The `git commit -am` command allows you to stage all modified files and commit them to the repository in a single step, along with a commit message in one concise command.
git commit -am "Your commit message here"
Understanding Git Commit
What is a Git Commit?
A commit serves as a snapshot of your project at a specific point in time. In essence, it’s a record of the changes you've made to the codebase. Every time you commit, you are essentially creating a link in the project's history, allowing you to track changes, revisit previous versions, and facilitate collaboration with other developers.
The Role of Commit Messages
Commit messages provide essential context and reasoning behind changes made. It's important to communicate the purpose of your changes clearly. Following best practices for writing effective commit messages, such as keeping them concise, using the imperative mood, and summarizing the changes, enhances collaboration and maintenance of the project.
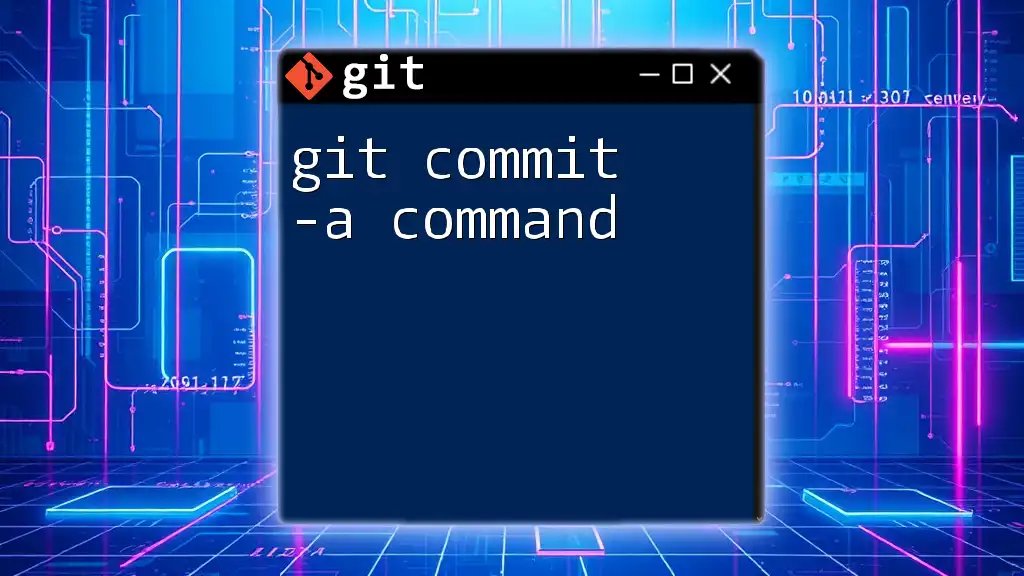
The `git commit` Command
Basic Syntax of the Command
The standard form of the `git commit` command involves providing a message using the `-m` flag. This is how you communicate what changes have been made in that commit.
git commit -m "Your commit message"
Commonly Used Flags with `git commit`
Besides the `-m` flag, there are other commonly used flags when committing changes. For instance:
- `-m`: This allows you to include a message directly in the command line, making it quick and efficient.
- `-a`: This flag stages all modified and tracked files automatically before committing them, which can save time.
Using these flags effectively can streamline your workflow, particularly when making multiple changes in one go.
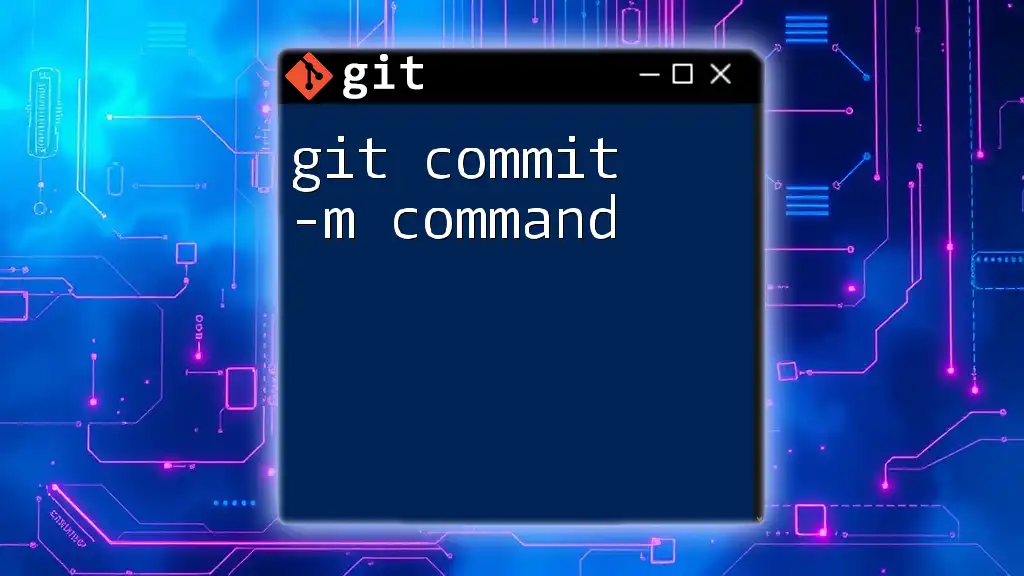
The `git commit -am` Command
What Does `git commit -am` Do?
