The `git commit -n` command allows you to commit changes without running the pre-commit hooks, which can be useful when you want to bypass any checks or automated processes during the commit.
git commit -n -m "Your commit message here"
What is `git commit`?
Definition of `git commit`
The `git commit` command is a critical part of the Git version control system. It is used to save changes in the local repository while creating a snapshot of the current state of the project. Each commit serves as a checkpoint that allows developers to track the progression of the code over time.
A well-structured commit message is essential for maintaining an understandable history of changes. This helps both current and future team members to understand the evolution of the project and the reasoning behind certain decisions.
Basic Syntax of `git commit`
The general syntax for the `git commit` command includes the `-m` option, which allows the user to add a commit message directly in the command line:
git commit -m "Your commit message here"
Using clear and descriptive messages enhances collaboration and code review processes.
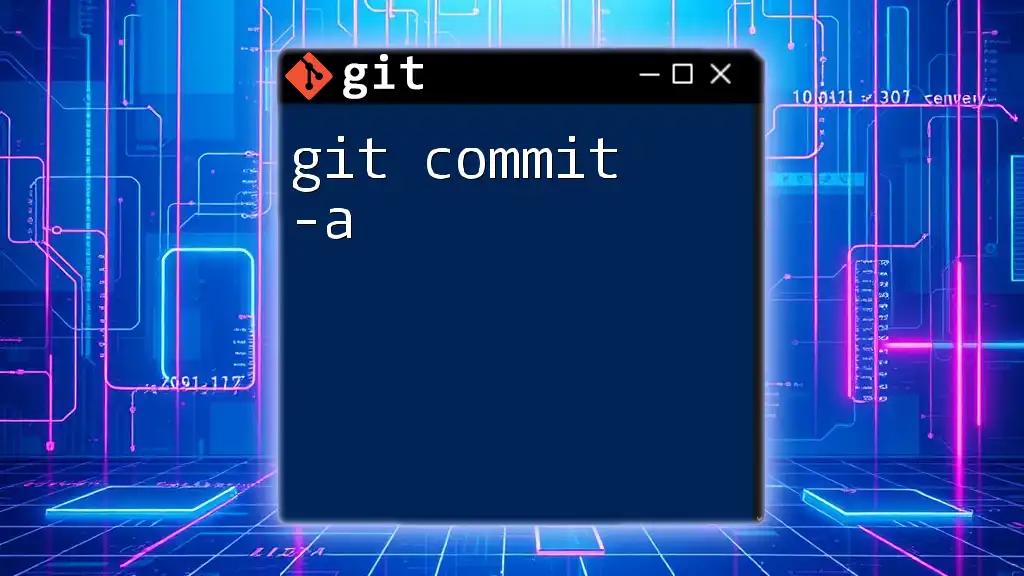
Understanding the `-n` Option
What Does `-n` Stand For?
The `-n` option is shorthand for `--no-verify`. This command tells Git to skip any pre-commit hooks that may be set up in the repository. Pre-commit hooks are scripts that run automatically before a commit is finalized, which can include tasks like running linters, running tests, or enforcing certain coding standards.
When to Use `git commit -n`
There are specific scenarios in which using `git commit -n` can be advantageous:
-
Rapid Local Testing: During a fast-paced development cycle, developers may want to quickly commit changes without waiting for hooks to execute. This can be especially useful for prototyping or testing.
-
Bypassing Hook Errors: If pre-commit hooks are malfunctioning or producing false errors, using `-n` can allow you to commit code quickly so you can address the issues later.
How to Use `git commit -n`
Using the command is straightforward. By appending `-n`, developers can finalize their commits while ignoring the hooks:
git commit -n -m "Your commit message without pre-commit hooks"
This command commits your changes, but it skips any pre-defined checks or scripts that could otherwise interrupt the workflow.
