The `git commit-msg` is a hook that allows you to enforce a format for commit messages before they are recorded in the repository.
#!/bin/sh
# commit-msg hook script to enforce message format
if ! grep -q '^\[JIRA-[0-9]\+\]' "$1"; then
echo "Commit message must start with a JIRA ticket reference, e.g., [JIRA-123]: Your message"
exit 1
fi
What is `git commit-msg`?
A commit message is a crucial part of version control that explains what changes have been made in a commit. It serves as a time-stamped record that allows team members—or even your future self—to understand the reasoning behind a change. A well-written commit message is vital for effective collaboration and helps maintain clarity in a project's history.
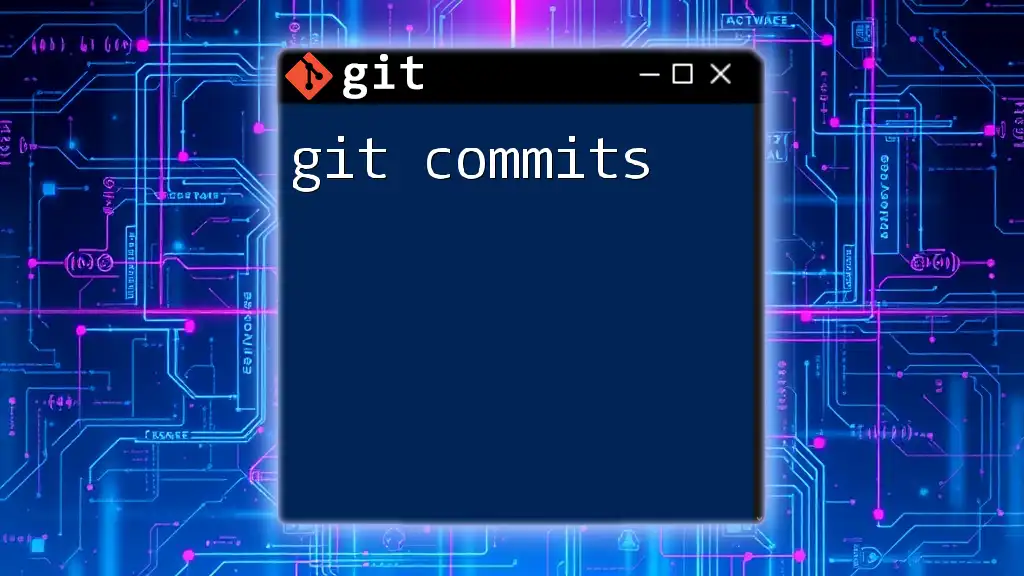
The Anatomy of a Commit Message
Structure of a Commit Message
A typical commit message consists of three components:
- Summary Line: A concise overview of the changes. Aim for a length of about 50 characters.
- Body: An optional section that elaborates on the changes, why they were made, and any additional information that might be helpful.
- Footer: This can include references to issue numbers, task IDs, or notes about breaking changes.
Example of a Well-Structured Commit Message
Here’s an example of how to structure a commit message effectively:
feat(authentication): add login functionality
- Added login feature using JWT for user authentication.
- Updated user model with a new email field.
- Ensured validation checks for login input.
Closes #123
In this example, the summary line is precise and informative, while the body provides context and details about what was changed and why. The footer includes a mention of the issue that this commit addresses, establishing a clearer link to project management.
