
git commit
This opens your text editor for a detailed commit message, providing the opportunity for more elaboration.
Other Useful Git Commands
To maximize your use of Git, familiarize yourself with other commands such as `git status`, `git log`, and `git diff`. These commands help you manage commits and understand the state of your repository better.
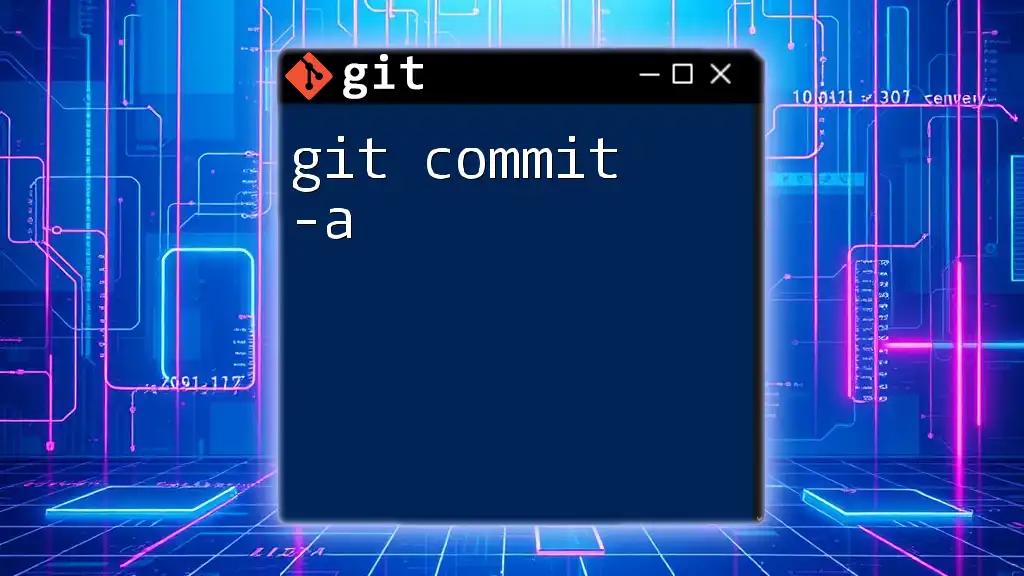
Conclusion
The `git commit -am` command is a powerful tool in the Git command-line toolkit, allowing you to streamline your development process. By mastering this command, you can enhance your productivity and maintain better control over your project's history. Practice incorporating this command wisely in your workflow, and watch your efficiency soar.