The `git commit -am` command is used to commit changes to the repository while automatically staging any tracked files that have been modified and allowing you to include a commit message in one concise command.
git commit -am "Your commit message here"
Understanding `git commit`
The `git commit` command is a fundamental aspect of using Git effectively. It is responsible for saving changes in your local repository. Whenever you execute a `git commit`, you are creating a new commit object in the Git history, capturing the current state of the project.
The basic syntax for `git commit` is as follows:
git commit [-options] [-m "commit message"]
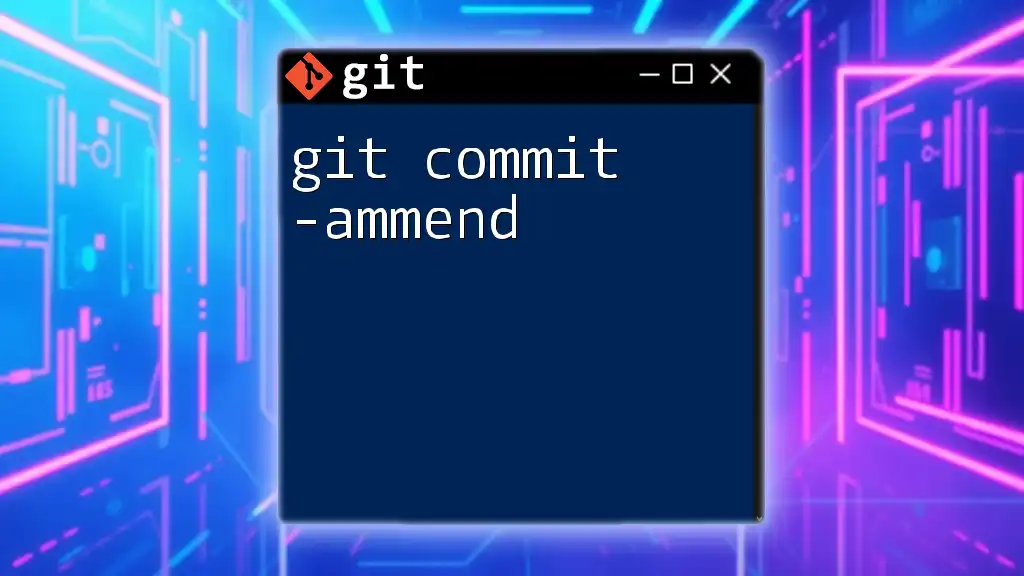
What Does the `-a` and `-m` Flag Do?
Overview of the `-a` flag
The `-a` flag, which stands for “all,” automatically stages tracked files that have been modified before creating the commit. This means that when you use `git commit -am`, you don’t need to run `git add` to stage changes to any already tracked files.
Using the `-a` flag is especially beneficial in scenarios where numerous files are being edited, and you want to avoid the extra step of staging them individually. It streamlines the workflow significantly.
Overview of the `-m` flag
The `-m` flag allows you to include a commit message directly in your command. Instead of opening the default text editor to write a message, you can write it inline. This is particularly useful for quick commits.
When writing commit messages, remember to follow best practices. Use clear and informative messages that describe the changes made. For example, instead of a vague message like “update,” opt for something more descriptive like “Fixed typo in README.” This approach helps you and your collaborators understand project history better.
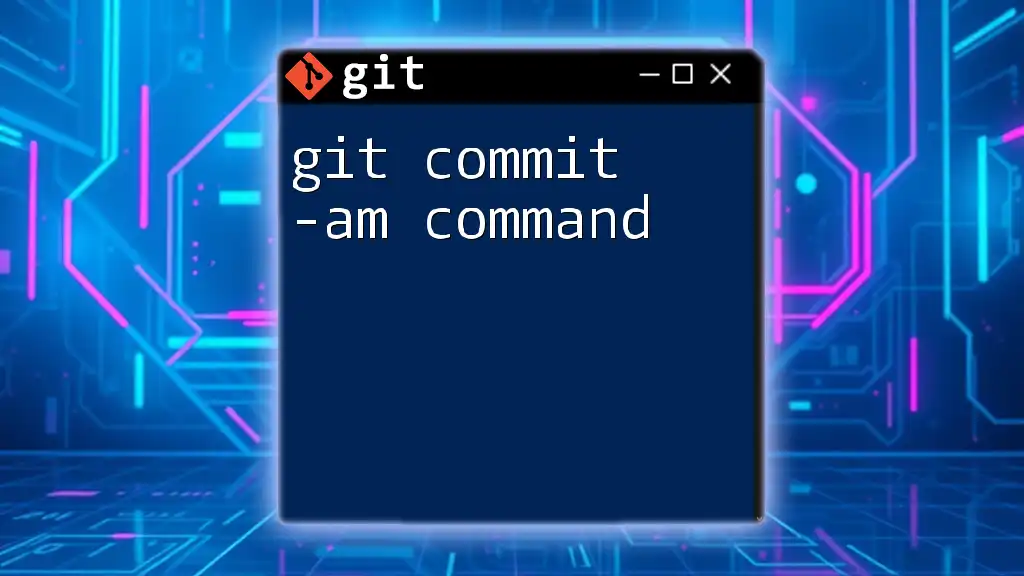
Using `git commit -am`: Syntax and Examples
Basic Usage
The basic usage of the `git commit -am` command looks as follows:
git commit -am "Fixed typo in README"
In this command:
- The `-a` flag stages all modified tracked files in the repository.