The `git commit -am` command is a powerful utility in Git that allows you to both stage and commit changes in a single command. This command is particularly useful for developers who frequently update existing files and want to keep their workflow efficient.
Example Command
git commit -am "Updated the README file and fixed typos"
In this command, `-a` automatically stages all modified files, while `-m` allows you to include a commit message directly.
Understanding Each Component
The `-a` Flag
The `-a` flag is a shortcut that stages all tracked files that have been modified. However, it is essential to note that this flag does not track new files. In other words, any files that were newly created will not be included in the commit unless you explicitly stage them using `git add`.
Consider this example:
git add file1.txt file2.txt # traditional staging
git commit -a -m "Updated files without staging manually"
Here, only modifications to `file1.txt` and `file2.txt` will be committed. Newly created files would need a separate `git add`.
The `-m` Flag
Including the `-m` option is essential for defining commit messages inline. If you omit this flag, Git will open your default text editor for you to write a commit message, which can interrupt your flow if you’re aiming for speed.
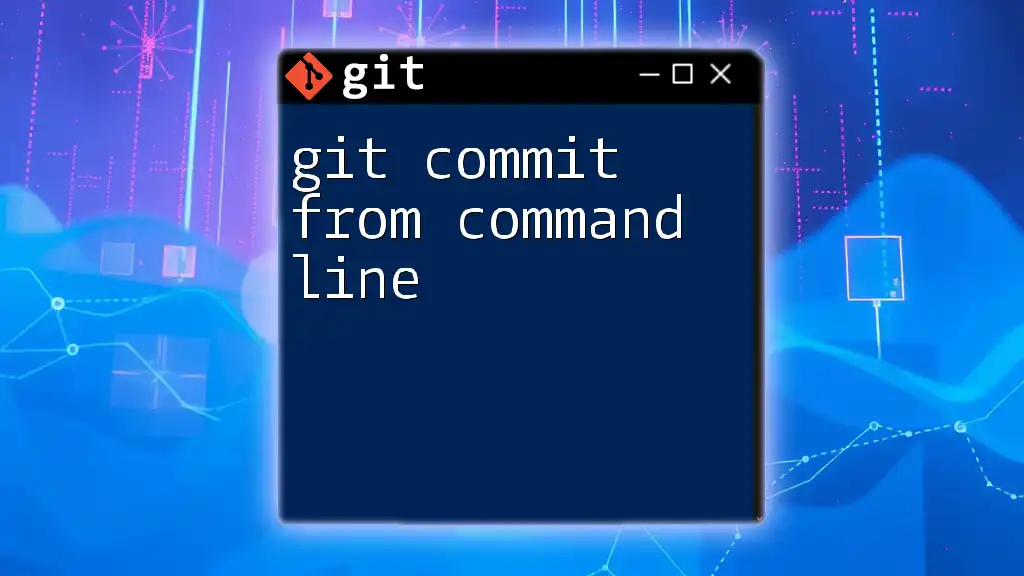
When to Use `git commit -am`
Advantages of Using `-am`
One of the main advantages of the `git commit -am` command is its ability to save time. By combining the staging and committing process, experienced users can streamline their workflow, especially during daily tasks where rapid updates are common.
Scenarios Best Suited for `-am`
- Quick Fixes: When making small changes or bug fixes, this command is ideal for efficiently committing your updates.
- Routine Tasks: It’s perfect for frequent commits throughout a workday, keeping your commit history clean and concise.
