The `git commit -p` command allows you to selectively commit changes by interactively reviewing and staging only the specific parts of files you want to include in your commit.
git commit -p
The Basics of Git Commit
What is a Basic Git Commit?
A standard Git commit is the fundamental way to save changes to your repository. It captures the current state of your files and records the changes in your project’s history. The basic syntax for a Git commit is:
git commit -m "Your commit message here"
In this command:
- `git commit` is the command used to create the commit.
- `-m` specifies that a message follows, which describes the changes made.
The commit message should be concise yet descriptive, providing context for the changes made.
Why Use `git commit -p`?
The `git commit -p` command, where `-p` stands for "patch," allows you to commit only a portion of the changes in your working directory. This is particularly useful in situations where you've made multiple edits across different lines or files but only want to include a particular set of changes in your commit. By using `git commit -p`, you can avoid cluttering your project history with unrelated changes and keep your commits clean and focused.
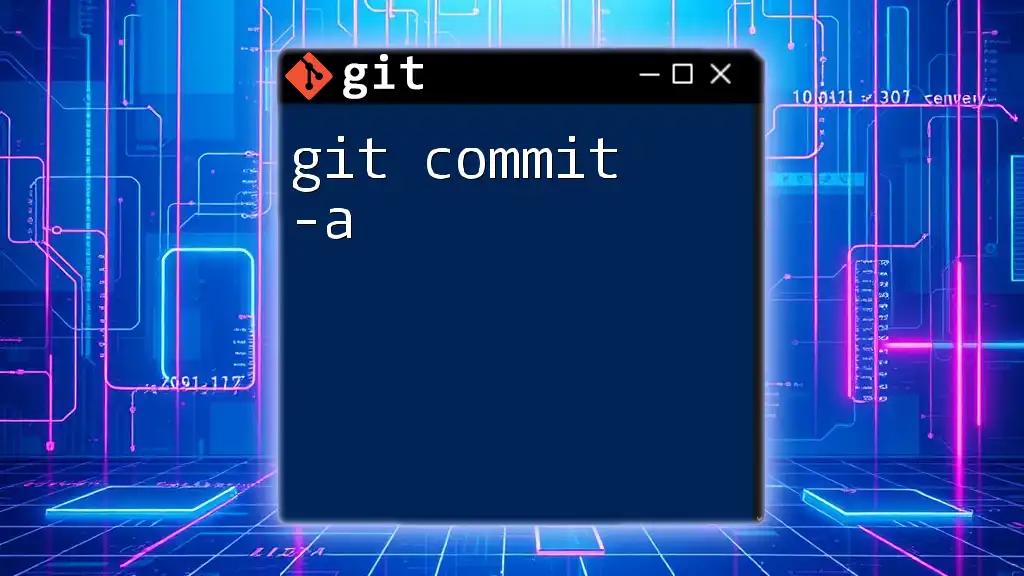
How to Use `git commit -p`
Prerequisites for Using `git commit -p`
Before you can use `git commit -p`, it's essential to have changes in your working directory that you want to commit. Ensure your files are saved and that you're aware of the current status:
git status
The output will show which files have changes and whether they’re staged for commit.
Step-by-Step Guide to Using `git commit -p`
Preparing Your Environment
Start with a clean working directory. Prior to running `git commit -p`, you want to review your changes. Use the `git status` command to see which files have changes and confirm that you indeed want to commit specific alterations.
Running the Command
To execute the command, simply type:
git commit -p
When you run this command, Git enters an interactive mode, presenting you with patches corresponding to the changes in your files. Here, you will choose which hunks (a hunk is a contiguous block of changes between two versions of a file) you'd like to commit.
Interactive Patch Selection
Understanding the Interactive Mode
During interactive patch selection, Git will present you with each hunk that can be staged. It will look something like this:
diff --git a/example.txt b/example.txt
index abcdef1..bcdef23 100644
--- a/example.txt
+++ b/example.txt
@@ -1,3 +1,3 @@
-Line of code 1
+Modified line of code 1
Line of code 2
Line of code 3
Here, the changes are shown in a diff format, with lines that have been removed denoted by a `-` and lines that have been added denoted by a `+`.
