The `git commit -a` command automatically stages all modified and deleted files before creating a commit, streamlining the process of committing changes in a Git repository.
Here's the command in a code snippet:
git commit -a -m "Your commit message here"
What is `git commit`?
The `git commit` command is a fundamental component of Git, playing a critical role in version control. When you commit changes, you're essentially creating a snapshot of your project's current state, allowing you to track and manage modifications over time. Each commit captures the modifications made to files in the repository and is identified by a unique hash.
Importance of Commit Messages
Commit messages are a crucial aspect of the `git commit` process. They describe the changes made, providing context for other developers (and your future self) about what was done and why. A well-written commit message can significantly improve code clarity and maintenance.
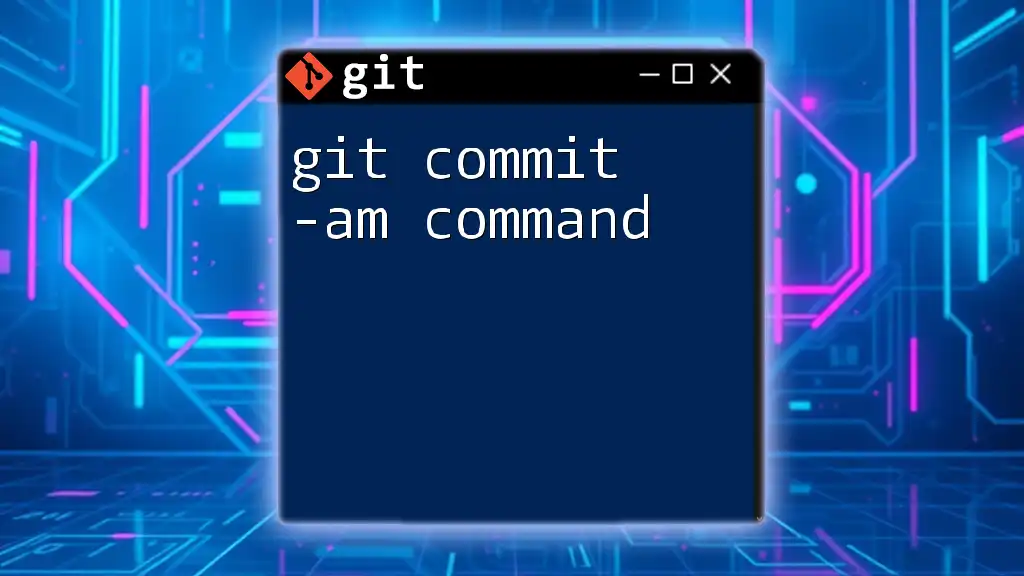
Understanding the `-a` Option
What Does `-a` Stand For?
The `-a` option stands for "all." When used with the `git commit` command, it tells Git to automatically stage any tracked files that have been modified or deleted before creating the commit. This streamlines the commit process by eliminating the need to manually stage these changes.
Difference Between `git commit` vs. `git commit -a`
Using `git commit` without the `-a` flag requires explicit staging of changes using the `git add` command. This means that only files that have been staged will be included in the commit. Conversely, the `git commit -a` command simplifies this process, automatically staging all modifications to currently tracked files, making it a more efficient choice for many workflows.