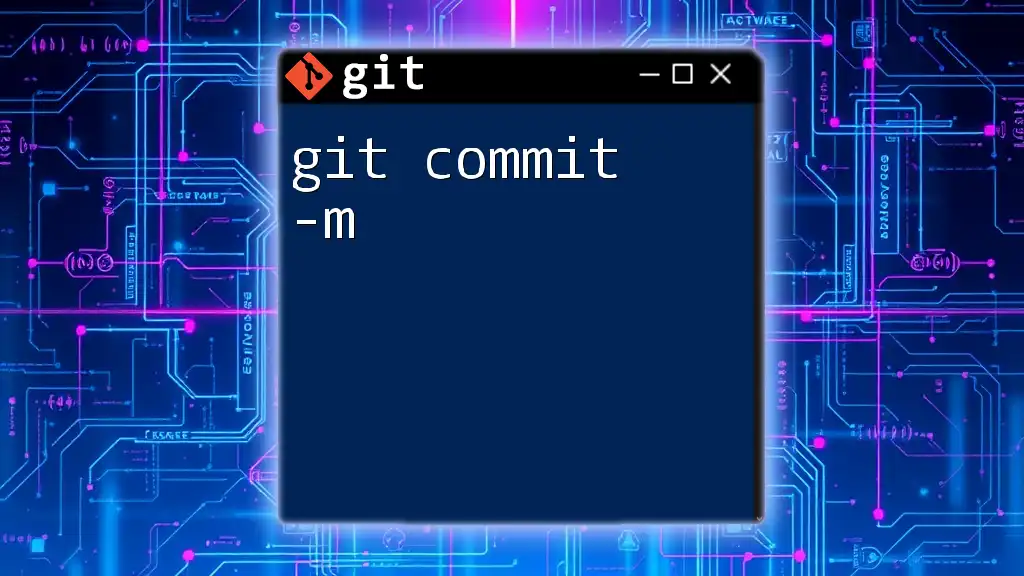
Best Practices for Writing Commit Messages
Keep it Concise
The summary line must be to the point. Aim for clarity without unnecessary words. If a change can be effectively communicated in fewer words, do it! For example, instead of writing, “Fixed the login issue that occurred when a user did not fill in the correct password,” you could phrase it as “Fix login validation error.”
Use Imperative Mood
Using the imperative mood makes your commit messages more commanding and direct. Instead of describing what you did, tell what to do. For example, use “Add user authentication” instead of “Added user authentication.” This approach aligns with conventional styles seen in many programming communities.
Include Context
In the body of the commit message, provide enough context. Explain why the change was necessary. This is particularly important for complex changes where reviewing the code alone may not be sufficient. If you identify a bug and provide a fix, you might say:
fix(authentication): correct JWT token expiration issue
This resolves a bug where the token expires prematurely, causing user sessions to expire unexpectedly. The solution extends the token expiration time to 24 hours.
Reference Issues and Pull Requests
When relevant, reference issue numbers and pull requests in the footer section of your commit message. This provides traceability and helps anyone who might be reviewing the commit understand its purpose. Here’s a useful format:
fix: address login bug in user authentication logic
Related to issue #456 and PR #78
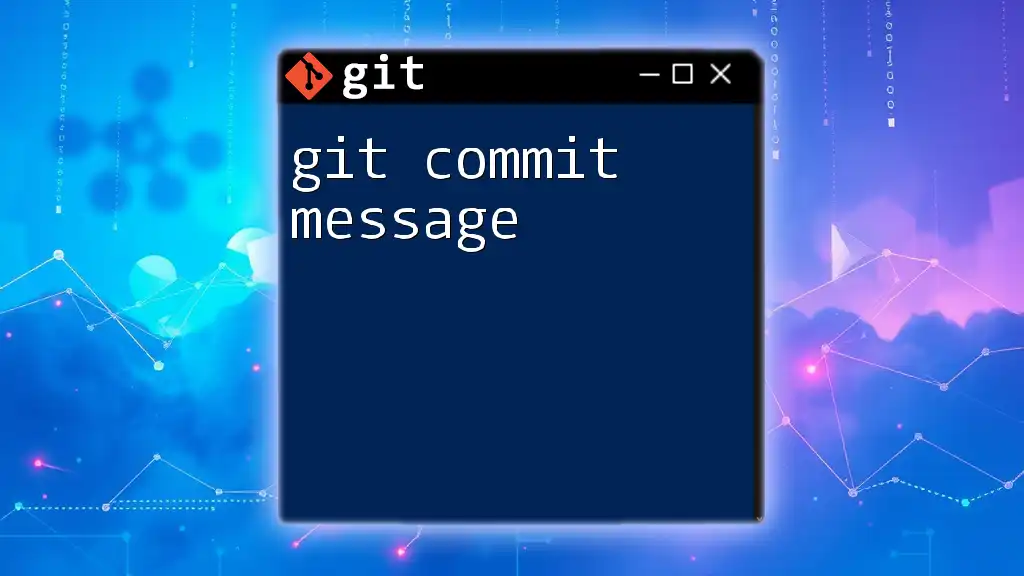
Configuring Commit Message Guidelines
Using Commit Templates
To ensure consistency in your commit messages, you can set up a commit message template. This is a pre-defined format that prompts contributors to follow a specific style when writing their commits. You can set a template by adding the following to your Git configuration:
git config --global commit.template ~/.gitmessage.txt
Here’s a sample content you might include in your `~/.gitmessage.txt` file:
# Subject: (required)
# Body: (optional)
# Footer: (optional)
When you make a commit, your template will appear, guiding you to fill in the relevant sections.