- The `-m` flag provides an inline commit message.
After running this command, the changes are committed, and you have a clear message recorded in your project’s history.
Handling Untracked Files
It’s essential to note that the `-a` flag only stages tracked files. If you have new untracked files, they won’t be included when you run `git commit -am`.
For example, if you create a new file `new_feature.txt`, you cannot just run:
git commit -am "Added new feature"
This command will not recognize `new_feature.txt` because it has not been tracked yet. Instead, you'll need to stage untracked files first:
git add new_feature.txt
git commit -am "Added new feature"
By executing `git add`, you inform Git to start tracking the file, allowing it to be captured in your commit.
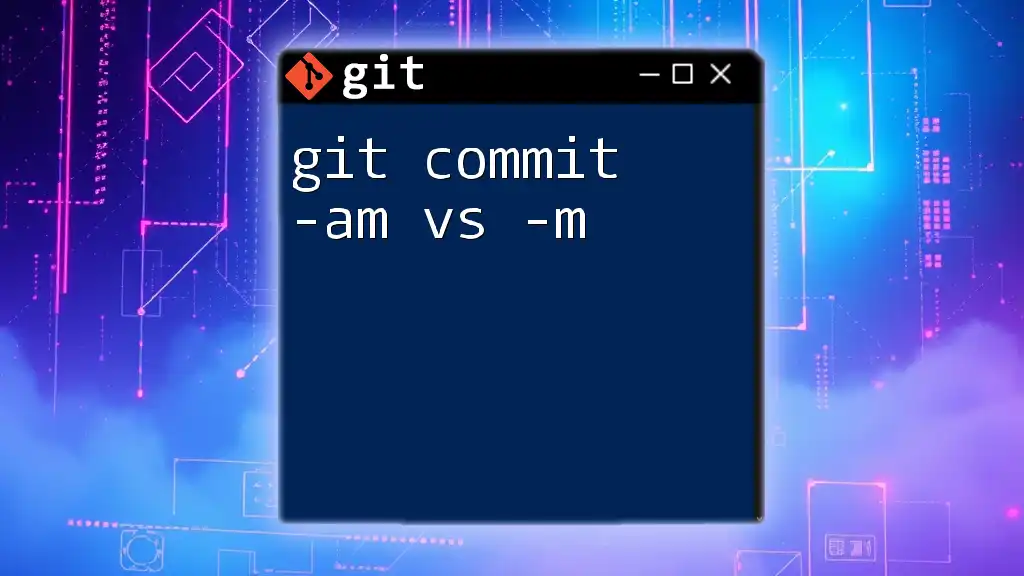
Advantages of Using `git commit -am`
Efficiency in Development
One of the primary advantages of using `git commit -am` is its efficiency. By consolidating the staging process and the commit into a single command, developers can save time, especially during rapid iterations in their workflow. Instead of executing multiple commands, you can perform a quick commit in just one go.
Reducing Errors
Using `git commit -am` reduces the likelihood of errors that can happen when entering multiple commands. For instance, if you forget to stage files before committing, your changes won’t be saved, potentially leading to confusion and lost work. By staging and committing in one step, you minimize these risks.