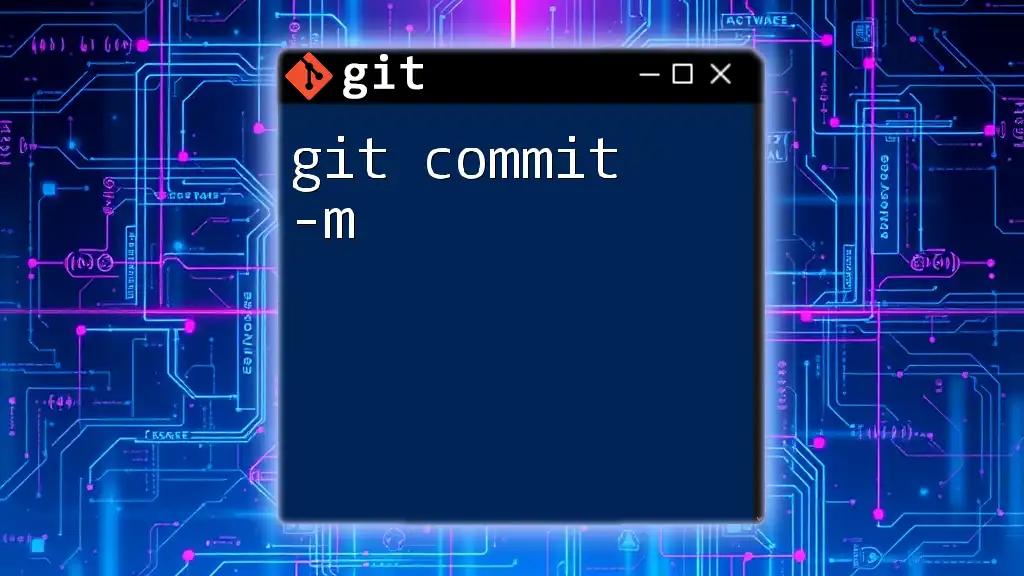
Pre-commit Hooks Explained
What Are Pre-commit Hooks?
Pre-commit hooks are custom scripts that get executed before a commit is finalized. They help enforce coding standards, run tests, and prevent problematic commits. Hooks play a vital role in maintaining the quality and integrity of the codebase.
Examples of Common Pre-commit Hooks
Common examples of pre-commit hooks include:
- Linting: Running a linter can identify problems in code style or potential errors before any code is committed.
- Tests: Automatically running unit or integration tests to ensure the new code doesn’t introduce any bugs.
These hooks enhance code quality, but they can also create bottlenecks if not appropriately managed.
How Hooks Can Impact Your Workflow
While pre-commit hooks do improve the coding process by maintaining quality, they can also lead to development slowdowns. Failing to pass the checks can cause frustration, especially in a fast-paced environment where rapid iterations are necessary. Thus, understanding when and why to use them is essential for efficient development.
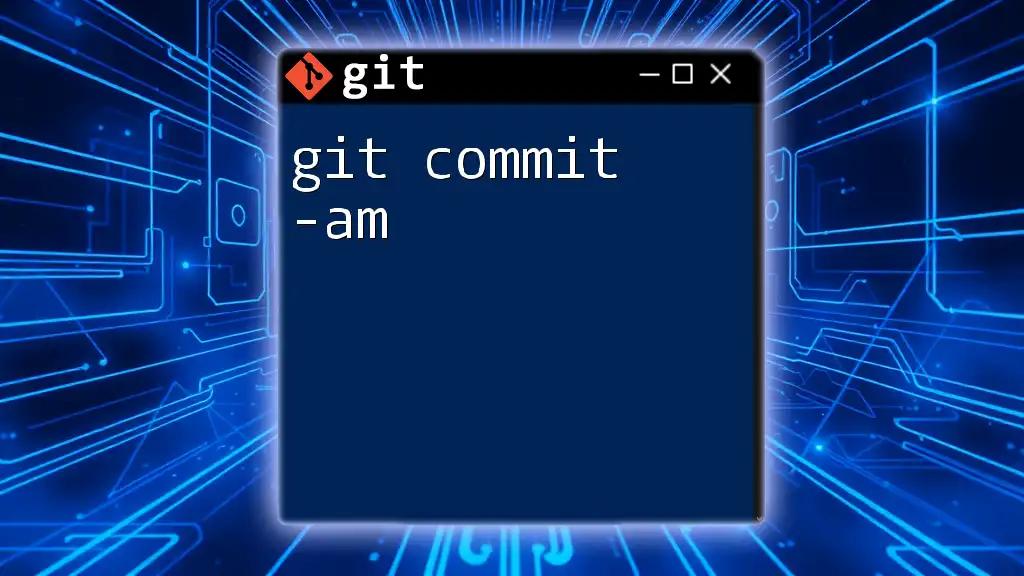
Advantages and Disadvantages of Using `git commit -n`
Advantages
Using `git commit -n` can provide several benefits:
- Quick Commits: It allows for rapid commits during development, which can be critical when timely iterations and feedback are needed.
- Flexibility: Developers working on personal projects or experimental features may prefer the ability to bypass hooks.
- Work-in-Progress Commits: Can be useful to commit incomplete or draft changes without interruptions.
Disadvantages
However, skipping hooks comes with risks:
- Compromised Code Quality: By ignoring automated checks, you risk introducing bugs or infringing coding standards.
- Collaboration Issues: In a collaborative environment, using `-n` recklessly can lead to other team members facing unexpected issues when they pull the latest changes.