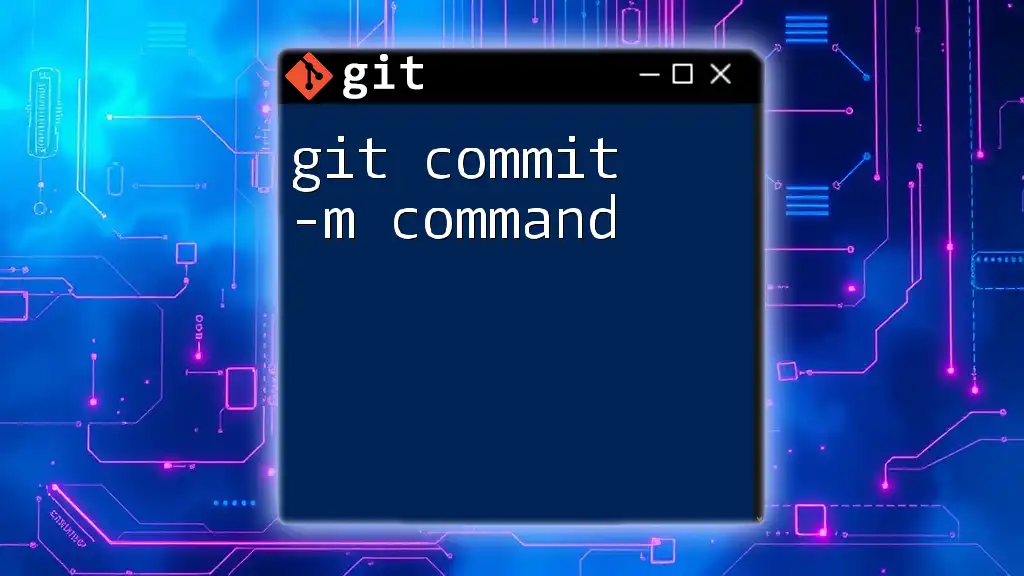
How to Use the `git commit -a` Command
Syntax of the Command
The basic structure of the `git commit -a` command is as follows:
git commit -a -m "Your commit message here"
Here, `-m` allows you to add a commit message directly in the command, streamlining the process.
Step-by-Step Guide
Consider a scenario where you've modified several tracked files in your project. Here’s how to use `git commit -a` effectively:
- Making Changes to Tracked Files: You make the necessary changes to your code files.
- Executing the Command: Use the command:
This command stages all modified and deleted files (but not untracked files) and commits them with the provided message.git commit -a -m "Updated README and fixed bugs in main.py"
In the background, Git records the changes, and a new commit snapshot is created in your project history.
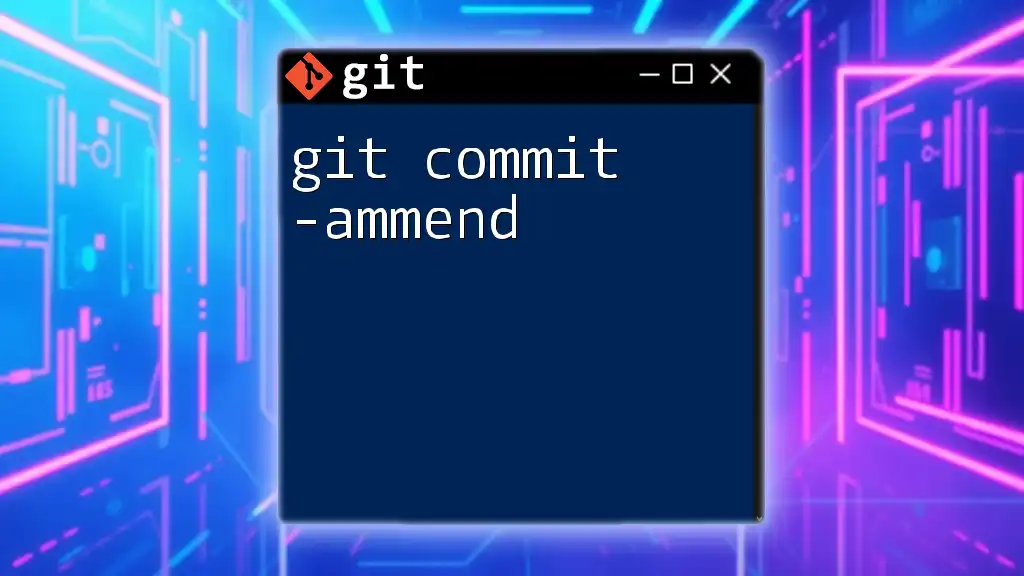
Practical Examples
Example 1: Basic Usage
Let's say you've just tweaked your `README.md` file and fixed a couple of bugs in `main.py`. To commit these changes, you would execute:
git commit -a -m "Updated README and fixed bugs in main.py"
In this example, both modified files are committed in one step, saving you the hassle of staging them manually.