Navigating the Patch Selection
When prompted, you will receive a series of options:
Stage this hunk [y,n,q,a,d,/,s,e,?]?
- `y`: Stage this hunk for commit.
- `n`: Do not stage this hunk.
- `q`: Quit; do not stage this hunk nor any of the remaining hunks.
- `a`: Stage this hunk and all later hunks in the file.
- `d`: Do not stage this hunk nor any of the later hunks in the file.
Choose according to your needs. This process allows you to tailor exactly which changes to include in your commit, enhancing the clarity of your version history.
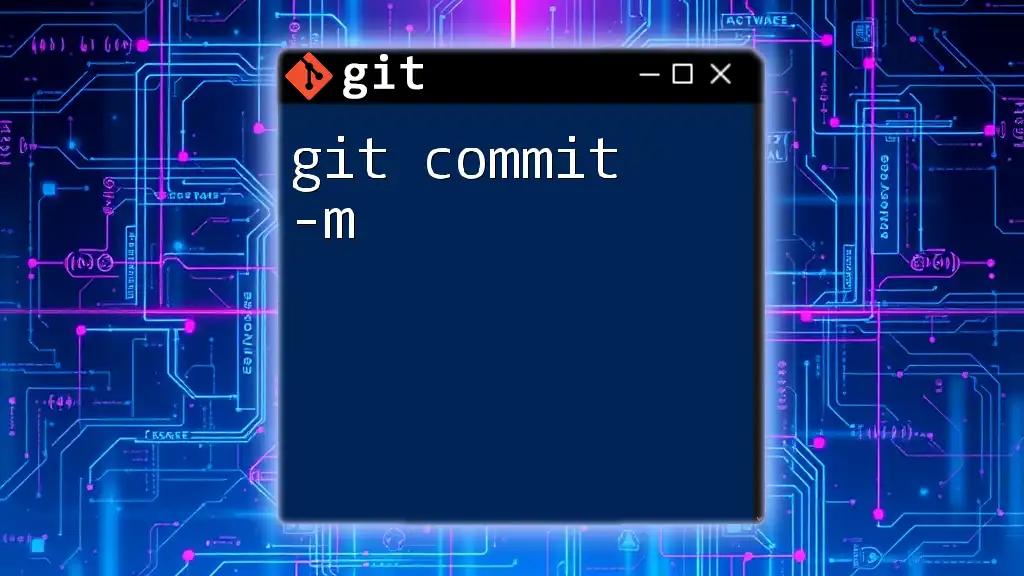
Real-World Examples of Using `git commit -p`
Scenario 1: Committing Part of a Large File
Imagine you’re working on a large file where only a specific section requires modifications. Let’s say you've changed one line and added another in a file. With `git commit -p`, you can selectively commit only the relevant changes, thus avoiding sending unintended edits to the repository.
Before committing, your `git diff` might show:
diff --git a/example.txt b/example.txt
index abcdef1..bcdef23 100644
--- a/example.txt
+++ b/example.txt
@@ -1,3 +1,3 @@
-Line of code 1
+Modified line of code 1
Line of code 2
Line of code 3
Using `git commit -p`, after navigating through the hunks, you could choose just to stage and commit the modified line, clearly documenting the intention of the changes.
Scenario 2: Staging Selective Changes Across Multiple Files
If you’ve been working on various features across multiple files, and you need to commit only certain changes, `git commit -p` allows you to include only the relevant ones. For instance, if you have changes in `file1.txt` and `file2.txt`, you can review and stage the necessary hunks individually, creating more meaningful commits.
