The `git commit -a -m` command creates a new commit in the Git repository while automatically staging all modified files and including a message to describe the changes.
git commit -a -m "Your commit message here"
Understanding Git Commits
What is a Git Commit?
A commit in Git is a snapshot of your work at a specific point in time. Each commit records changes you’ve made to the files in your repository, allowing you to track the history of those files and revert back if needed. This is essential for effective version control, as it provides a clear history of what changes were made, who made them, and why.
In Git, there are two main areas to understand: staging and committing. When you make changes to files, those changes first exist in your working directory. To save those changes, you usually stage them using `git add`, which prepares the changes for a commit. A commit then permanently records those staged changes in the project history.
The Role of `-m` Flag
The `-m` flag stands for "message" and is used to provide a brief description of the changes you are committing. This is particularly important for understanding the purpose of each commit when revisiting the project later or when working with other team members.
Consider this example:
git commit -m "Initial commit of project structure"
With the `-m` flag, you can create a commit without entering a text editor, streamlining the process. However, it’s crucial to create meaningful commit messages. A well-written commit message helps clarify the intent behind changes and adds value to the project's documentation.
Understanding the `-a` Flag
The `-a` flag is short for "all." When you use this flag, Git automatically stages all tracked files (those that have previously been added to your repository), so you don’t have to manually add them with `git add`. This can greatly speed up your workflow when you're making multiple file changes and are sure you want to commit them all.
For example, using:
git commit -a -m "Refactor code for better readability"
will stage all your modified files and then commit the changes with the provided message. However, note that this command will not stage new files that have not yet been added to the repository.
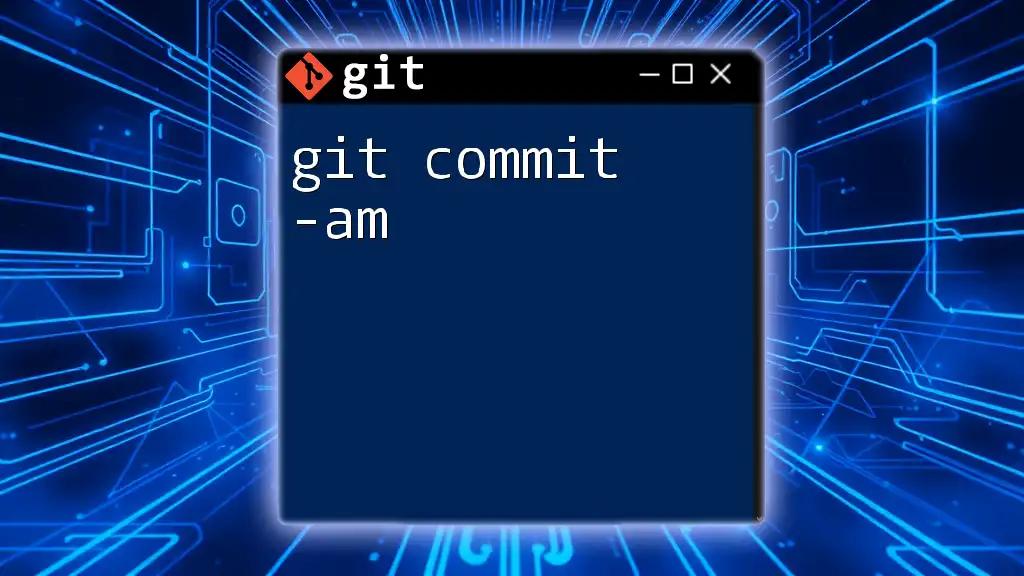
Syntax of the Command
Basic Syntax
The syntax for the command is straightforward:
git commit -a -m "commit message"
- git: The command-line tool itself.
- commit: The action you are performing.
- -a: Tells Git to stage all modified files.
- -m: Allows you to include a commit message inline.
- "commit message": The message that describes the changes you are committing.
Example of Basic Usage
When you run:
git commit -a -m "Update README with installation instructions"
Git stages all modified files and creates a new commit with the message provided. This not only saves your changes but also documents what was done in that specific commit, making it easier to understand the project’s history.