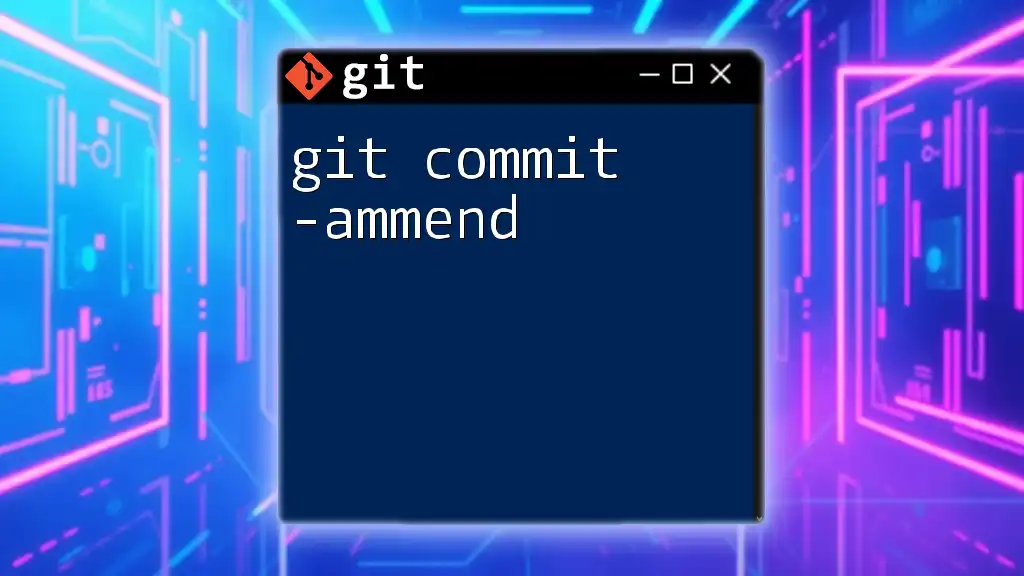
Limitations of `git commit -am`
Limitations of the `-a` Flag
While the `-a` flag is a powerful feature, it does come with limitations. Specifically, it does not stage newly created files. Therefore, if you've added new files to your project, you cannot rely solely on `git commit -am`.
For instance:
touch newfile.txt # creates a new file
git commit -am "Adding new file" # Won't include newfile.txt
In this case, the newly created file `newfile.txt` will not be committed, as it was never staged.
Recommendations for New Users
For those new to Git, it's recommended to become familiar with staging changes explicitly using `git add` before committing. This method helps build a deeper understanding of the version control system and encourages meticulous practices.
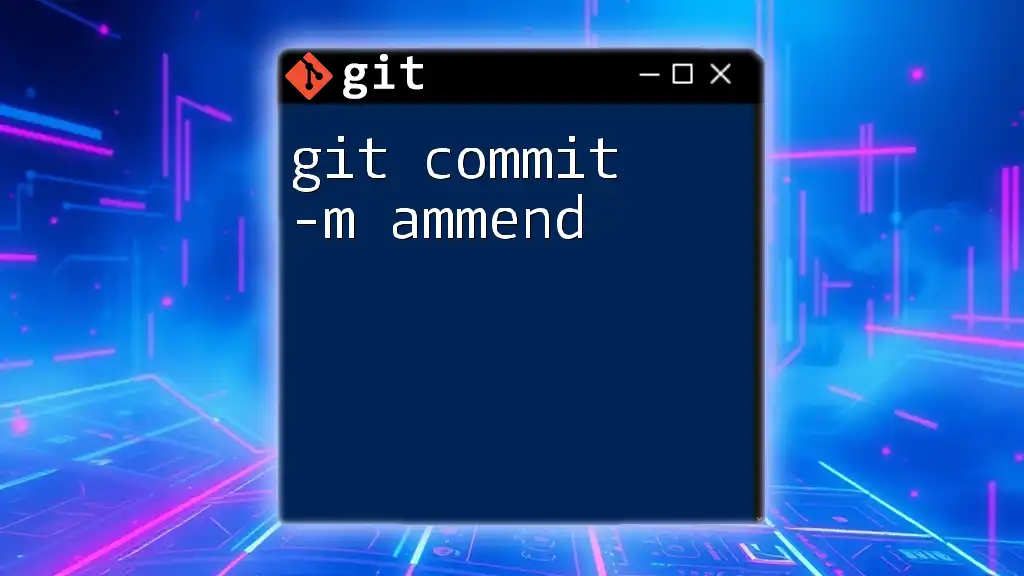
Best Practices for Using `git commit -am`
Structuring Your Commit Messages
An effective commit message can dramatically improve your project's documentation. Some tips include:
- Be Concise: Aim for brevity without losing meaning.
- Use Imperative Mood: Write messages as commands, e.g., "Fix bug" or "Update documentation".
- Summarize Changes: Provide a brief overview of what changes were made and why.
Combining Flags for Optimal Workflow
To maximize your productivity, consider combining the `-a` and `-m` options when committing frequently updated files. However, remain diligent about staging new files separately to maintain accuracy in your project’s commit history.