Enforcing Message Guidelines with Hooks
Git provides a powerful feature called hooks, enabling you to enforce commit message standards. For example, you can create a pre-commit hook to validate the format of the commit message. Here’s a sample script:
#!/bin/sh
if ! grep -q '^[A-Z].{1,50}$' "$1"; then
echo "Commit message must start with an uppercase letter and be less than 50 characters."
exit 1
fi
This script checks that the commit message starts with an uppercase letter and does not exceed the character limit you set, helping to standardize messaging practices across your team.
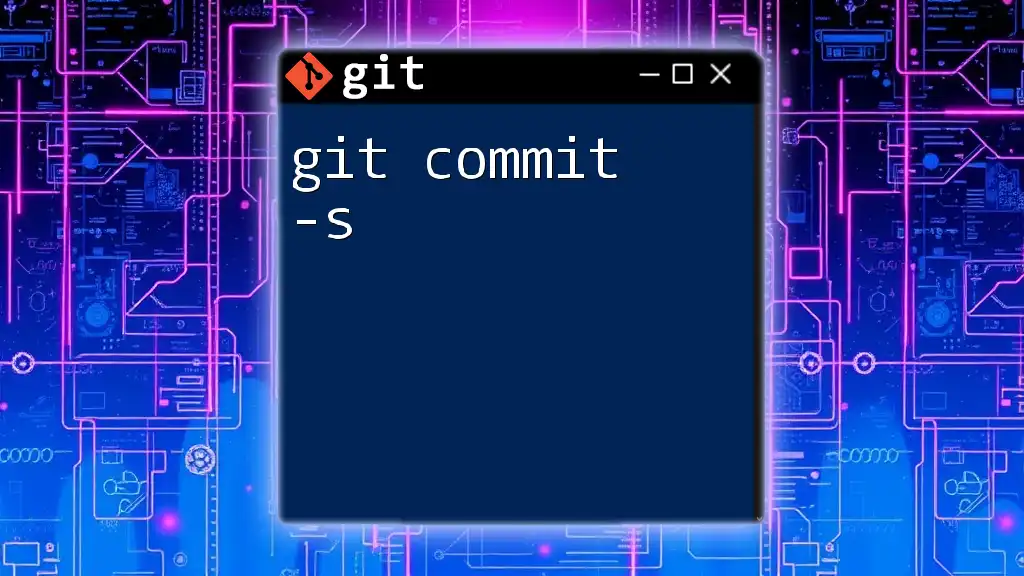
Common Mistakes to Avoid
Vague Messages
Avoid vague messages like "fix bug." Such messages provide no context and can lead to confusion. Instead, specify what bug was fixed. A better message would be:
fix: resolve null pointer exception in user profile view
Inconsistent Formatting
Maintain a consistent formatting style across your project. When multiple contributors are involved, inconsistency can lead to confusion and make it difficult to navigate the commit history. To avoid this, establish a common format and leverage tools to enforce it.