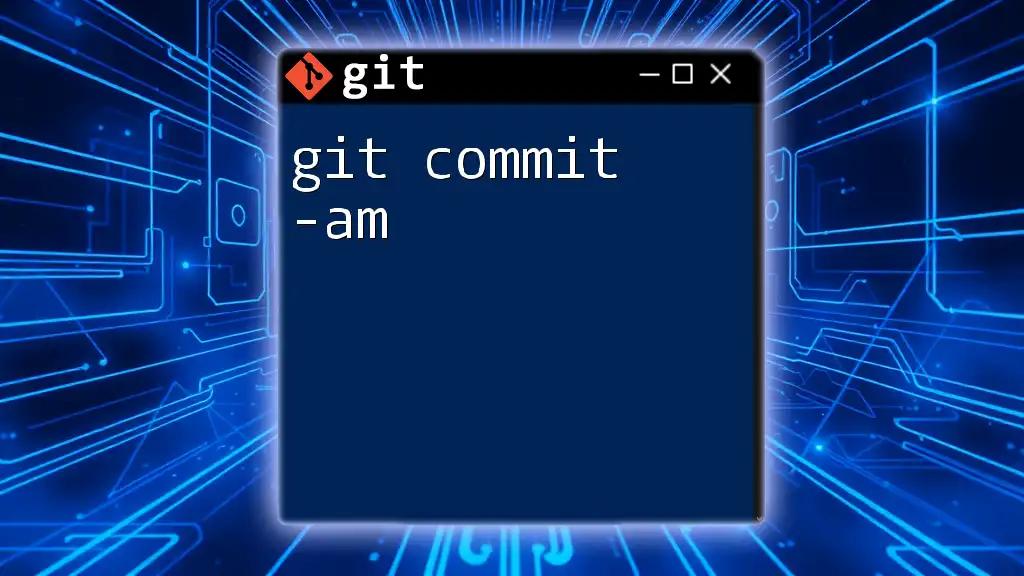
Tips and Best Practices for Using `git commit -p`
Keep Your Commits Small and Focused
One of the core principles of effective version control is to ensure your commits are small and focused. Large commits can obscure the intention behind changes and complicate future debugging processes. By utilizing `git commit -p`, you can create meaningful commits that encapsulate a single idea or feature enhancement, making the project history clearer and easier to navigate.
Writing Meaningful Commit Messages
With `git commit -p`, you have the chance to attach specific messages to the hunks you selected to stage. This not only clarifies what each change accomplishes but also aids in later reviews of the project history. Good commit messages follow the format of describing what was changed and why it was necessary.
Testing Before Committing
Before you commit your changes, it’s a good practice to test them. Ensure that the chunks you’re staging indeed work as expected. You can use tools like linters or automated test suites to verify these changes before they are written to the project history.
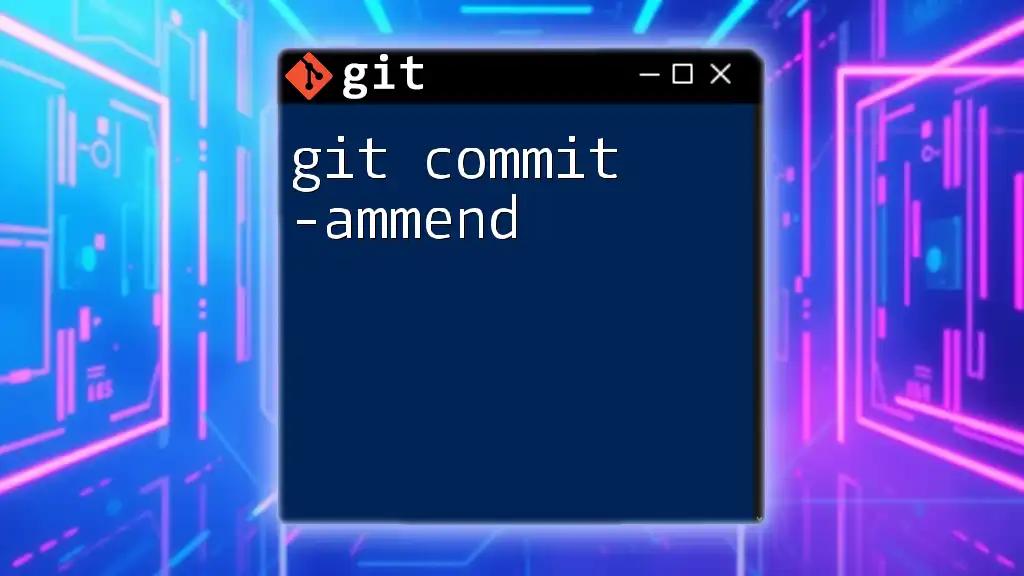
Common Challenges with `git commit -p`
Dealing with Merge Conflicts
Using `git commit -p` can sometimes complicate merge conflicts, especially when dealing with changes across multiple branches or files. If patches contain conflicting changes, Git will provide you with the option to resolve conflicts first. Carefully manage your conflicts before proceeding with committing changes to ensure a clean history.