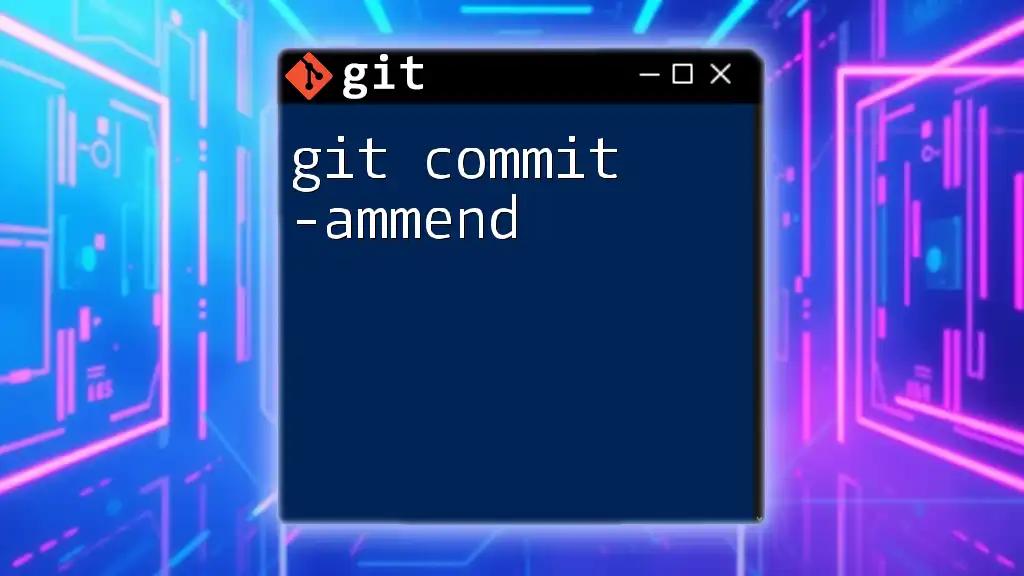
Best Practices for Using `git commit -n`
Using with Caution
It's crucial to use `git commit -n` judiciously. Understand the implications of skipping pre-commit hooks. Develop a habit of reviewing and cleaning up your code after you’ve utilized this command. Balancing the urgency of development with the need for high-quality code is key.
Alternatives to Skipping Hooks
Instead of skipping hooks entirely, consider these alternatives:
- Creating Temporary Branches: Use a separate branch to make experimental changes without affecting the main codebase.
- Partial Commits: Leverage staging areas to commit only specific changes while avoiding problematic aspects.
These alternatives allow for flexibility without sacrificing integrity.
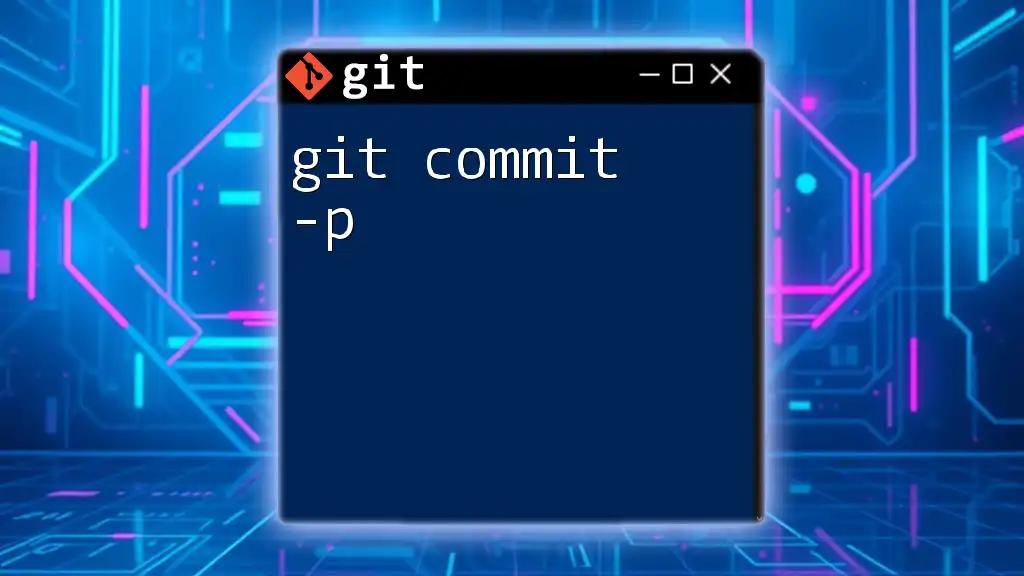
Conclusion
In summary, `git commit -n` is a powerful tool that can accelerate your development process, but it should be wielded with care. Understanding when it's appropriate to skip hooks, while also recognizing the potential trade-offs, is essential for navigating the complexities of version control effectively. Striking a balance between speed and quality will ultimately lead to a more healthy and maintainable codebase.