Example 2: Committing Changes with Multiple File Types
Imagine you are working on a project containing various file types, such as Python and JavaScript files. After modifying both `.py` and `.js` files, you can use the same command:
git commit -a -m "Refactored code in main.py and updated app.js for compatibility"
This approach ensures that all modified tracked files across different formats are committed together, maintaining a cohesive project history.
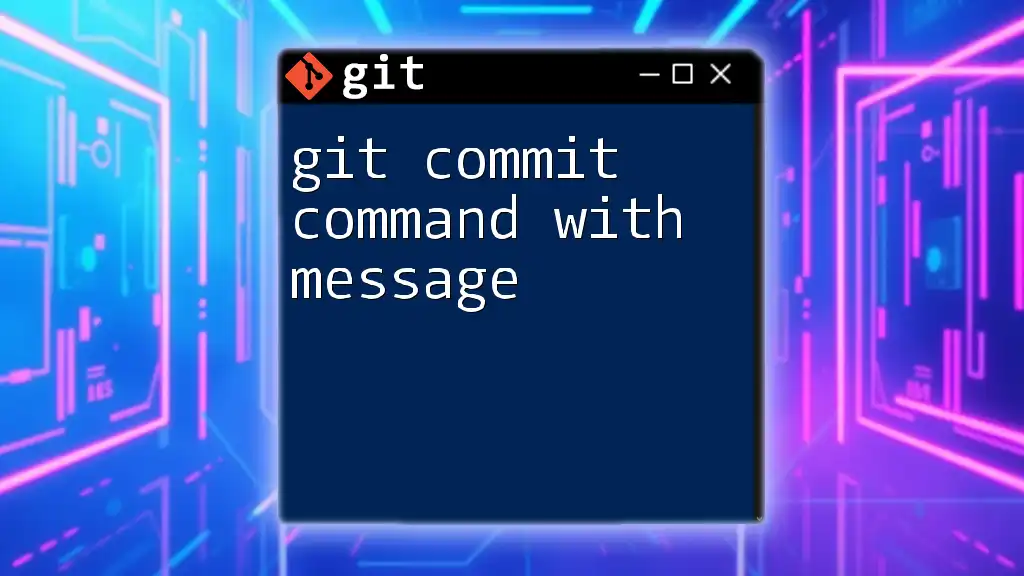
Customizing Your Commit
Adding Commit Messages
Crafting clear and concise commit messages is essential. A good commit message typically follows this format:
- Header: A short summary of the changes (50 characters or less).
- Body: An optional detailed description, explaining what changes were made and why.
Best practices for writing commit messages include:
- Use the imperative mood (e.g., "Fix bug in feature" instead of "Fixed bug in feature").
- Be specific about what was changed and why.
Example: A good commit message might look like:
git commit -a -m "Add error handling to user authentication"
Checking Status Before Committing
Before making a commit, it’s wise to check your changes. Use the `git status` command to review what modifications are staged and unstaged:
git status
This command provides a clear overview of your working directory and the staging area, ensuring that you're aware of what will be included in the commit.