What To Do If You Committed the Wrong Changes
If, after executing `git commit -p`, you realize that you’ve committed the wrong changes, don’t panic. You can easily undo the last commit with:
git reset HEAD~1
This command removes the last commit while keeping your changes in the working directory, allowing you to re-select hunks using `git commit -p`.
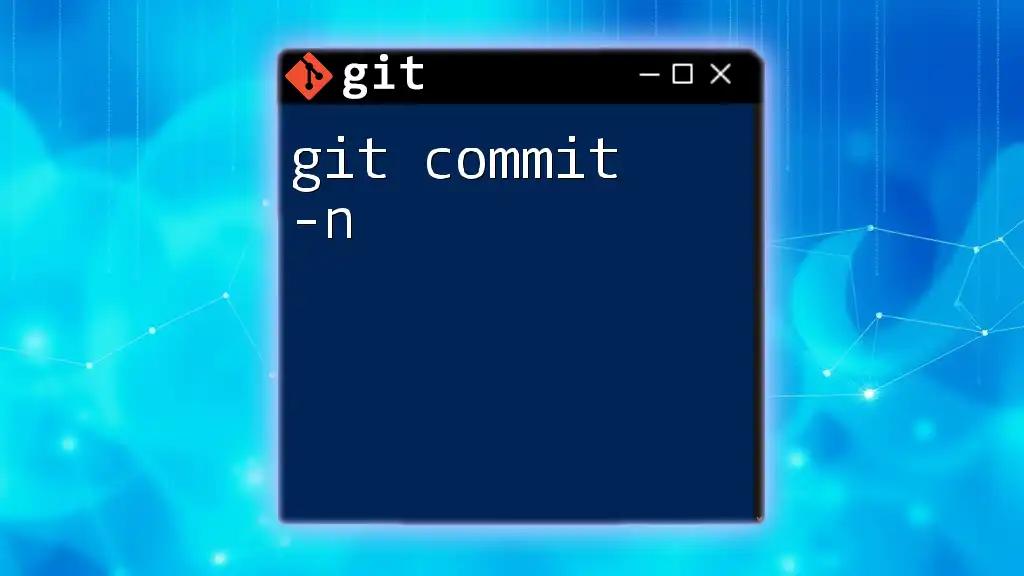
Conclusion
Using `git commit -p` empowers developers to take control of their commits, ensuring that each commit is purposeful and well-organized. This not only improves version history clarity but also aids in collaboration among team members. Practicing with this command will enhance your Git proficiency and streamline your development process.