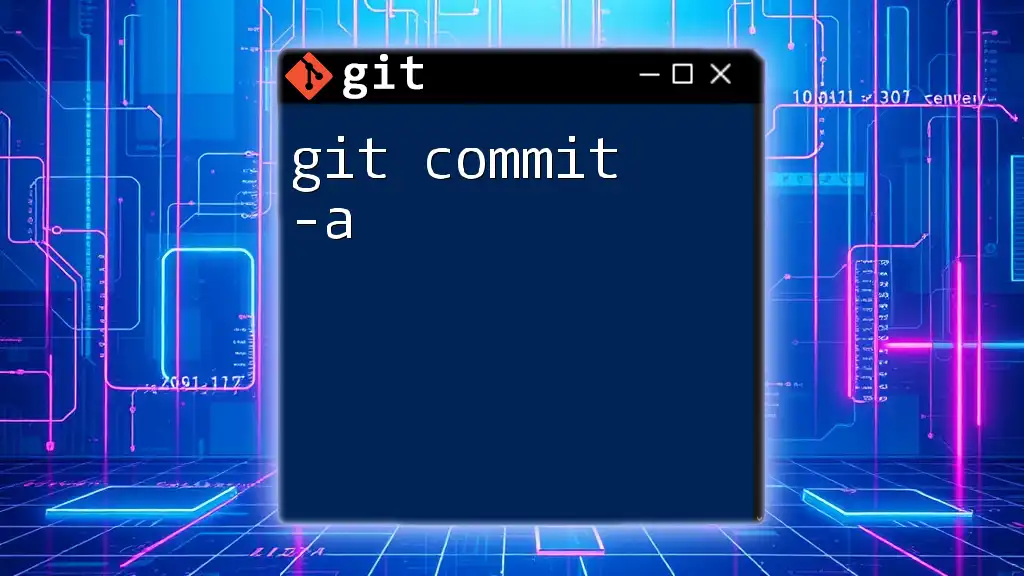
Caveats and Best Practices
Situations to Avoid `-am`
While `git commit -am` is convenient, there are instances where it may not be the ideal choice. For example, if you want to be selective about which changes to stage and commit, you should avoid using `-a`.
Consider the following situation: You modified two files, but only want to commit changes made in one. In this case, you should run:
git add <specific_file>
git commit -m "Updated feature X"
This approach allows for more precise control over what is being committed.
Commit Granularity
Another best practice is to focus on commit granularity. Aim for small, atomic commits that encapsulate a single change or improvement. This practice not only makes it easier to track project history but also simplifies the process of pinpointing issues when they arise.
When breaking down larger changes, you could use multiple small commits like so:
git commit -am "Initial setup for Feature Y"
git commit -am "Updated styling for Feature Y"
git commit -am "Implemented functionality for Feature Y"
These commits allow reviewers and collaborators to understand changes step-by-step.
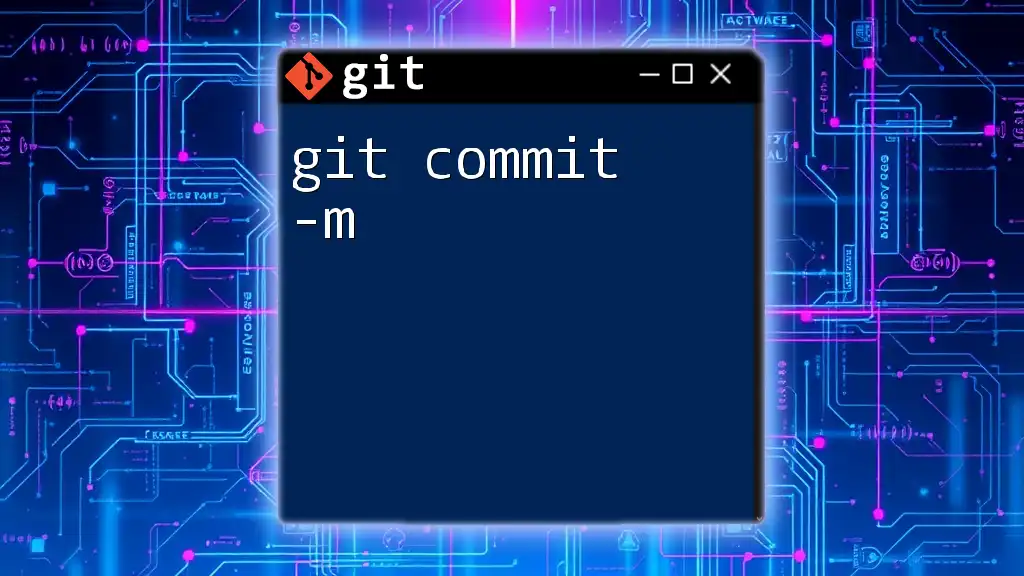
Conclusion
In summary, the `git commit -am` command provides a powerful means of streamlining the commit process, combining both staging and committing in one step. It enhances development efficiency, reduces the risk of errors, and maintains a clear project history through descriptive commit messages.
Practicing the use of `git commit -am` within your daily version control tasks can significantly improve your Git workflow. Don’t hesitate to experiment with this command and integrate it into your project practices.