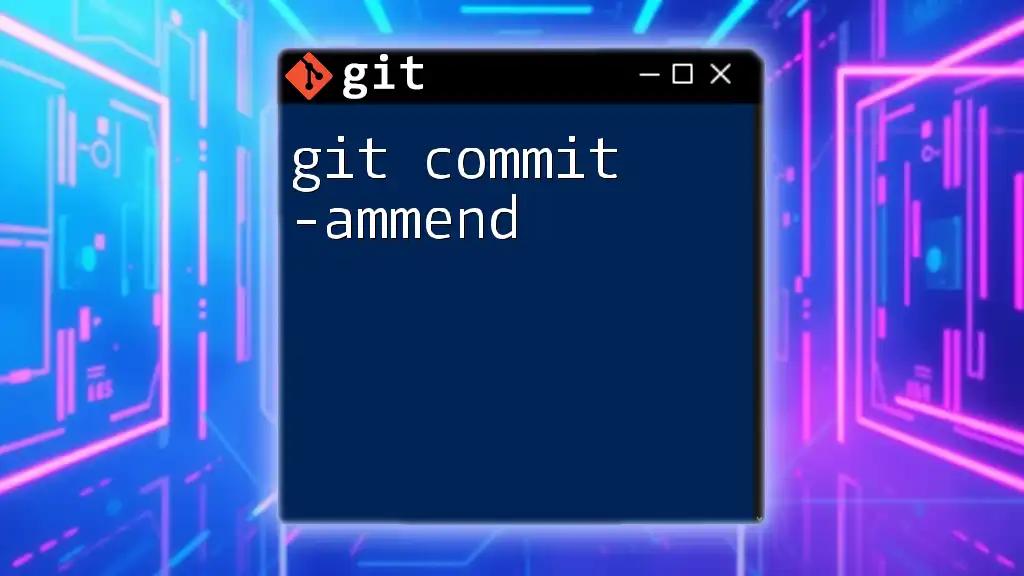
Best Practices for Commit Messages
Importance of Good Commit Messages
Good commit messages are vital for effectively managing a project. They help communicate what changes were made and why. A clear and concise message helps both your future self and collaborators understand the changes and the intent behind them.
Structure of a Good Commit Message
When crafting a commit message, aim for a structure that includes:
- Subject line: A brief summary, ideally kept under 50 characters. For example, "Fix bug in filtering function".
- Body (optional): When necessary, include a more detailed explanation, especially for significant changes or complex reasoning. Aim for clarity and brevity to ensure that anyone reading it can quickly grasp the modifications made.
Ultimately, a well-structured commit message enhances communication within development teams and improves project documentation.
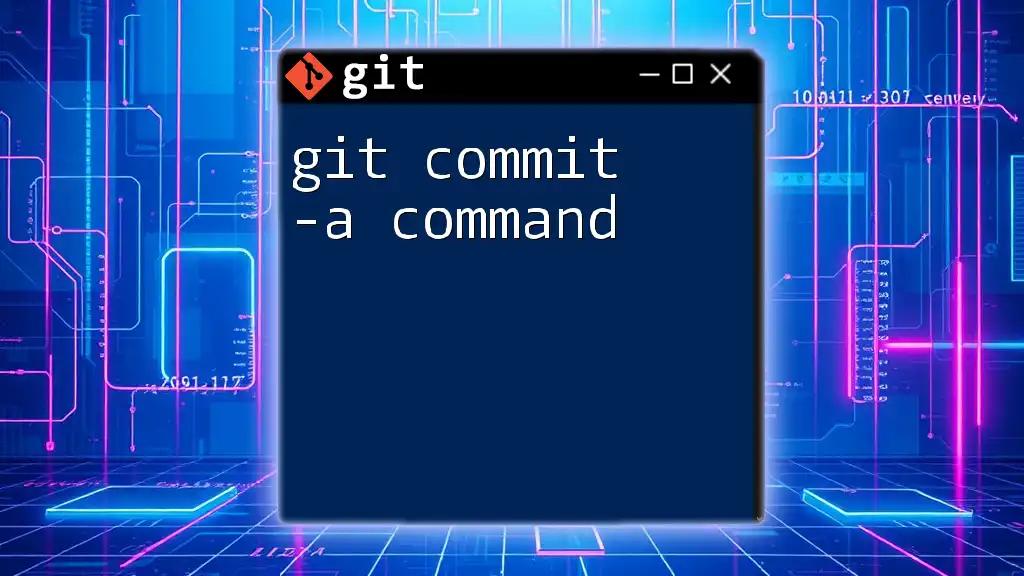
Common Use Cases
Quick Fixes
When you find a minor error in your code and want to fix it quickly, using `git commit -a -m` can be incredibly efficient. For example:
git commit -a -m "Fix typo in main file"
This command stages the fixed file and creates a commit without any further hassle.
Updating Documentation
Documentation updates often accompany code changes. Using:
git commit -a -m "Update API documentation for new features"
ensures that both the code and its documentation are kept in sync, further enhancing the clarity and usability of your project.
Combining with Other Commands
You can enhance your command-line efficiency by combining `git commit -a -m` with other commands like `git push`. For instance:
git commit -a -m "Add new feature" && git push origin main
This command commits your changes and immediately pushes them to the remote repository, streamlining your workflow.