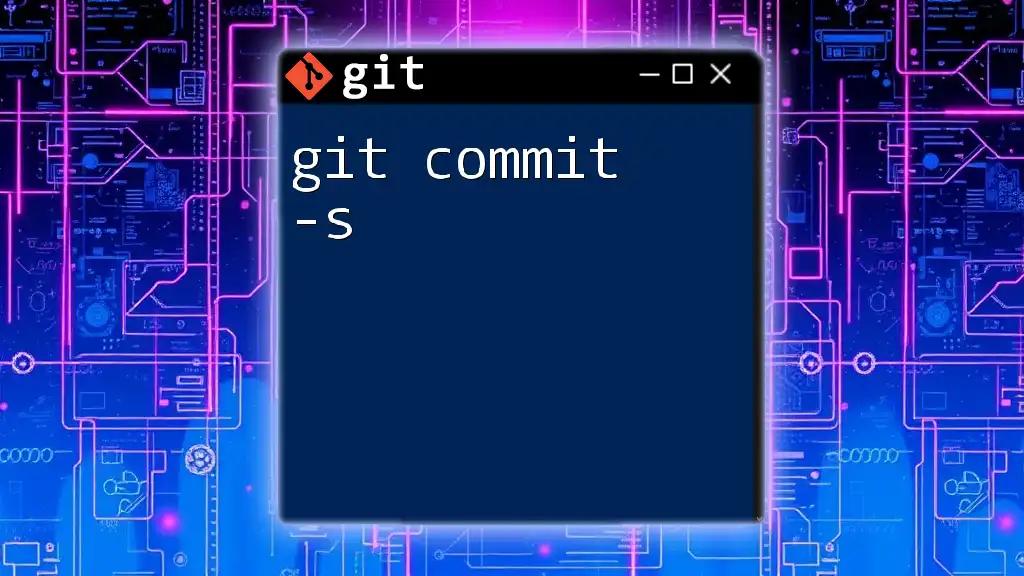
Additional Resources
For further reading and a deeper understanding, consider exploring the official Git documentation, which provides extensive insights into all Git commands and their practical applications. Seeking out community tutorials can also offer varied perspectives on mastering Git.
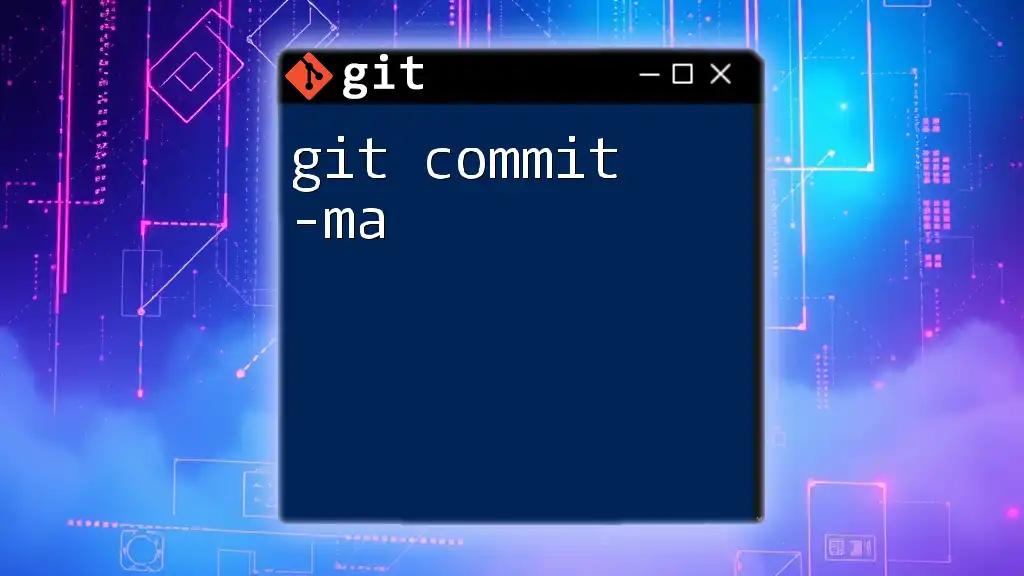
Call to Action
Take the next step in your Git journey. Introduce yourself to the myriad ways `git commit -p` can enhance your productivity and clarity in version control. Subscribe for more insights, tutorials, and resources designed to boost your software development skills!