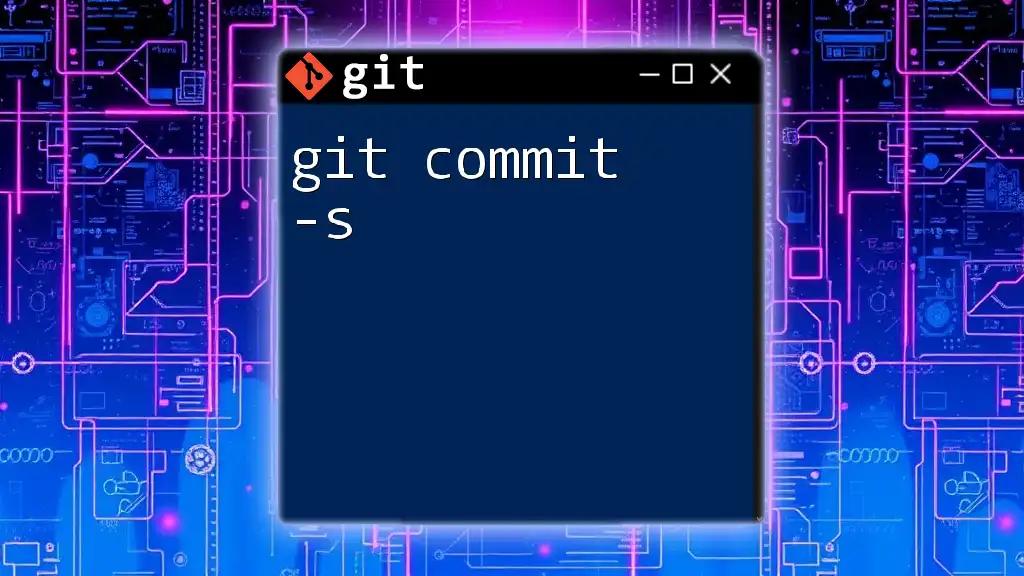
References and Further Reading
To deepen your understanding of `git commit -n` and best practices in Git, explore the official Git documentation and consider accessing resources such as books or online tutorials on version control strategies.
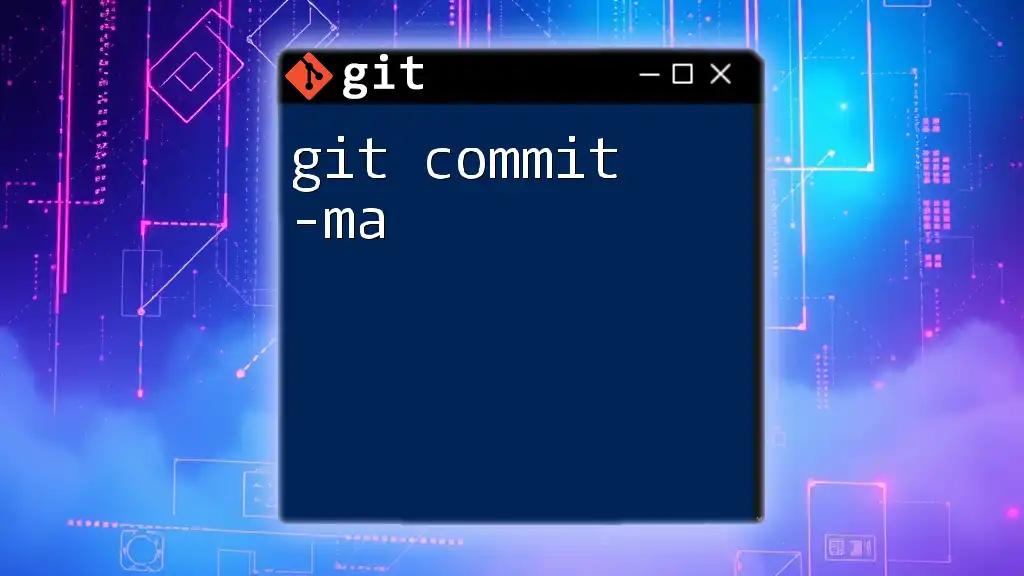
FAQ Section
Common Questions About `git commit -n`
-
What happens when I use `git commit -n`? Using `git commit -n` allows you to skip the execution of pre-commit hooks, meaning your changes are committed without running any scripts that could validate or enforce code standards.
-
Can I still use hooks later? Yes, you can still utilize hooks in future commits. Using `-n` does not remove or disable the hooks; it only bypasses them for that particular commit.
-
Is it safe to skip hooks in a collaborative environment? It can pose risks to your project’s quality and stability. Use this option thoughtfully and preferably within personal development contexts or when you're running very controlled tests.