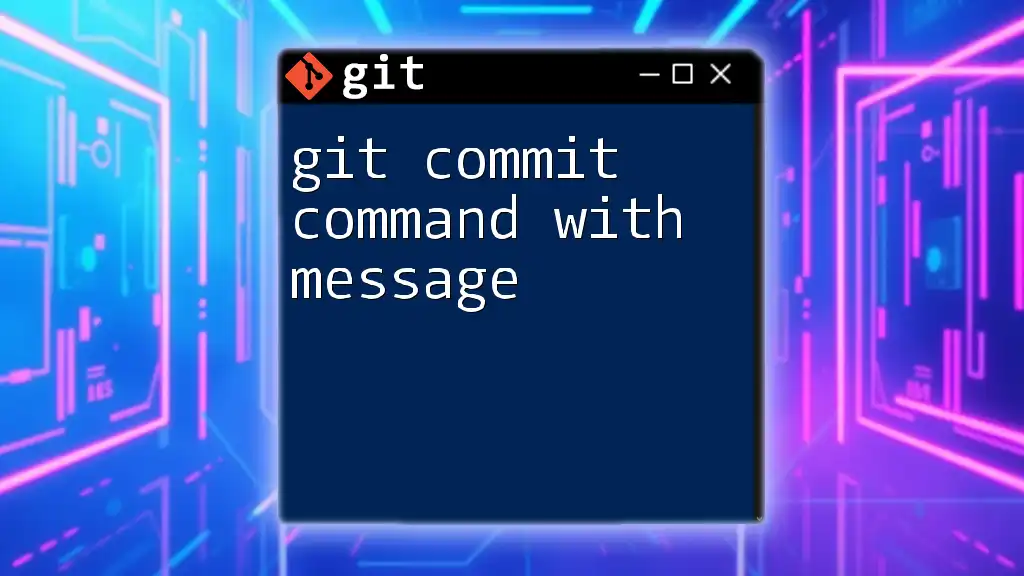
Conclusion
The `git commit -am` command is a significant tool that can speed up your workflow by combining file staging and committing into a single command. Understanding its capabilities and limitations will empower you to use Git more efficiently, making your development process smoother and more productive.
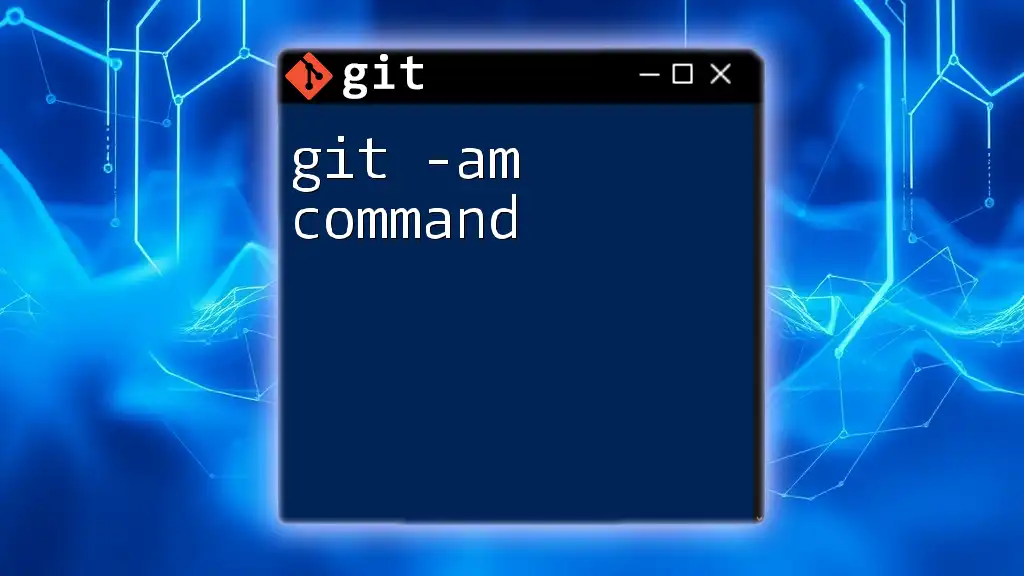
Additional Resources
Official Git Documentation
For further reading and advanced concepts, refer to the official Git documentation. This will provide comprehensive details on all Git commands and their uses.
Tutorials and Guides for Beginners
Consider exploring tutorials and guides geared towards beginners to strengthen your understanding of Git and version control practices. This foundational knowledge will be invaluable as you navigate the complexities of collaborative coding environments.