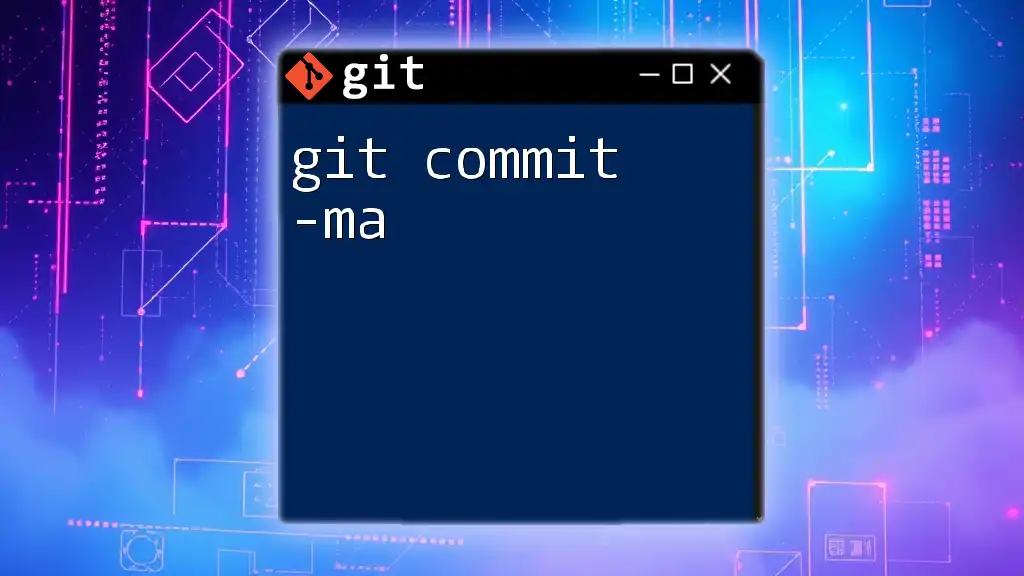
Tools and Resources for Better Commit Messages
Commitizen
Commitizen is a helpful tool that encourages structured commit messages by providing a command line interface for generating them. It promotes adherence to a conventional commit style, which can be beneficial for projects that use semantic versioning. Start by installing Commitizen:
npm install -g commitizen
Following installation, initialize Commitizen in your project:
commitizen init cz-conventional-changelog --save-dev --save-prefix='[FIX]'
Git Hooks Library
Numerous libraries are available that can help you implement Git hooks for enforcing commit message standards. Libraries like Husky streamline the process of setting up hooks directly in your Git workflows.
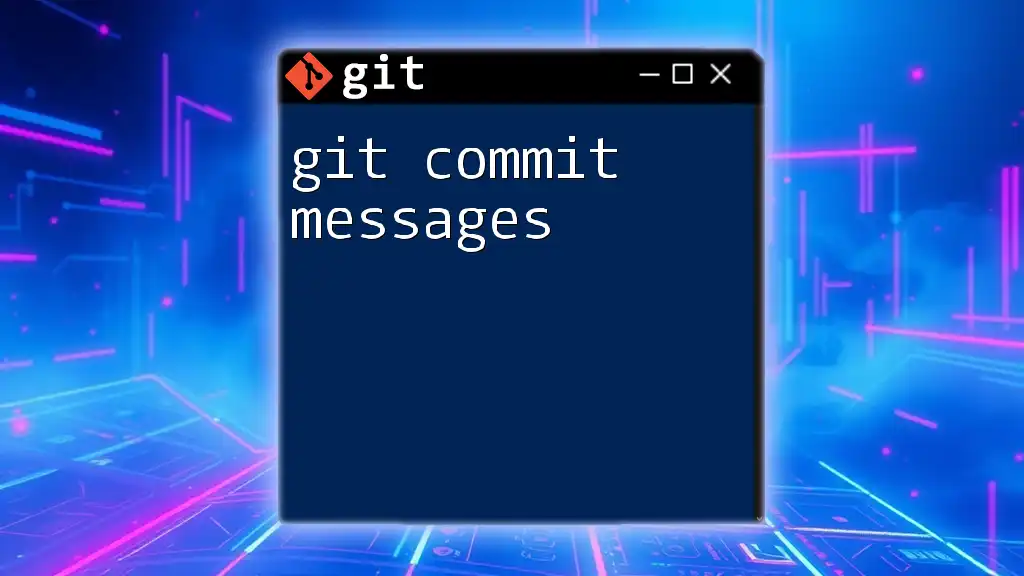
Advanced Techniques
Using Git Commit Message Guidelines in CI/CD
Integrating commit message validation into your CI/CD pipelines can enhance your project's integrity by ensuring that commit messages conform to established standards before merges occur. Tools like Husky can facilitate these validations directly in your Git workflow.
Example setting up a validation step using Husky might look like this in your `package.json`:
"husky": {
"hooks": {
"commit-msg": "commitlint --edit $1"
}
}
This example specifies that Husky should run `commitlint`, which checks the commit message structure against your defined guidelines.
Customizing Git Commit Behavior
You can customize your Git configuration to make commit behavior align more closely with your team's needs. This may include setting author information, enabling colored output for more accessible readability, or even creating aliases for frequently used commands.
For example, you can set an alias for your commit command:
git config --global alias.co '!git commit -m'
Now, you can commit changes quickly by using `git co "Your message here"`.