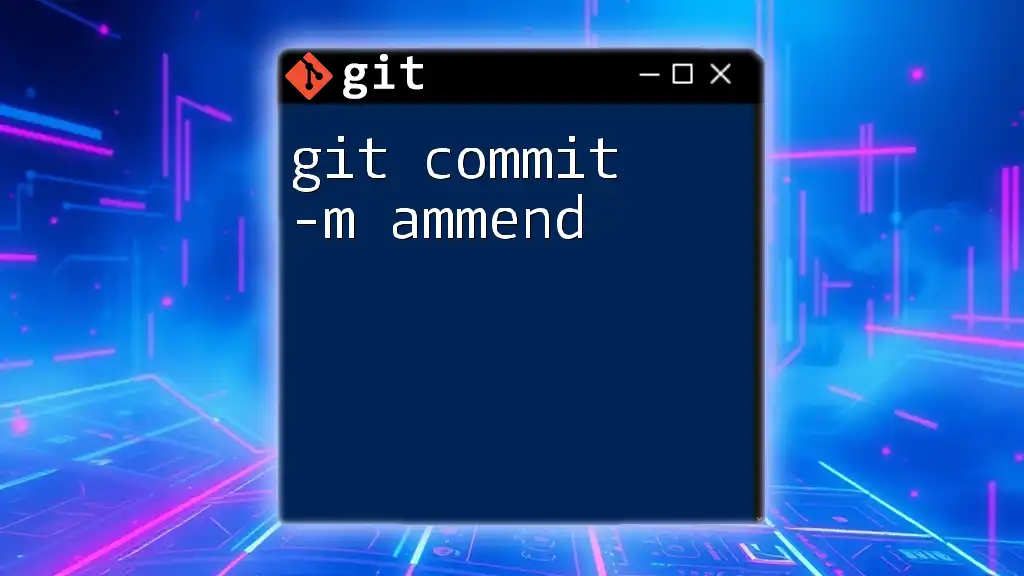
Common Mistakes and Troubleshooting
Forgetting to Stage New Files
One common oversight is assuming that `git commit -a` will include newly created files. Remember, `-a` only stages changes to tracked files. If you have new files that aren’t yet tracked, they won’t be included in the commit. To add untracked files, you need to use the `git add` command:
git add [filename]
After adding new files, you can safely run the `git commit -a` command to commit changes to tracked files.
Misunderstanding the Impact of `-a`
It’s crucial to recognize what `-a` does not do. It will not stage:
- Untracked files.
- Files that were newly created and not yet added to the repository.
Understanding this distinction helps avoid surprises when committing code.
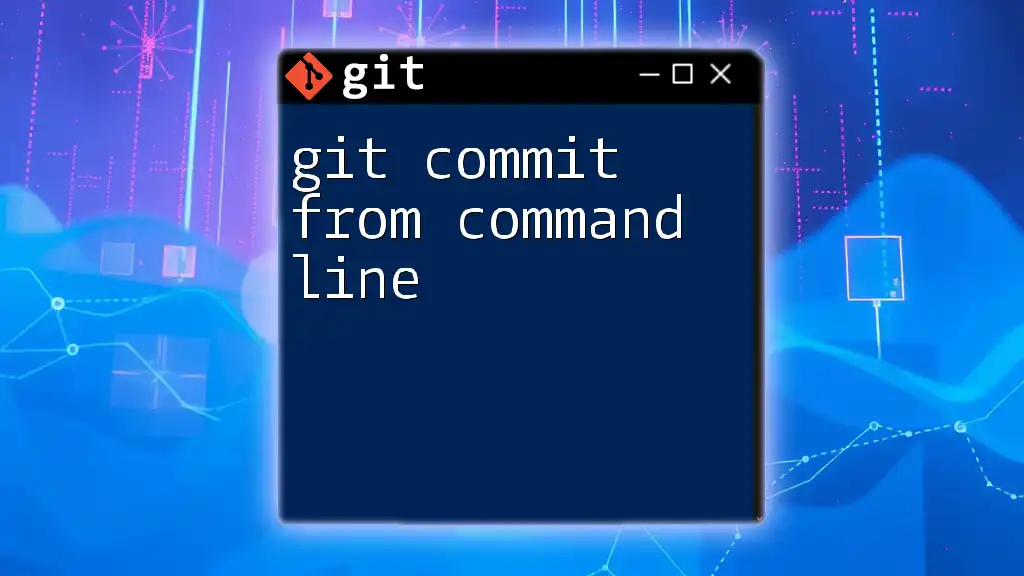
When Not to Use `git commit -a`
While `git commit -a` is efficient, it may not always be the best choice. There are scenarios where you should consider using `git commit` without `-a`:
- When you need to selectively stage changes: If you want granular control over which changes to include in a commit, using `git add` allows you to stage individual files selectively.
- For new or untracked files: Opt for staging new files manually with `git add` before committing.