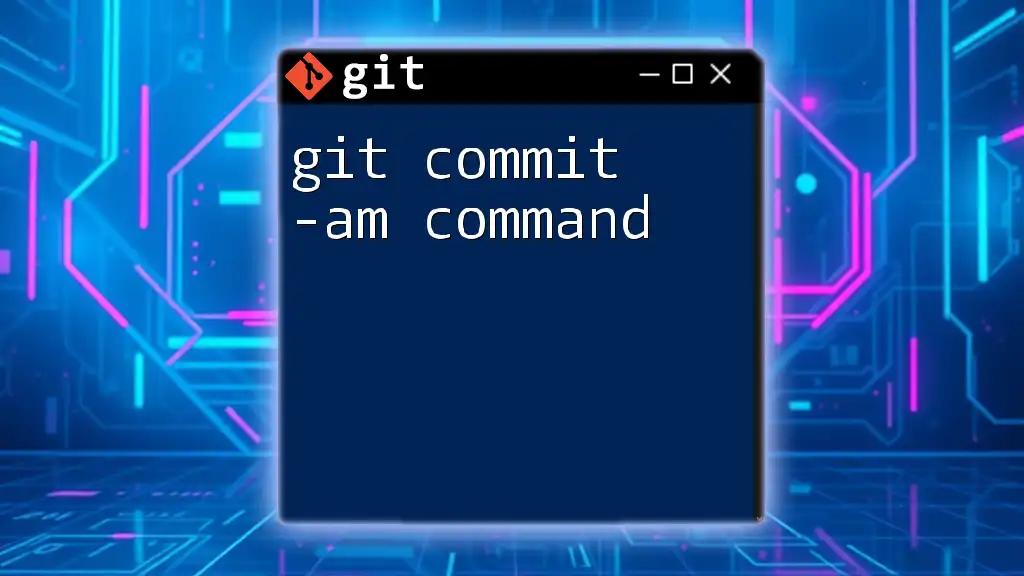
Potential Pitfalls and Misunderstandings
Overusing `-a`
While the `-a` flag can save time, it’s essential to use it judiciously. Overcommitting can lead to committing unintended changes. Therefore, consider if all modified files should be included in the commit. When in doubt, it's often safer to `git add` specific files individually.
Misinterpreting Commit Messages
Vague commit messages can lead to confusion down the line. It’s important to provide context; for example, instead of writing "Fixed stuff", opt for "Fix crash when input is null". This clarity ensures that anyone reviewing the commit history can quickly understand the rationale behind decisions and changes.
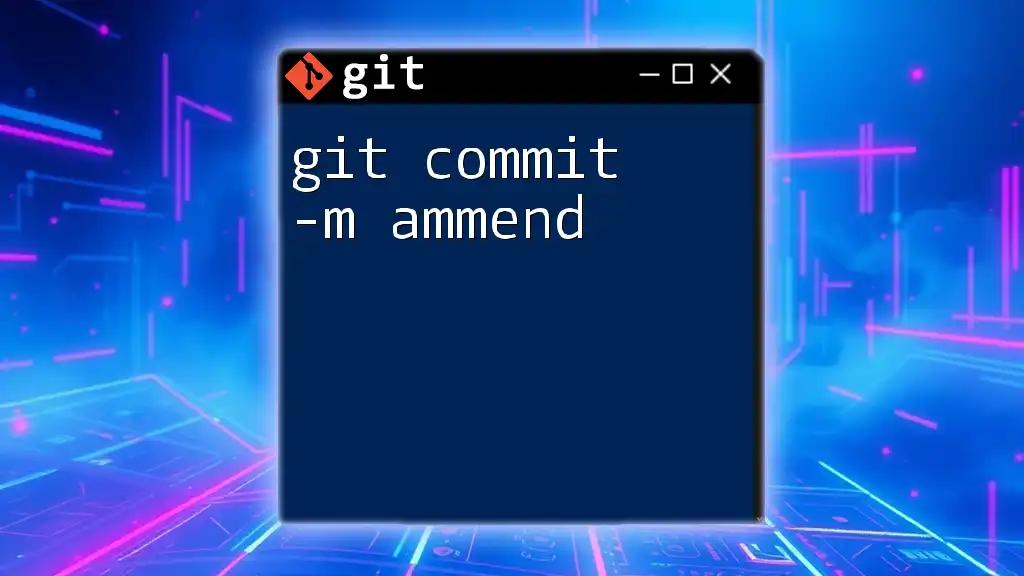
Conclusion
Recap of `git commit -a -m`
The command `git commit -a -m` is a powerful tool for managing your changes efficiently. By succinctly staging all tracked modifications and providing meaningful messages, you create not only a clean project history but also facilitate collaboration among team members.
Additional Resources
For more in-depth guides, best practices, and community-cluttered insights, consider checking the official Git documentation. Additionally, exploring further resources can help solidify your understanding of Git and enhance your productivity in using it effectively.