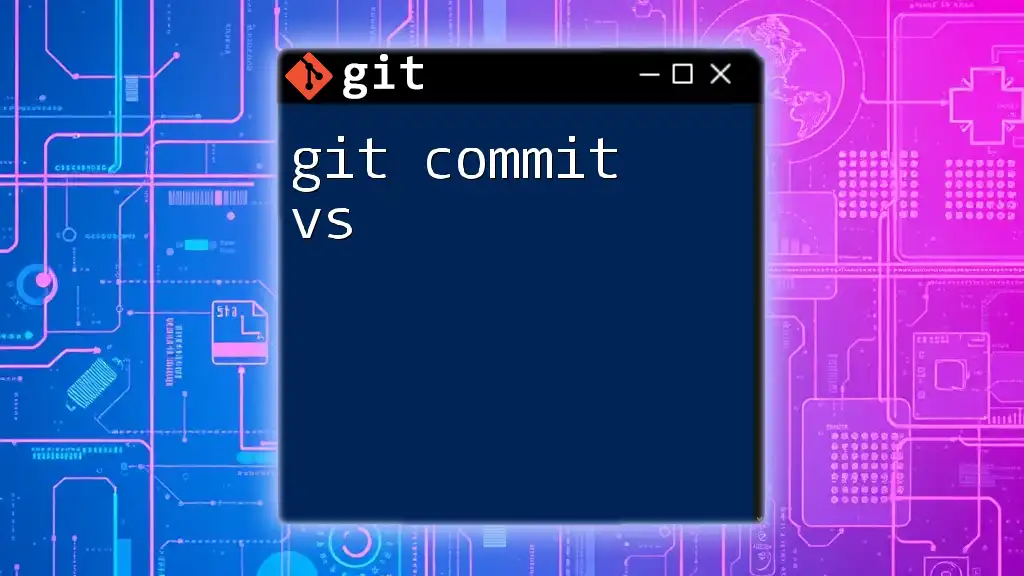
Conclusion
A disciplined approach to writing git commit-msg can significantly enhance your project's communicative capacity. Remember to keep your messages concise, informative, and centered on context. Implementing guidelines and using tools can help maintain a clean commit history, making collaboration more effective. Practice these techniques, and you'll find that not only will your commit history be easier to navigate, but the overall project collaboration will improve as well.
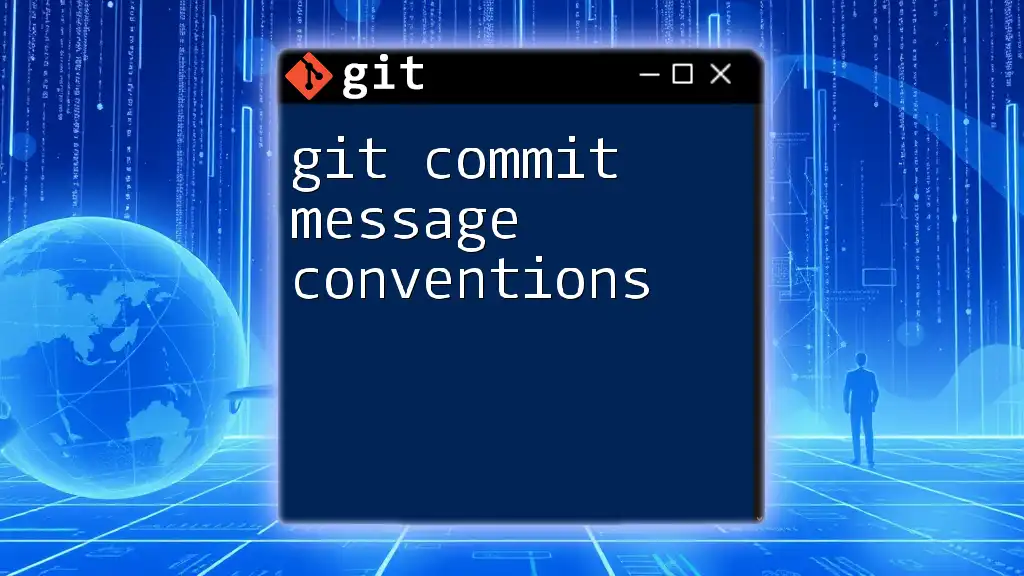
Additional Resources
- Explore the [Git documentation](https://git-scm.com/doc) for in-depth guidance.
- Check out additional [reading materials and tools](https://www.conventionalcommits.org/) recommended for enhancing your commit message writing skills.