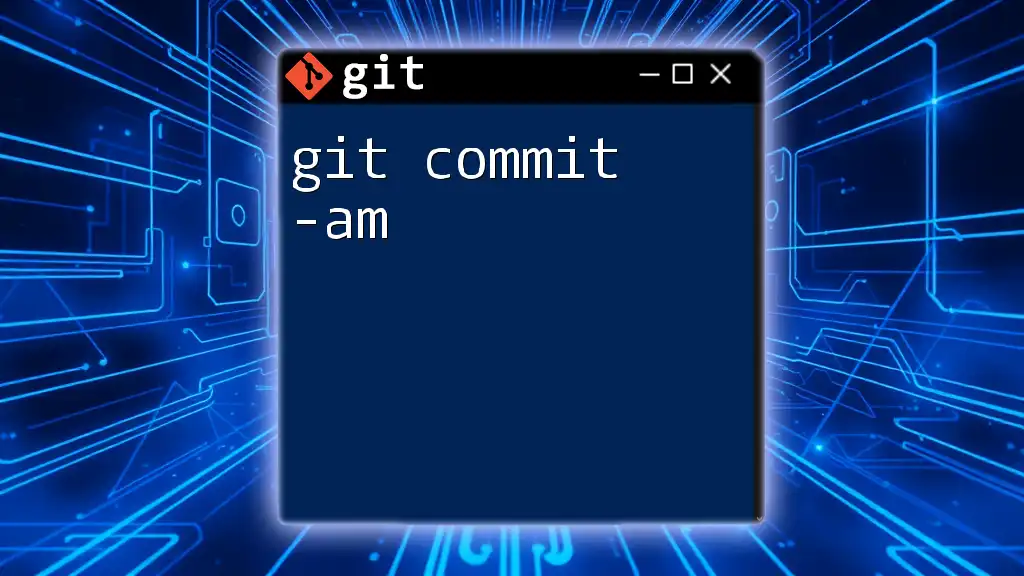
Conclusion
The `git commit -a command` is a powerful tool that simplifies the commit process by automatically staging tracked changes. With a firm understanding of its functionality, you can streamline your workflow and enhance your coding efficiency. Remember to craft meaningful commit messages, review your changes, and use the command wisely in conjunction with other Git commands.
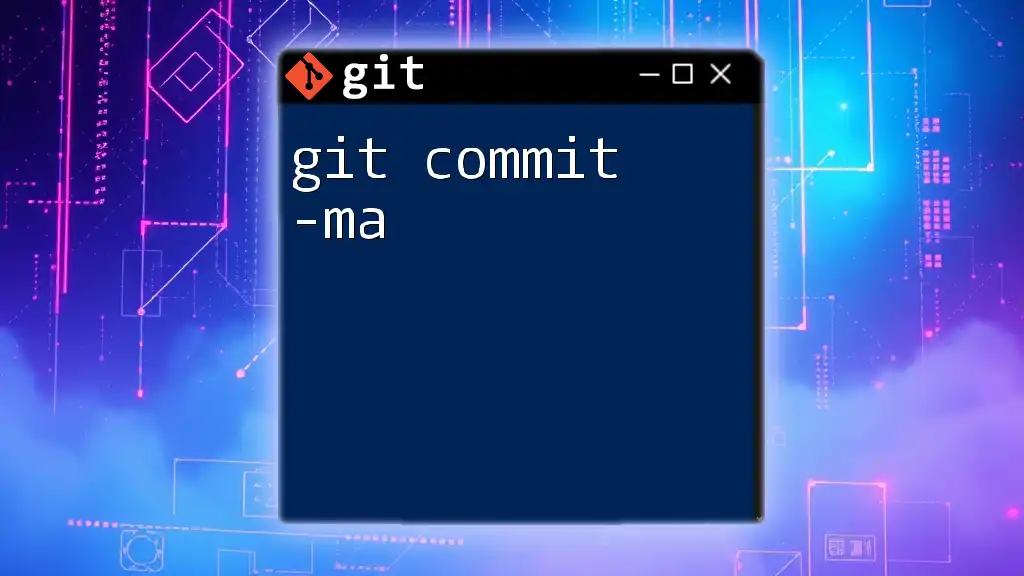
Additional Resources
To deepen your understanding of Git and its commands, consider exploring the following resources:
- Git's official documentation
- Online tutorials for practical experience
- Books focused on version control best practices
By leveraging these resources, you'll further sharpen your skills and become more proficient in using Git effectively.