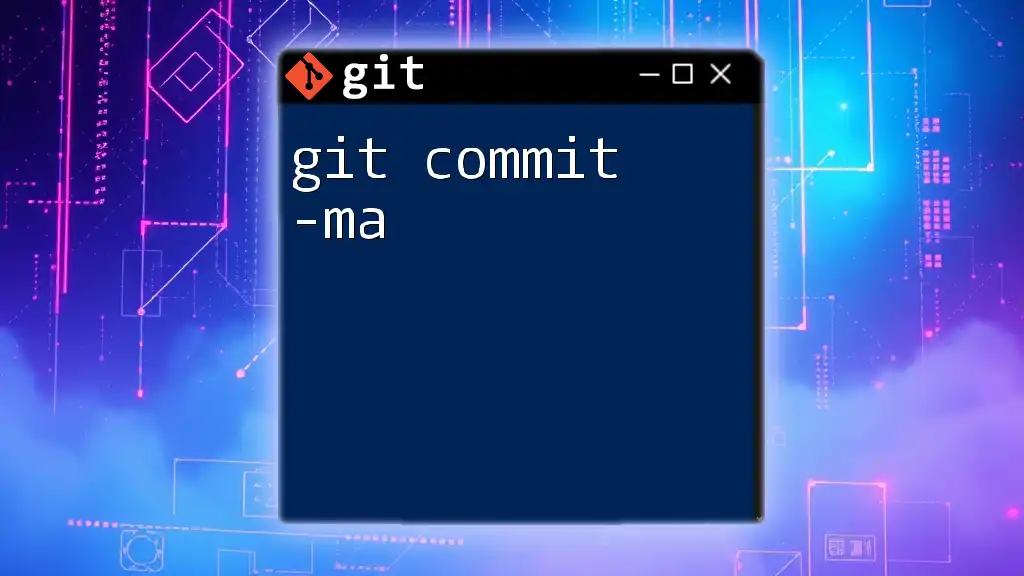
Additional Resources
For those looking to deepen their understanding of Git, further readings on Git best practices are available. Consider exploring tools and plugins that can enhance your Git experience and support your version control journey.
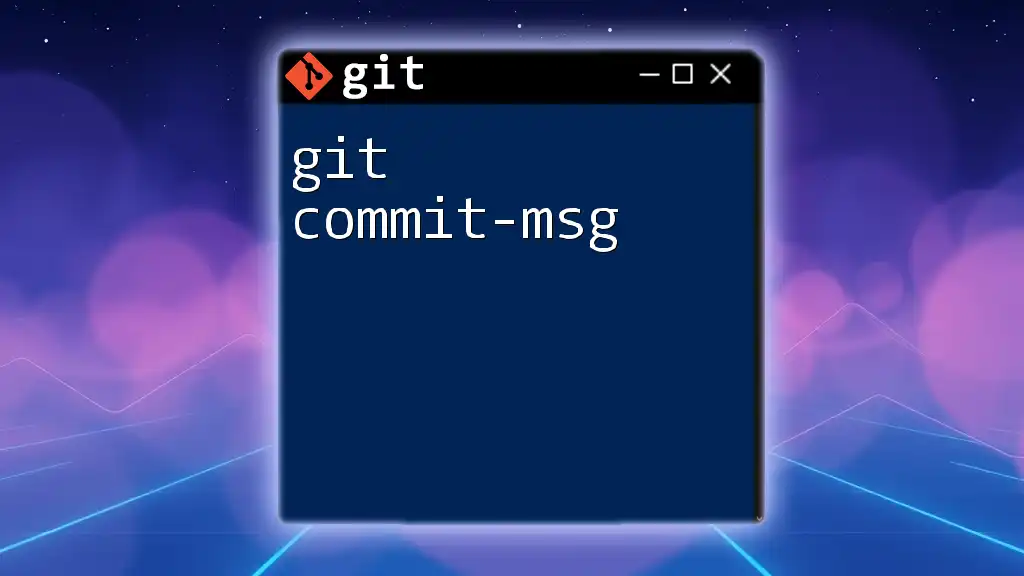
Frequently Asked Questions (FAQs)
Can I combine `git commit -am` with other flags?
Yes, you can combine the `-a` and `-m` flags with others. For example, adding the `-v` flag will show the diff of changes in the commit message.
Is it safe to use the `-a` option on a large repository?
It is generally safe to use the `-a` option, but be mindful of performance considerations. If you are working with a very large repository, you may want to selectively stage your changes to ensure better control and oversight over what you're committing.